Using the Nimble API to Get Contacts in PHP
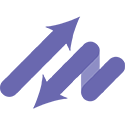
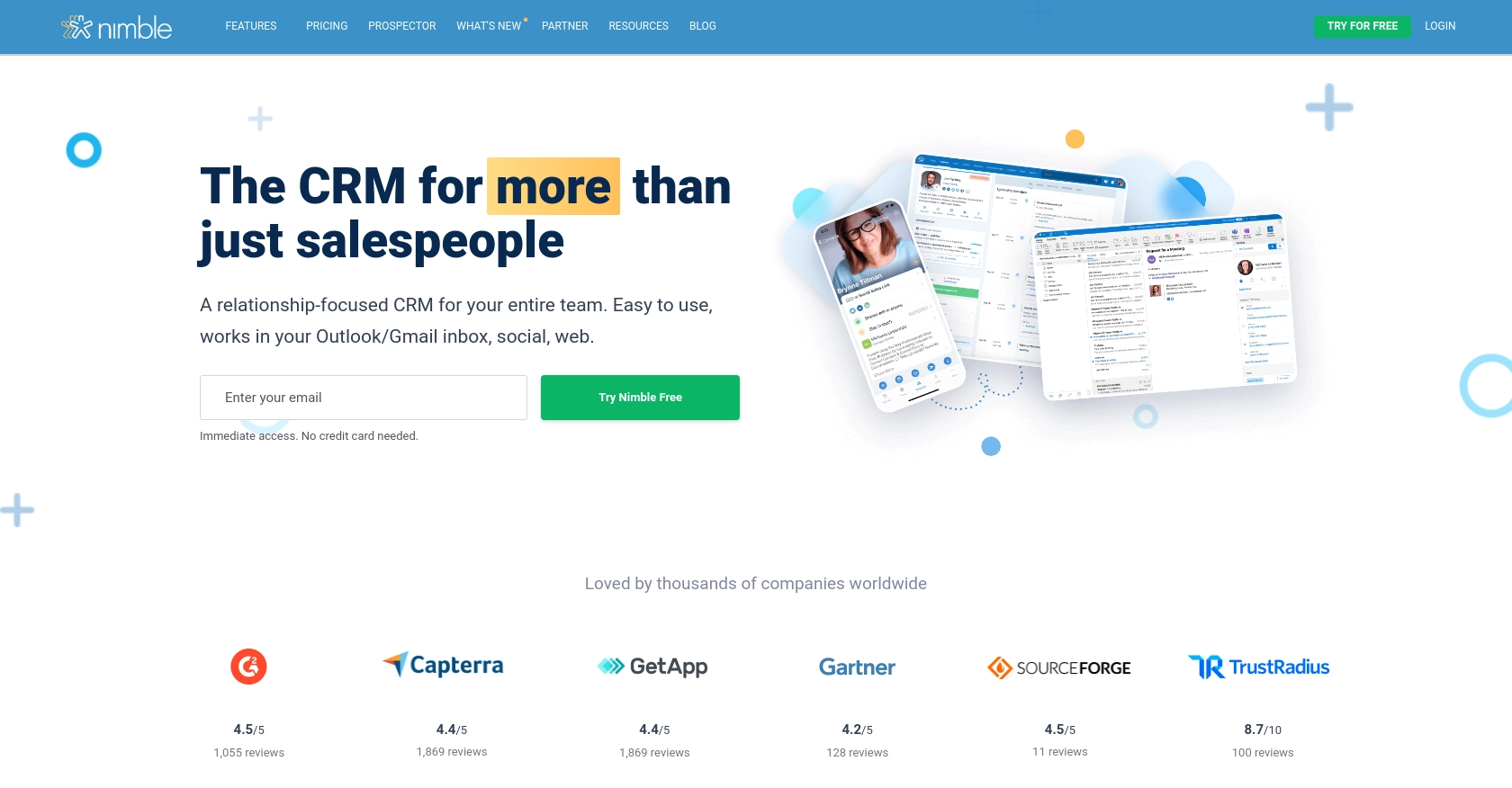
Introduction to Nimble CRM
Nimble is a relationship-focused CRM platform that helps businesses manage their contacts, tasks, and deals efficiently. With its seamless integration capabilities, Nimble allows users to connect with both Microsoft 365 and Google Workspace, making it a versatile choice for businesses looking to streamline their customer relationship management processes.
Developers might want to integrate with Nimble's API to automate and enhance their CRM functionalities. For example, using the Nimble API, a developer can retrieve contact information to synchronize it with other applications, ensuring that all customer data is up-to-date and accessible across platforms.
Setting Up Your Nimble API Test Account
Before you can start using the Nimble API to retrieve contacts in PHP, you'll need to set up a test account. This involves generating an API key, which will allow you to authenticate your requests to the Nimble API.
Creating a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial on the Nimble website. This will give you access to the platform's features and allow you to explore its capabilities.
Generating an API Key for Nimble
Once you have your Nimble account, follow these steps to generate an API key:
- Log in to your Nimble account.
- Navigate to the Settings section.
- Under API Token, select Generate New Token.
- Provide a description for your token and select the necessary scopes.
- Click Generate to create your API token.
Make sure to copy and securely store your API key, as you'll need it to authenticate your API requests.
Granting API Access
Ensure that API access is granted by the admin of your Nimble account. Admins can manage API access permissions in the Settings under the Users page.
Authenticating API Requests with Nimble
To authenticate your API requests, include the API key in the HTTP header as follows:
$apikey = "YOUR_API_KEY";
$headers = [
"Authorization: Bearer " . $apikey
];
Replace YOUR_API_KEY
with the API key you generated. This header will be used in all your API requests to Nimble.
For more details on generating an API key, refer to the Nimble API documentation.
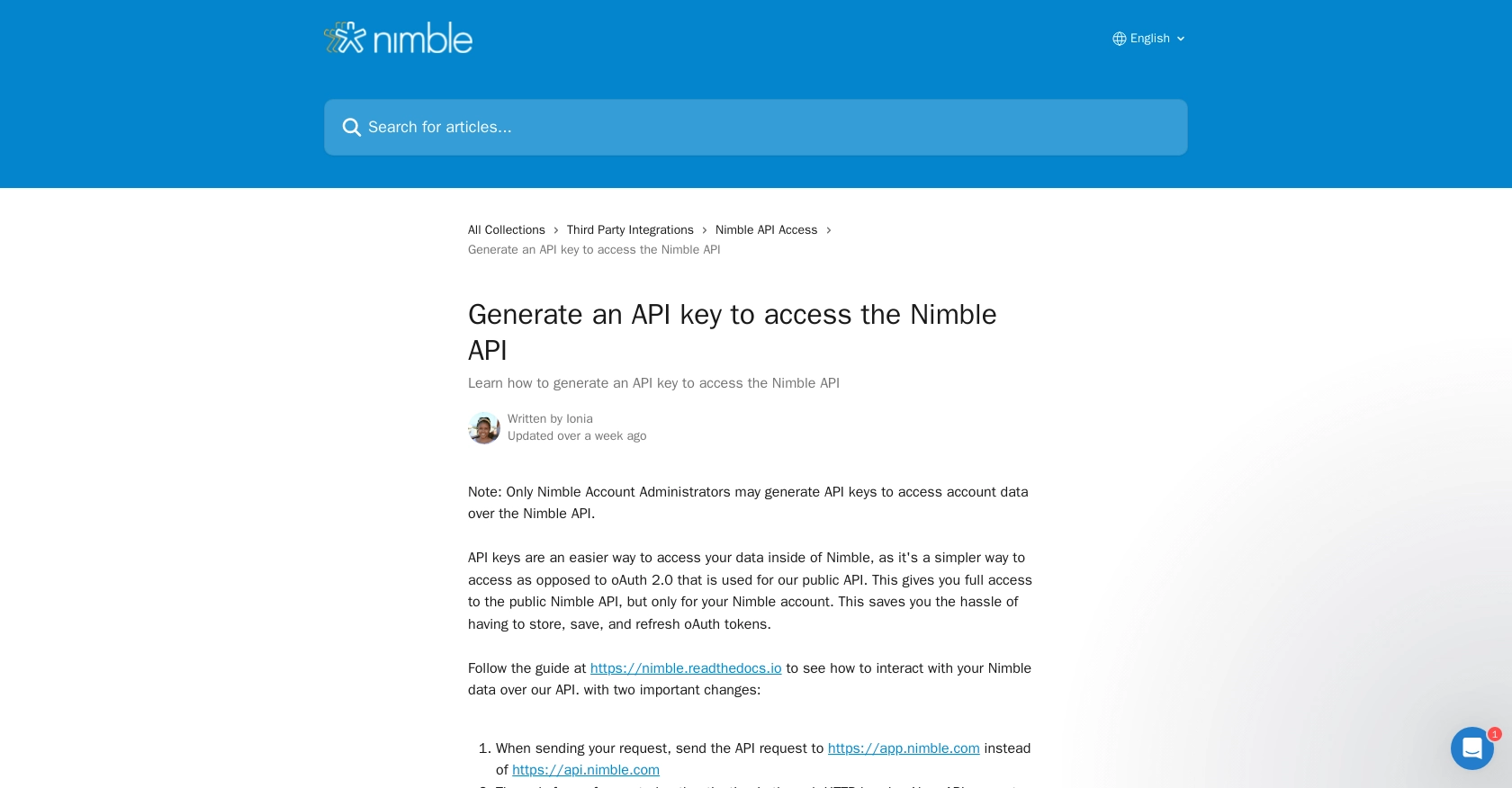
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Nimble Using PHP
To interact with the Nimble API and retrieve contact information using PHP, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you have everything you need to get started.
Setting Up Your PHP Environment
Before making API calls, ensure you have PHP installed on your machine. We recommend using PHP version 7.4 or higher for compatibility and security reasons. Additionally, you'll need to install the cURL
extension, which is commonly used for making HTTP requests in PHP.
sudo apt-get install php-curl
Installing Required PHP Dependencies
To handle HTTP requests, we'll use the cURL
library. Ensure it's enabled in your PHP configuration. You can verify this by checking your php.ini
file or running the following command:
php -m | grep curl
Writing PHP Code to Retrieve Contacts from Nimble
Create a new PHP file named get_nimble_contacts.php
and add the following code:
<?php
$apikey = "YOUR_API_KEY";
$url = "https://app.nimble.com/api/v1/contacts";
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer " . $apikey
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display the response
$contacts = json_decode($response, true);
foreach ($contacts['resources'] as $contact) {
echo "Name: " . $contact['fields']['first name'][0]['value'] . " " . $contact['fields']['last name'][0]['value'] . "\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace YOUR_API_KEY
with the API key you generated earlier. This script initializes a cURL session, sets the necessary headers for authentication, and makes a GET request to the Nimble API to retrieve contacts.
Running Your PHP Script
To execute the script, run the following command in your terminal:
php get_nimble_contacts.php
If successful, you should see a list of contacts retrieved from your Nimble account displayed in the terminal.
Handling API Response and Errors
It's crucial to handle potential errors when making API requests. The script checks for cURL errors and outputs them if any occur. Additionally, ensure you handle HTTP response codes to manage different scenarios, such as unauthorized access or rate limits.
For more information on error handling and response codes, refer to the Nimble API documentation.
Best Practices for Using Nimble API in PHP
When working with the Nimble API, it's essential to follow best practices to ensure security and efficiency. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely, preferably in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Nimble API. Implement retry logic and exponential backoff to handle rate limit errors gracefully.
- Validate API Responses: Always check the response status codes and handle errors appropriately. This includes managing validation errors, quota limits, and server errors.
- Optimize Data Handling: When retrieving large datasets, consider implementing pagination to manage data efficiently and reduce load times.
Enhancing Your Integration with Endgrate
Integrating multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Nimble. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Deploy Everywhere: Create a single integration that works across multiple platforms, reducing development overhead.
- Improve Customer Experience: Offer your users a seamless and intuitive integration experience.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
Ready to get started?