Using the Microsoft Dynamics 365 API to Create or Update Custom Objects in Python
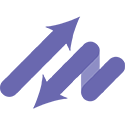
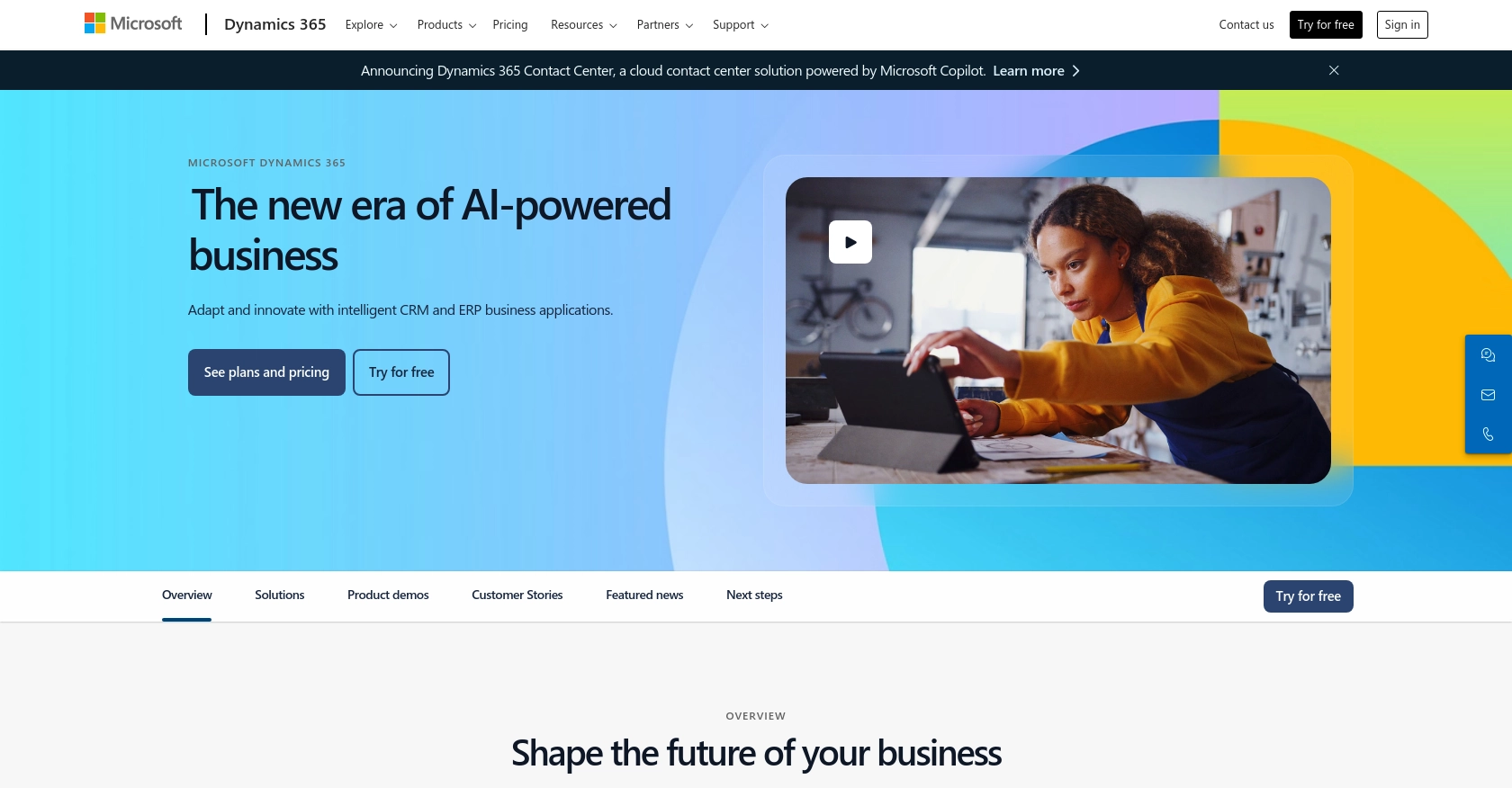
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a powerful suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a comprehensive set of tools for sales, customer service, finance, operations, and more, making it a popular choice for businesses looking to enhance their operational efficiency.
Integrating with the Microsoft Dynamics 365 API allows developers to create or update custom objects, enabling seamless data management and automation within the platform. For example, a developer might use the API to automatically update customer records based on interactions from other systems, ensuring that all data remains consistent and up-to-date across the organization.
Setting Up a Microsoft Dynamics 365 Test or Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your integrations without affecting live data.
Creating a Microsoft Dynamics 365 Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Microsoft Dynamics 365 website and sign up for a free trial if you don't already have an account.
- Once logged in, navigate to the admin center and select the option to create a new sandbox environment.
- Follow the prompts to configure your sandbox environment, including setting up any necessary security roles and permissions.
Registering an Application for OAuth Authentication
Microsoft Dynamics 365 uses OAuth 2.0 for authentication. To interact with the API, you need to register an application in your Microsoft Entra ID tenant. Here's how:
- Go to the Azure portal and sign in with your Microsoft account.
- Navigate to "Azure Active Directory" and select "App registrations."
- Click on "New registration" and fill in the required details, such as the application name and redirect URI.
- After registration, note down the Application (client) ID and Directory (tenant) ID.
- Under "Certificates & secrets," create a new client secret and save it securely. This will be used for authentication.
For detailed instructions, refer to the Microsoft documentation on OAuth authentication.
Configuring API Permissions
To allow your application to access Microsoft Dynamics 365 data, you need to configure API permissions:
- In the Azure portal, navigate to your registered application and select "API permissions."
- Click on "Add a permission" and choose "Dynamics CRM."
- Select the permissions required for your application, such as "user_impersonation" for public clients or ".default" for confidential clients.
- Grant admin consent for the selected permissions.
With your sandbox account and application registration complete, you're now ready to start making API calls to Microsoft Dynamics 365.
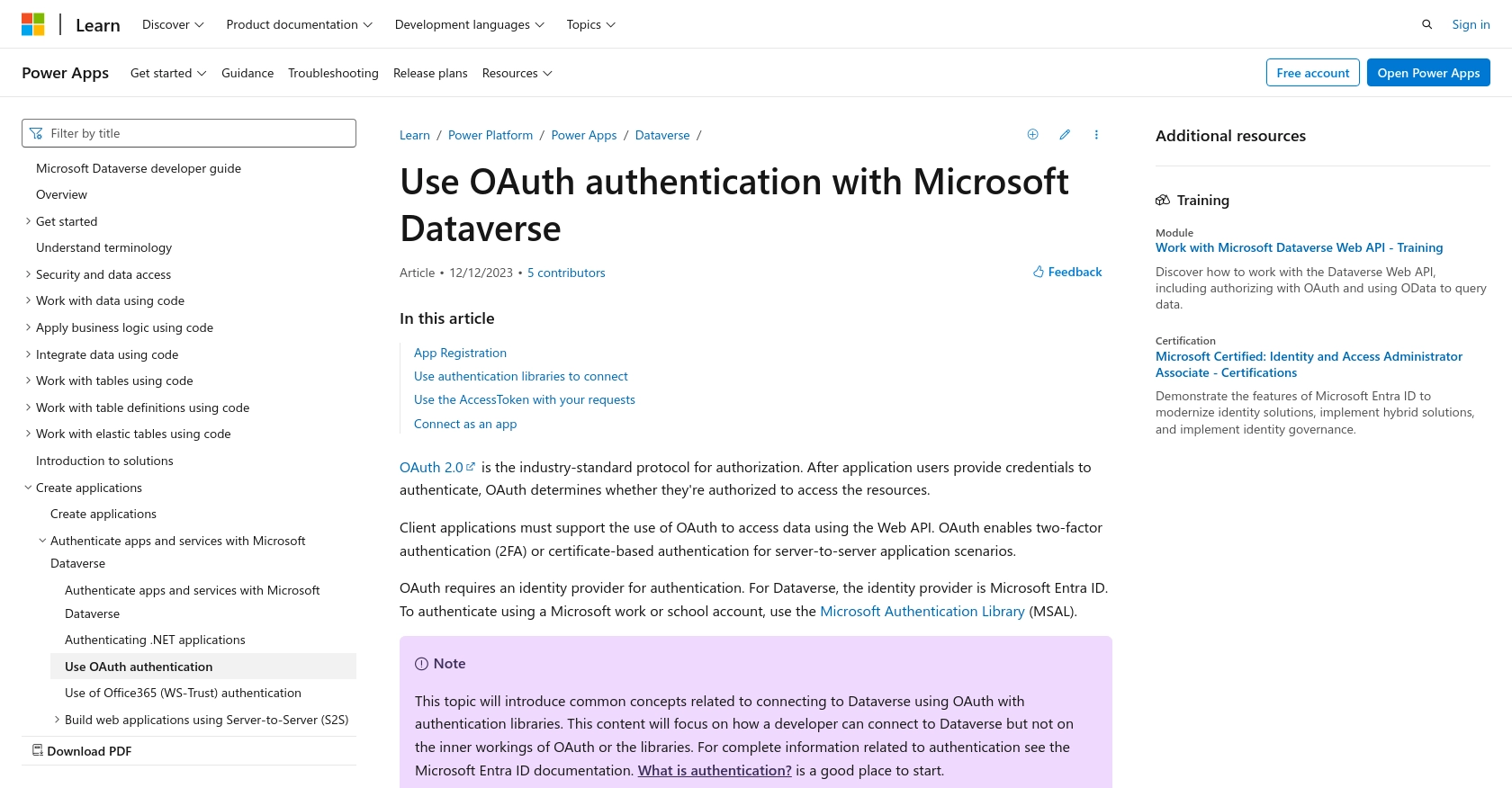
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Using Python
To interact with the Microsoft Dynamics 365 API, you'll need to use Python to create or update custom objects. This section will guide you through the process of setting up your Python environment, making API requests, and handling responses effectively.
Setting Up Your Python Environment for Microsoft Dynamics 365 API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Next, install the required libraries using pip:
pip install requests msal
The requests
library will help you make HTTP requests, while msal
is used for OAuth authentication.
Authenticating with Microsoft Dynamics 365 API Using OAuth
To authenticate with the Microsoft Dynamics 365 API, you'll use the Microsoft Authentication Library (MSAL) to acquire an access token. Here's how you can set it up:
import msal
client_id = 'your_client_id'
client_secret = 'your_client_secret'
tenant_id = 'your_tenant_id'
authority = f'https://login.microsoftonline.com/{tenant_id}'
scope = ['https://yourorg.crm.dynamics.com/.default']
app = msal.ConfidentialClientApplication(client_id, authority=authority, client_credential=client_secret)
token_response = app.acquire_token_for_client(scopes=scope)
if 'access_token' in token_response:
access_token = token_response['access_token']
else:
raise Exception("Authentication failed.")
Replace your_client_id
, your_client_secret
, and your_tenant_id
with your actual credentials. This code will authenticate your application and provide an access token for API requests.
Creating or Updating Custom Objects in Microsoft Dynamics 365
Once authenticated, you can make API calls to create or update custom objects. Here's an example of how to create a custom object:
import requests
url = 'https://yourorg.crm.dynamics.com/api/data/v9.2/entities'
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json',
'OData-MaxVersion': '4.0',
'OData-Version': '4.0'
}
data = {
"name": "New Custom Object",
"description": "Description of the custom object"
}
response = requests.post(url, headers=headers, json=data)
if response.status_code == 201:
print("Custom object created successfully.")
else:
print(f"Failed to create custom object: {response.status_code} - {response.text}")
Replace yourorg
with your organization's domain. This code sends a POST request to create a new custom object. If successful, it prints a confirmation message.
Handling API Responses and Errors
It's crucial to handle API responses and potential errors gracefully. Check the status code of the response to determine success or failure. For more information on error codes, refer to the Microsoft Dynamics 365 API documentation.
In case of errors, log the response details for troubleshooting and ensure your application can handle retries or alternative actions if necessary.
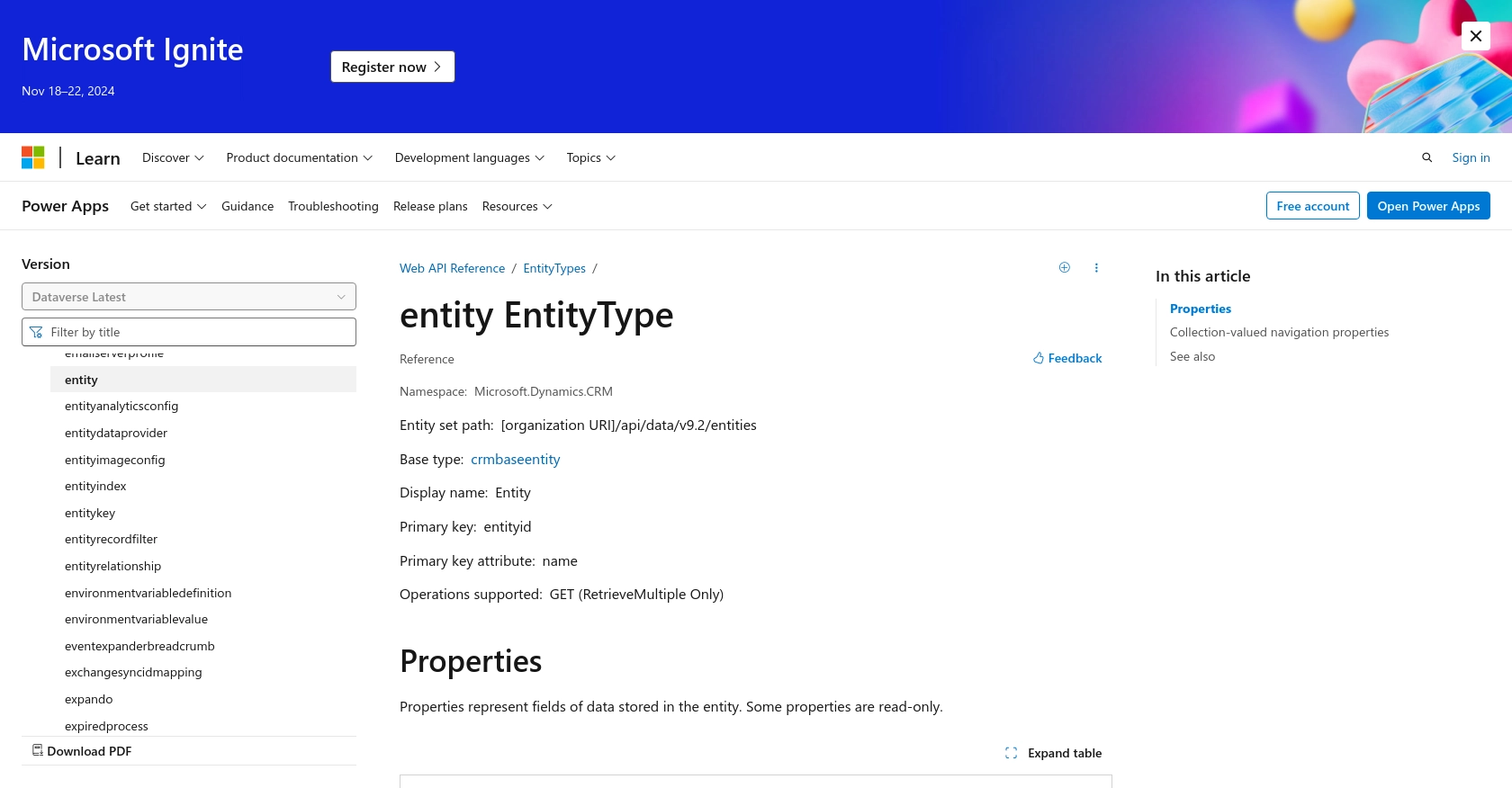
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API using Python allows developers to efficiently manage and automate data processes within the Dynamics 365 ecosystem. By following the steps outlined in this guide, you can create or update custom objects seamlessly, ensuring your business operations remain streamlined and effective.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store client IDs, secrets, and tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully.
- Standardize Data Fields: Ensure data consistency by standardizing fields across different systems. This helps in maintaining data integrity and simplifies integration processes.
- Monitor and Log API Calls: Implement logging to monitor API interactions. This aids in troubleshooting and provides insights into integration performance.
Enhance Your Integration Strategy with Endgrate
While integrating with Microsoft Dynamics 365 can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/entity?view=dataverse-latest
Ready to get started?