Using the Microsoft Dynamics 365 API to Get Contacts (with PHP examples)
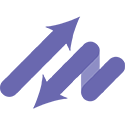
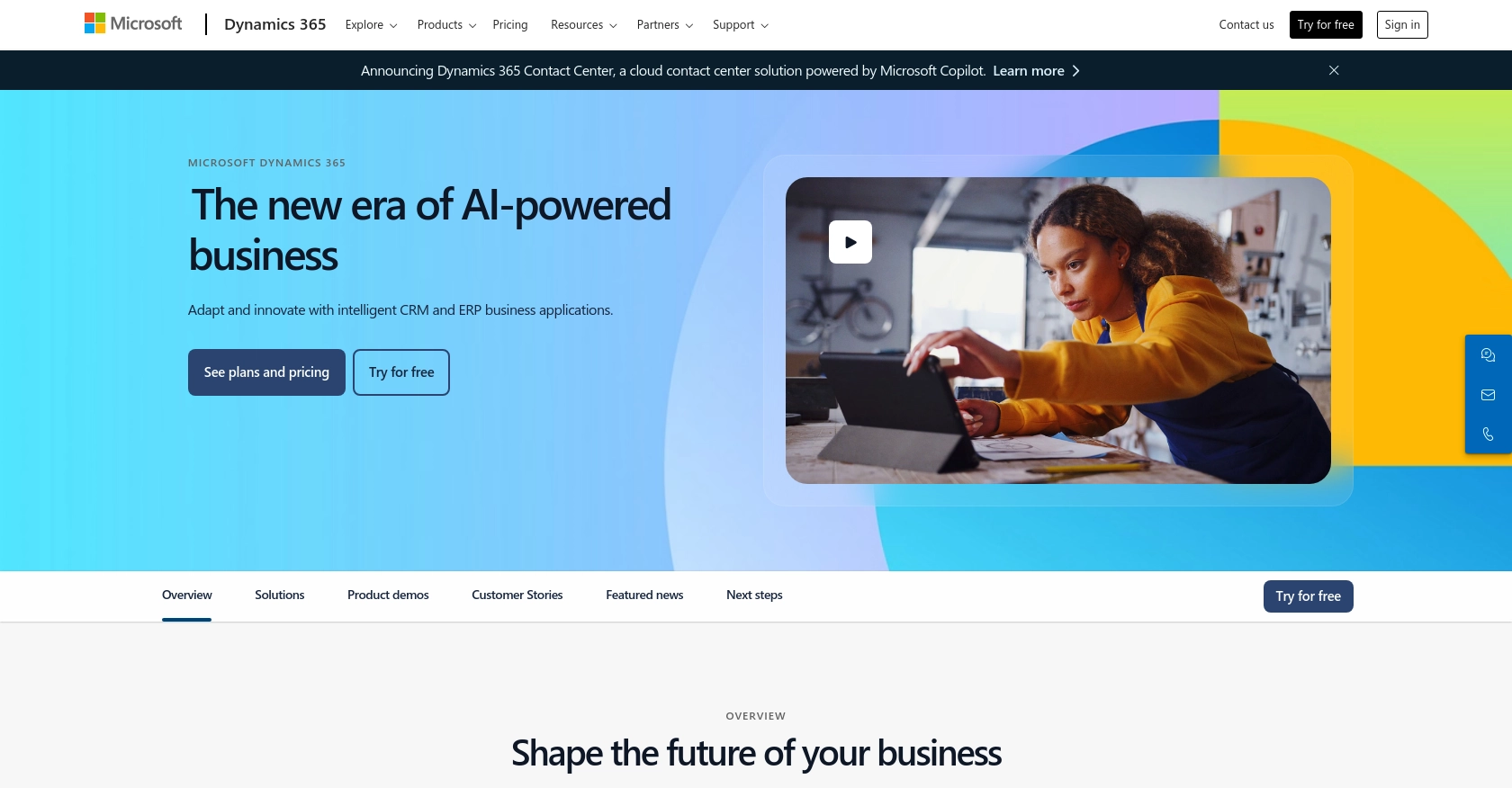
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a wide range of tools for sales, customer service, finance, operations, and more, making it a popular choice for businesses looking to enhance their operational efficiency.
Developers often integrate with Microsoft Dynamics 365 to access and manage customer data, such as contacts, to improve business processes and customer interactions. For example, a developer might use the Microsoft Dynamics 365 API to retrieve contact information and integrate it with other business systems, enabling seamless data flow and enhanced customer insights.
Setting Up Your Microsoft Dynamics 365 Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Microsoft Dynamics 365 offers a trial version that developers can use to explore its features and capabilities.
Step-by-Step Guide to Creating a Microsoft Dynamics 365 Sandbox Account
- Sign Up for a Free Trial: Visit the Microsoft Dynamics 365 Free Trial page and sign up for a trial account. Follow the on-screen instructions to complete the registration process.
- Access the Admin Center: Once your account is set up, log in to the Microsoft Dynamics 365 Admin Center. Here, you can manage your environments and create a sandbox instance.
- Create a Sandbox Environment: In the Admin Center, navigate to the "Environments" section and select "New" to create a new environment. Choose "Sandbox" as the type and configure the necessary settings.
Configuring OAuth Authentication for Microsoft Dynamics 365 API
Microsoft Dynamics 365 uses OAuth 2.0 for authentication, which requires you to register an application and obtain the necessary credentials.
- Register Your Application: Go to the Azure Portal and navigate to "Azure Active Directory" > "App registrations". Click "New registration" to create a new application.
-
Configure Redirect URI: During registration, set the redirect URI to a valid endpoint where the authentication response can be sent. This is typically a URL like
https://localhost
for local development. - Obtain Client ID and Client Secret: After registration, note down the Application (client) ID. Navigate to "Certificates & secrets" to generate a new client secret. Store these credentials securely as they will be used for API authentication.
- Set API Permissions: Under "API permissions", add the necessary permissions for accessing Microsoft Dynamics 365 data. Ensure you have the "Access Dynamics 365 as organization users" permission.
For more detailed instructions on OAuth authentication, refer to the official documentation: Use OAuth authentication with Microsoft Dataverse.
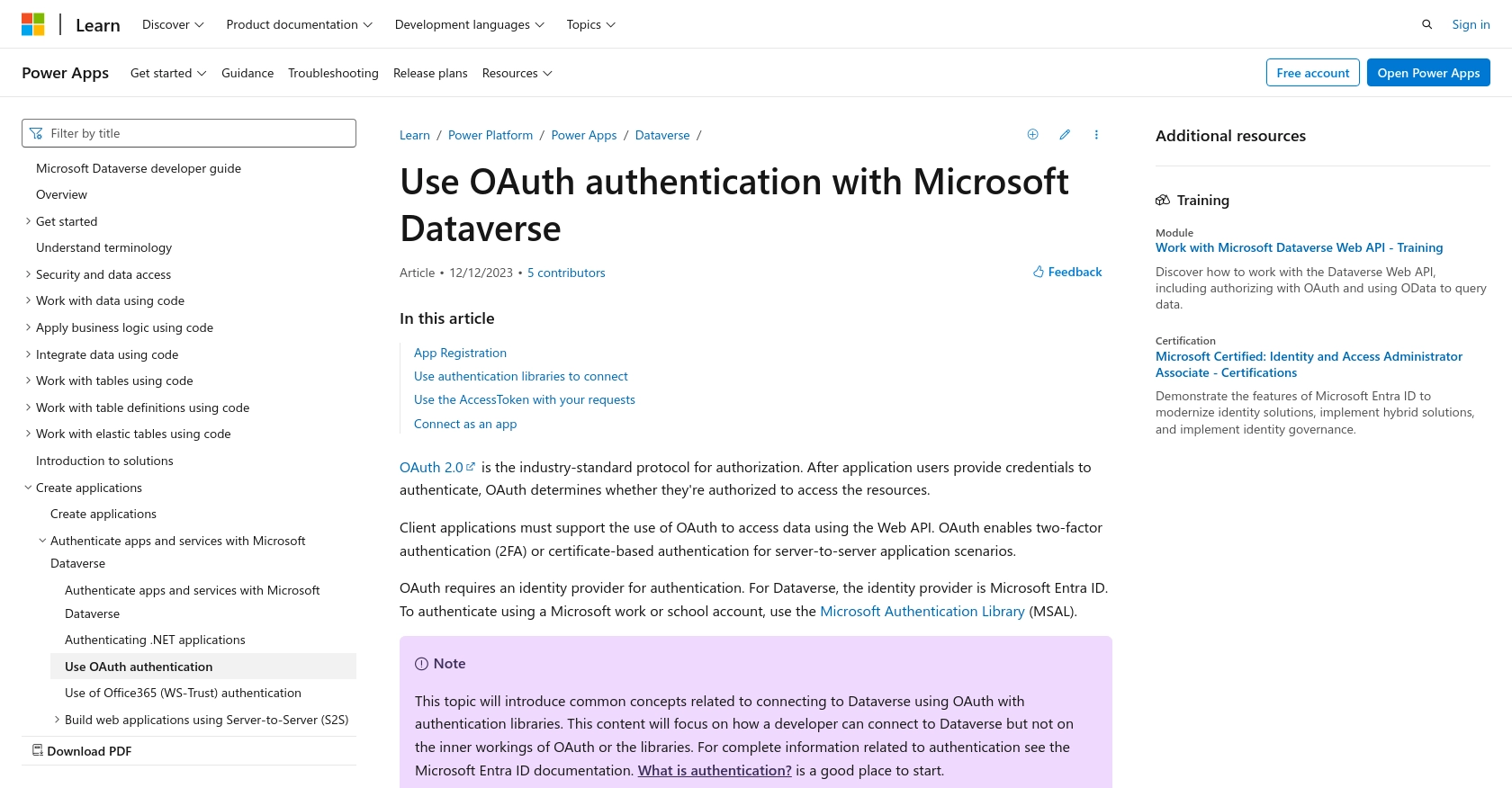
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 to Retrieve Contacts Using PHP
To interact with the Microsoft Dynamics 365 API and retrieve contact data, you'll need to set up your PHP environment and make authenticated requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses.
Setting Up Your PHP Environment for Microsoft Dynamics 365 API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the following dependencies:
- cURL: A PHP extension for making HTTP requests.
- JSON: PHP's built-in JSON extension for handling JSON data.
Ensure these extensions are enabled in your php.ini
file.
Writing PHP Code to Authenticate and Retrieve Contacts from Microsoft Dynamics 365
Once your environment is set up, you can write the PHP code to authenticate and retrieve contacts from Microsoft Dynamics 365. Follow these steps:
// Step 1: Set up your OAuth credentials
$clientId = 'your_client_id';
$clientSecret = 'your_client_secret';
$tenantId = 'your_tenant_id';
$resource = 'https://yourorg.crm.dynamics.com';
// Step 2: Obtain an access token
$tokenUrl = "https://login.microsoftonline.com/$tenantId/oauth2/token";
$tokenData = [
'grant_type' => 'client_credentials',
'client_id' => $clientId,
'client_secret' => $clientSecret,
'resource' => $resource
];
$ch = curl_init($tokenUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($tokenData));
$response = curl_exec($ch);
curl_close($ch);
$tokenResponse = json_decode($response, true);
$accessToken = $tokenResponse['access_token'];
// Step 3: Make the API call to retrieve contacts
$apiUrl = "$resource/api/data/v9.2/contacts";
$headers = [
"Authorization: Bearer $accessToken",
"OData-MaxVersion: 4.0",
"OData-Version: 4.0",
"Accept: application/json"
];
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec($ch);
curl_close($ch);
$contacts = json_decode($response, true);
// Step 4: Display the retrieved contacts
foreach ($contacts['value'] as $contact) {
echo "Contact Name: " . $contact['fullname'] . "\n";
}
Replace your_client_id
, your_client_secret
, and your_tenant_id
with your actual OAuth credentials. This script authenticates with Microsoft Dynamics 365 using OAuth 2.0, retrieves contacts, and displays their full names.
Verifying Successful API Requests and Handling Errors
After running the script, verify the contacts are retrieved successfully by checking the output. If the request fails, handle errors by checking the HTTP status code and response message. Common error codes include:
- 400 Bad Request: The request was invalid. Check your query parameters.
- 401 Unauthorized: Authentication failed. Verify your credentials.
- 403 Forbidden: You do not have permission to access the resource.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
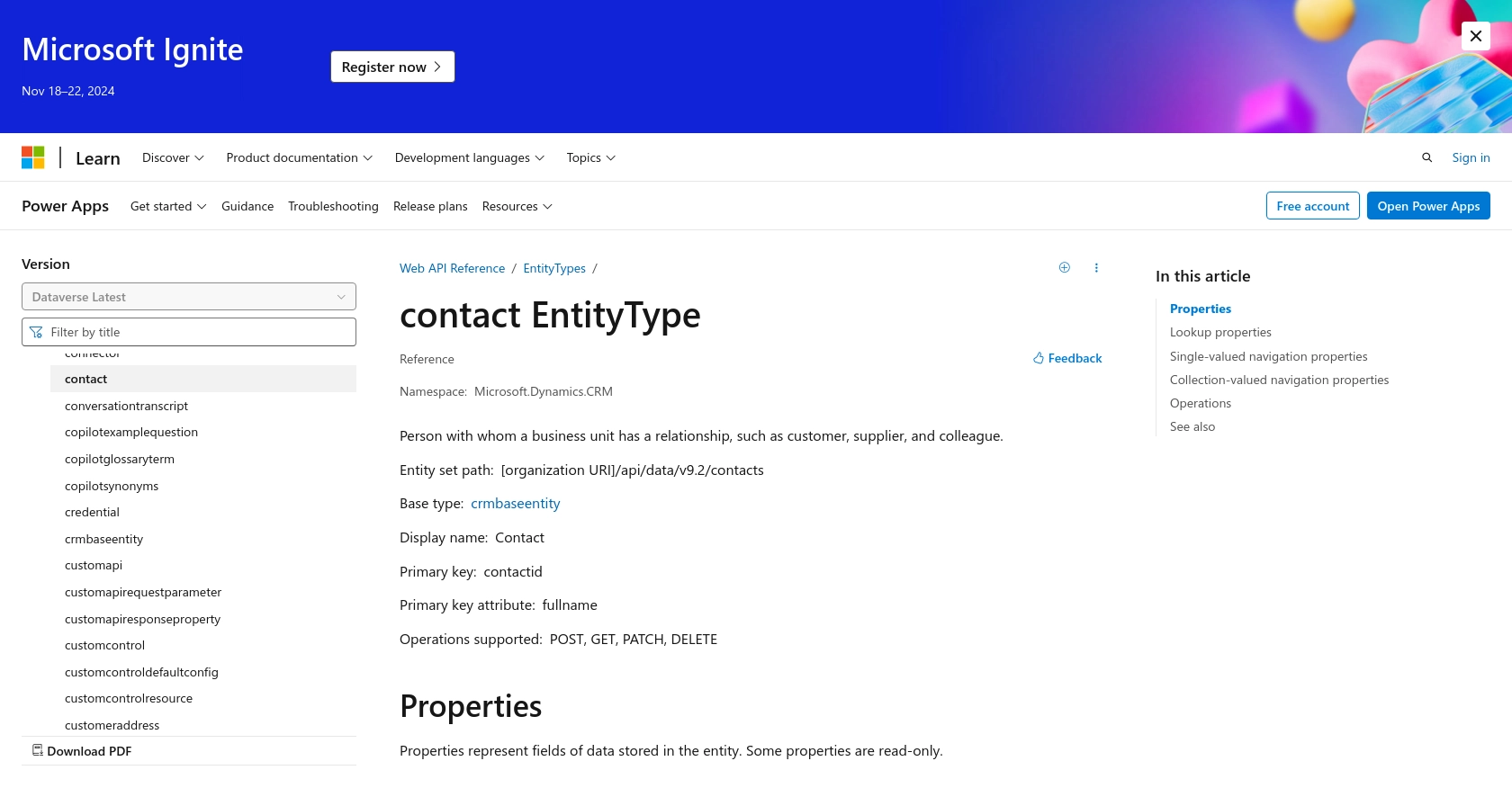
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API using PHP can significantly enhance your business processes by providing seamless access to customer data. By following the steps outlined in this guide, you can efficiently retrieve and manage contacts, enabling better customer insights and interactions.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Consider using environment variables or a secure vault to keep them safe.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data retrieved from Microsoft Dynamics 365 is standardized and transformed as needed to fit your application's data model.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
By adhering to these best practices, you can ensure a robust and secure integration with Microsoft Dynamics 365, maximizing the benefits for your business.
Streamline Your Integrations with Endgrate
If you're looking to simplify your integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can help you scale your integrations efficiently by visiting Endgrate.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/contact?view=dataverse-latest
Ready to get started?