Using the Todoist API to Create Tasks in Javascript
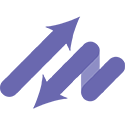
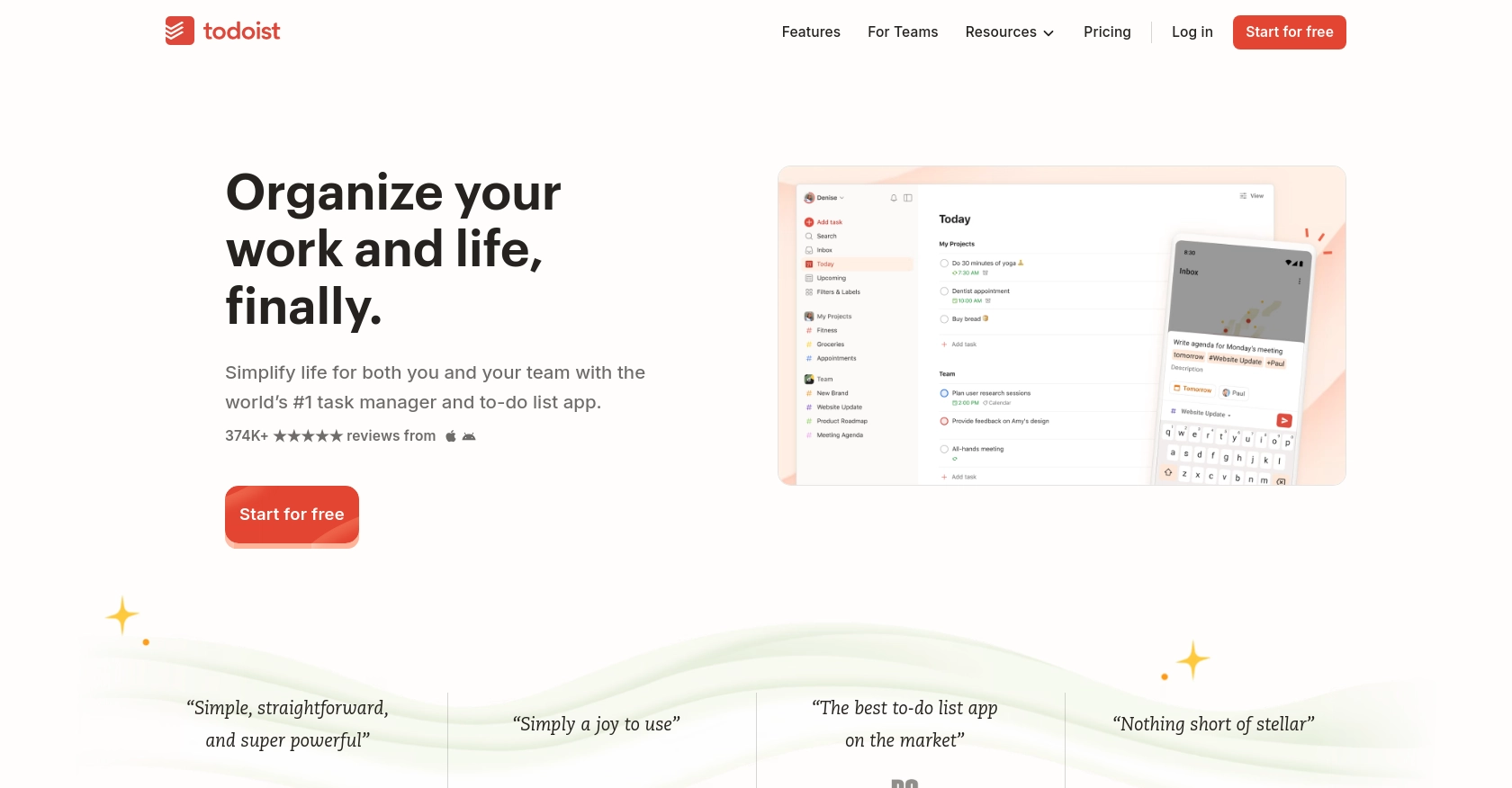
Introduction to Todoist API for Task Management
Todoist is a powerful task management platform that helps individuals and teams organize their tasks and projects efficiently. With its intuitive interface and robust features, Todoist is a popular choice for users looking to enhance productivity and streamline their workflow.
Integrating with the Todoist API allows developers to automate task management processes, such as creating, updating, and managing tasks programmatically. For example, a developer might use the Todoist API to automatically create tasks based on incoming emails or project management updates, ensuring that no important task is overlooked.
This article will guide you through using JavaScript to interact with the Todoist API, specifically focusing on creating tasks. By the end of this tutorial, you'll be able to seamlessly integrate Todoist's task management capabilities into your applications.
Setting Up Your Todoist Developer Account for API Integration
Before you can start creating tasks using the Todoist API, you'll need to set up a developer account and obtain the necessary credentials for authentication. Todoist uses OAuth for secure access, allowing you to interact with the API on behalf of users.
Creating a Todoist Developer Account
If you don't have a Todoist account, begin by signing up for a free account on the Todoist website. Once your account is set up, you can proceed to create a developer account to access the API.
- Visit the Todoist Developer Portal.
- Log in using your Todoist account credentials.
- Navigate to the "Manage App" section to create a new application.
Registering Your Application for OAuth Authentication
To interact with the Todoist API, you'll need to register your application and obtain an OAuth client ID and client secret. Follow these steps to set up your application:
- In the "Manage App" section, click on "Create App."
- Fill in the required details, such as the application name and description.
- Specify the redirect URI, which is the URL to which users will be redirected after authorizing your application.
- Submit the form to create your application.
Once your application is created, you'll receive a client ID and client secret. Keep these credentials secure, as they are essential for authenticating API requests.
Obtaining a User Token for API Requests
To make authorized API calls, you'll need a user token. This token can be obtained by implementing the OAuth flow:
- Direct users to the Todoist authorization URL with your client ID and redirect URI.
- Upon user consent, Todoist will redirect to your specified URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the Todoist token endpoint.
For detailed instructions on implementing OAuth, refer to the Todoist API documentation.
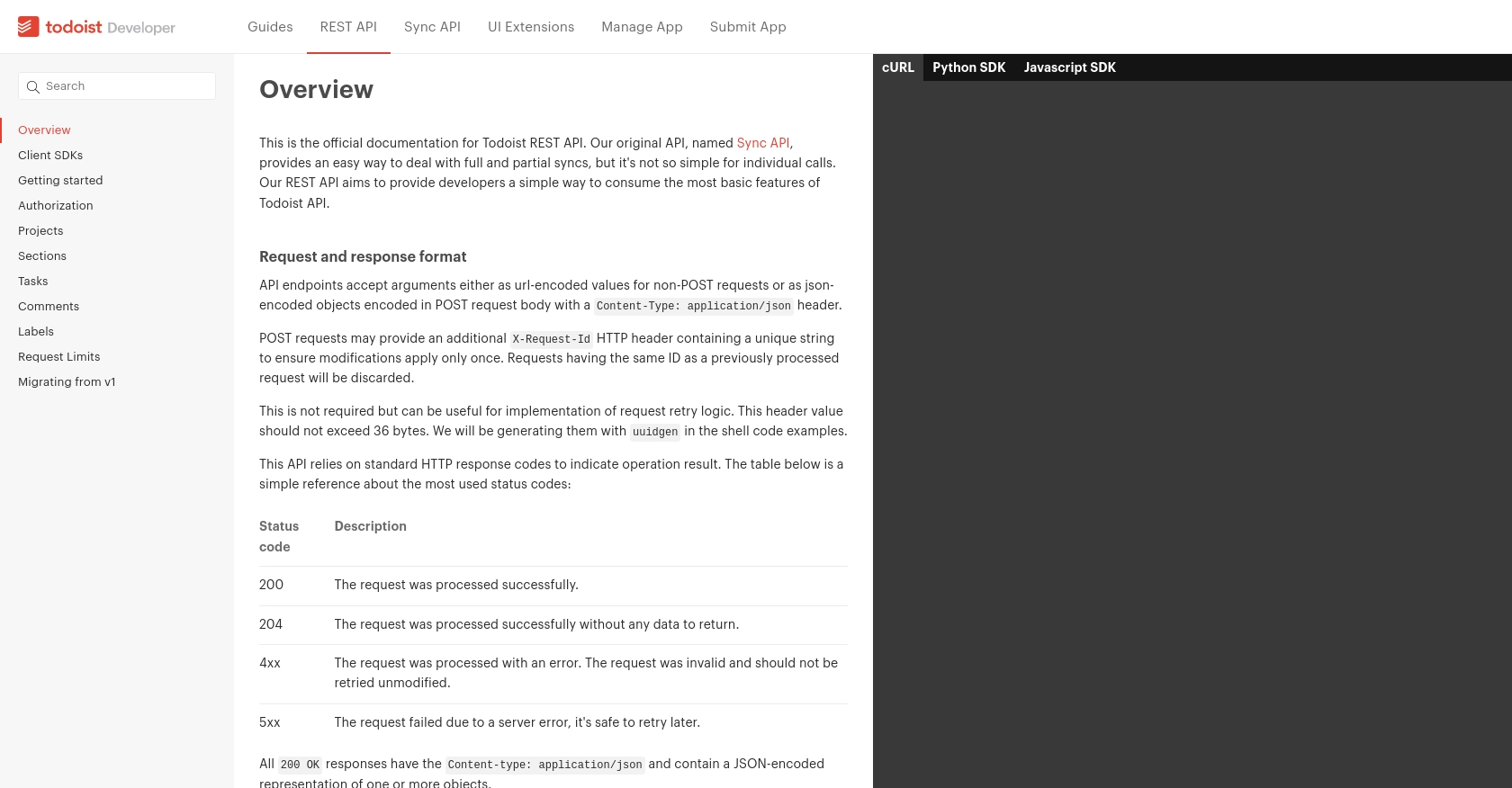
sbb-itb-96038d7
Making API Calls to Create Tasks in Todoist Using JavaScript
To create tasks in Todoist using JavaScript, you'll need to interact with the Todoist API. This involves setting up your development environment, installing necessary dependencies, and writing code to make API requests. Let's walk through the process step-by-step.
Setting Up Your JavaScript Environment for Todoist API Integration
Before making API calls, ensure you have Node.js installed on your machine. You'll also need npm, the Node package manager, to install the Todoist JavaScript SDK.
- Download and install Node.js if you haven't already.
- Open your terminal and run the following command to install the Todoist JavaScript SDK:
npm install @doist/todoist-api-typescript
Writing JavaScript Code to Create Tasks with Todoist API
Now that your environment is set up, you can write JavaScript code to create tasks in Todoist. Below is a sample script to demonstrate how to do this:
import { TodoistApi } from "@doist/todoist-api-typescript";
// Initialize the Todoist API with your access token
const api = new TodoistApi('your_access_token_here');
// Function to create a new task
async function createTask() {
try {
const task = await api.addTask({
content: 'Complete the project report',
dueString: 'tomorrow at 5pm',
priority: 4
});
console.log('Task created successfully:', task);
} catch (error) {
console.error('Error creating task:', error);
}
}
// Call the function to create a task
createTask();
Replace your_access_token_here
with the access token obtained through the OAuth flow. This script initializes the Todoist API client and defines a function to create a new task with specified content, due date, and priority.
Verifying Task Creation in Todoist Sandbox Environment
After running the script, you can verify the task creation by checking your Todoist account. The new task should appear in your task list with the specified details. If the task does not appear, ensure your access token is correct and that the API call was successful.
Handling Errors and Understanding Todoist API Response Codes
When making API calls, it's crucial to handle potential errors. The Todoist API uses standard HTTP response codes to indicate the result of an operation:
- 200 OK: The request was processed successfully.
- 204 No Content: The request was successful, but there is no content to return.
- 4xx Client Error: The request was invalid. Check the request parameters and try again.
- 5xx Server Error: The request failed due to a server error. Retry the request later.
For more detailed information on error handling, refer to the Todoist API documentation.
Conclusion and Best Practices for Using Todoist API in JavaScript
Integrating the Todoist API into your JavaScript applications can significantly enhance task management capabilities, allowing for seamless automation and improved productivity. By following the steps outlined in this guide, you can efficiently create tasks and manage them programmatically.
Best Practices for Secure and Efficient Todoist API Integration
- Secure Storage of Credentials: Always store your OAuth client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Todoist API allows a maximum of 1000 requests per user within a 15-minute period. Implement logic to handle rate limiting gracefully, such as exponential backoff or queuing requests.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across your application. This includes using consistent date formats and task priorities.
- Error Handling: Implement robust error handling to manage API response codes effectively. This will help you identify and resolve issues quickly, ensuring a smooth user experience.
Leverage Endgrate for Streamlined Integration Solutions
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Todoist, allowing you to focus on your core product while outsourcing integration complexities. Visit Endgrate to learn more about how it can enhance your integration strategy.
By adopting these best practices and leveraging tools like Endgrate, you can ensure a secure, efficient, and scalable integration with the Todoist API, ultimately enhancing your application's functionality and user satisfaction.
Read More
Ready to get started?