Using the Outreach API to Get Prospects in Python
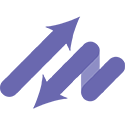
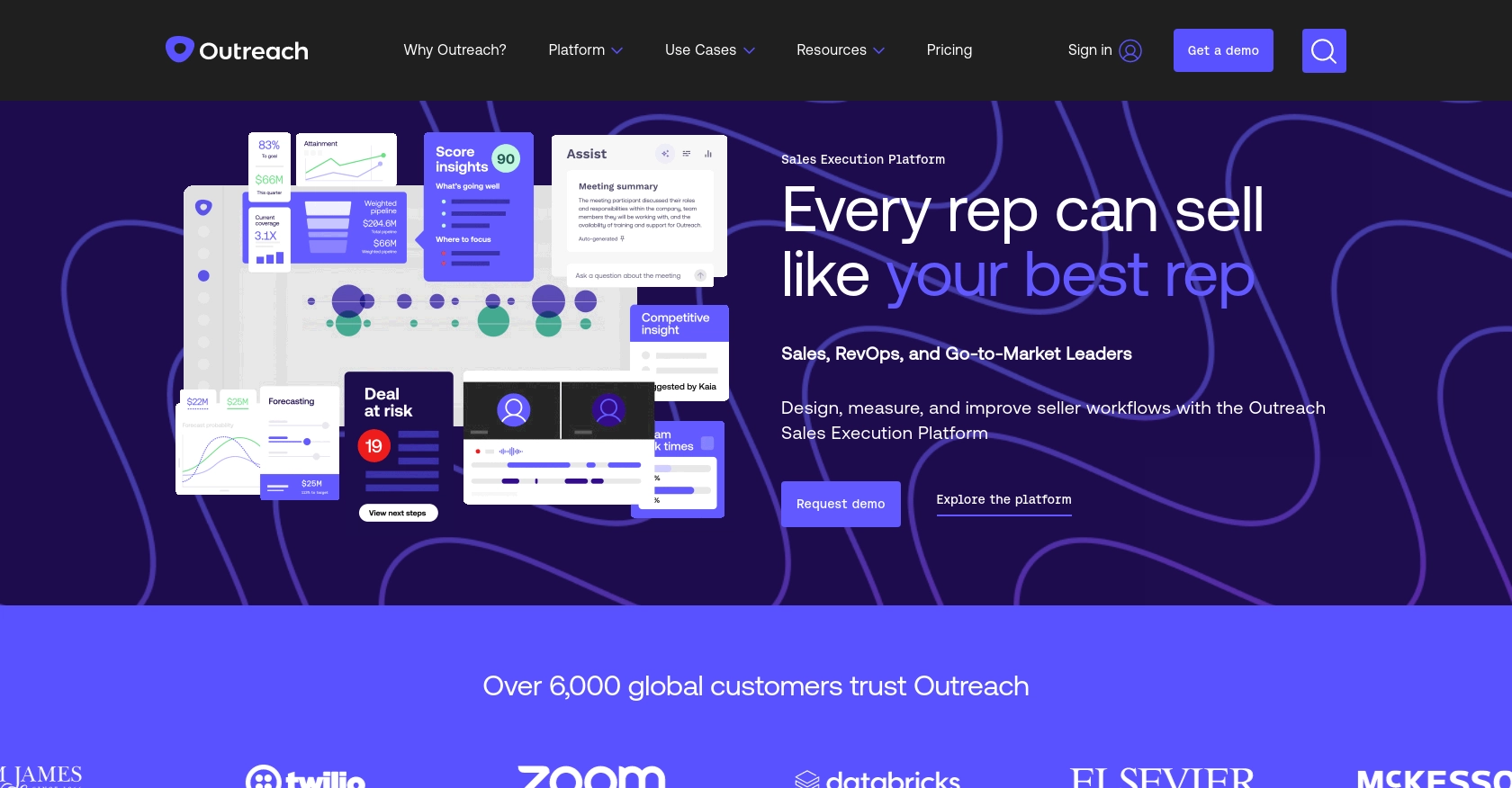
Introduction to Outreach API
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. With features like email tracking, task automation, and analytics, Outreach enables sales teams to engage with prospects more effectively and efficiently.
Integrating with the Outreach API allows developers to access and manage prospect data, automate workflows, and enhance sales strategies. For example, a developer might use the Outreach API to retrieve prospect information and integrate it with a CRM system, ensuring that sales teams have up-to-date data for targeted outreach campaigns.
Setting Up Your Outreach Test/Sandbox Account
Before you can start using the Outreach API to retrieve prospects, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your production data.
Create an Outreach Developer Account
If you don't already have an Outreach account, you can sign up for a developer account on the Outreach website. This account will give you access to the necessary tools and resources to create and manage your applications.
- Visit the Outreach Developer Portal.
- Follow the instructions to create a new account.
- Once your account is created, log in to access the developer dashboard.
Set Up an Outreach App for OAuth Authentication
To interact with the Outreach API, you'll need to create an app that uses OAuth 2.0 for authentication. This involves generating client credentials and setting up the necessary permissions.
- Navigate to the "My Apps" section in the developer dashboard.
- Click on "Create New App" and fill in the required details.
- Specify the redirect URIs and select the OAuth scopes your application will need. Make sure to include scopes like
prospects.read
for accessing prospect data. - Save your app to generate the client ID and client secret.
Obtain OAuth Credentials
Once your app is set up, you'll need to obtain OAuth credentials to authenticate API requests.
- Redirect users to the following URL to obtain an authorization code:
- After the user authorizes the app, they will be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token using the following POST request:
- Store the access token securely, as it will be used to authenticate your API requests.
https://api.outreach.io/oauth/authorize?client_id=<Your_Client_ID>&redirect_uri=<Your_Redirect_URI>&response_type=code&scope=prospects.read
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<Your_Client_ID> \
-d client_secret=<Your_Client_Secret> \
-d redirect_uri=<Your_Redirect_URI> \
-d grant_type=authorization_code \
-d code=<Authorization_Code>
For more detailed information on setting up OAuth, refer to the Outreach OAuth Documentation.
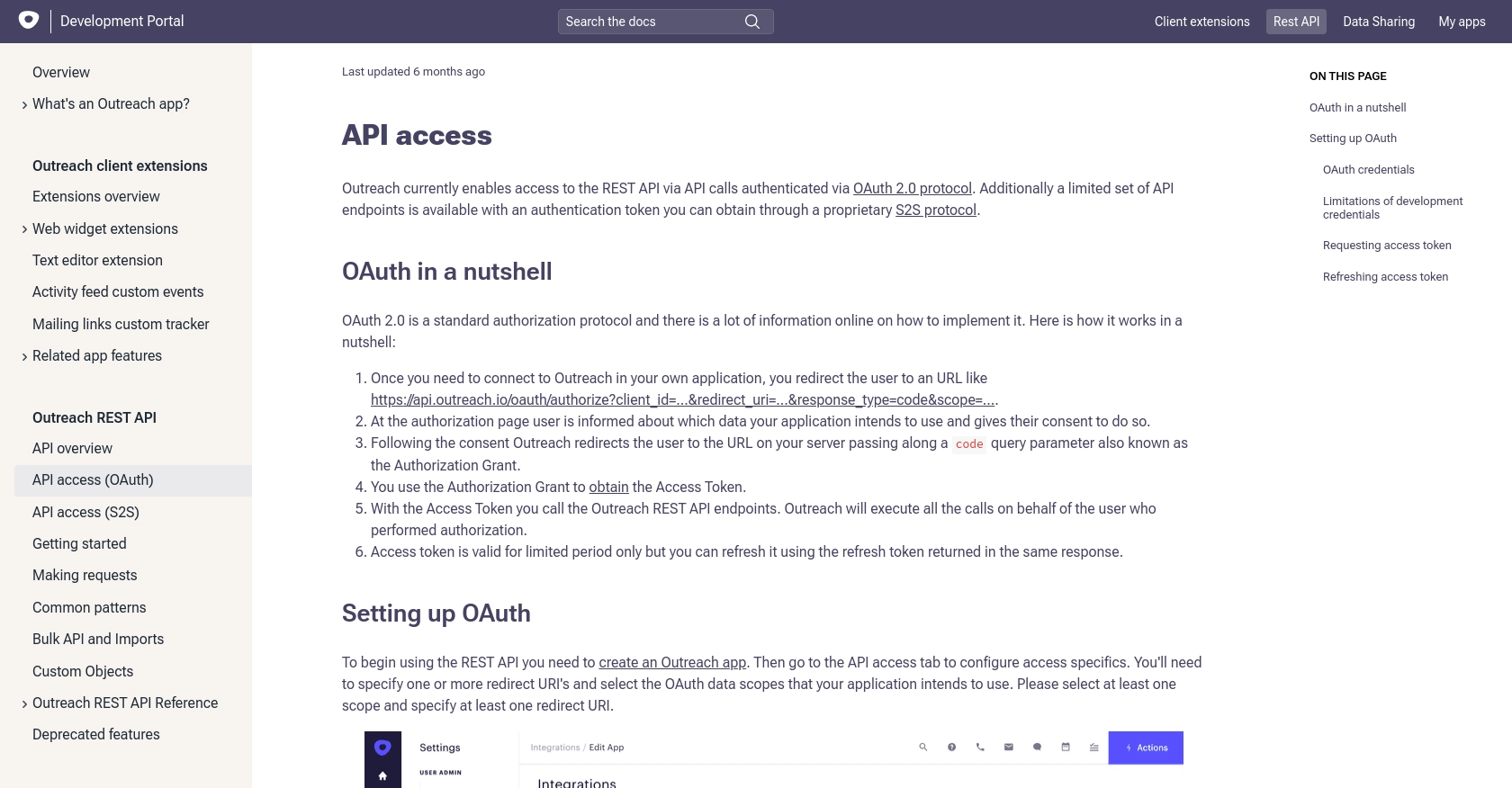
sbb-itb-96038d7
Making API Calls to Retrieve Prospects Using the Outreach API in Python
To interact with the Outreach API and retrieve prospect data, you'll need to make authenticated API calls using Python. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Outreach API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests to the Outreach API:
pip install requests
Writing Python Code to Retrieve Prospects from Outreach
Create a new Python file named get_outreach_prospects.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.outreach.io/api/v2/prospects"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/vnd.api+json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the prospects and print their information
for prospect in data["data"]:
print(prospect["attributes"]["firstName"], prospect["attributes"]["lastName"])
else:
print("Failed to retrieve prospects:", response.status_code, response.text)
Replace Your_Access_Token
with the access token obtained during the OAuth authentication process.
Running the Code and Verifying the Output
Execute the script from your terminal or command line:
python get_outreach_prospects.py
If successful, you should see a list of prospect names printed to the console. If there are any issues, the script will output the error code and message.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The Outreach API may return various HTTP status codes, such as:
- 200 OK: The request was successful.
- 401 Unauthorized: The access token is invalid or expired.
- 403 Forbidden: The request lacks the necessary permissions.
- 429 Too Many Requests: The rate limit has been exceeded. The Outreach API allows up to 10,000 requests per hour.
For more detailed information on error handling, refer to the Outreach API Documentation.
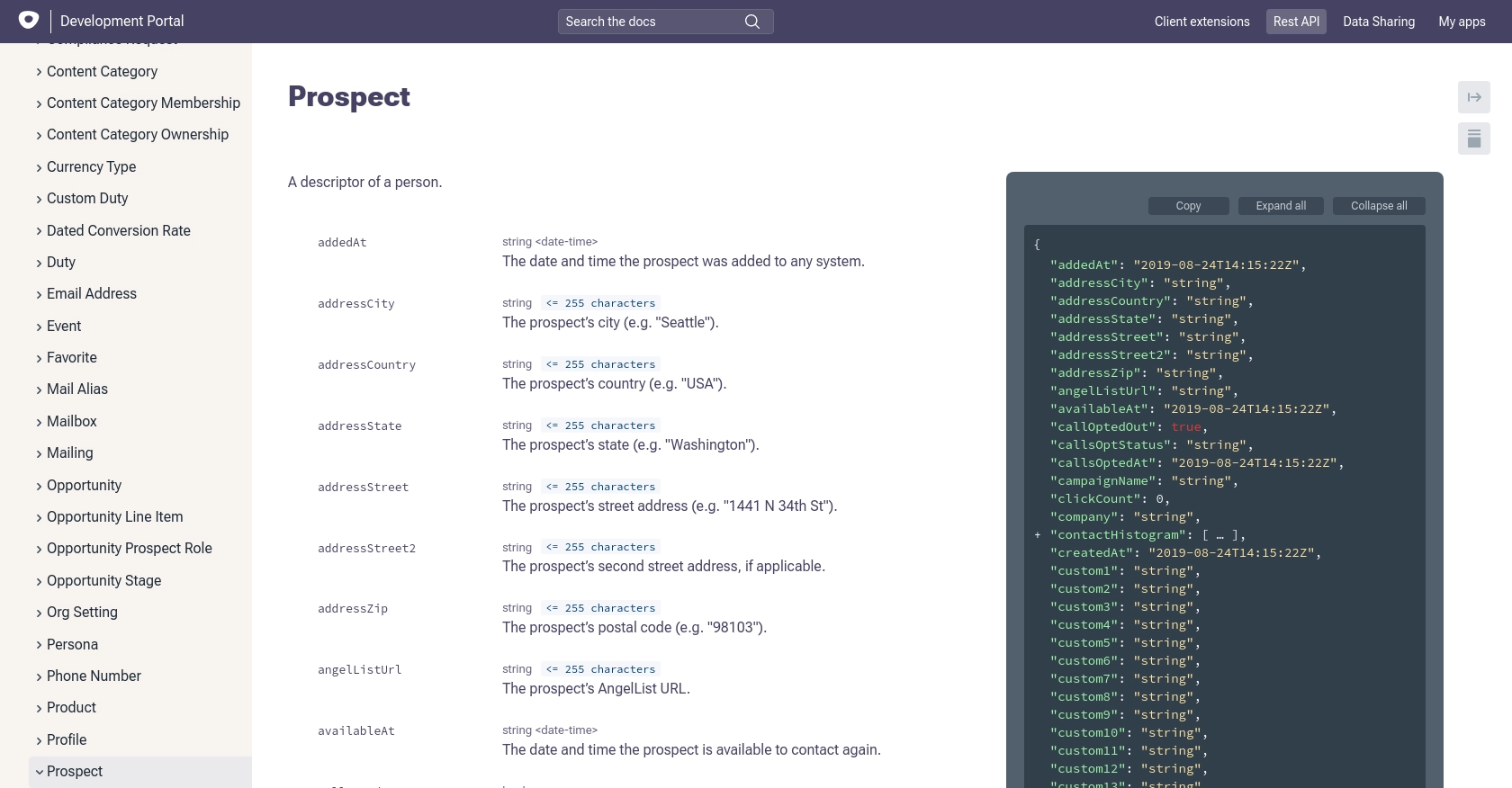
Conclusion and Best Practices for Using the Outreach API in Python
Integrating with the Outreach API using Python can significantly enhance your sales engagement strategies by providing seamless access to prospect data. By following the steps outlined in this guide, you can efficiently retrieve and manage prospects, ensuring your sales team has the most up-to-date information for targeted outreach campaigns.
Best Practices for Secure and Efficient API Integration with Outreach
- Secure Storage of Credentials: Always store your OAuth credentials securely. Consider using environment variables or secure vaults to protect sensitive information like client secrets and access tokens.
- Handling Rate Limits: Be mindful of the Outreach API's rate limit of 10,000 requests per hour. Implement logic to handle
429 Too Many Requests
errors by retrying requests after the rate limit resets. - Refreshing Access Tokens: Access tokens are short-lived. Use the refresh token to obtain new access tokens before they expire to maintain uninterrupted access to the API.
- Data Standardization: Standardize and transform data fields as needed to ensure compatibility with your existing systems and workflows.
Enhancing Integration Capabilities with Endgrate
While integrating with the Outreach API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outreach. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
Ready to get started?