Using the Stripe API to Get Balance Transactions (with Javascript examples)
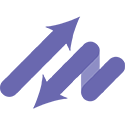
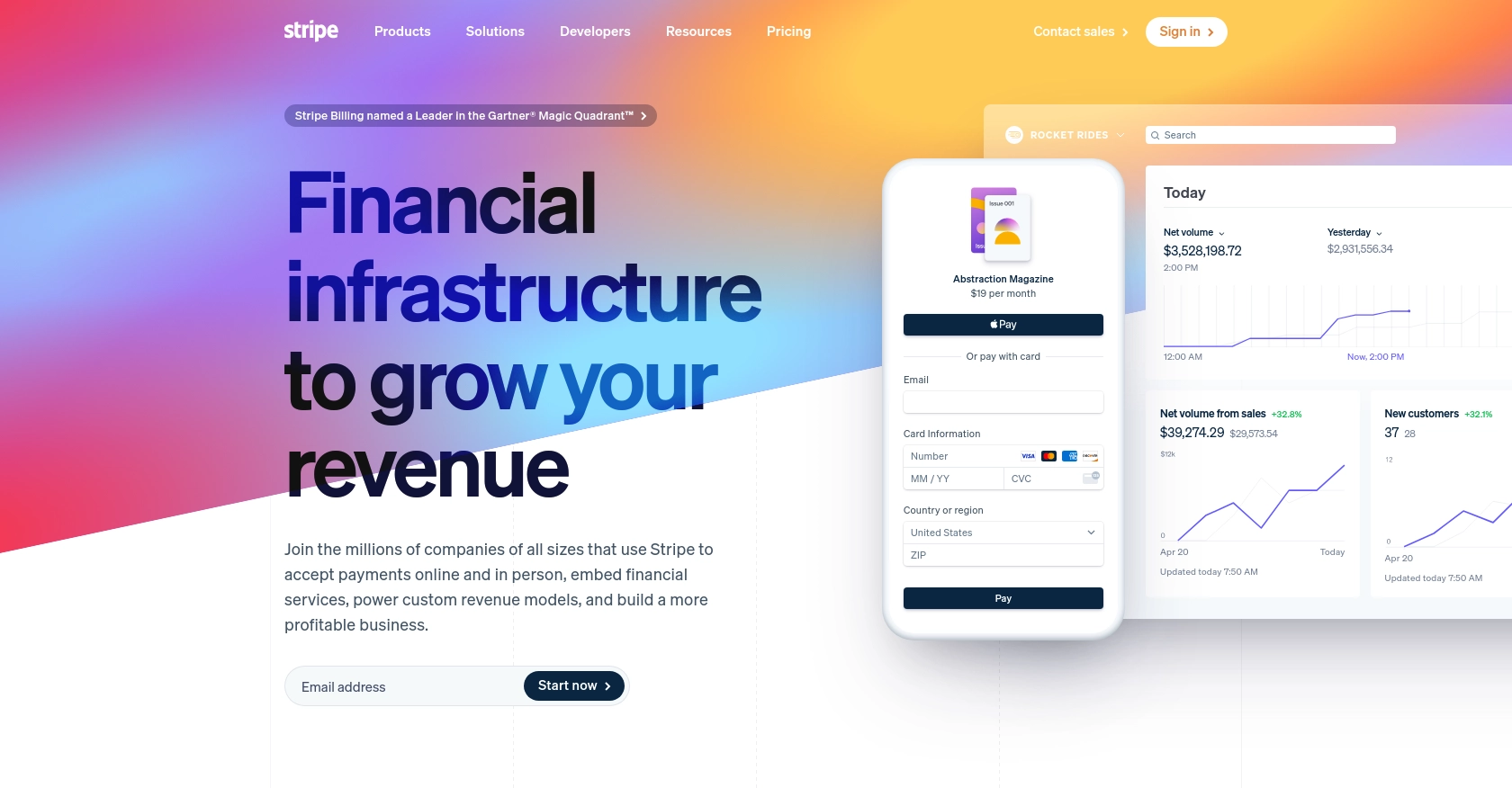
Introduction to Stripe API Integration
Stripe is a powerful payment processing platform that enables businesses to manage online transactions seamlessly. Known for its robust API and extensive documentation, Stripe is a popular choice among developers for integrating payment solutions into web applications.
Developers often connect with Stripe to access financial data, such as balance transactions, which can be crucial for financial reporting and analysis. For example, a developer might use the Stripe API to retrieve balance transaction details to generate financial reports or reconcile accounts.
This article will guide you through using JavaScript to interact with the Stripe API, specifically focusing on retrieving balance transactions. By following this tutorial, you will learn how to efficiently access and manage financial data using Stripe's API.
Setting Up Your Stripe Test Account for API Integration
Before diving into the Stripe API, it's essential to set up a test account to safely experiment with API calls without affecting live data. Stripe provides a robust test environment that mimics real-world scenarios, allowing developers to test their integrations thoroughly.
Creating a Stripe Account
If you don't already have a Stripe account, start by signing up on the Stripe website. The registration process is straightforward and requires basic information about your business.
Accessing the Stripe Dashboard
Once your account is created, log in to the Stripe Dashboard. This is your central hub for managing your Stripe account, including accessing API keys, monitoring transactions, and configuring settings.
Generating API Keys for Authentication
Stripe uses API keys to authenticate requests. Follow these steps to obtain your test API keys:
- Navigate to the Developers section in the Stripe Dashboard.
- Click on API keys to view your keys.
- Use the sk_test_ prefix key for test mode. Keep your keys secure and do not share them publicly.
Configuring API Key-Based Authentication
Stripe's API requires all requests to be made over HTTPS and authenticated using your API key. Here's how to set it up in your JavaScript code:
// Example of setting up Stripe API key in JavaScript
const stripe = require('stripe')('sk_test_your_test_key_here');
Testing with Stripe's Sandbox Environment
Stripe's test mode allows you to simulate transactions and test various scenarios. Use the provided test card numbers and other resources from the Stripe Testing Documentation to ensure your integration works as expected.
With your test account and API keys set up, you're ready to start making API calls to retrieve balance transactions using JavaScript.
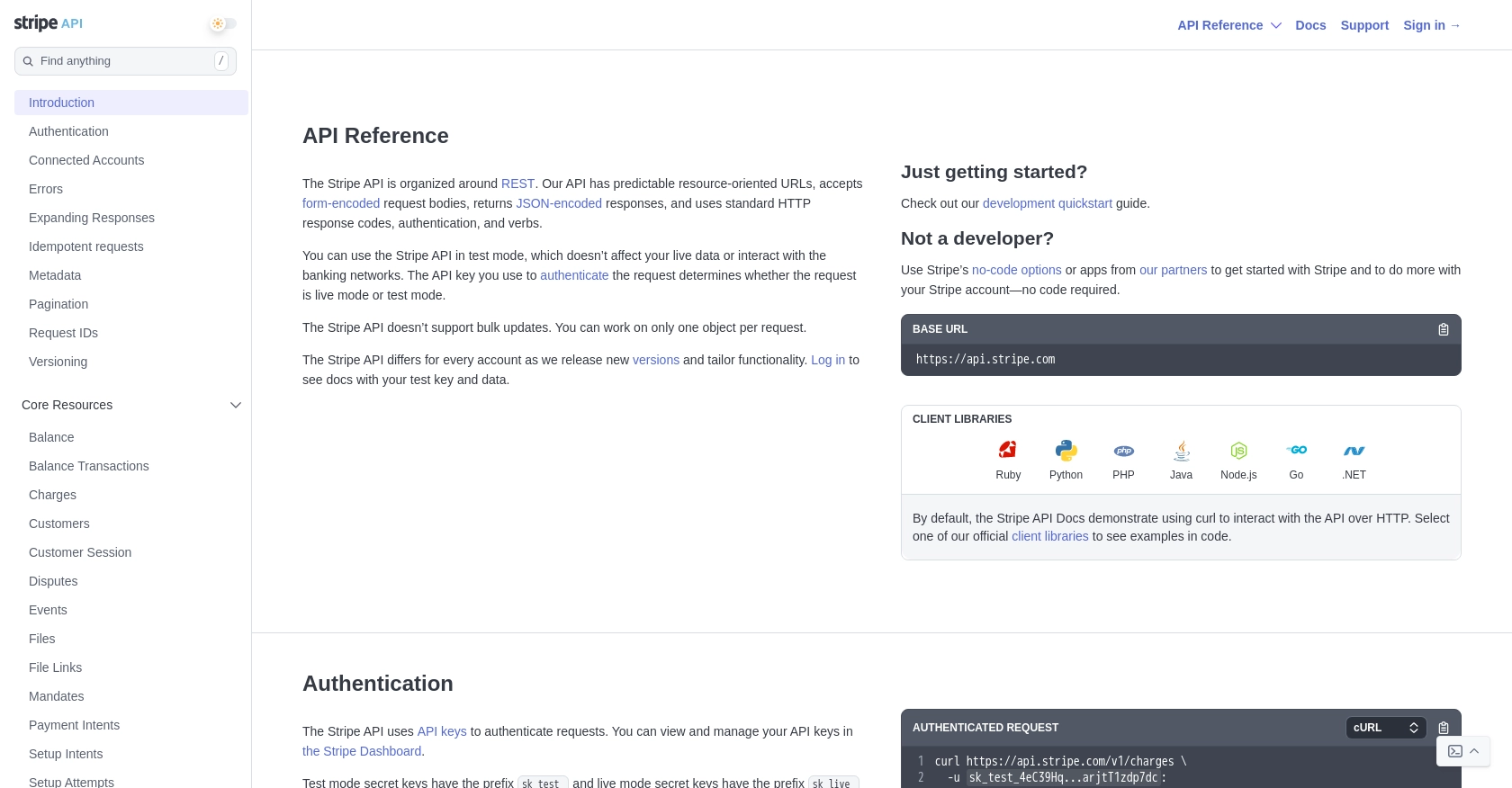
sbb-itb-96038d7
Making API Calls to Retrieve Balance Transactions with Stripe Using JavaScript
To interact with the Stripe API and retrieve balance transactions, you'll need to set up your JavaScript environment and make use of Stripe's official Node.js library. This section will guide you through the process of making API calls to access balance transaction data.
Setting Up Your JavaScript Environment for Stripe API Integration
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once installed, you can use npm (Node Package Manager) to install the Stripe library.
npm install stripe
Writing JavaScript Code to Retrieve Stripe Balance Transactions
With the Stripe library installed, you can now write JavaScript code to interact with the Stripe API. Below is an example of how to retrieve balance transactions:
// Import the Stripe library
const stripe = require('stripe')('sk_test_your_test_key_here');
// Function to get balance transactions
async function getBalanceTransactions() {
try {
const transactions = await stripe.balanceTransactions.list({
limit: 10 // Adjust the limit as needed
});
console.log(transactions);
} catch (error) {
console.error('Error retrieving balance transactions:', error);
}
}
// Call the function
getBalanceTransactions();
In this code, replace 'sk_test_your_test_key_here'
with your actual test API key. The function getBalanceTransactions
retrieves a list of balance transactions, which you can adjust by changing the limit
parameter.
Understanding the Sample Output of Stripe Balance Transactions
When you run the above code, you should see a JSON object containing details of the balance transactions. Each transaction includes information such as the amount, currency, and type of transaction.
Verifying Successful API Requests in Stripe's Dashboard
To verify that your API requests are successful, check the Stripe Dashboard under the "Developers" section. Here, you can view logs of all API requests, including their status and any errors encountered.
Handling Errors and Stripe API Error Codes
Stripe uses standard HTTP response codes to indicate the success or failure of an API request. Common error codes include:
- 400 Bad Request: The request was unacceptable, often due to missing parameters.
- 401 Unauthorized: No valid API key provided.
- 402 Request Failed: Parameters were valid but the request failed.
- 403 Forbidden: The API key doesn’t have permissions to perform the request.
- 429 Too Many Requests: Too many requests hit the API too quickly. Implement exponential backoff.
For more detailed error handling, refer to the Stripe API documentation.
Conclusion and Best Practices for Using Stripe API with JavaScript
Integrating with the Stripe API using JavaScript provides developers with powerful tools to manage financial transactions and data. By following the steps outlined in this guide, you can efficiently retrieve balance transactions and incorporate them into your financial workflows.
Best Practices for Secure and Efficient Stripe API Integration
- Secure API Keys: Always keep your API keys secure and never expose them in client-side code or public repositories.
- Handle Rate Limiting: Stripe enforces rate limits on API requests. Implement exponential backoff strategies to handle
429 Too Many Requests
errors gracefully. - Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application.
- Error Handling: Implement robust error handling to manage different HTTP response codes and provide meaningful feedback to users.
Streamlining Integration Development with Endgrate
While integrating with Stripe is powerful, managing multiple integrations can be time-consuming. Endgrate offers a unified API that simplifies integration processes, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
Ready to get started?