Using the Notion API to Get Records in PHP
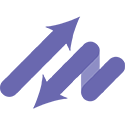
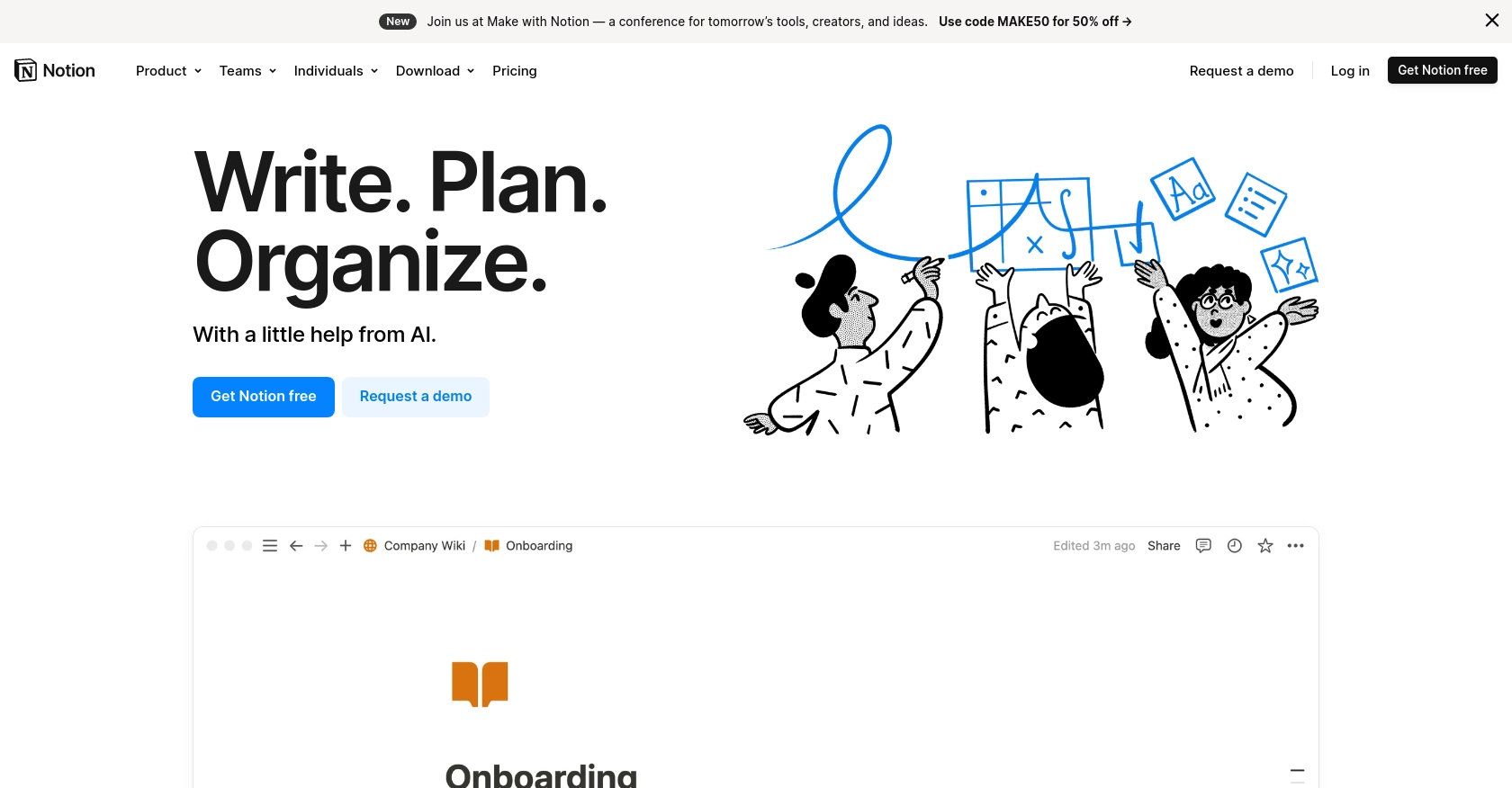
Introduction to Notion API Integration
Notion is a versatile all-in-one workspace that allows teams to organize work, manage projects, and store information in a highly customizable way. With its robust features for pages, databases, and user management, Notion has become a popular choice for businesses looking to streamline their workflows.
Integrating with the Notion API enables developers to programmatically interact with Notion workspaces, allowing for seamless automation and data synchronization. For example, a developer might use the Notion API to retrieve records from a Notion database and display them on a custom dashboard, enhancing data visibility and accessibility.
This article will guide you through using PHP to interact with the Notion API, focusing on retrieving records from a Notion database. By following this tutorial, you'll learn how to set up your environment, authenticate with OAuth, and make API calls to efficiently access and manage your Notion data.
Setting Up Your Notion Test or Sandbox Account
Before you can start interacting with the Notion API using PHP, you'll need to set up a Notion account and create an integration. This process involves creating a Notion workspace, setting up an integration, and obtaining the necessary credentials for OAuth authentication.
Create a Notion Account and Workspace
If you don't already have a Notion account, you can sign up for free on the Notion website. Once your account is created, you'll be able to set up a workspace where you can manage your pages and databases.
Setting Up a Notion Integration
To interact with the Notion API, you need to create an integration. Follow these steps to set up your integration:
- Navigate to the My Integrations page in your Notion account.
- Click on New Integration to create a new integration.
- Fill in the required details, such as the integration name and associated workspace.
- Ensure that you select the appropriate permissions for your integration, such as read content capabilities.
- Once created, you'll receive a client ID and client secret, which are essential for OAuth authentication.
Obtaining Your Integration Token
After setting up your integration, you'll need to obtain the integration token to authenticate your API requests. Here's how:
- Go to the integration settings page.
- Locate the integration token under the Configuration tab.
- Copy the token and store it securely, as you'll need it for API requests.
Sharing Notion Resources with Your Integration
To allow your integration to access specific pages or databases, you must share these resources with the integration:
- Open the page or database you want to share in your Notion workspace.
- Click on the ••• menu at the top right corner.
- Select Add connections and choose your integration from the dropdown list.
By following these steps, you'll have your Notion account and integration set up, ready to make authenticated API calls using PHP.
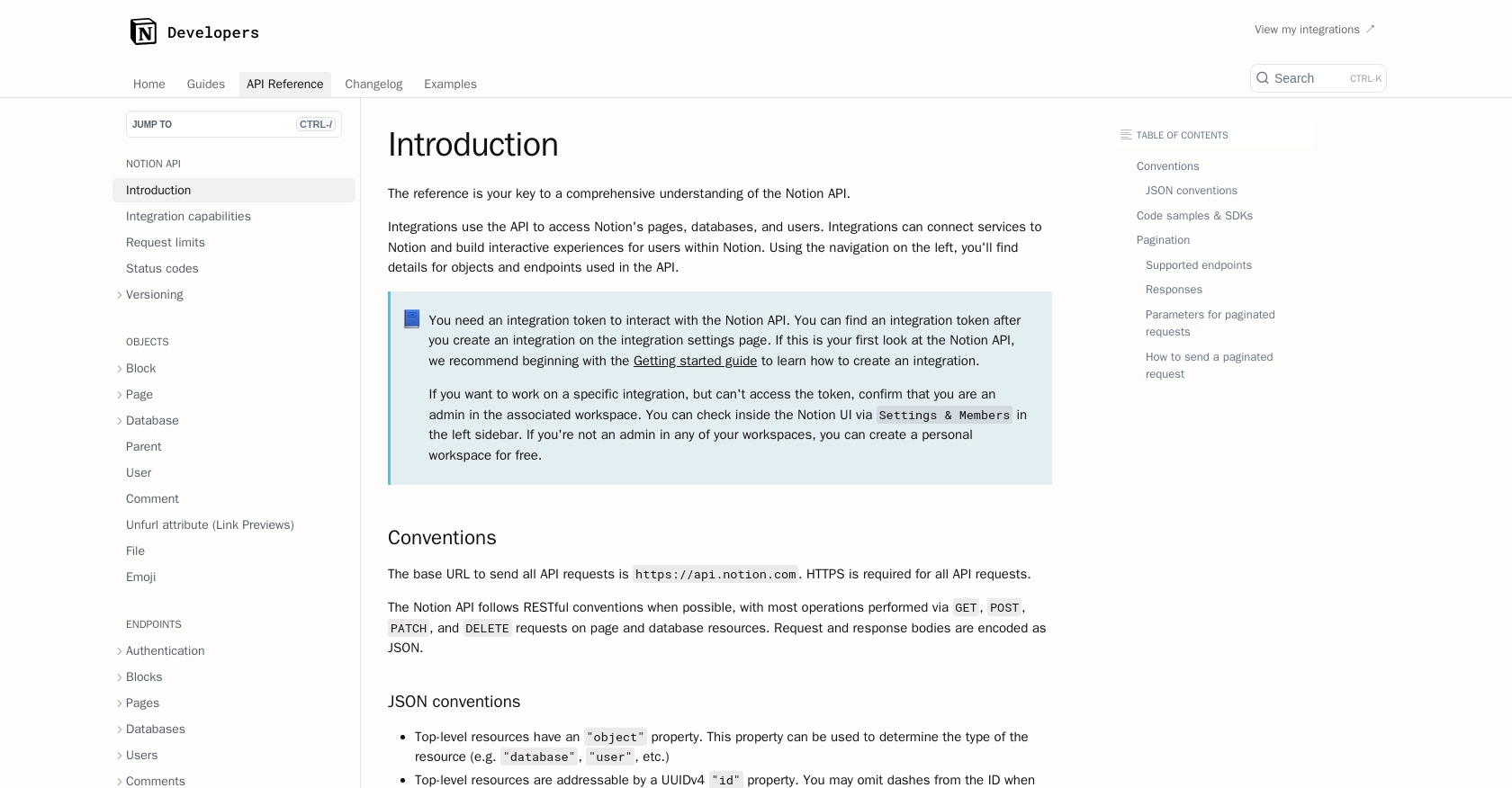
sbb-itb-96038d7
Making API Calls to Retrieve Notion Database Records Using PHP
To interact with the Notion API using PHP, you'll need to set up your environment and make authenticated API calls. This section will guide you through the process of retrieving records from a Notion database, ensuring you have the necessary tools and understanding to manage your data effectively.
Setting Up Your PHP Environment for Notion API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL
extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure
cURL
is installed by checking your PHP configuration withphp -m
.
Installing Required PHP Dependencies for Notion API
To simplify HTTP requests, you can use the Guzzle library. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Records from Notion Database
With your environment set up, you can now write PHP code to interact with the Notion API. Below is a sample script to retrieve records from a Notion database:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$databaseId = 'your_database_id';
$token = 'your_integration_token';
$response = $client->request('POST', "https://api.notion.com/v1/databases/{$databaseId}/query", [
'headers' => [
'Authorization' => "Bearer {$token}",
'Content-Type' => 'application/json',
'Notion-Version' => '2022-06-28'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['results'] as $page) {
echo "Page ID: " . $page['id'] . "\n";
// Add more logic to handle the retrieved data
}
Replace your_database_id
and your_integration_token
with your actual database ID and integration token.
Understanding the Notion API Response and Handling Errors
After executing the API call, the response will contain the records from your Notion database. You can parse and manipulate this data as needed. Ensure you handle potential errors by checking the response status codes:
- 200: Success - The request was successful.
- 400: Invalid request - Check your request parameters.
- 401: Unauthorized - Verify your integration token.
- 429: Rate limited - Reduce request frequency.
For more details on error codes, refer to the Notion API status codes documentation.
Verifying API Call Success in Notion Workspace
To confirm that your API call was successful, check your Notion workspace for the retrieved data. If you encounter issues, ensure your integration has the necessary permissions and that the database is shared with your integration.
By following these steps, you can efficiently retrieve and manage records from your Notion database using PHP. For more information on querying databases, visit the Notion API documentation.
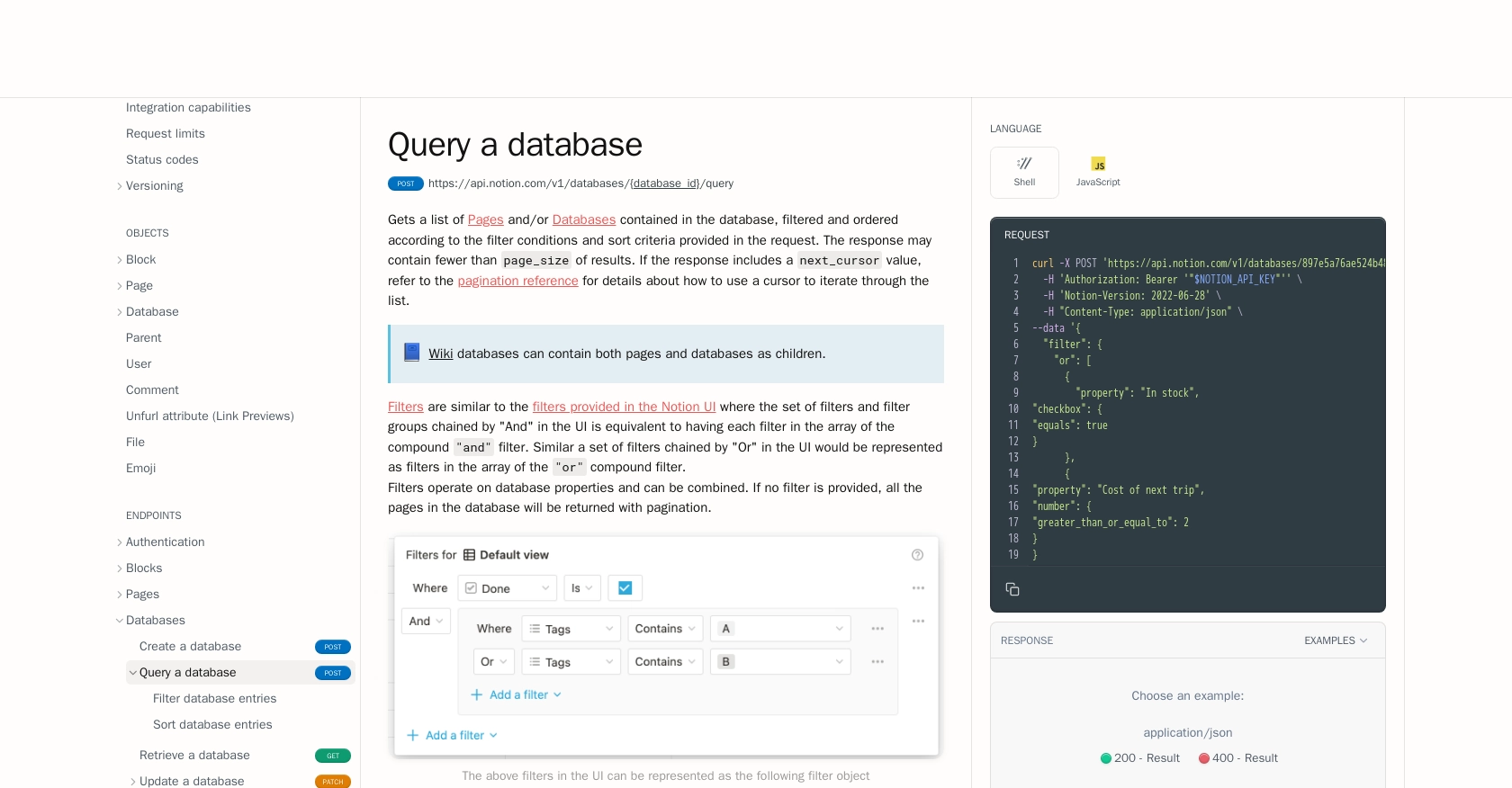
Conclusion and Best Practices for Using the Notion API with PHP
Integrating with the Notion API using PHP allows developers to automate workflows and enhance data accessibility within Notion workspaces. By following the steps outlined in this article, you can efficiently retrieve and manage records from Notion databases, leveraging the power of PHP and the Notion API.
Best Practices for Secure and Efficient Notion API Integration
- Secure Storage of Credentials: Always store your integration token and client credentials securely. Use environment variables or a secret manager to prevent unauthorized access.
- Handle Rate Limiting: The Notion API has a rate limit of three requests per second. Implement logic to handle HTTP 429 responses and respect the
Retry-After
header to avoid disruptions. - Data Standardization: Ensure consistent data formats when retrieving and storing information from Notion databases. This will help maintain data integrity across different systems.
Explore More with Endgrate for Seamless Integration Solutions
While integrating with the Notion API can be straightforward, managing multiple integrations across various platforms can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your product offerings.
Read More
- https://endgrate.com/provider/Notion
- https://developers.notion.com/reference/intro
- https://developers.notion.com/docs/getting-started
- https://developers.notion.com/docs/authorization
- https://developers.notion.com/reference/request-limits
- https://developers.notion.com/reference/status-codes
- https://developers.notion.com/reference/post-database-query
- https://developers.notion.com/reference/post-search
Ready to get started?