Using the Odoo Online API to Create or Update Records in Javascript
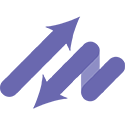
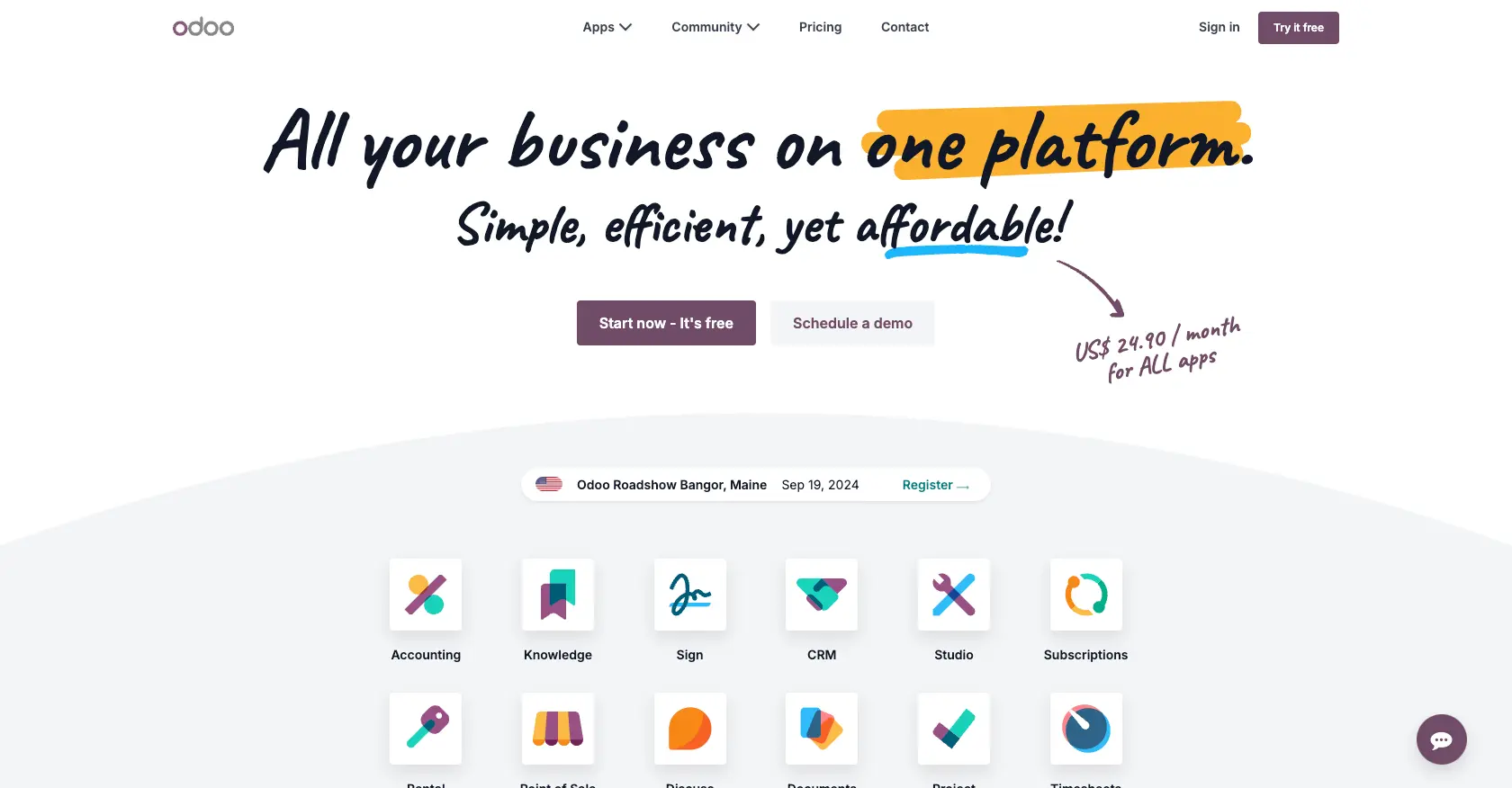
Introduction to Odoo Online
Odoo Online is a comprehensive suite of business applications designed to streamline operations across various departments, including sales, inventory, accounting, and human resources. Its modular approach allows businesses to customize their software stack according to their specific needs, making it a popular choice among enterprises seeking flexibility and scalability.
Developers may want to integrate with Odoo Online's API to automate and enhance business processes. For example, using the Odoo Online API, a developer can create or update records in real-time, such as synchronizing product inventory levels between Odoo and an e-commerce platform. This integration can significantly improve operational efficiency and data accuracy.
Setting Up Your Odoo Online Test/Sandbox Account
Before you can start integrating with the Odoo Online API, you need to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data.
Creating an Odoo Online Account
If you don't already have an Odoo Online account, you can sign up for a free trial on the Odoo website. Follow the on-screen instructions to create your account. Once your account is set up, you'll have access to a sandbox environment where you can test API interactions.
Generating API Credentials for Odoo Online
Odoo Online uses a custom authentication method to secure API access. To interact with the API, you'll need to generate the necessary credentials.
- Log in to your Odoo Online account.
- Navigate to the "Settings" section from the main dashboard.
- Under "Users & Companies," select "Users" to manage user access.
- Create a new user or select an existing user to generate API credentials.
- Ensure that the user has the necessary permissions to access the API.
- Save the user details and note down the API key or token provided.
Configuring OAuth for Odoo Online API Access
For applications requiring OAuth-based authentication, you will need to create an app within your Odoo Online account:
- Go to the "Integrations" section in your Odoo Online dashboard.
- Select "OAuth Apps" and click on "Create App."
- Fill in the required details, such as the app name and redirect URI.
- Once the app is created, you'll receive a client ID and client secret.
- Use these credentials to authenticate API requests via OAuth.
With your sandbox account and API credentials ready, you can now proceed to make API calls to create or update records in Odoo Online using JavaScript.
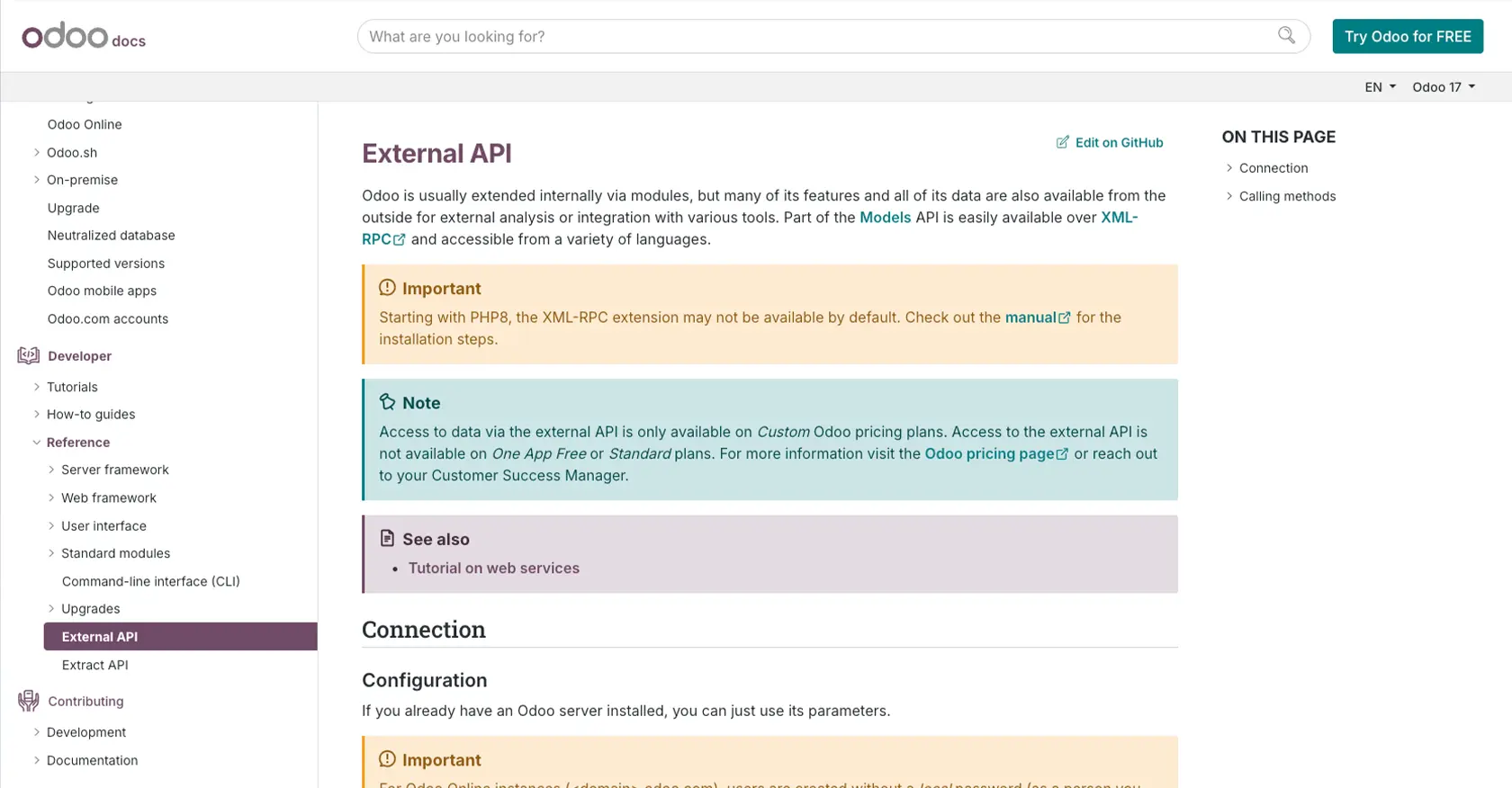
sbb-itb-96038d7
Making API Calls to Odoo Online Using JavaScript
To interact with the Odoo Online API using JavaScript, you'll need to ensure you have the right setup and dependencies. This section will guide you through the process of making API calls to create or update records in Odoo Online.
Setting Up Your JavaScript Environment for Odoo Online API
Before making API calls, ensure you have a modern JavaScript environment set up. This includes having Node.js installed, which provides the runtime for executing JavaScript code outside a browser.
- Download and install Node.js if you haven't already.
- Use npm (Node Package Manager) to install necessary packages. For HTTP requests, you can use the popular
axios
library:
npm install axios
Creating or Updating Records in Odoo Online
With your environment ready, you can now proceed to make API calls. Below is a sample code snippet demonstrating how to create or update records in Odoo Online using JavaScript and the axios
library.
const axios = require('axios');
// Set your Odoo Online API endpoint
const apiUrl = 'https://your-odoo-instance.odoo.com/api/endpoint';
// Set your API credentials
const apiKey = 'Your_API_Key';
// Define the data for creating or updating a record
const data = {
model: 'product.product',
fields: {
name: 'New Product',
list_price: 100.0
}
};
// Make a POST request to create or update a record
axios.post(apiUrl, data, {
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log('Record created/updated successfully:', response.data);
})
.catch(error => {
console.error('Error creating/updating record:', error.response ? error.response.data : error.message);
});
In this code:
- Replace
Your_API_Key
with the API key generated from your Odoo Online account. - Specify the appropriate
apiUrl
for the Odoo Online API endpoint you wish to interact with. - The
data
object contains the model and fields you want to create or update.
Verifying API Call Success and Handling Errors
After executing the API call, you should verify the success of the operation:
- Check the response data to confirm the record has been created or updated as expected.
- In case of errors, the
catch
block will log the error details. Ensure you handle errors gracefully to improve user experience.
By following these steps, you can efficiently create or update records in Odoo Online using JavaScript, enhancing your application's integration capabilities.
Conclusion and Best Practices for Odoo Online API Integration
Integrating with the Odoo Online API using JavaScript offers a powerful way to automate and enhance your business processes. By following the steps outlined in this guide, you can efficiently create or update records, ensuring seamless data synchronization across platforms.
Best Practices for Secure and Efficient Odoo Online API Usage
- Secure Storage of API Credentials: Always store your API keys and tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Odoo Online's rate limits to avoid service disruptions. Implement retry logic with exponential backoff to manage API call limits effectively.
- Data Standardization: Ensure consistent data formats when interacting with Odoo Online to maintain data integrity and avoid errors during API calls.
- Error Handling: Implement robust error handling to manage API call failures gracefully. Log errors for troubleshooting and provide user-friendly messages to enhance the user experience.
Enhancing Integration Capabilities with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API solution that simplifies the process. By leveraging Endgrate, you can focus on your core product while outsourcing complex integrations, saving time and resources.
Endgrate's intuitive platform allows you to build once for each use case, reducing the need for repetitive integration efforts. Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?