Using the Microsoft Dynamics 365 Business Central API to Create or Update Customers in PHP
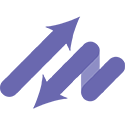
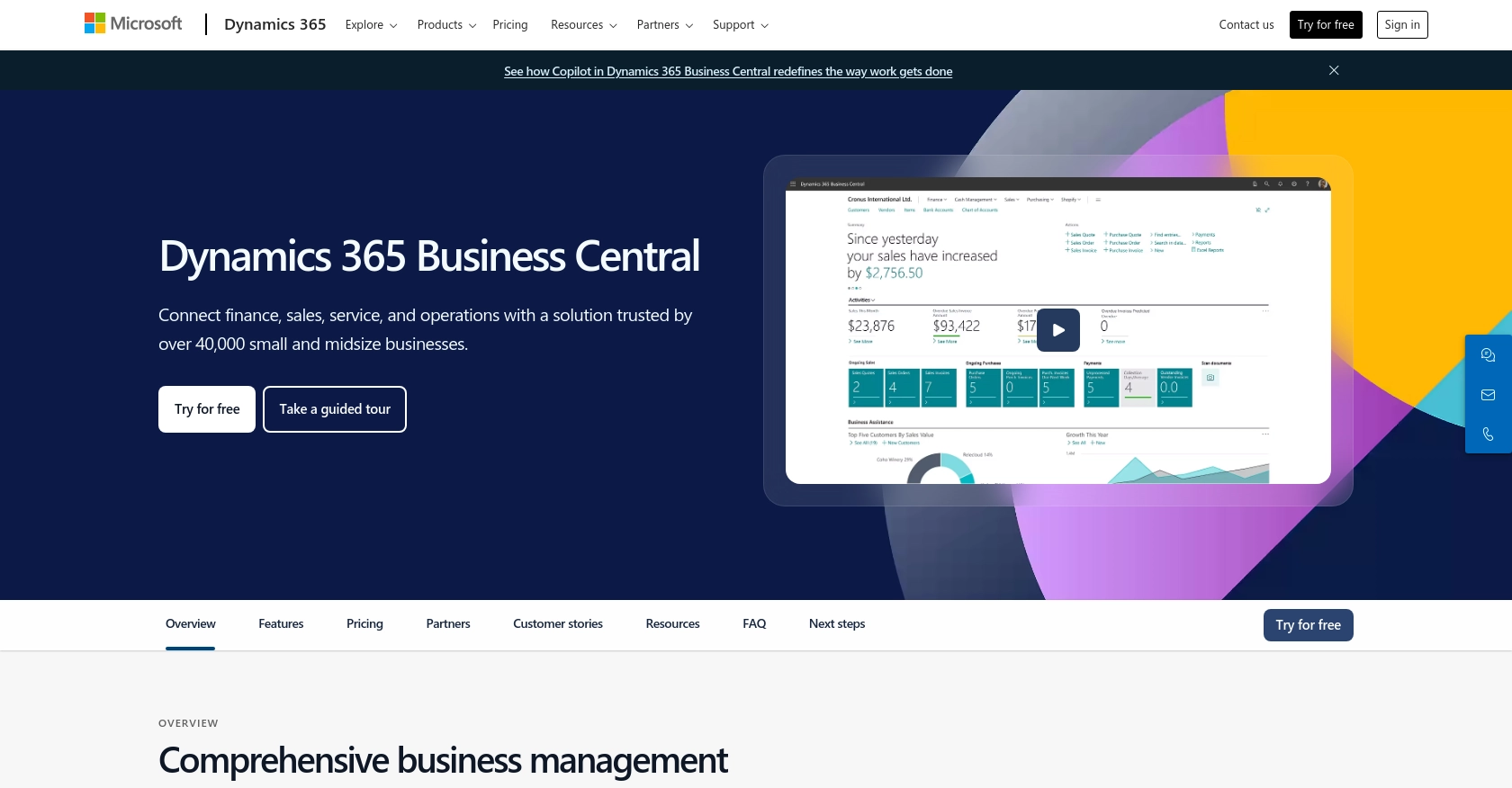
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including finance, sales, service, and operations, all integrated into a single platform. This enables businesses to streamline their processes, improve customer interactions, and make informed decisions based on real-time data.
For developers, integrating with the Microsoft Dynamics 365 Business Central API can significantly enhance the capabilities of their applications. By connecting to this powerful platform, developers can automate tasks such as creating or updating customer records, thereby improving efficiency and reducing manual errors. For example, a developer might use the API to automatically update customer information in Business Central whenever a change is made in an external CRM system, ensuring data consistency across platforms.
Setting Up a Microsoft Dynamics 365 Business Central Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 Business Central API, you'll need to set up a sandbox account. This allows you to test your API calls in a safe environment without affecting live data. Follow these steps to create your sandbox account and prepare for API integration.
Creating a Microsoft Dynamics 365 Business Central Sandbox Account
- Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial if you don't already have an account.
- Once logged in, navigate to the Admin Center and select Environments.
- Click on New to create a new environment. Choose Sandbox as the type to ensure it's a test environment.
- Fill in the necessary details and click Create to set up your sandbox environment.
Registering an Application for OAuth Authentication
To interact with the Microsoft Dynamics 365 Business Central API, you'll need to register an application in Microsoft Entra ID for OAuth authentication. This process involves obtaining a client ID and client secret, which are essential for making authorized API requests.
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory and select App registrations.
- Click on New registration and provide a name for your application.
- Set the Redirect URI to
https://localhost
for local development. - Click Register to create the application.
- After registration, note down the Application (client) ID and Directory (tenant) ID.
- Navigate to Certificates & secrets and create a new client secret. Copy the secret value as it will not be shown again.
Configuring API Permissions
Next, you'll need to configure the necessary API permissions for your registered application to access Microsoft Dynamics 365 Business Central data.
- In the Azure Portal, go to your registered application and select API permissions.
- Click on Add a permission and choose Dynamics 365 Business Central.
- Select the permissions required for your application, such as user_impersonation for delegated access.
- Click Add permissions and ensure you grant admin consent for the permissions.
With your sandbox account and application setup complete, you're now ready to start making API calls to Microsoft Dynamics 365 Business Central using PHP.
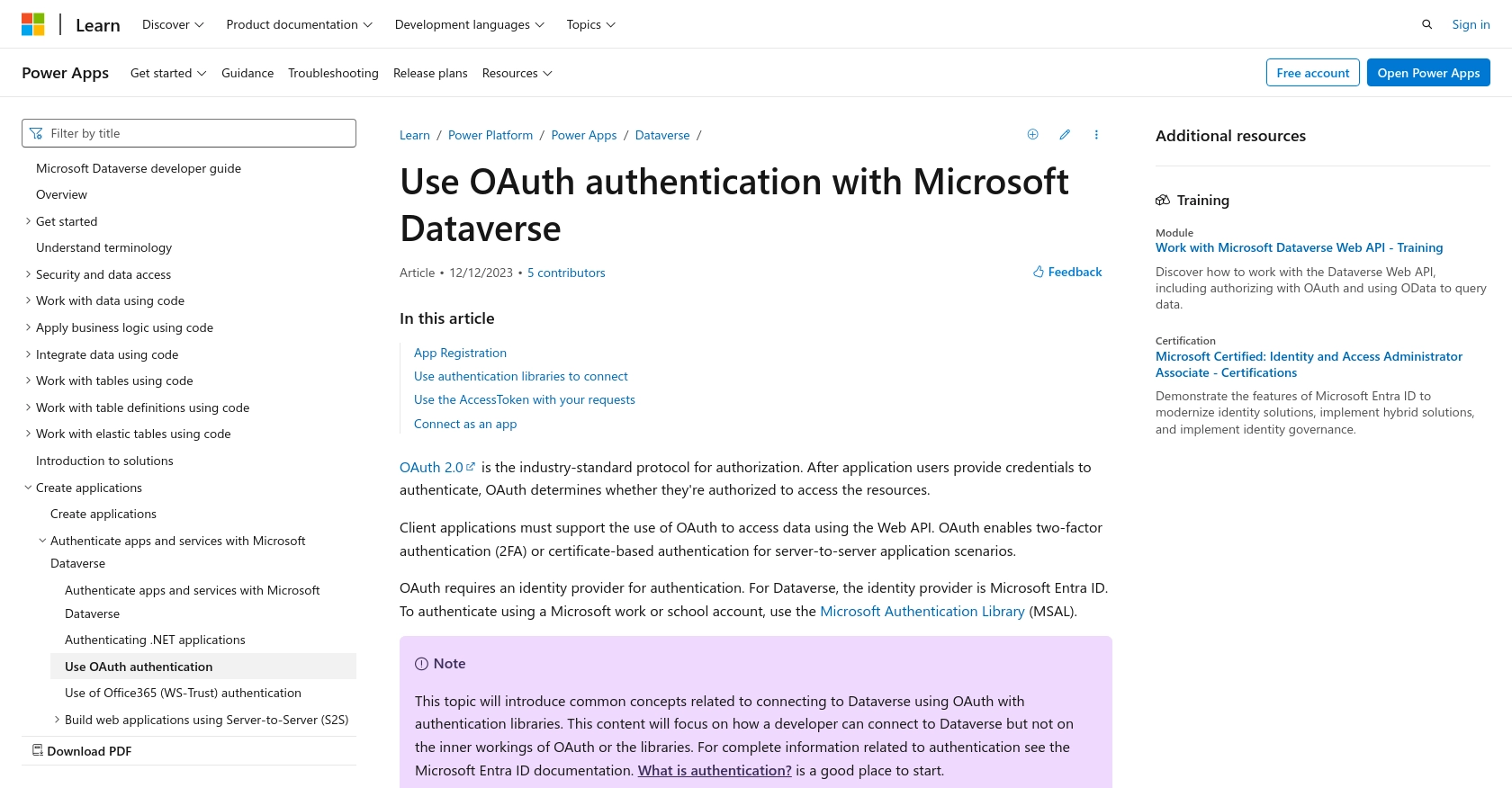
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Business Central Using PHP
To interact with the Microsoft Dynamics 365 Business Central API using PHP, you'll need to set up your environment and write code to create or update customer records. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API requests.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
Once you have these installed, open your terminal and create a new project directory:
mkdir dynamics-api-integration
cd dynamics-api-integration
Installing Required PHP Libraries
To handle HTTP requests and OAuth authentication, you'll need to install the Guzzle HTTP client and the league/oauth2-client library. Run the following command in your project directory:
composer require guzzlehttp/guzzle league/oauth2-client
Writing PHP Code to Create or Update Customers
With your environment set up, you can now write the PHP code to interact with the Microsoft Dynamics 365 Business Central API. Create a new file named customer_api.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
use League\OAuth2\Client\Provider\GenericProvider;
// Set up OAuth provider
$provider = new GenericProvider([
'clientId' => 'your-client-id',
'clientSecret' => 'your-client-secret',
'redirectUri' => 'https://localhost',
'urlAuthorize' => 'https://login.microsoftonline.com/your-tenant-id/oauth2/v2.0/authorize',
'urlAccessToken' => 'https://login.microsoftonline.com/your-tenant-id/oauth2/v2.0/token',
'urlResourceOwnerDetails' => '',
'scopes' => 'https://api.businesscentral.dynamics.com/.default'
]);
// Obtain an access token
$accessToken = $provider->getAccessToken('client_credentials');
// Set up HTTP client
$client = new Client([
'base_uri' => 'https://api.businesscentral.dynamics.com/v2.0/your-environment-id/',
'headers' => [
'Authorization' => 'Bearer ' . $accessToken->getToken(),
'Content-Type' => 'application/json'
]
]);
// Create or update a customer
$response = $client->post('companies(your-company-id)/customers', [
'json' => [
'displayName' => 'Adatum Corporation',
'type' => 'Company',
'addressLine1' => '192 Market Square',
'city' => 'Atlanta',
'state' => 'GA',
'country' => 'US',
'postalCode' => '31772',
'email' => 'robert.townes@contoso.com'
]
]);
// Handle the response
if ($response->getStatusCode() === 201) {
echo "Customer created successfully.";
} else {
echo "Failed to create customer.";
}
?>
Replace your-client-id
, your-client-secret
, your-tenant-id
, your-environment-id
, and your-company-id
with your actual values.
Verifying the API Request Success
After running the script, check your Microsoft Dynamics 365 Business Central sandbox environment to verify that the customer record has been created or updated. If successful, the response will indicate a 201 status code, confirming the creation of the customer.
Handling Errors and Error Codes
When making API calls, it's important to handle potential errors. The Microsoft Dynamics 365 Business Central API may return various HTTP status codes to indicate success or failure. For example, a 400 status code indicates a bad request, while a 401 status code indicates unauthorized access. Be sure to implement error handling in your code to manage these scenarios gracefully.
For more detailed information on error codes, refer to the official documentation.
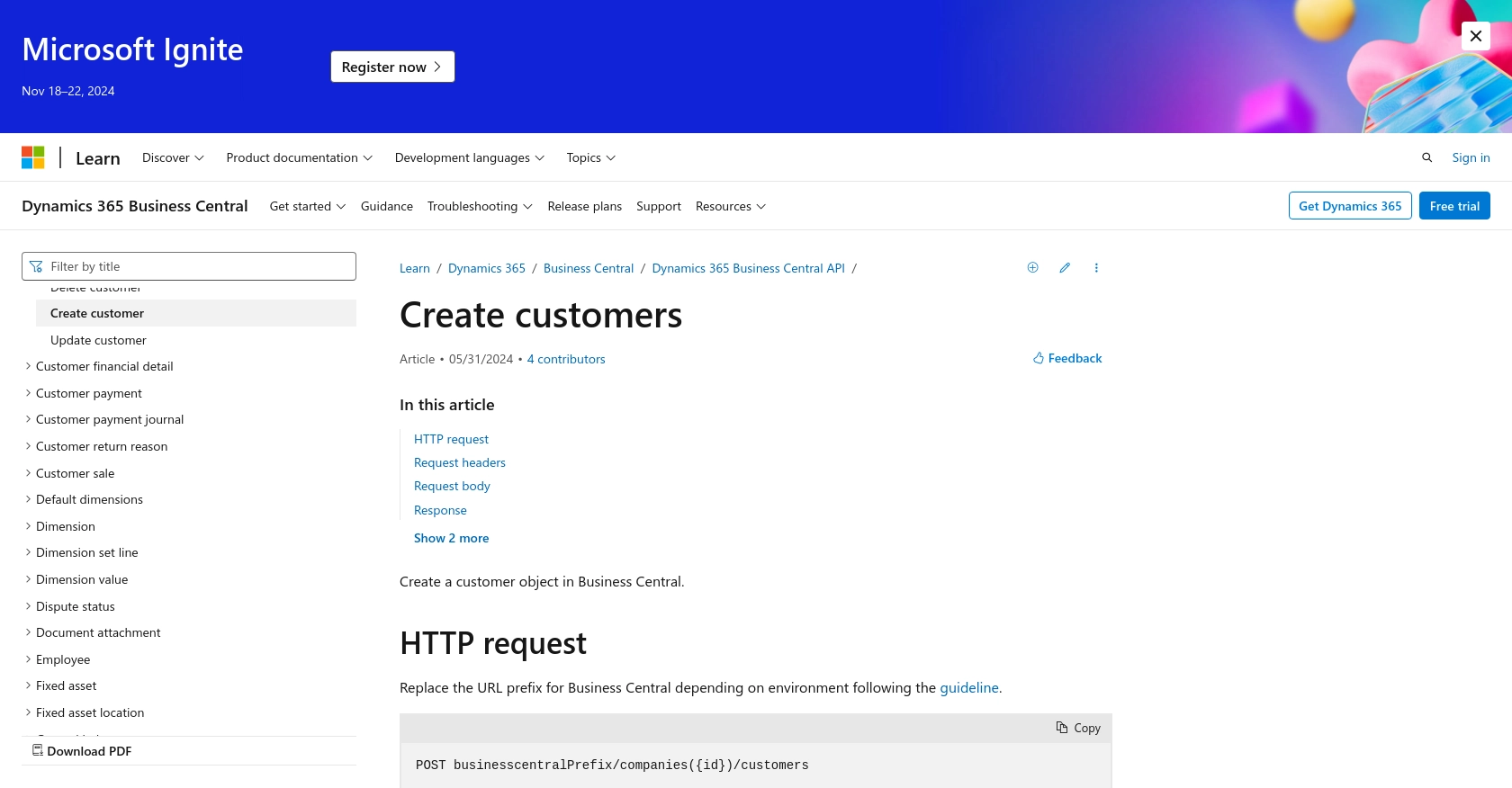
Best Practices for Using Microsoft Dynamics 365 Business Central API
When integrating with the Microsoft Dynamics 365 Business Central API, it's crucial to follow best practices to ensure secure and efficient operations. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be aware of the API rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully. For more details, refer to the official documentation.
- Data Transformation: Ensure that data fields are standardized and transformed as needed to maintain consistency across systems.
- Error Handling: Implement robust error handling to manage different HTTP status codes and exceptions. Log errors for monitoring and debugging purposes.
Enhance Your Integrations with Endgrate
Integrating with Microsoft Dynamics 365 Business Central can be complex, especially when managing multiple integrations. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365 Business Central.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_customer_create
Ready to get started?