Using the Linear API to Create Or Update Issues in PHP
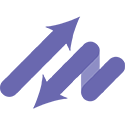
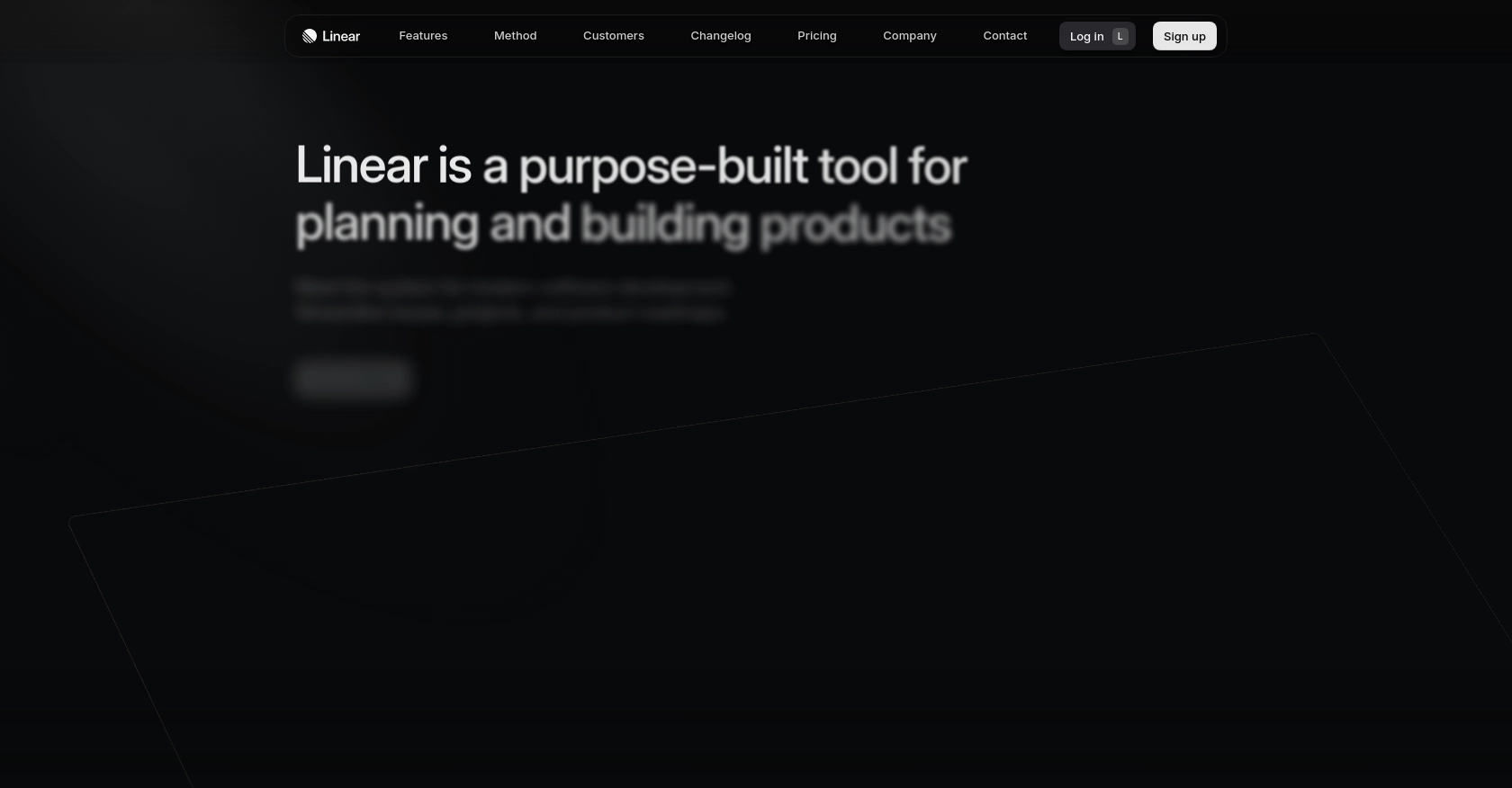
Introduction to Linear API Integration
Linear is a powerful project management tool designed to streamline issue tracking and team collaboration for software development teams. Its intuitive interface and robust features make it a popular choice for developers looking to enhance productivity and manage tasks efficiently.
Integrating with Linear's API allows developers to automate and manage issues programmatically, providing seamless interaction with project data. For example, a developer might want to create or update issues in Linear using PHP to automate task assignments or synchronize project updates across different platforms.
Setting Up a Linear Test Account and OAuth Application
Before you can start creating or updating issues in Linear using PHP, you need to set up a Linear account and configure an OAuth application. This setup will allow you to authenticate API requests securely.
Creating a Linear Account
If you don't already have a Linear account, you can sign up for a free trial on the Linear website. Follow the instructions to create your account and log in to the Linear dashboard.
Configuring an OAuth Application in Linear
To interact with the Linear API, you'll need to create an OAuth application. This will provide you with the necessary credentials to authenticate your API requests.
- Navigate to the Linear dashboard and click on your profile icon in the top right corner.
- Select Settings from the dropdown menu.
- Under the Integrations section, click on OAuth Applications.
- Click on Create OAuth Application and fill in the required details, such as the application name and description.
- Configure the redirect callback URLs to match your application's URL.
Obtaining Client ID and Client Secret
Once your OAuth application is created, you will receive a Client ID and Client Secret. These credentials are essential for the OAuth authentication process.
Authorizing Users with OAuth 2.0
To authorize users, redirect them to the Linear authorization URL with the appropriate parameters:
GET https://linear.app/oauth/authorize?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URL&scope=read,write
Replace YOUR_CLIENT_ID
and YOUR_REDIRECT_URL
with your application's client ID and redirect URI.
Exchanging Authorization Code for Access Token
After the user authorizes your application, they will be redirected back to your application with an authorization code. Exchange this code for an access token using the following request:
POST https://api.linear.app/oauth/token
Content-Type: application/x-www-form-urlencoded
client_id=YOUR_CLIENT_ID
&client_secret=YOUR_CLIENT_SECRET
&code=AUTHORIZATION_CODE
&redirect_uri=YOUR_REDIRECT_URL
&grant_type=authorization_code
Upon successful exchange, you will receive an access token, which you can use to authenticate your API requests.
For more detailed information on OAuth setup, refer to the Linear OAuth documentation.
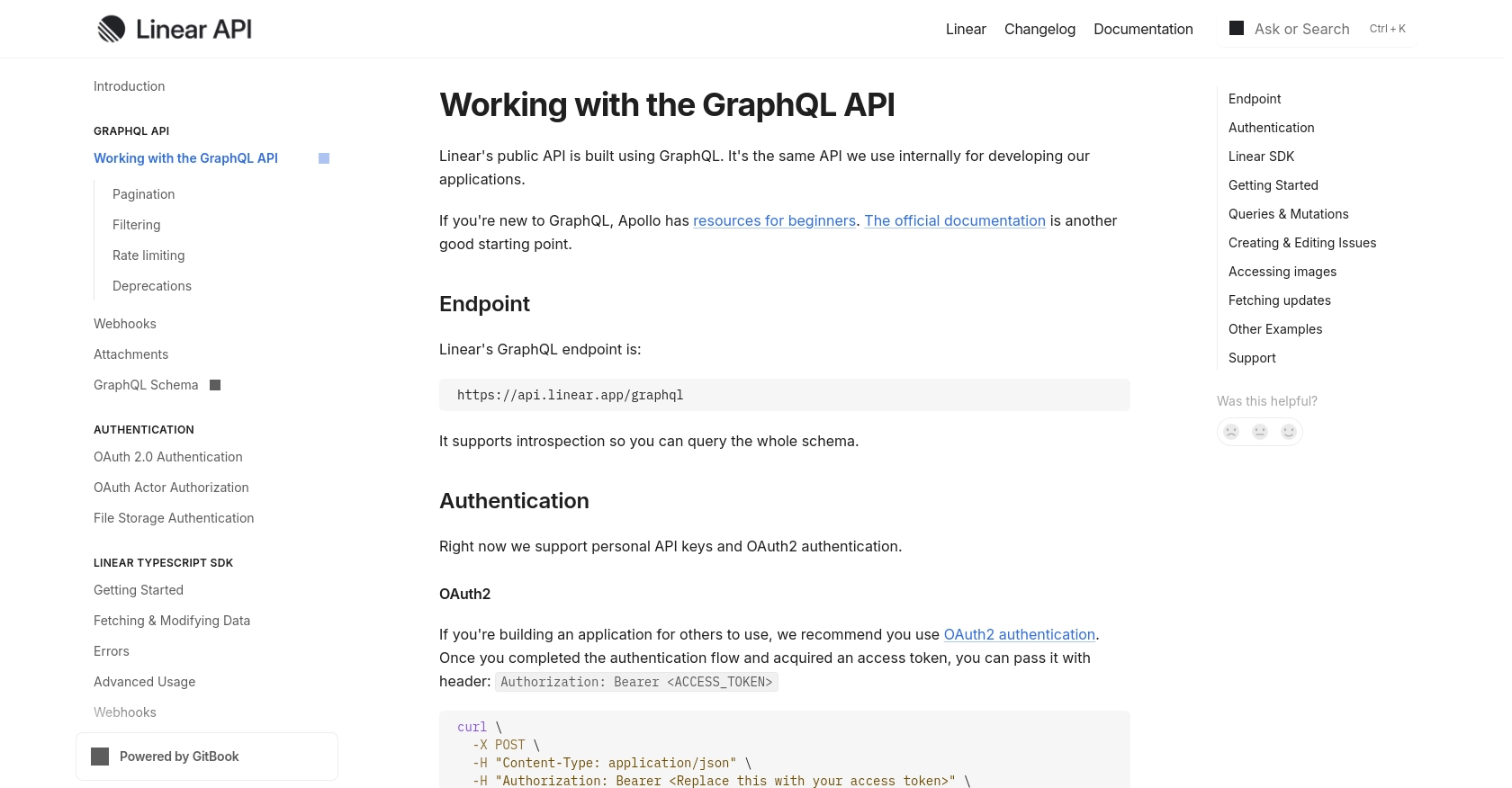
sbb-itb-96038d7
Making API Calls to Linear for Issue Management in PHP
To interact with the Linear API using PHP, you need to set up your environment and write the necessary code to create or update issues. This section will guide you through the process, including setting up PHP, installing dependencies, and making API calls.
Setting Up PHP Environment for Linear API Integration
Ensure you have PHP installed on your machine. You can download the latest version from the official PHP website. Additionally, you'll need Composer, a dependency manager for PHP, which you can install from Composer's website.
Installing Required PHP Dependencies
For making HTTP requests to the Linear API, you'll need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Creating and Updating Issues in Linear Using PHP
Once your environment is set up, you can start writing the PHP code to interact with the Linear API. Below is an example of how to create or update issues using the Linear API.
Example Code to Create an Issue in Linear
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$response = $client->post('https://api.linear.app/graphql', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
],
'json' => [
'query' => 'mutation {
issueCreate(input: {
title: "New Issue Title",
description: "Detailed description of the issue",
teamId: "YOUR_TEAM_ID"
}) {
success
issue {
id
title
}
}
}'
]
]);
$data = json_decode($response->getBody(), true);
if ($data['data']['issueCreate']['success']) {
echo "Issue Created Successfully: " . $data['data']['issueCreate']['issue']['title'];
} else {
echo "Failed to Create Issue";
}
Example Code to Update an Issue in Linear
$response = $client->post('https://api.linear.app/graphql', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
],
'json' => [
'query' => 'mutation {
issueUpdate(id: "ISSUE_ID", input: {
title: "Updated Issue Title",
description: "Updated description of the issue"
}) {
success
issue {
id
title
}
}
}'
]
]);
$data = json_decode($response->getBody(), true);
if ($data['data']['issueUpdate']['success']) {
echo "Issue Updated Successfully: " . $data['data']['issueUpdate']['issue']['title'];
} else {
echo "Failed to Update Issue";
}
Verifying API Call Success and Handling Errors
After executing the API call, check the response to verify if the operation was successful. The response will include a success
field indicating the result. If the operation fails, inspect the error messages returned by the API.
For more information on handling errors, refer to the Linear API documentation.
Handling Rate Limiting in Linear API
Linear API has rate limits to prevent abuse. If you exceed these limits, you'll receive a rate limit error. Implement retry logic with exponential backoff to handle such scenarios gracefully. For detailed rate limit information, consult the Linear API documentation.
Conclusion and Best Practices for Linear API Integration in PHP
Integrating with the Linear API using PHP allows developers to efficiently manage issues and streamline project workflows. By automating tasks such as creating and updating issues, teams can enhance productivity and maintain consistency across platforms.
Best Practices for Secure and Efficient Linear API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as the client ID, client secret, and access tokens, securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Implement retry logic with exponential backoff to gracefully handle rate limit errors. This ensures your application remains responsive even when API limits are reached.
- Data Standardization: Ensure that data fields are standardized across different systems to maintain consistency and avoid data discrepancies.
Enhancing Integration Capabilities with Endgrate
For developers looking to simplify and expand their integration capabilities, Endgrate offers a unified API endpoint that connects to multiple platforms, including Linear. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
Ready to get started?