Using the Salesflare API to Get Users in PHP
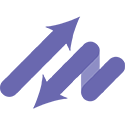
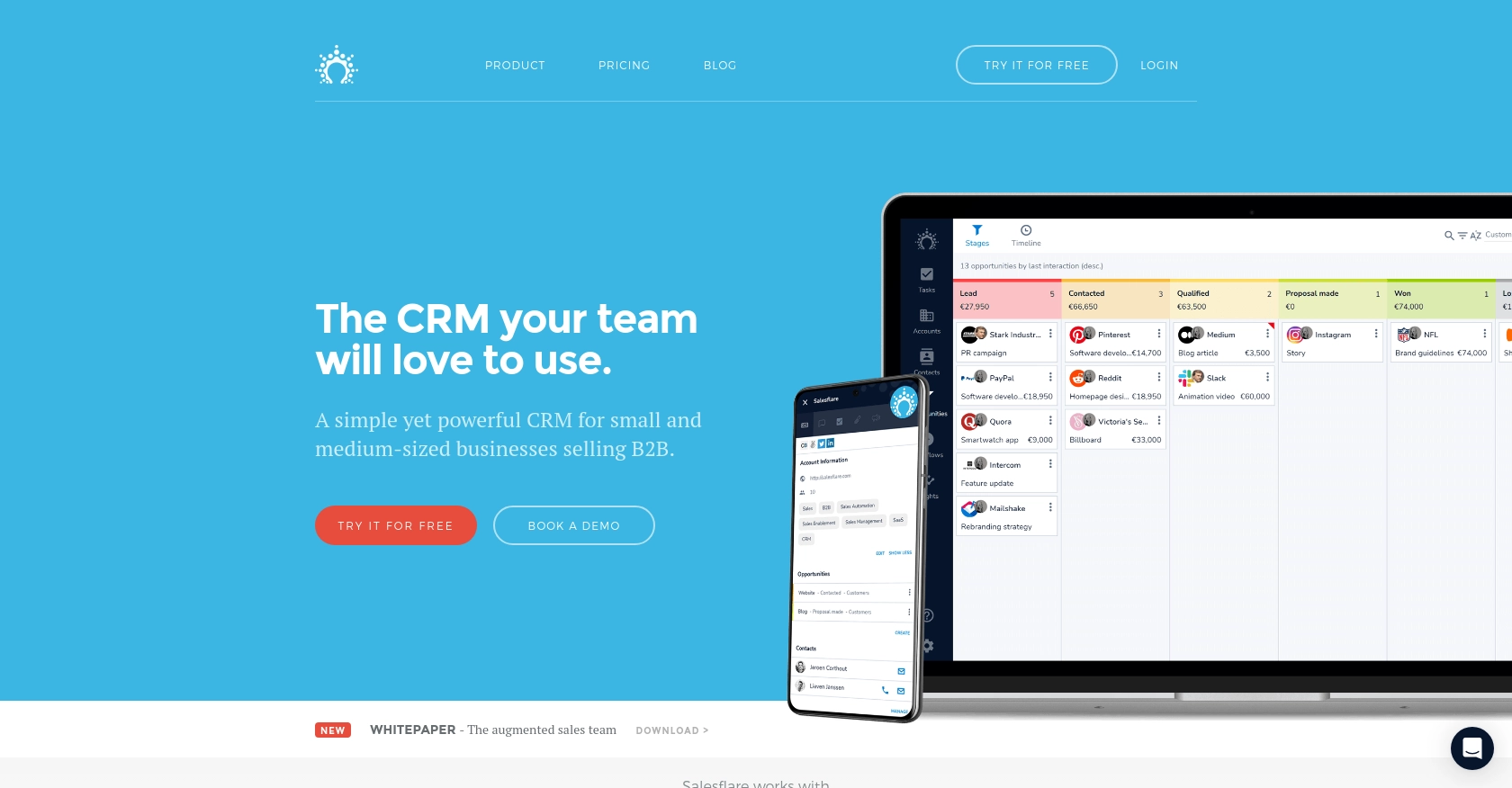
Introduction to Salesflare API
Salesflare is a powerful CRM platform designed to simplify sales processes for small and medium-sized businesses. It offers a range of features, including contact management, email tracking, and sales automation, all aimed at enhancing productivity and efficiency.
Developers might want to integrate with the Salesflare API to access and manage user data, enabling seamless synchronization of CRM information with other business applications. For example, a developer could use the Salesflare API to retrieve user details and integrate them into a custom dashboard, providing sales teams with real-time insights and analytics.
Setting Up Your Salesflare Test Account
Before you can start interacting with the Salesflare API, you need to set up a test account. Salesflare offers a straightforward process to get started with their CRM platform, allowing developers to explore its features and capabilities.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on their website. This trial will give you access to the platform's features, enabling you to test API interactions without any initial cost.
- Visit the Salesflare website and click on the "Try for Free" button.
- Follow the on-screen instructions to create your account by providing the necessary information.
- Once your account is created, you will be logged into the Salesflare dashboard.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Salesflare account and navigate to the settings section.
- Locate the "API Keys" option under the integrations or developer settings.
- Click on "Generate New API Key" and provide a name for your key to identify its purpose.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
With your API key ready, you can now proceed to make API calls to retrieve user data from Salesflare.
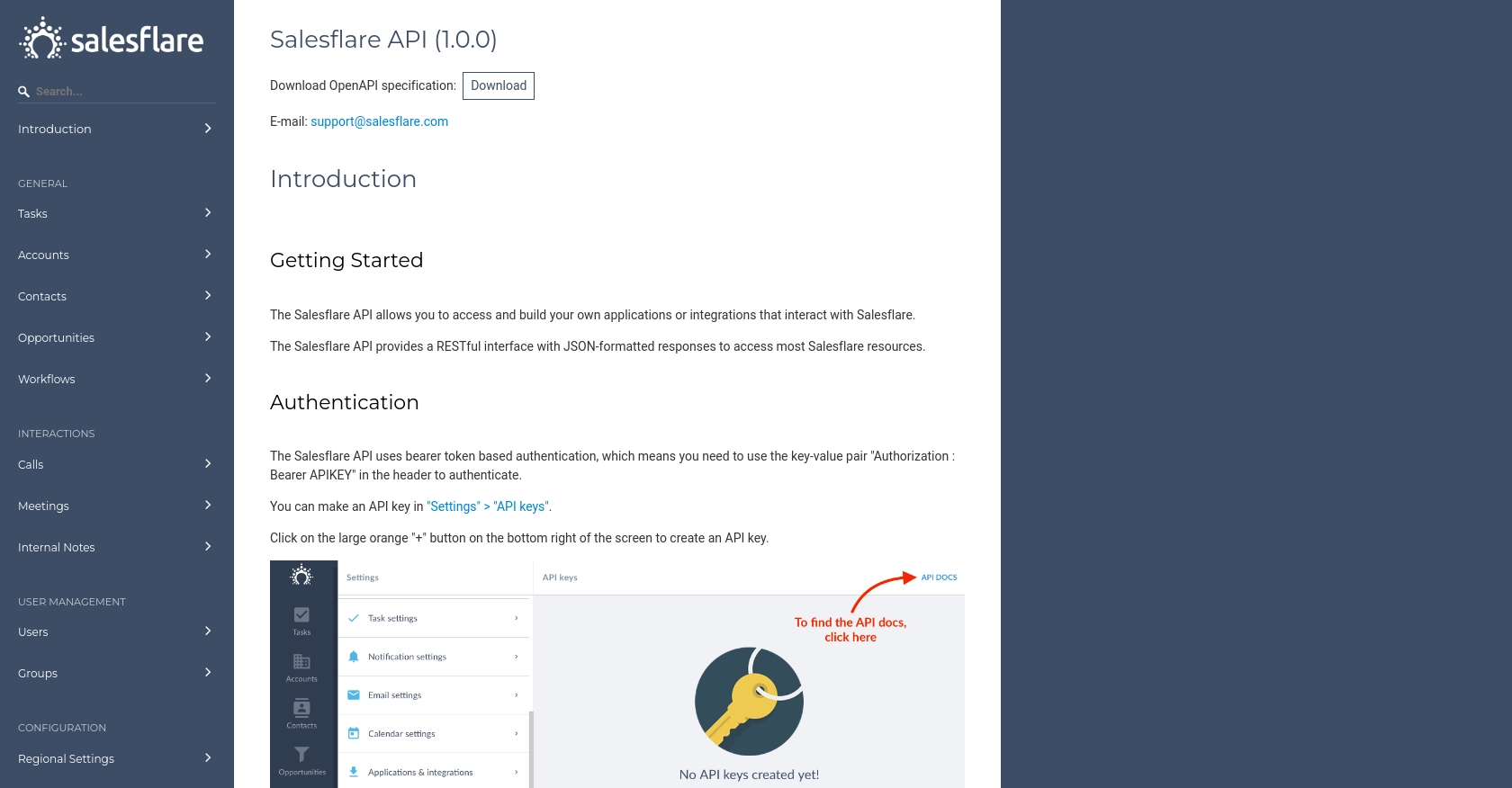
sbb-itb-96038d7
Making API Calls to Retrieve Users from Salesflare Using PHP
To interact with the Salesflare API and retrieve user data, you'll need to use PHP, a popular server-side scripting language known for its ease of use and flexibility. This section will guide you through the process of setting up your PHP environment, making the necessary API call, and handling the response effectively.
Setting Up Your PHP Environment for Salesflare API Integration
Before making API calls, ensure that your development environment is ready. You'll need:
- PHP version 7.4 or higher installed on your machine.
- The
cURL
extension enabled, as it allows you to make HTTP requests from PHP.
To verify your PHP version and installed extensions, you can run the following command in your terminal:
php -v
php -m | grep curl
Installing Dependencies for Salesflare API Calls
For this tutorial, we'll use the cURL
library to handle HTTP requests. Ensure that it's enabled in your php.ini
file. If not, you can enable it by uncommenting the following line:
extension=curl
Writing PHP Code to Retrieve Users from Salesflare
Now, let's write the PHP code to make a GET request to the Salesflare API and retrieve user data. Create a new PHP file named get_salesflare_users.php
and add the following code:
<?php
// Set the API endpoint and your API key
$endpoint = "https://api.salesflare.com/users";
$apiKey = "Your_API_Key";
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Authorization: Bearer $apiKey"
));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
foreach ($data['users'] as $user) {
echo "User ID: " . $user['id'] . " - Name: " . $user['name'] . "<br>";
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_API_Key
with the API key you generated earlier. This script initializes a cURL session, sets the necessary headers, and executes a GET request to the Salesflare API endpoint. It then parses the JSON response and displays user information.
Running the PHP Script and Verifying the Output
To run the script, execute the following command in your terminal:
php get_salesflare_users.php
You should see a list of users retrieved from your Salesflare account. If there are any errors, ensure that your API key is correct and that your cURL extension is properly configured.
Handling Errors and Troubleshooting Salesflare API Requests
When working with APIs, it's crucial to handle errors gracefully. The Salesflare API may return various HTTP status codes to indicate success or failure. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Ensure you check the response status code and handle errors appropriately in your application logic.
Conclusion and Best Practices for Using Salesflare API in PHP
Integrating with the Salesflare API using PHP can significantly enhance your CRM capabilities by allowing seamless access to user data. By following the steps outlined in this guide, you can efficiently retrieve and manage user information, enabling better synchronization with other business applications.
Best Practices for Secure and Efficient API Integration with Salesflare
- Secure API Key Storage: Always store your API keys securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Salesflare API. Implement retry logic and exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from Salesflare is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Streamline Your Integration Process with Endgrate
While integrating with individual APIs like Salesflare can be rewarding, it can also be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and scaling your capabilities effortlessly. Explore the possibilities with Endgrate and enhance your business's integration strategy today.
Read More
Ready to get started?