Using the Nutshell API to Get Contacts in Javascript
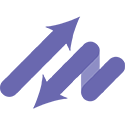
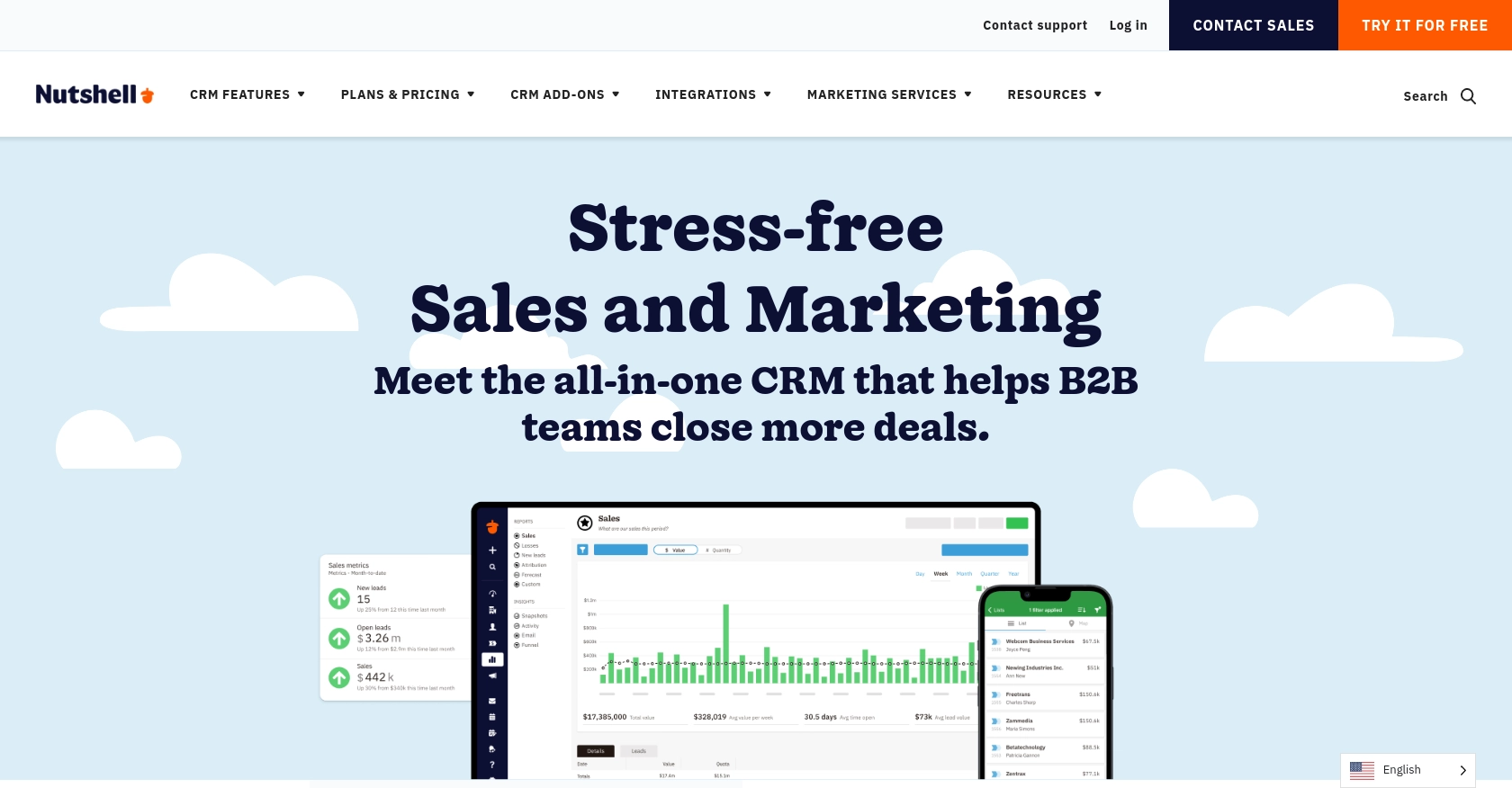
Introduction to Nutshell CRM
Nutshell is a powerful CRM platform designed to help businesses manage their sales processes efficiently. With its user-friendly interface and robust features, Nutshell enables companies to streamline their customer relationship management, making it a popular choice for businesses looking to enhance their sales operations.
Developers may want to integrate with Nutshell's API to access and manage customer data, such as contacts, to improve sales strategies and customer interactions. For example, a developer could use the Nutshell API to retrieve contact information and integrate it with other applications to provide personalized customer experiences.
Setting Up Your Nutshell Test Account
Before you can start integrating with the Nutshell API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Nutshell Account
If you don't already have a Nutshell account, you can sign up for a free trial on the Nutshell website. Follow the instructions to create your account, and you'll be ready to start testing.
Generating an API Key for Authentication
To interact with the Nutshell API, you'll need an API key for authentication. Follow these steps to generate one:
- Log in to your Nutshell account.
- Navigate to the Setup tab.
- Click on API to access the API settings.
- Create a new API key with the necessary permissions for accessing contacts.
- Copy the API key and store it securely, as you'll need it for authentication in your API calls.
Understanding Nutshell's Authentication Method
Nutshell uses HTTP Basic authentication for its API. You'll need to include the API key in the Authentication header of your requests. The username for authentication is either your company's domain or a specific user's email address, and the password is the API key.
Building the Endpoint URL
Once you have your API key, you need to construct the endpoint URL for your API calls. Follow these steps:
- Use the domain name provided by the
getApiForUsername
method to determine the correct domain for your account. - Prefix the domain with
https://
and append/api/v1/json
to form the complete endpoint URL.
For example, if your domain is app.nutshell.com
, your endpoint URL will be https://app.nutshell.com/api/v1/json
.
For more details, refer to the Nutshell API documentation.
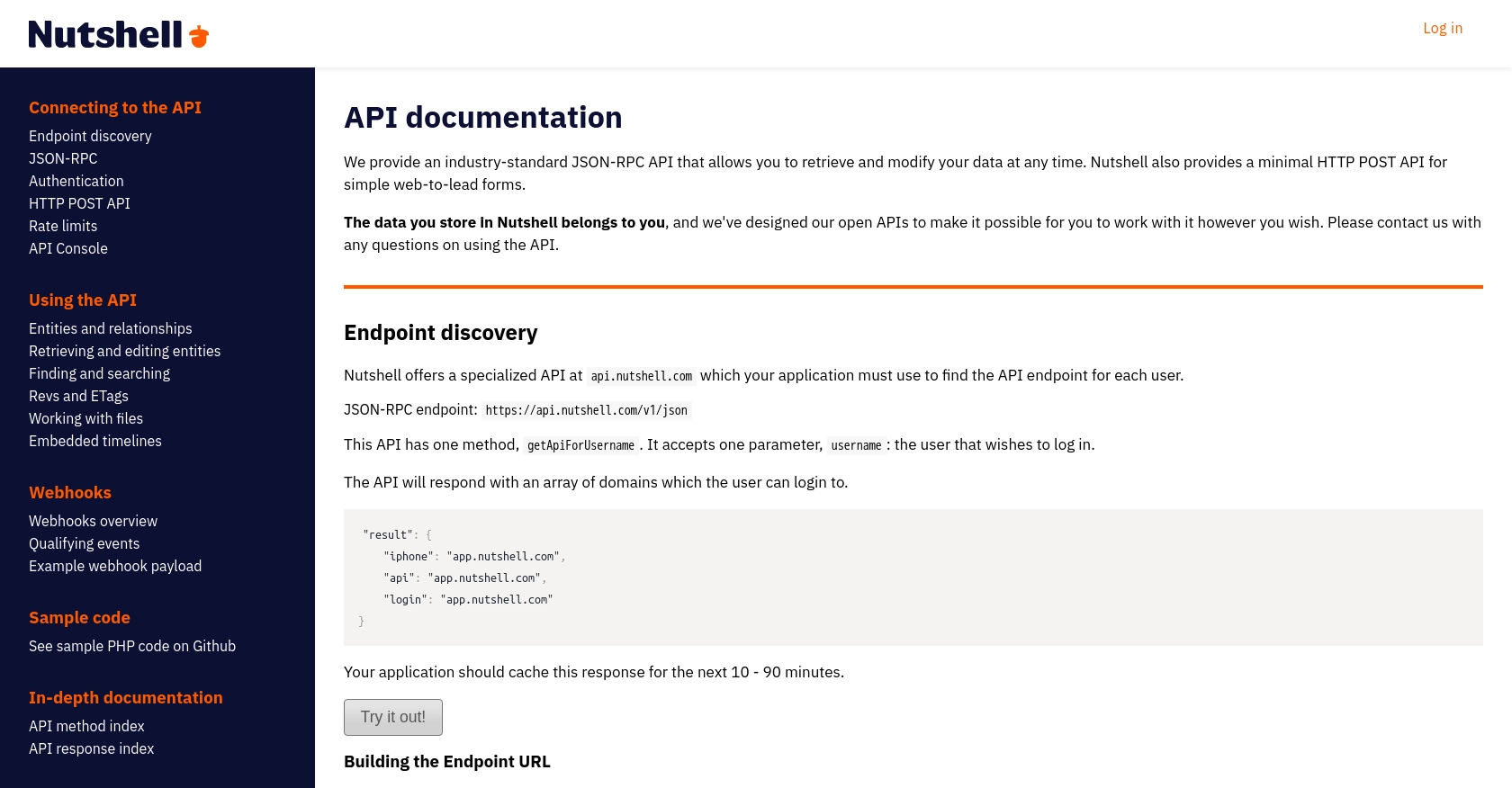
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Nutshell Using JavaScript
Setting Up Your JavaScript Environment for Nutshell API Integration
To interact with the Nutshell API using JavaScript, you'll need to ensure your environment is properly set up. This includes having Node.js installed, which provides the runtime for executing JavaScript code outside of a browser.
Additionally, you'll need to install the axios
library, which simplifies making HTTP requests. You can install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Contacts from Nutshell
Once your environment is ready, you can write the JavaScript code to make API calls to Nutshell. Below is an example of how to retrieve contacts using the Nutshell API:
const axios = require('axios');
// Set the API endpoint and authentication details
const endpoint = 'https://app.nutshell.com/api/v1/json';
const username = 'your_company_domain_or_email';
const apiKey = 'your_api_key';
// Configure the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Basic ${Buffer.from(`${username}:${apiKey}`).toString('base64')}`
};
// Define the request payload for retrieving contacts
const payload = {
method: 'findContacts',
params: {
orderBy: 'id',
orderDirection: 'ASC',
limit: 10,
page: 1
},
id: 1
};
// Make the API call
axios.post(endpoint, payload, { headers })
.then(response => {
const contacts = response.data.result;
console.log('Retrieved Contacts:', contacts);
})
.catch(error => {
console.error('Error fetching contacts:', error);
});
Replace your_company_domain_or_email
and your_api_key
with your actual Nutshell domain or email and API key.
Understanding the API Response and Handling Errors
Upon a successful API call, the response will contain an array of contact objects. You can iterate over this array to access individual contact details.
If the request fails, the error handling block will log the error details. Common issues include incorrect authentication credentials or network errors.
Verifying API Call Success in Nutshell
To verify that your API call was successful, you can log into your Nutshell account and check the contacts section. The contacts retrieved by your API call should match those displayed in the Nutshell interface.
For more details on handling errors and understanding the API response, refer to the Nutshell API documentation.
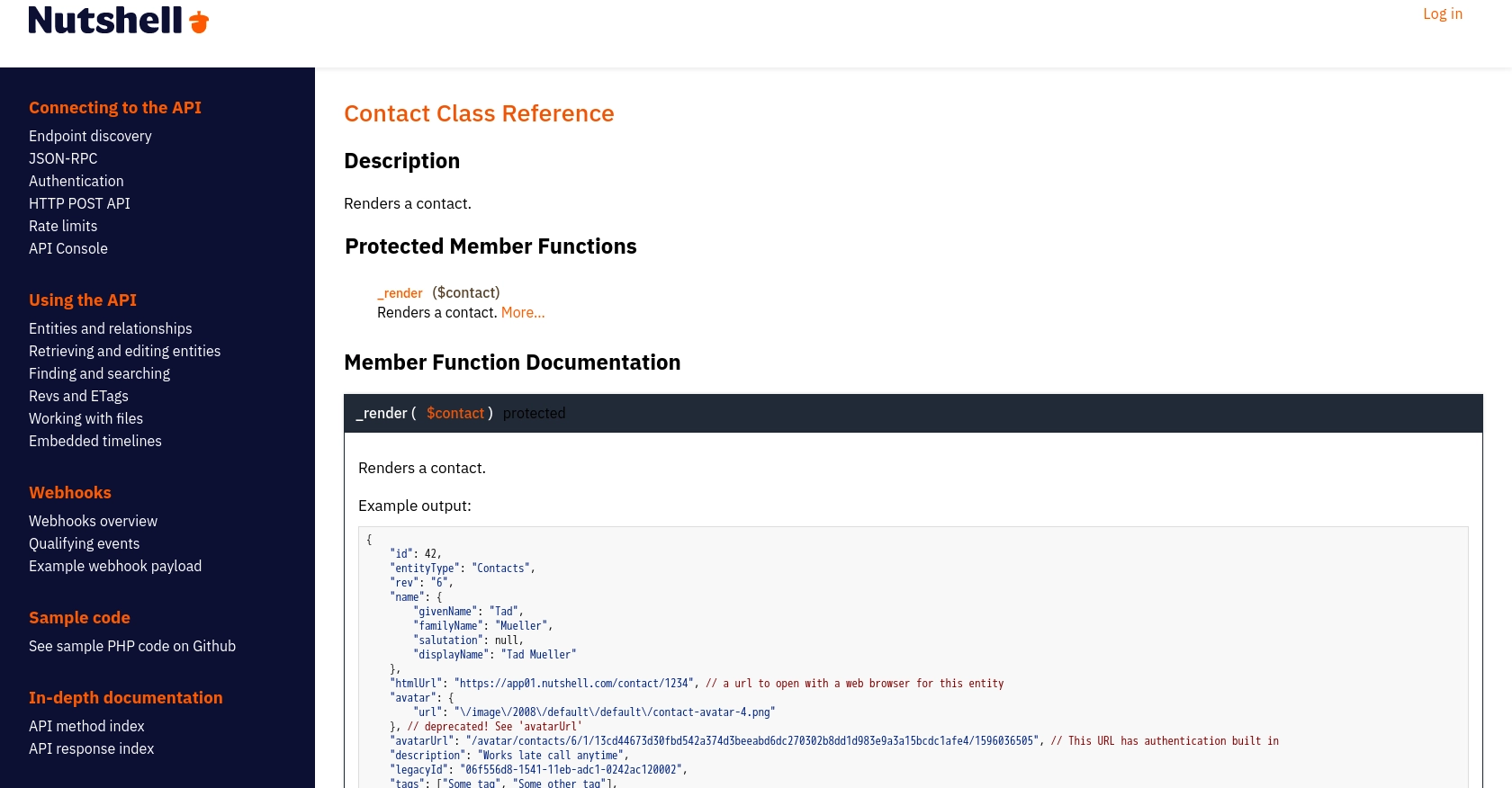
Best Practices for Using the Nutshell API in JavaScript
When working with the Nutshell API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store API Credentials: Always store your API key securely and avoid hardcoding it in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that data retrieved from Nutshell is standardized and transformed as needed to integrate seamlessly with other systems.
- Implement Error Handling: Robust error handling is crucial. Log errors and provide meaningful feedback to users when API calls fail.
Enhancing Your Integration Experience with Endgrate
Building and maintaining integrations can be time-consuming and complex. By leveraging Endgrate, you can streamline this process and focus on your core product development. Endgrate provides a unified API endpoint that connects to multiple platforms, including Nutshell, allowing you to build once and deploy across various services.
With Endgrate, you can offer your customers an intuitive integration experience, saving time and resources. Explore how Endgrate can enhance your integration capabilities by visiting Endgrate.
Read More
Ready to get started?