Using the PipelineCRM API to Create or Update Companies (with Javascript examples)
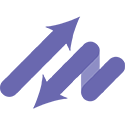
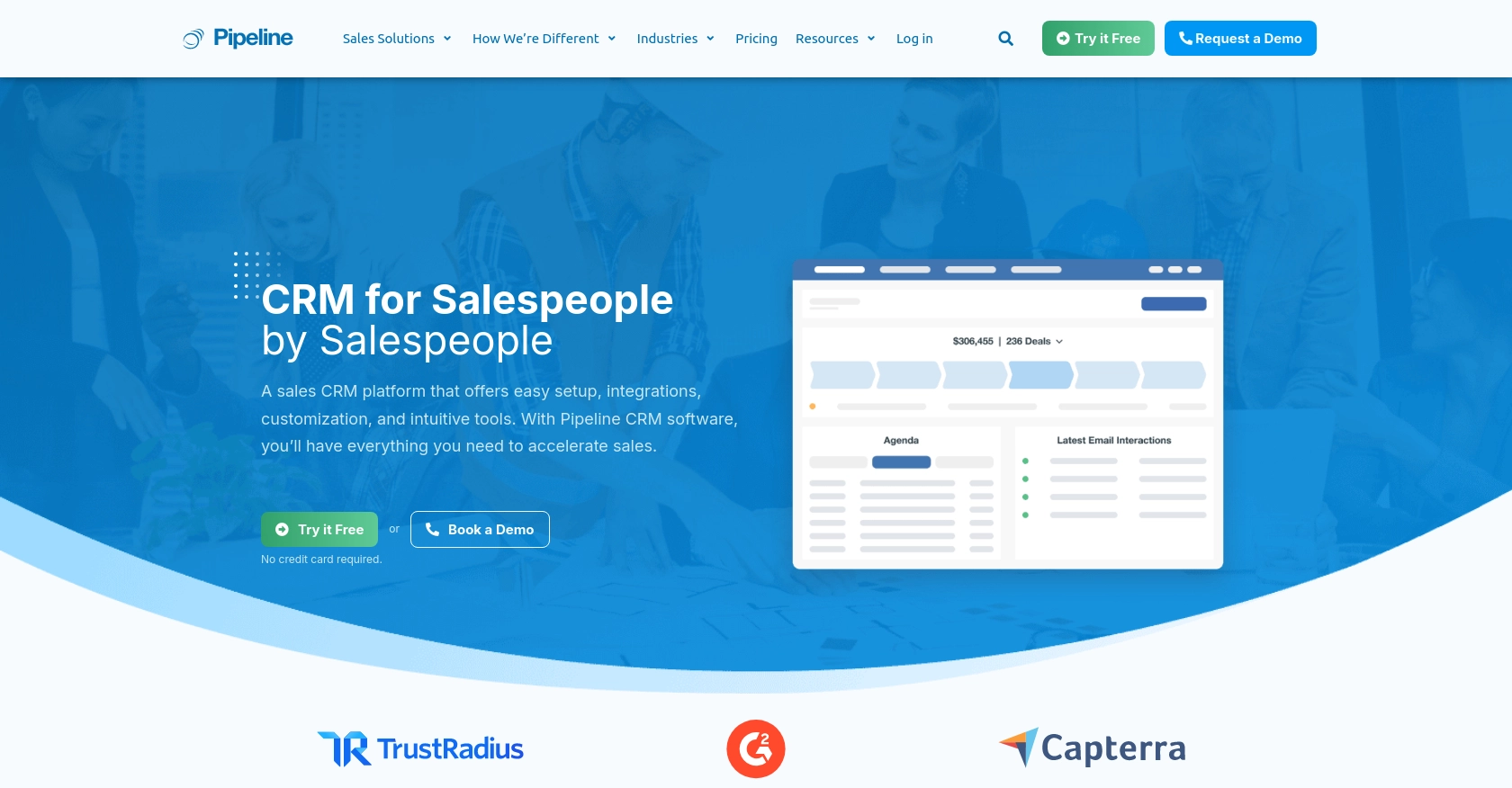
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses streamline their sales processes and manage customer interactions effectively. With features like contact management, sales tracking, and reporting, PipelineCRM empowers sales teams to close deals faster and maintain strong customer relationships.
Developers may want to integrate with PipelineCRM's API to automate and enhance their sales workflows. For example, using the PipelineCRM API, a developer can create or update company records directly from a custom application, ensuring that sales data is always up-to-date and accessible across platforms.
Setting Up Your PipelineCRM Account for API Access
Before you can start integrating with the PipelineCRM API, you'll need to set up your account to access the necessary API credentials. This involves creating a PipelineCRM account and generating an API key, which will be used to authenticate your requests.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, follow these steps to create one:
- Visit the PipelineCRM website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is created, log in to access the PipelineCRM dashboard.
Generating an API Key for PipelineCRM
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account and navigate to the "Settings" section.
- Under "API Access," click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your API key in hand, you're now ready to start making API calls to PipelineCRM. In the next section, we'll explore how to use JavaScript to create or update company records using the PipelineCRM API.
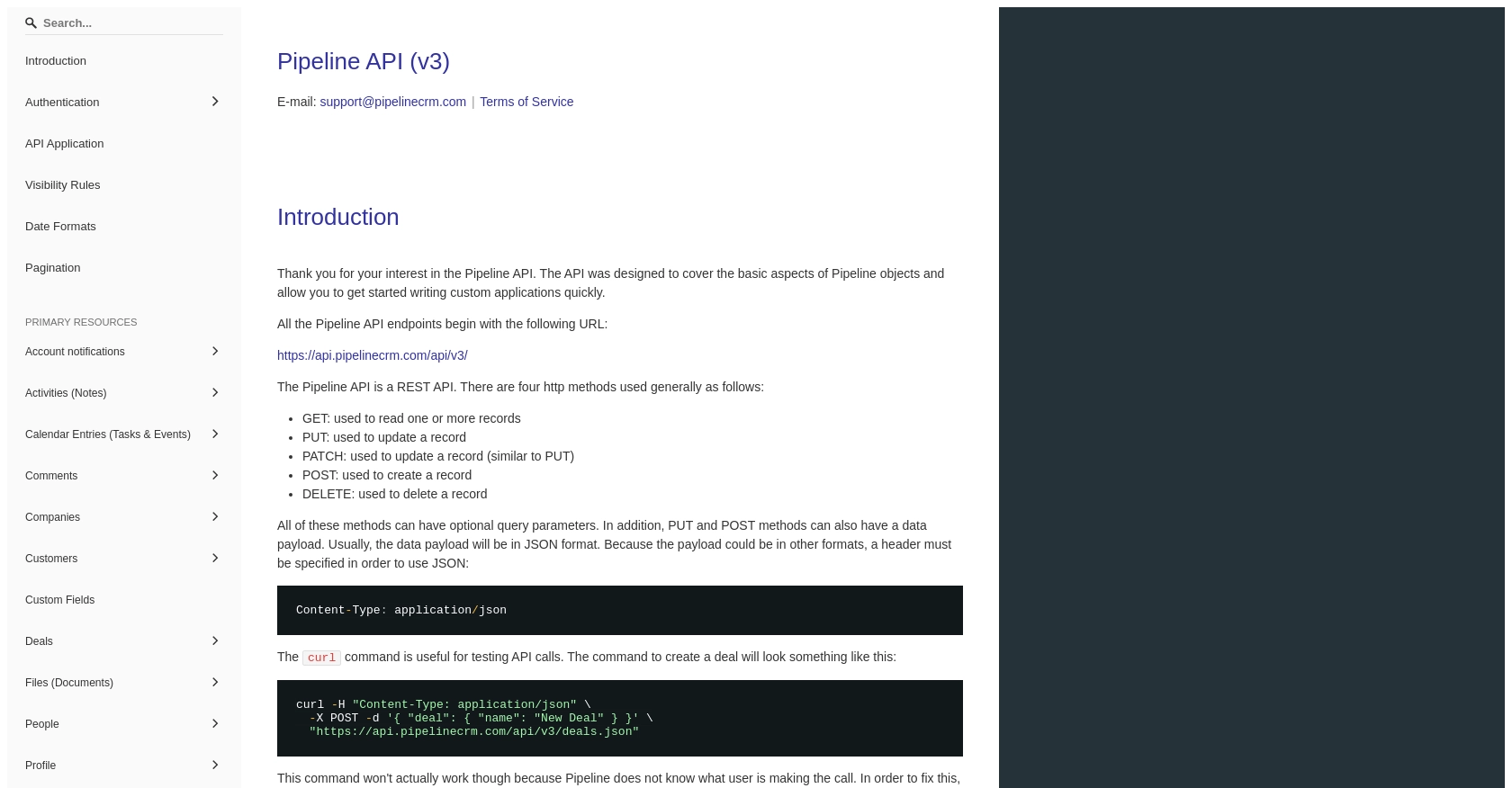
sbb-itb-96038d7
Making API Calls to PipelineCRM Using JavaScript
To interact with the PipelineCRM API and perform operations such as creating or updating company records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment and writing the necessary code.
Setting Up Your JavaScript Environment for PipelineCRM API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Creating or Updating Companies with PipelineCRM API
With your environment ready, you can now write JavaScript code to interact with the PipelineCRM API. Below is an example of how to create or update a company record.
const axios = require('axios');
// Your PipelineCRM API key
const apiKey = 'YOUR_API_KEY';
// Function to create or update a company
async function createOrUpdateCompany(companyData) {
try {
const response = await axios.post('https://api.pipelinecrm.com/v3/companies', companyData, {
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
}
});
console.log('Company created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating company:', error.response ? error.response.data : error.message);
}
}
// Example company data
const companyData = {
name: 'Example Company',
industry: 'Software',
website: 'https://example.com'
};
// Call the function
createOrUpdateCompany(companyData);
In the code above, replace YOUR_API_KEY
with the API key you generated from your PipelineCRM account. The createOrUpdateCompany
function sends a POST request to the PipelineCRM API endpoint to create or update a company record. The companyData
object contains the details of the company you want to create or update.
Verifying API Call Success in PipelineCRM
After running the script, you can verify the success of the API call by checking your PipelineCRM dashboard. The newly created or updated company should appear in your list of companies.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. The example code includes error handling using a try-catch
block. Common error codes you might encounter include:
- 400 Bad Request: The request was invalid. Check the request parameters.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed information on error codes, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for Using the PipelineCRM API
Integrating with the PipelineCRM API using JavaScript can significantly enhance your sales processes by automating the creation and updating of company records. By following the steps outlined in this article, you can ensure that your sales data remains consistent and accessible across platforms.
Best Practices for Secure and Efficient API Integration with PipelineCRM
- Secure API Key Storage: Always store your API key securely and avoid hardcoding it in your source code. Consider using environment variables or a secure vault service.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid being throttled. Implement retry logic with exponential backoff if necessary.
- Data Standardization: Ensure that the data you send to PipelineCRM is standardized to maintain consistency across your applications.
- Error Handling: Implement robust error handling to manage API call failures gracefully and provide meaningful feedback to users.
Streamline Your Integrations with Endgrate
While integrating with PipelineCRM can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint for all your integration needs.
With Endgrate, you can save time and resources by outsourcing your integration efforts, allowing you to focus on your core product. Whether you need to build once for each use case or provide an intuitive integration experience for your customers, Endgrate can help you achieve your goals efficiently.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?