Using the Pipedrive API to Create or Update Deals in Javascript
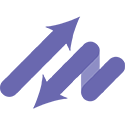
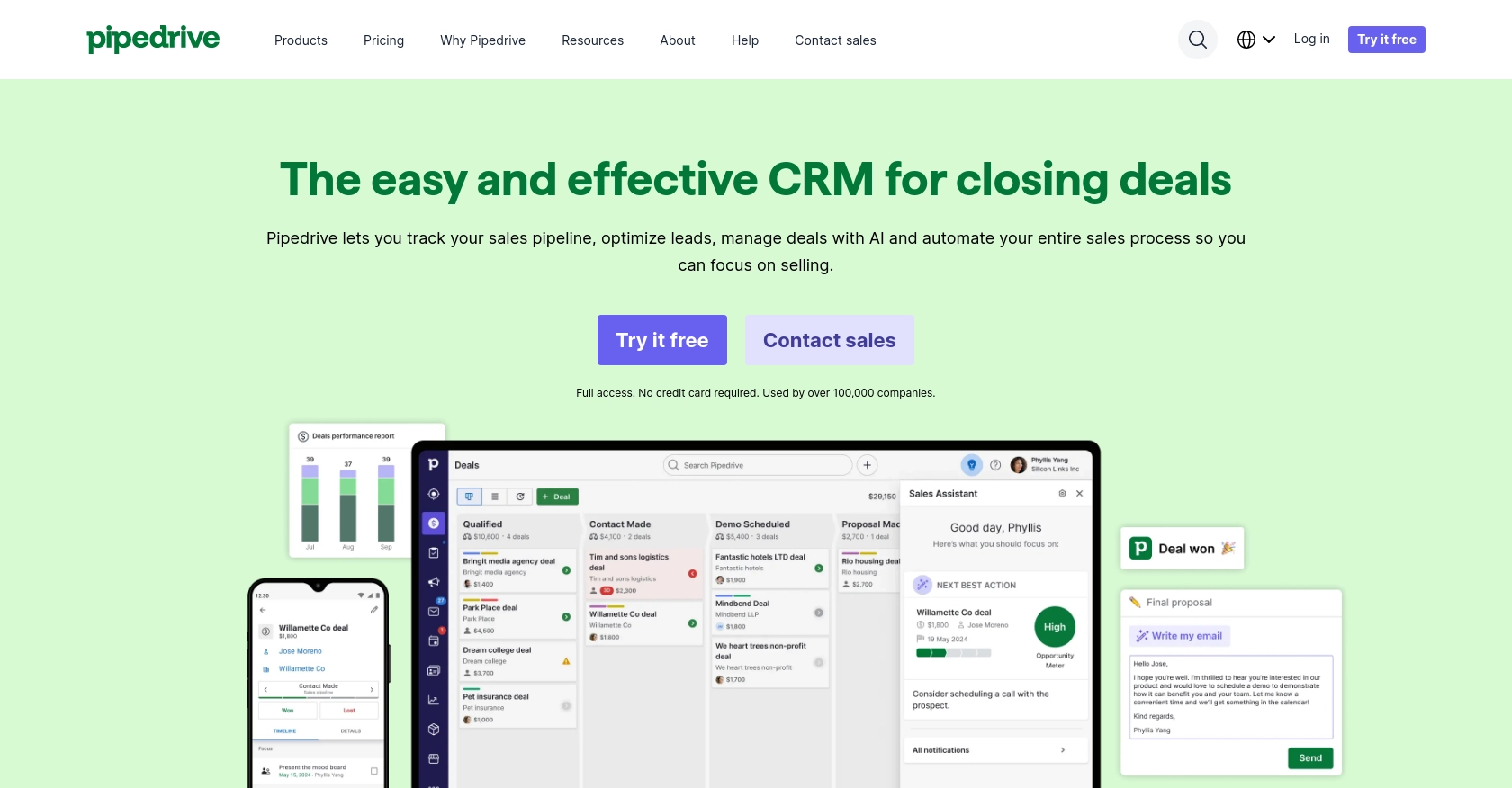
Introduction to Pipedrive CRM and API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes efficiently. With its intuitive interface and robust features, Pipedrive enables sales teams to track deals, manage contacts, and automate workflows, making it a popular choice for businesses looking to enhance their sales operations.
Integrating with Pipedrive's API allows developers to automate and streamline sales processes by interacting directly with the CRM's data. For example, using the Pipedrive API, developers can create or update deals programmatically, ensuring that sales data is always up-to-date and reducing manual data entry. This can be particularly useful for businesses that need to sync their sales data across multiple platforms or automate deal management based on specific triggers.
In this article, we will explore how to use JavaScript to interact with the Pipedrive API, focusing on creating and updating deals. This integration can help developers build seamless sales automation solutions that enhance productivity and accuracy in managing sales pipelines.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you'll need to set up a developer sandbox account. This account provides a risk-free environment where you can test and develop your applications without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To get started, follow these steps to create your sandbox account:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account will allow you to perform testing and development in a safe environment.
- Once your account is set up, log in to access the Developer Hub, where you can manage your apps and integrations.
Generating OAuth Credentials for Pipedrive API
The Pipedrive API uses OAuth 2.0 for authentication. To create an app and obtain the necessary credentials, follow these steps:
- Navigate to the Developer Hub within your sandbox account.
- Register your app by providing the required details. This will generate a client ID and client secret, which are essential for OAuth authentication.
- Ensure your app includes proper installation flows to handle user permissions and scopes. For more information, refer to the Pipedrive app creation guide.
With your sandbox account and OAuth credentials ready, you can now proceed to make API calls to create or update deals in Pipedrive using JavaScript.
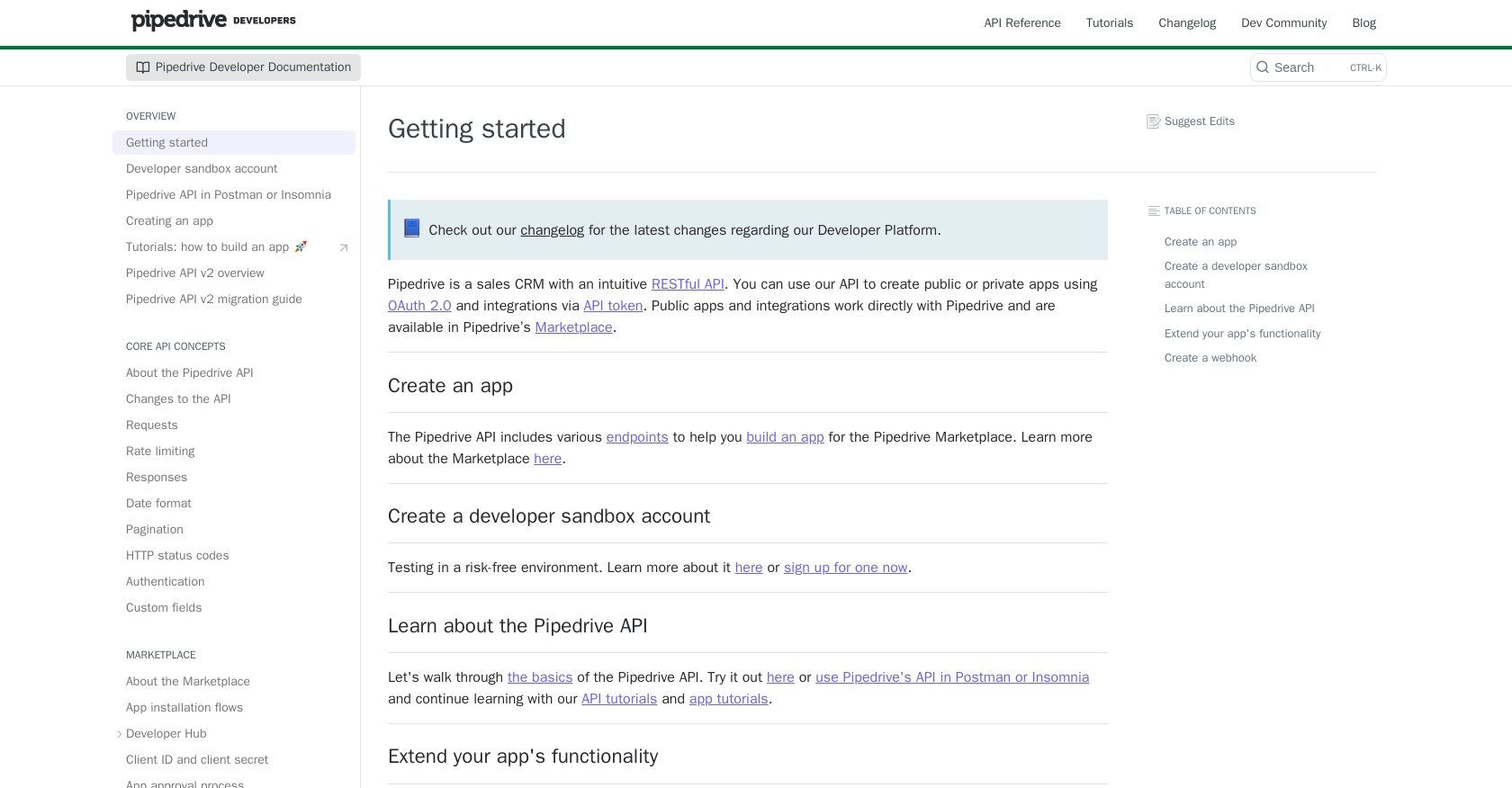
sbb-itb-96038d7
Making API Calls to Pipedrive for Creating or Updating Deals Using JavaScript
To interact with the Pipedrive API using JavaScript, you need to set up your environment and write the necessary code to create or update deals. This section will guide you through the process, including setting up your JavaScript environment, making API calls, and handling responses.
Setting Up Your JavaScript Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14.x or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it:
mkdir pipedrive-integration
cd pipedrive-integration
npm init -y
Next, install the axios
library to handle HTTP requests:
npm install axios
Creating a Deal in Pipedrive Using JavaScript
To create a new deal in Pipedrive, use the following JavaScript code. Replace Your_Access_Token
with your OAuth access token:
const axios = require('axios');
const createDeal = async () => {
const url = 'https://api.pipedrive.com/v1/deals';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
const data = {
title: 'New Deal',
value: 1000,
currency: 'USD'
};
try {
const response = await axios.post(url, data, { headers });
console.log('Deal Created:', response.data);
} catch (error) {
console.error('Error creating deal:', error.response.data);
}
};
createDeal();
Run the script using the following command:
node createDeal.js
Upon successful execution, the console should display the details of the newly created deal. Verify the creation by checking your Pipedrive sandbox account.
Updating an Existing Deal in Pipedrive Using JavaScript
To update an existing deal, use the following code. Replace Your_Access_Token
and Deal_ID
with your OAuth access token and the ID of the deal you wish to update:
const axios = require('axios');
const updateDeal = async (dealId) => {
const url = `https://api.pipedrive.com/v1/deals/${dealId}`;
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
const data = {
title: 'Updated Deal Title',
value: 1500
};
try {
const response = await axios.put(url, data, { headers });
console.log('Deal Updated:', response.data);
} catch (error) {
console.error('Error updating deal:', error.response.data);
}
};
updateDeal(Deal_ID);
Run the script using the following command:
node updateDeal.js
Check your Pipedrive sandbox account to ensure the deal has been updated with the new information.
Handling Errors and Rate Limiting in Pipedrive API
When making API calls, it's crucial to handle potential errors and respect Pipedrive's rate limits. The Pipedrive API allows up to 80 requests per 2 seconds per access token for the Essential plan. If you exceed this limit, you will receive a 429 "Too Many Requests" error.
To handle errors, use try-catch blocks in your code and check the response status codes. For more details on error handling, refer to the Pipedrive HTTP status codes documentation.
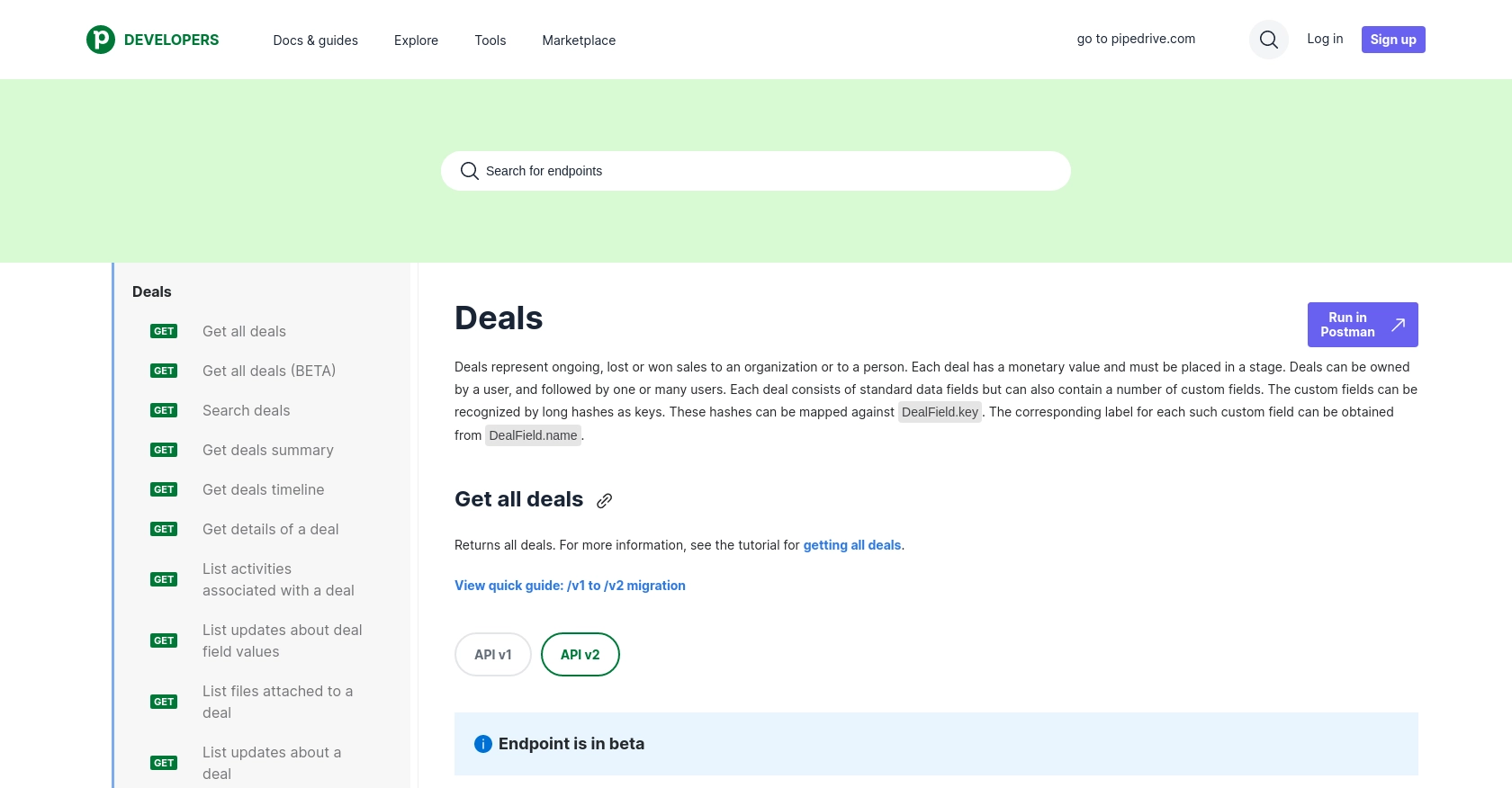
Conclusion and Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API using JavaScript offers a powerful way to automate and enhance your sales processes. By programmatically creating and updating deals, you can ensure that your sales data remains accurate and up-to-date, reducing manual effort and increasing efficiency.
Best Practices for Secure and Efficient Pipedrive API Usage
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Pipedrive's rate limits. Implement retry logic with exponential backoff to handle 429 errors gracefully. For more details, refer to the Pipedrive rate limiting documentation.
- Data Standardization: Ensure consistent data formats when interacting with Pipedrive. This helps in maintaining data integrity across different systems.
- Error Handling: Implement robust error handling by checking HTTP status codes and handling exceptions. This ensures your application can recover gracefully from API errors.
Enhance Your Integration Strategy with Endgrate
While building custom integrations with Pipedrive can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution to manage multiple integrations efficiently. By using Endgrate, you can focus on your core product while outsourcing the integration complexities. Explore how Endgrate can simplify your integration strategy by visiting Endgrate.
By following these best practices and leveraging tools like Endgrate, you can create a seamless and efficient integration experience for your team and customers, ultimately driving better sales outcomes.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Deals
- https://developers.pipedrive.com/docs/api/v1/DealFields
Ready to get started?