Using the Microsoft Dynamics 365 Business Central API to Create or Update Customers in Javascript
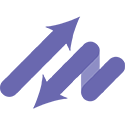
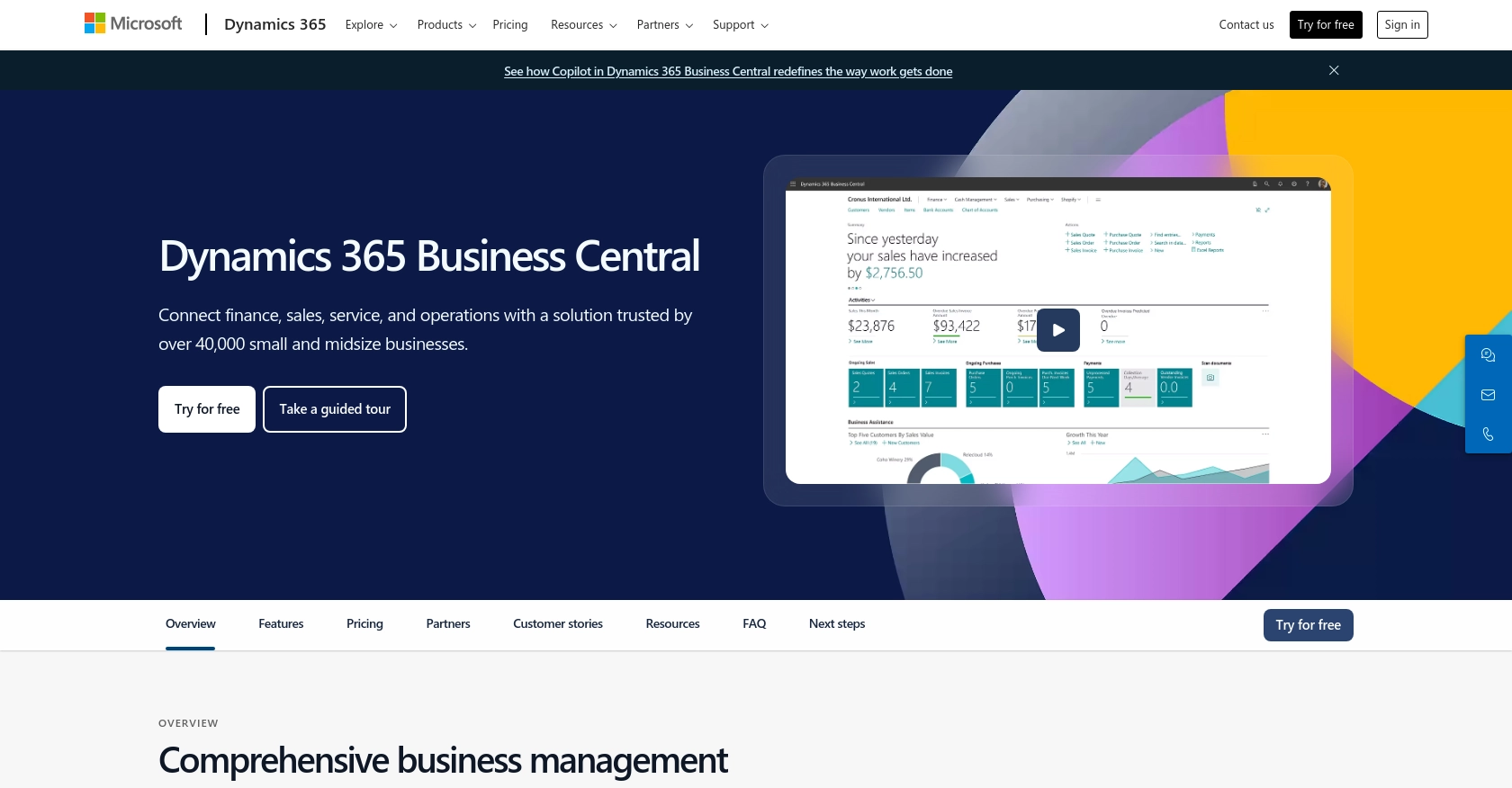
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including finance, sales, service, and operations, all integrated into a single platform. This allows businesses to streamline their processes and make informed decisions with real-time data insights.
For developers, integrating with Microsoft Dynamics 365 Business Central's API can significantly enhance business operations by automating tasks such as customer management. For example, you can create or update customer records directly from your application, ensuring that your business data remains consistent and up-to-date across all platforms.
Setting Up a Microsoft Dynamics 365 Business Central Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 Business Central API, you need to set up a sandbox account. This environment allows you to test your API interactions without affecting live data, providing a safe space to develop and refine your integration.
Creating a Microsoft Dynamics 365 Business Central Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial if you haven't already.
- Once logged in, navigate to the Admin Center from the main dashboard.
- In the Admin Center, select Environments from the left-hand menu.
- Click on New to create a new environment.
- Select Sandbox as the environment type and provide a name for your sandbox.
- Click Create to set up your sandbox environment.
Registering an Application for OAuth Authentication
Microsoft Dynamics 365 Business Central uses OAuth for authentication. To interact with the API, you need to register an application in your Microsoft Entra ID tenant. Follow these steps:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory and select App registrations.
- Click on New registration.
- Enter a name for your application and select the appropriate account type.
- In the Redirect URI section, select Web and enter a URL where the authentication response can be sent. For development, you can use
https://localhost
. - Click Register to create your application.
Generating Client ID and Client Secret
Once your application is registered, you need to generate a Client ID and Client Secret:
- In the Azure Portal, navigate to your registered application.
- Under Certificates & secrets, click on New client secret.
- Provide a description and set an expiration period for the client secret.
- Click Add and copy the generated client secret. Store it securely as it will not be shown again.
- Copy the Application (client) ID from the application's overview page.
With your sandbox environment and application registration complete, you are now ready to authenticate and interact with the Microsoft Dynamics 365 Business Central API.
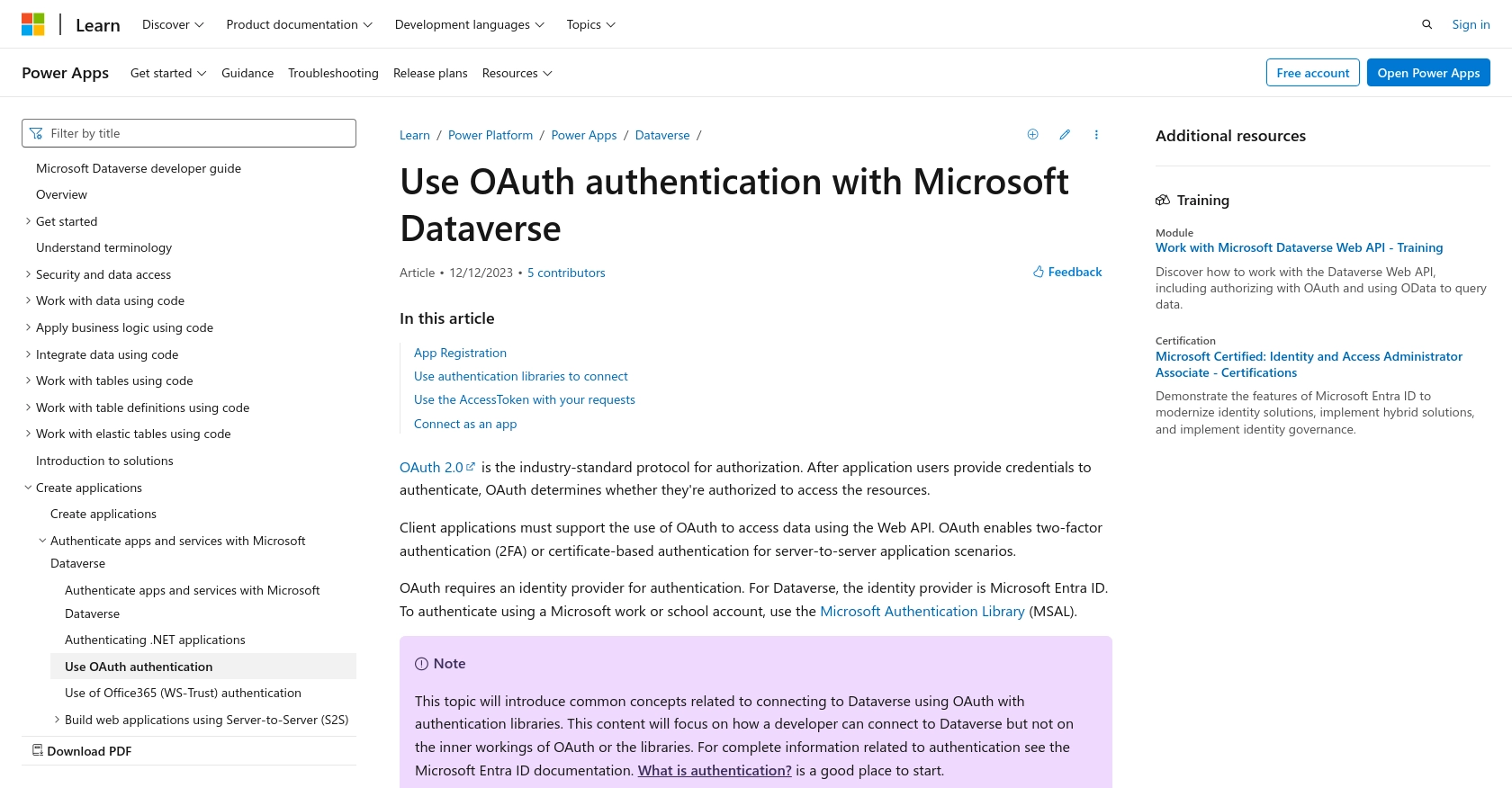
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Business Central Using JavaScript
To interact with the Microsoft Dynamics 365 Business Central API, you'll need to make HTTP requests using JavaScript. This section will guide you through setting up your environment, writing the code to create or update customer records, and handling responses and errors effectively.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
axios
library for making HTTP requests. You can install it using the following command:
npm install axios
Creating or Updating Customers with Microsoft Dynamics 365 Business Central API
To create or update customer records, you'll use the POST
method provided by the API. Below is a sample code snippet demonstrating how to perform this operation:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://{businesscentralPrefix}/api/v2.0/companies({id})/customers';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Define the customer data
const customerData = {
"displayName": "Adatum Corporation",
"type": "Company",
"addressLine1": "192 Market Square",
"city": "Atlanta",
"state": "GA",
"country": "US",
"postalCode": "31772",
"email": "robert.townes@contoso.com",
"currencyCode": "USD"
};
// Make the POST request
axios.post(endpoint, customerData, { headers })
.then(response => {
console.log('Customer Created:', response.data);
})
.catch(error => {
console.error('Error creating customer:', error.response ? error.response.data : error.message);
});
Replace Your_Access_Token
with the token obtained during the OAuth authentication process. This code sends a POST request to the API endpoint with the customer data in JSON format.
Verifying API Call Success in Microsoft Dynamics 365 Business Central
After executing the API call, verify the success by checking the response status code. A successful creation will return a 201 Created
status. You can also log in to your sandbox environment to confirm the new or updated customer record.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. Common error codes include:
400 Bad Request
: The request was malformed or contained invalid data.401 Unauthorized
: Authentication failed due to invalid credentials.403 Forbidden
: The authenticated user does not have permission to perform the operation.404 Not Found
: The specified resource could not be found.
Refer to the official documentation for more details on handling errors.
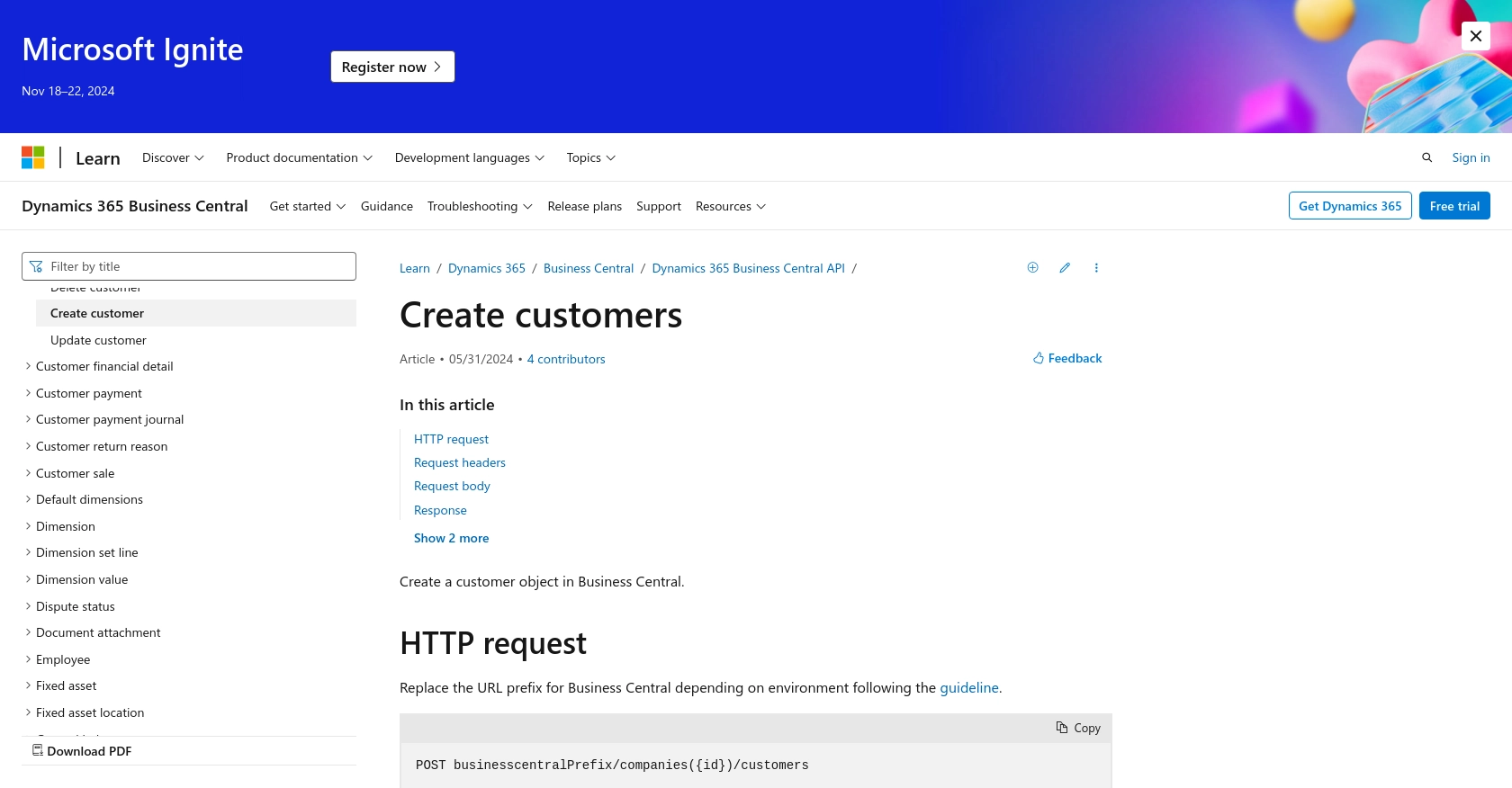
Conclusion and Best Practices for Using Microsoft Dynamics 365 Business Central API
Integrating with the Microsoft Dynamics 365 Business Central API using JavaScript offers a powerful way to automate and streamline customer management processes. By following the steps outlined in this guide, developers can efficiently create or update customer records, ensuring data consistency across platforms.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth tokens, client IDs, and client secrets securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized before making API calls. This helps maintain data integrity and reduces errors during integration.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365 Business Central. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover how it can help you build once for each use case, rather than multiple times for different integrations.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_customer_create
Ready to get started?