Using the Lemlist API to Get Leads in Javascript
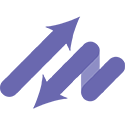
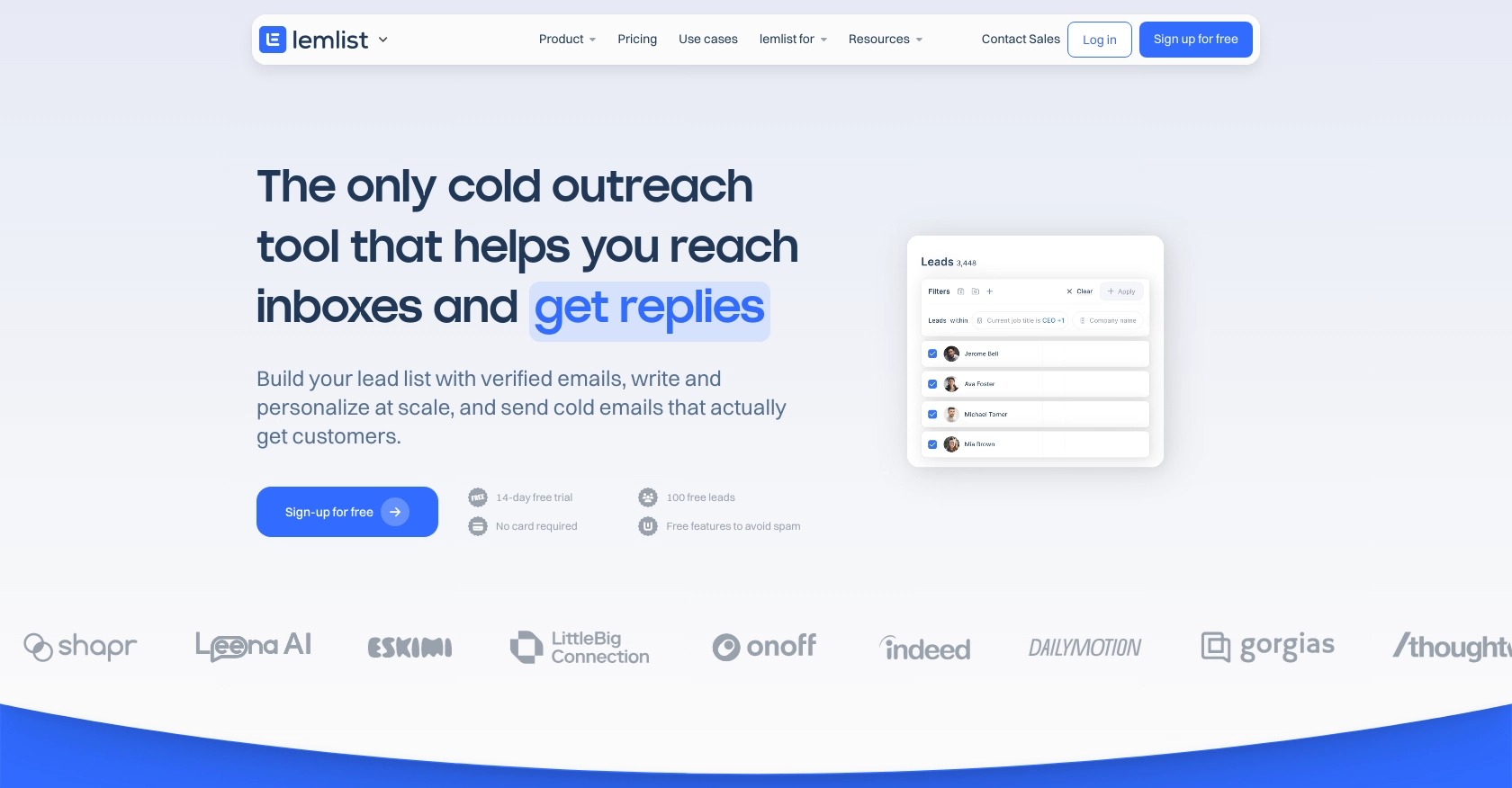
Introduction to Lemlist API
Lemlist is a powerful email outreach platform designed to help businesses personalize and automate their email campaigns. With its robust features, Lemlist enables users to create engaging email sequences, track performance metrics, and optimize their outreach strategies.
For developers, integrating with the Lemlist API offers the opportunity to streamline lead management processes. By accessing and managing leads programmatically, developers can automate tasks such as retrieving lead information and updating lead statuses. For example, a developer might use the Lemlist API to fetch leads and integrate them into a CRM system, enhancing the efficiency of sales and marketing efforts.
Setting Up Your Lemlist Account for API Access
Before you can start using the Lemlist API to manage leads, you'll need to set up your Lemlist account to obtain the necessary API key. This key will allow you to authenticate your requests and interact with the Lemlist platform programmatically.
Creating a Lemlist Account
If you don't already have a Lemlist account, follow these steps to create one:
- Visit the Lemlist website and click on the "Sign Up" button.
- Fill in the required information, such as your email address and password, and complete the registration process.
- Once your account is created, log in to access the Lemlist dashboard.
Generating Your Lemlist API Key
To interact with the Lemlist API, you'll need to generate an API key. Follow these steps to obtain your key:
- Log in to your Lemlist account and navigate to the "Settings" section.
- Look for the "API" or "Integrations" tab within the settings menu.
- Click on the option to generate a new API key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed information on setting up your Lemlist account and generating an API key, you can refer to the Lemlist API documentation.
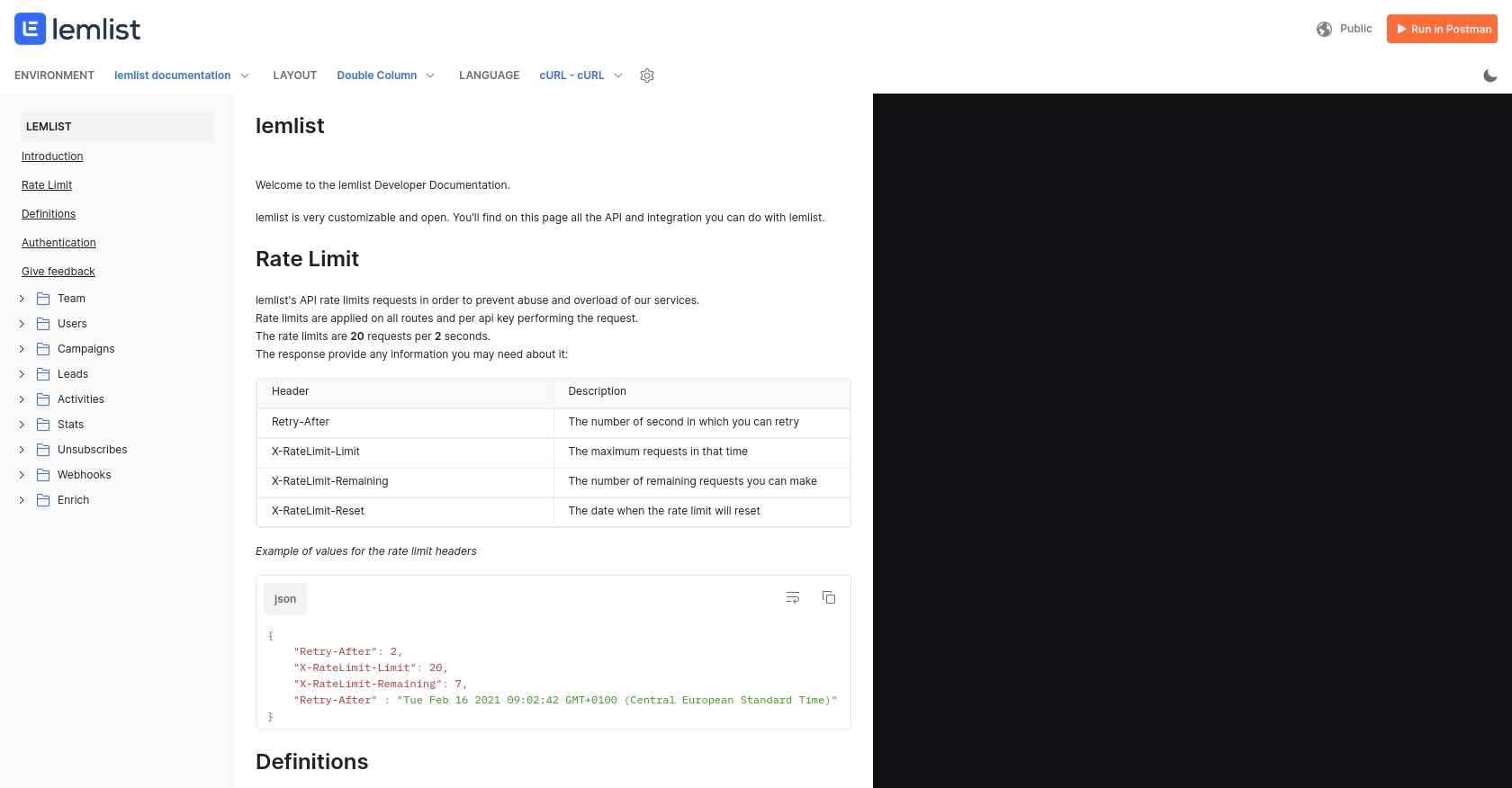
sbb-itb-96038d7
Making API Calls to Retrieve Leads Using Lemlist API in JavaScript
To interact with the Lemlist API and retrieve leads, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Lemlist API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor such as Visual Studio Code.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Leads from Lemlist API
Now, let's write the JavaScript code to retrieve leads from the Lemlist API. Create a file named getLemlistLeads.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.lemlist.com/leads';
const headers = {
'Authorization': 'Bearer YOUR_API_KEY'
};
// Function to fetch leads
async function getLeads() {
try {
const response = await axios.get(endpoint, { headers });
const leads = response.data;
console.log('Leads:', leads);
} catch (error) {
console.error('Error fetching leads:', error.response ? error.response.data : error.message);
}
}
// Call the function
getLeads();
Replace YOUR_API_KEY
with the API key you generated from your Lemlist account.
Understanding the API Response and Handling Errors
When you run the script using node getLemlistLeads.js
, you should see the list of leads printed in the console. The axios
library handles the HTTP request, and the response data is logged for your review.
In case of errors, the code captures and logs them, providing insights into what went wrong. Common issues might include invalid API keys or network errors.
Verifying API Call Success in Lemlist Dashboard
After running the script, you can verify the success of your API call by checking the leads in your Lemlist dashboard. Ensure that the data retrieved matches the leads displayed in your account.
For more detailed information on handling API responses and errors, refer to the Lemlist API documentation.
Conclusion and Best Practices for Using Lemlist API in JavaScript
Integrating the Lemlist API into your JavaScript applications can significantly enhance your lead management capabilities. By automating the retrieval and handling of leads, you can streamline your sales and marketing processes, ultimately improving efficiency and productivity.
Best Practices for Storing Lemlist API Credentials Securely
- Always store your API keys in environment variables or secure vaults rather than hardcoding them in your source code.
- Regularly rotate your API keys to minimize security risks.
- Limit the permissions of your API keys to only what is necessary for your application.
Handling Lemlist API Rate Limits and Optimizing Requests
While interacting with the Lemlist API, it's crucial to be mindful of rate limits to avoid disruptions:
- Implement exponential backoff strategies to handle rate limit errors gracefully.
- Batch requests where possible to reduce the number of API calls.
- Monitor your API usage to ensure compliance with Lemlist's rate limits.
Transforming and Standardizing Lemlist API Data
When integrating Lemlist data into other systems, consider transforming and standardizing data fields to maintain consistency:
- Map Lemlist lead fields to your CRM's data structure for seamless integration.
- Use data validation techniques to ensure data integrity across platforms.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider leveraging Endgrate to simplify the process. Endgrate allows you to build once for each use case, providing a unified API endpoint that connects to various platforms, including Lemlist. This approach saves time and resources, allowing you to focus on your core product while offering an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?