Using the Shopify API to Create or Update Custom Collections (with PHP examples)
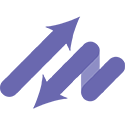
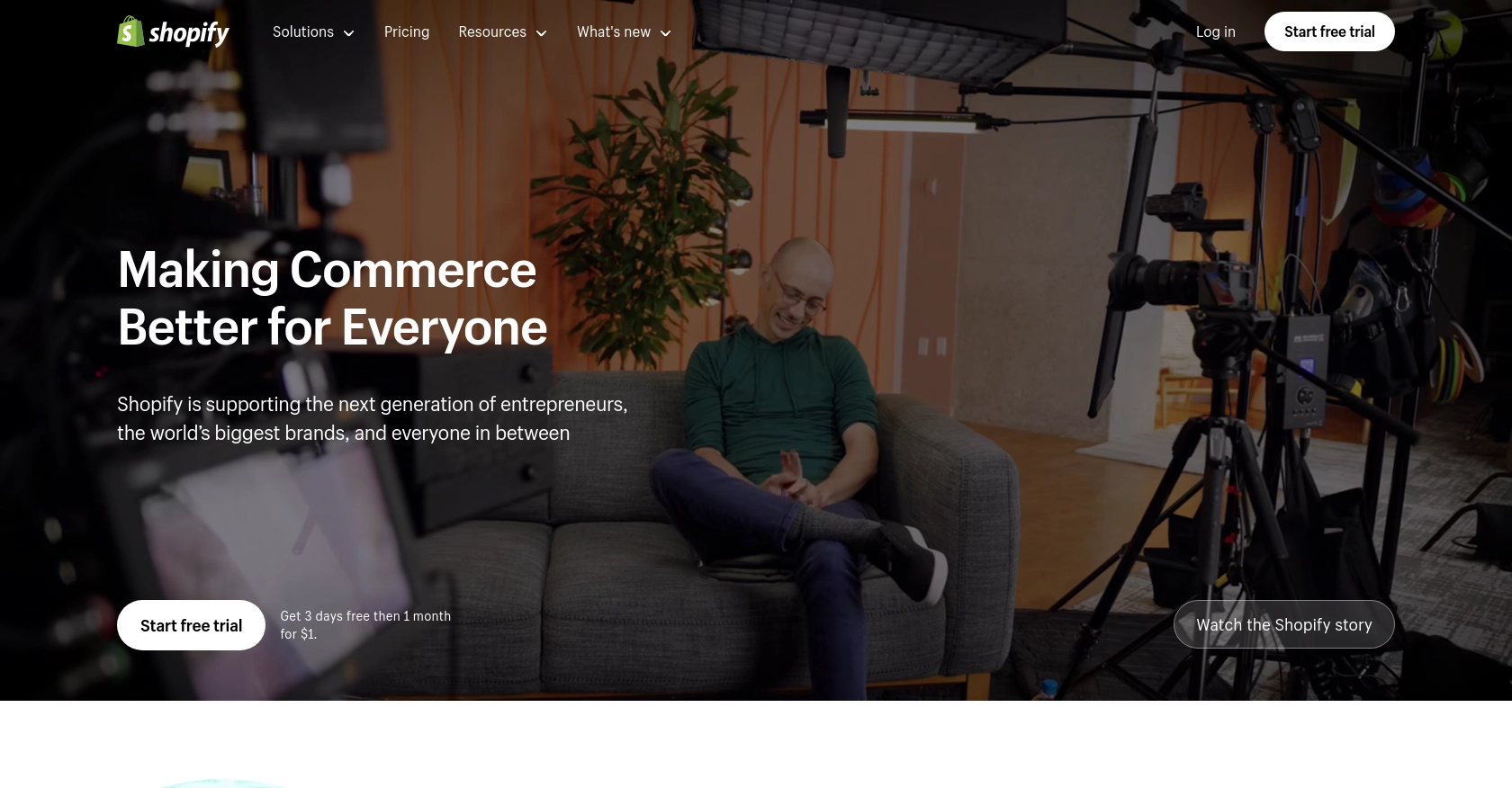
Introduction to Shopify API for Custom Collections
Shopify is a leading e-commerce platform that empowers businesses to create and manage their online stores with ease. It offers a robust suite of tools and features that cater to businesses of all sizes, enabling them to sell products and services online efficiently.
Developers often integrate with Shopify's API to enhance store functionality and automate various tasks. One common use case is managing custom collections, which are groupings of products that merchants can create to organize their stores better. For example, a developer might use the Shopify API to create a custom collection for seasonal products, ensuring that the store's offerings are always relevant and up-to-date.
This article will guide you through using the Shopify API to create or update custom collections using PHP, providing detailed steps and code examples to streamline your integration process.
Setting Up a Shopify Test or Sandbox Account for API Integration
Before diving into the Shopify API integration for creating or updating custom collections, it's essential to set up a test or sandbox account. This environment allows developers to experiment and test their integrations without affecting a live store.
Creating a Shopify Partner Account
To begin, you need a Shopify Partner account, which provides access to development stores. Follow these steps to create one:
- Visit the Shopify Partners page and sign up for a free account.
- Fill in the required information, including your business details and contact information.
- Once registered, log in to your Shopify Partner dashboard.
Setting Up a Shopify Development Store
With your Partner account ready, you can create a development store:
- In the Shopify Partner dashboard, navigate to the "Stores" section.
- Click on "Add store" and select "Development store."
- Fill in the store details, such as store name and login credentials.
- Choose the "Development store" option to ensure it's for testing purposes.
- Click "Save" to create your development store.
Configuring OAuth Authentication for Shopify API
Shopify uses OAuth for API authentication. Here's how to set it up:
- In your Shopify Partner dashboard, go to "Apps" and click "Create app."
- Enter the app name and select the development store you created earlier.
- Under "App setup," configure the app's URL and redirect URLs.
- In the "API credentials" section, note down the API key and API secret key.
- Set the necessary scopes for your app, such as
write_products
andread_products
, to manage collections. - Save your changes to generate the OAuth credentials.
For more detailed information on authentication, refer to the Shopify API Authentication documentation.
Generating Access Tokens
To interact with the Shopify API, you'll need an access token:
- Direct your app users to the OAuth authorization URL, which includes your API key and requested scopes.
- Once users authorize the app, Shopify redirects them to your specified redirect URL with a temporary code.
- Exchange this code for a permanent access token using the API secret key.
With these steps completed, you're ready to start integrating with the Shopify API in your development environment.
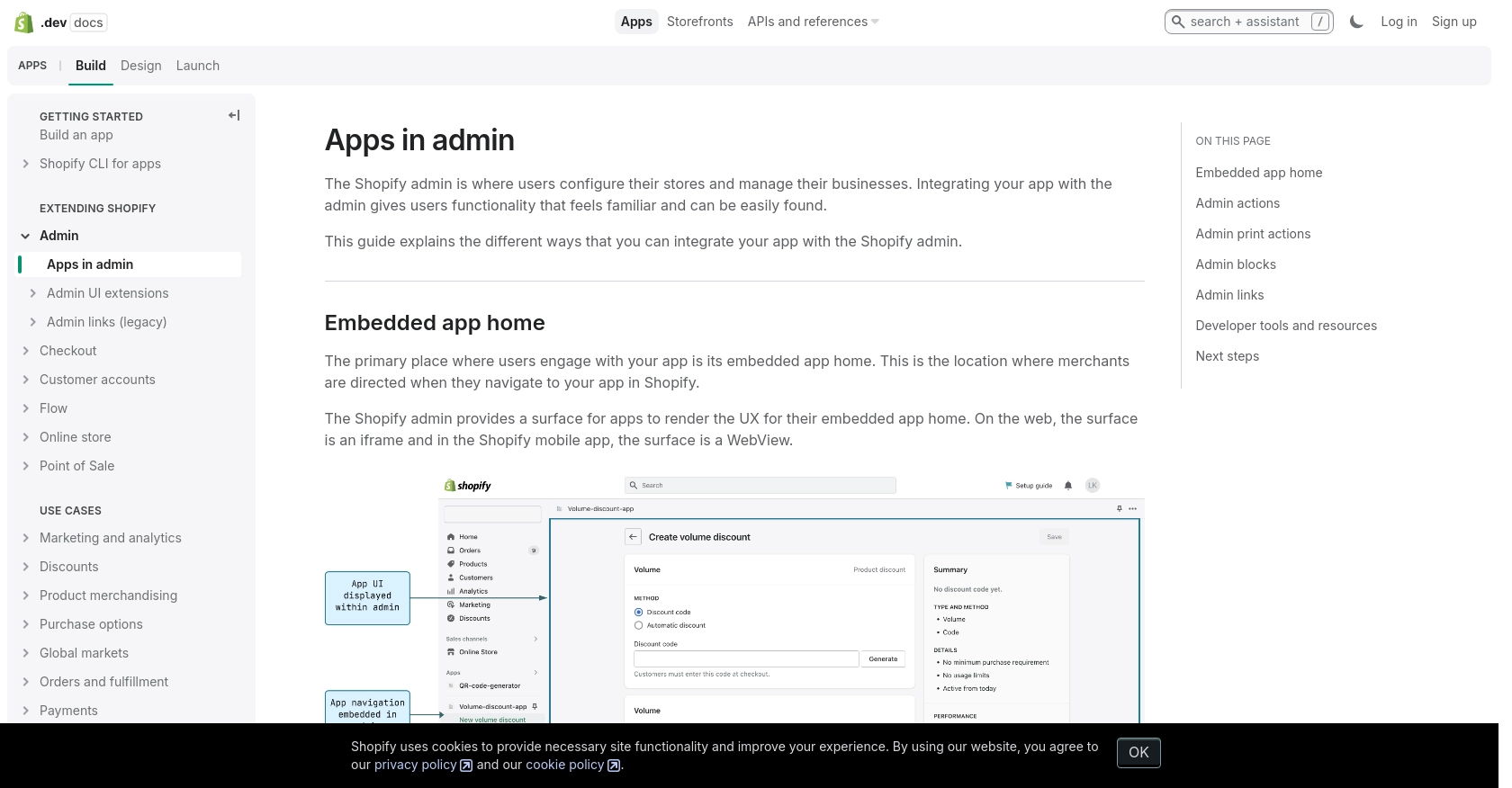
sbb-itb-96038d7
Making Shopify API Calls to Create or Update Custom Collections Using PHP
To interact with Shopify's API for creating or updating custom collections, you'll need to use PHP to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Shopify API Integration
Before making API calls, ensure your PHP environment is ready:
- Install PHP 7.4 or higher.
- Ensure the
cURL
extension is enabled in yourphp.ini
file. - Use Composer to install any necessary packages, such as
guzzlehttp/guzzle
for HTTP requests.
Creating a Custom Collection with Shopify API Using PHP
To create a custom collection, you'll need to send a POST request to the Shopify API. Here's an example:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'your_access_token';
$storeUrl = 'https://your-development-store.myshopify.com';
$response = $client->post("$storeUrl/admin/api/2024-07/custom_collections.json", [
'headers' => [
'Content-Type' => 'application/json',
'X-Shopify-Access-Token' => $accessToken
],
'json' => [
'custom_collection' => [
'title' => 'Seasonal Products',
'body_html' => '<p>A collection of seasonal products</p>',
'published' => true
]
]
]);
echo $response->getBody();
?>
Replace your_access_token
and your-development-store.myshopify.com
with your actual access token and store URL. This code creates a new custom collection titled "Seasonal Products."
Updating a Custom Collection with Shopify API Using PHP
To update an existing custom collection, use a PUT request. Here's how:
<?php
$collectionId = 'your_collection_id';
$response = $client->put("$storeUrl/admin/api/2024-07/custom_collections/$collectionId.json", [
'headers' => [
'Content-Type' => 'application/json',
'X-Shopify-Access-Token' => $accessToken
],
'json' => [
'custom_collection' => [
'title' => 'Updated Seasonal Products'
]
]
]);
echo $response->getBody();
?>
Replace your_collection_id
with the ID of the collection you wish to update. This code updates the title of the specified collection.
Handling Shopify API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors:
- Check the HTTP status code to verify success. A 200 status code indicates a successful request.
- Handle errors such as 401 Unauthorized or 429 Too Many Requests by checking the response and implementing retry logic if necessary.
- Log errors for further analysis and debugging.
For more details on error codes, refer to the Shopify API Authentication documentation.
By following these steps, you can effectively interact with Shopify's API to manage custom collections, enhancing your store's organization and functionality.
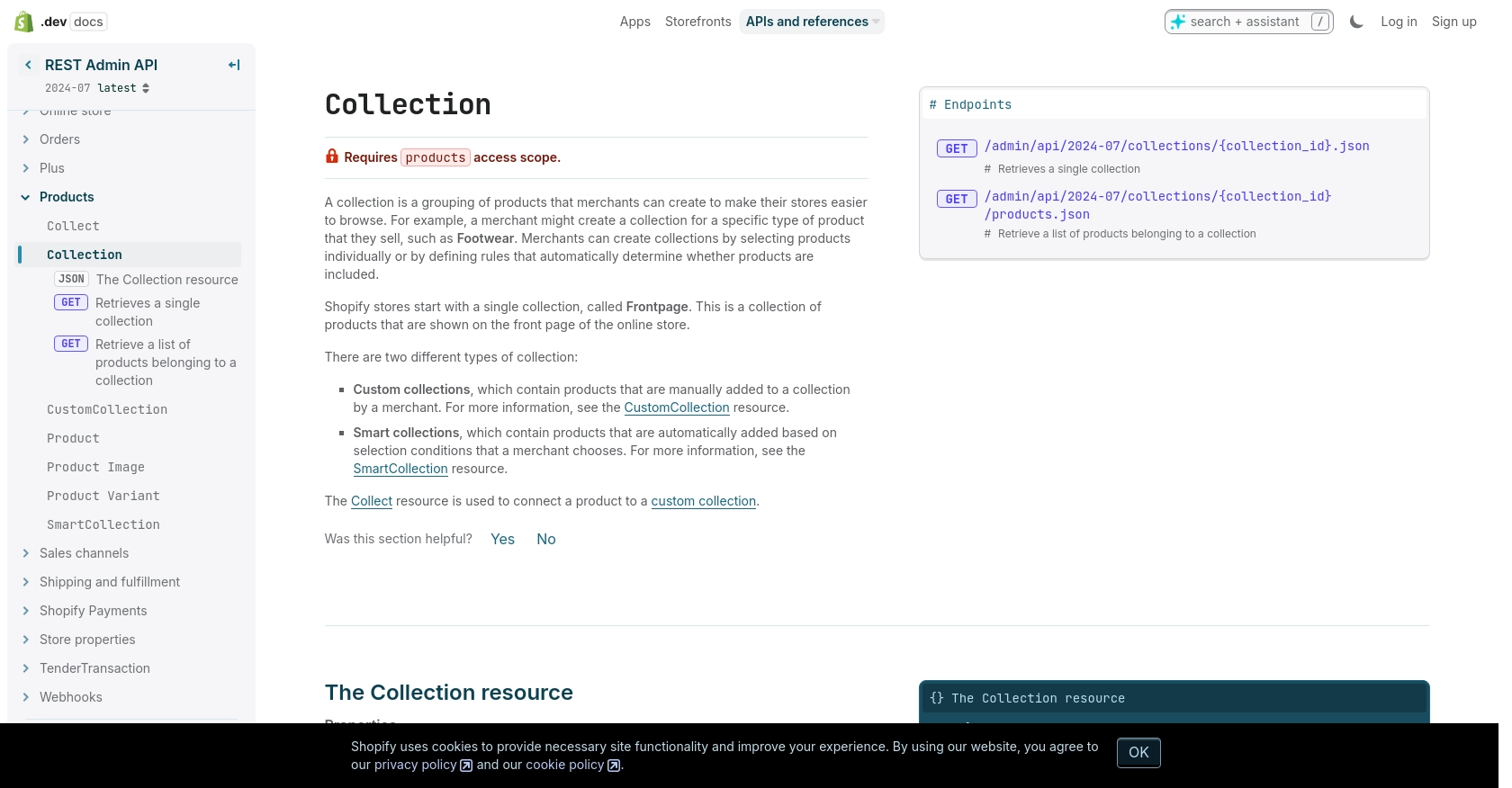
Conclusion and Best Practices for Shopify API Integration Using PHP
Integrating with the Shopify API to manage custom collections can significantly enhance the functionality and organization of your online store. By following the steps outlined in this article, you can efficiently create and update collections, ensuring your store's offerings remain relevant and well-organized.
Best Practices for Secure and Efficient Shopify API Usage
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Shopify's API has a rate limit of 40 requests per app per store per minute. Implement logic to handle 429 Too Many Requests errors by pausing and retrying after the specified time in the
Retry-After
header. For more details, refer to the Shopify API Rate Limits documentation. - Log and Monitor API Calls: Implement logging for all API interactions to monitor usage and troubleshoot issues. This will help in identifying patterns and optimizing your API usage.
- Data Standardization: Ensure that the data you send and receive is standardized and consistent. This will help in maintaining data integrity across different systems and platforms.
Leverage Endgrate for Streamlined Integration Management
While integrating with Shopify's API can be rewarding, it can also be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Shopify. By using Endgrate, you can:
- Save time and resources by outsourcing integration management and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, simplifying your development process.
- Provide an easy and intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Read More
Ready to get started?