Using the Quickbooks API to Create Or Update Products (with Javascript examples)
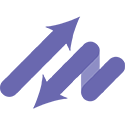
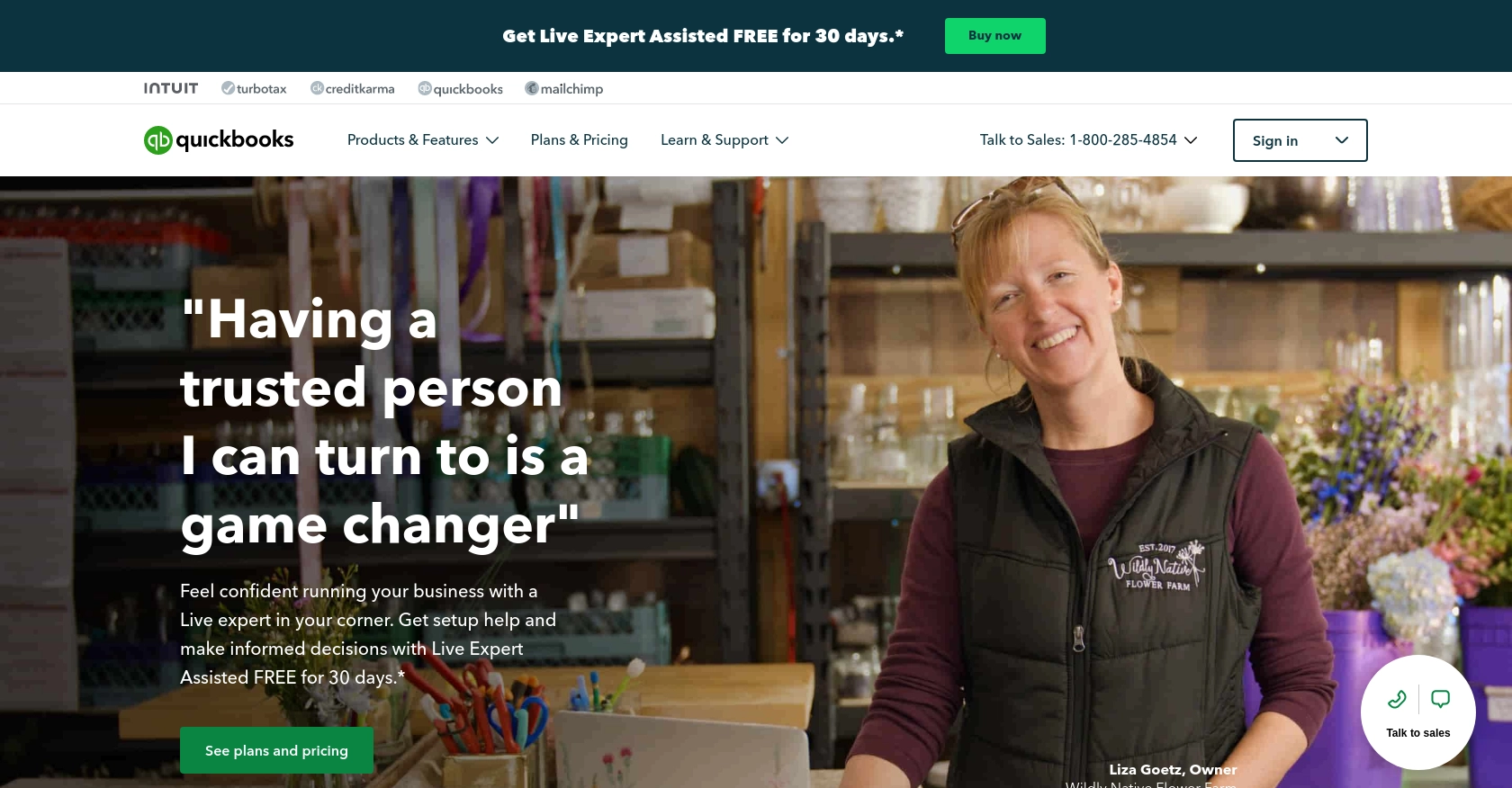
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a comprehensive suite of tools for invoicing, expense tracking, payroll, and more, making it a popular choice for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate and streamline financial processes, such as managing product inventories. For example, a developer might use the QuickBooks API to create or update product information directly from an e-commerce platform, ensuring that inventory levels are always accurate and up-to-date.
This article will guide you through using JavaScript to interact with the QuickBooks API, specifically focusing on creating or updating products. By the end of this tutorial, you'll be equipped with the knowledge to efficiently manage product data within QuickBooks using JavaScript.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you begin interacting with the QuickBooks API, you need to set up a sandbox account. This allows you to test your integration without affecting live data. QuickBooks provides a developer portal where you can create a sandbox environment to simulate real-world scenarios.
Creating a QuickBooks Developer Account
To get started, you'll need a QuickBooks developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you can set up a sandbox company:
- Navigate to the "Dashboard" and select "Sandbox" from the menu.
- Click on "Add Sandbox" to create a new sandbox environment.
- Choose the type of company you want to simulate and complete the setup process.
Creating a QuickBooks App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- In your developer dashboard, go to "My Apps" and click on "Create an App."
- Select "QuickBooks Online and Payments" as the platform.
- Fill in the app details and save your app.
Obtaining Client ID and Client Secret
Once your app is created, you'll need the client ID and client secret for authentication:
- Go to the "Keys & OAuth" section of your app settings.
- Copy the client ID and client secret. These will be used to authenticate API requests.
- Ensure you set the redirect URI to a valid endpoint where you can handle OAuth responses.
For more detailed information, refer to the QuickBooks OAuth Documentation.
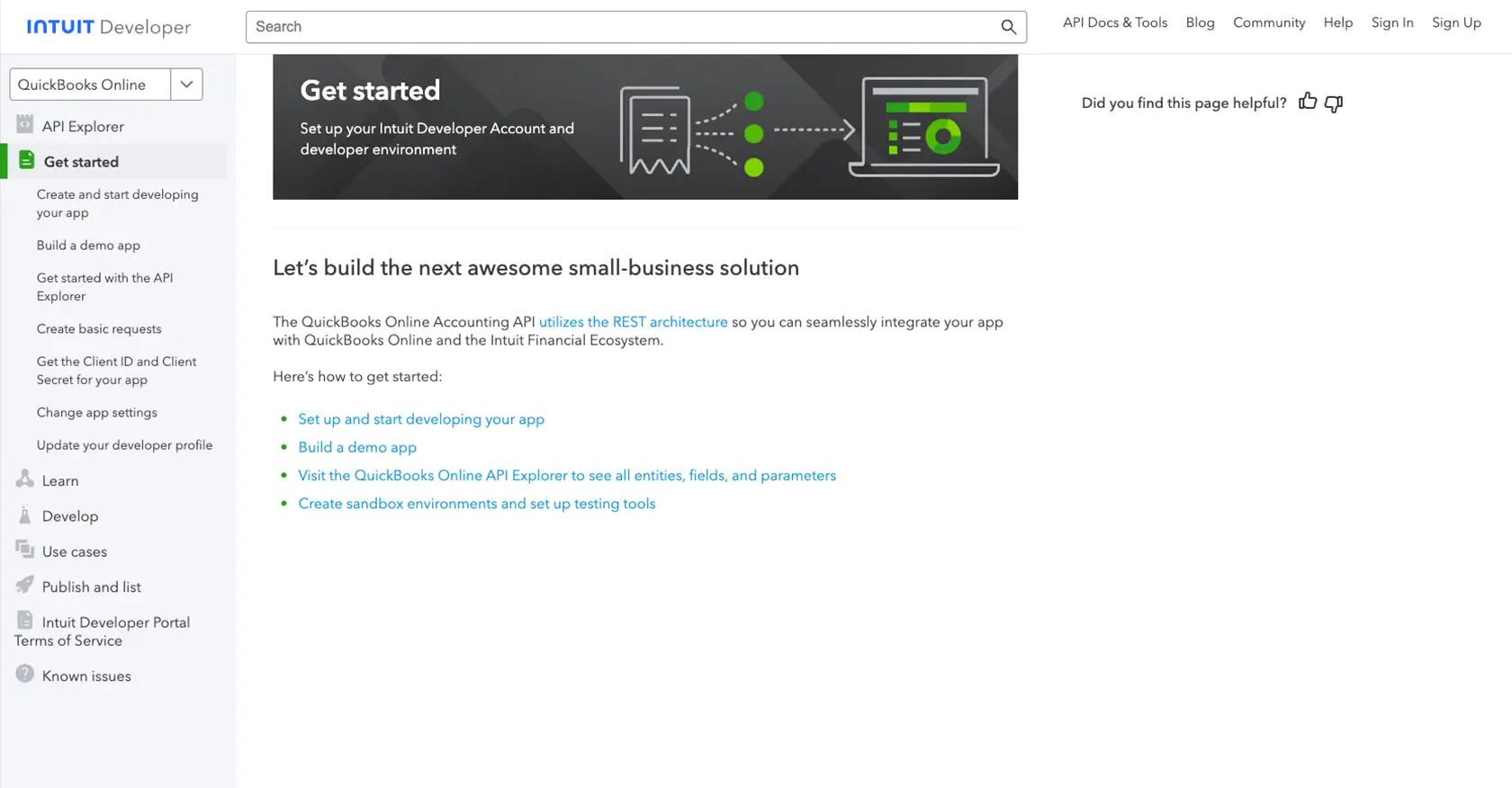
sbb-itb-96038d7
Making API Calls to QuickBooks for Product Management Using JavaScript
To interact with the QuickBooks API for creating or updating products, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and executing API calls effectively.
Setting Up Your JavaScript Environment for QuickBooks API
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14.x or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can set up a new project and install the necessary dependencies:
mkdir quickbooks-api-integration
cd quickbooks-api-integration
npm init -y
npm install axios
Creating or Updating Products with QuickBooks API
Now that your environment is ready, you can create a JavaScript file to handle the API calls. Follow these steps to create or update products:
Step 1: Import Required Modules
Create a file named manageProducts.js
and import the necessary modules:
const axios = require('axios');
Step 2: Set Up API Credentials and Endpoints
Define your API credentials and endpoints. Replace Your_Client_ID
, Your_Client_Secret
, and Your_Access_Token
with your actual credentials:
const clientId = 'Your_Client_ID';
const clientSecret = 'Your_Client_Secret';
const accessToken = 'Your_Access_Token';
const companyId = 'Your_Company_ID';
const apiUrl = `https://sandbox-quickbooks.api.intuit.com/v3/company/${companyId}/item`;
Step 3: Create or Update a Product
Use the following function to create or update a product in QuickBooks:
async function createOrUpdateProduct(productData) {
try {
const response = await axios({
method: 'post', // Use 'post' for creating or 'put' for updating
url: apiUrl,
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
},
data: productData
});
console.log('Product operation successful:', response.data);
} catch (error) {
console.error('Error in product operation:', error.response.data);
}
}
const productData = {
"Name": "New Product",
"Type": "Inventory",
"IncomeAccountRef": {
"value": "79",
"name": "Sales of Product Income"
}
};
createOrUpdateProduct(productData);
Step 4: Execute the Script
Run the script using Node.js to create or update a product:
node manageProducts.js
Upon successful execution, you should see a confirmation message with the product details. If there's an error, the error message will be logged for troubleshooting.
Verifying API Call Success in QuickBooks Sandbox
After running the script, log in to your QuickBooks sandbox account to verify the product creation or update. Navigate to the product list to ensure the changes reflect accurately.
Handling Errors and QuickBooks API Error Codes
When making API calls, it's crucial to handle errors gracefully. QuickBooks API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Always check the error response and implement appropriate error handling in your code.
For more detailed information on error codes, refer to the QuickBooks API Documentation.
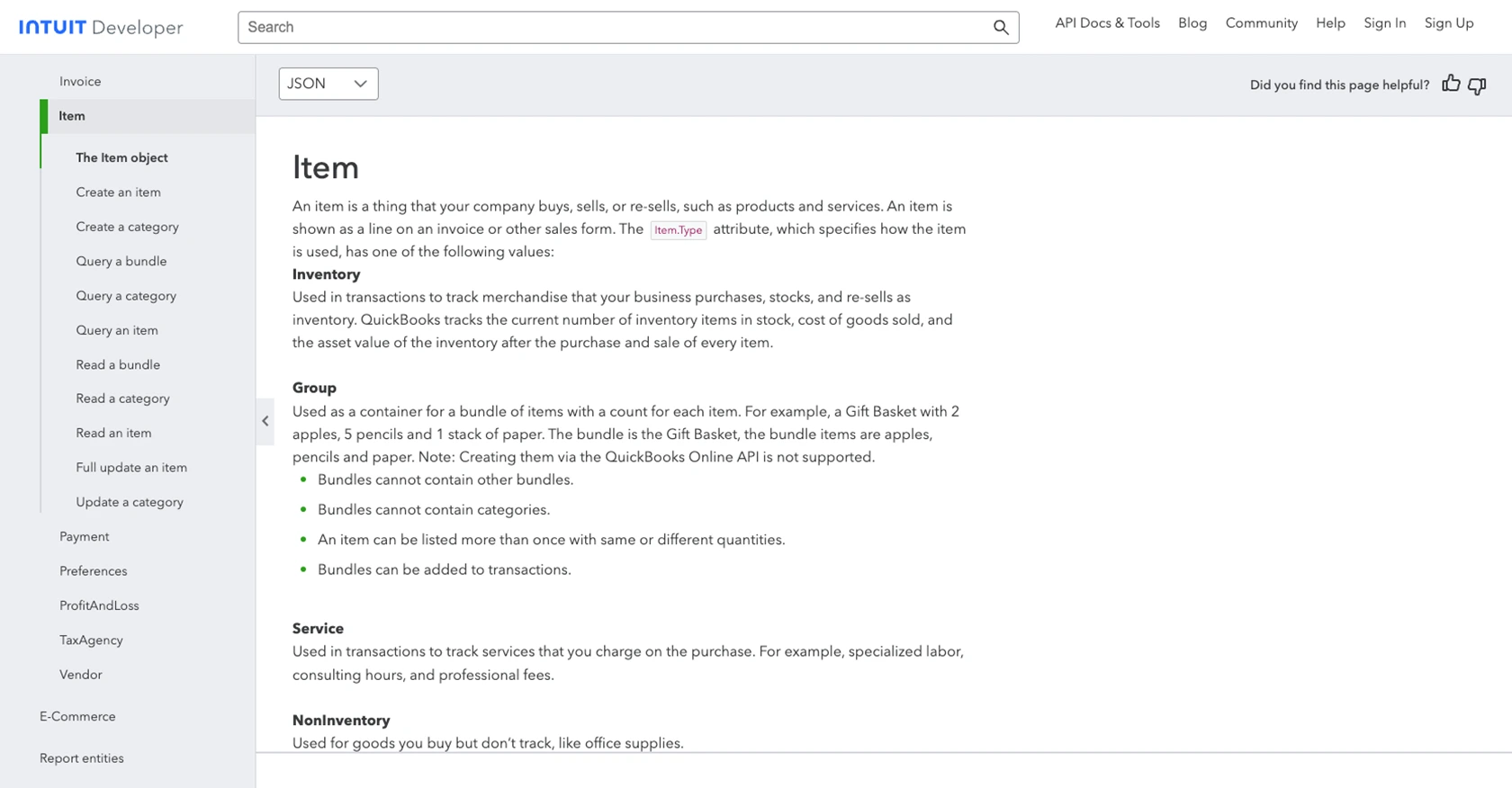
Conclusion: Best Practices for QuickBooks API Integration Using JavaScript
Integrating with the QuickBooks API using JavaScript offers a powerful way to automate and manage financial data, such as product inventories, directly from your applications. By following the steps outlined in this guide, you can efficiently create or update products within QuickBooks, ensuring your data remains accurate and up-to-date.
Best Practices for Secure and Efficient QuickBooks API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Ensure your application handles rate limiting gracefully by implementing retry logic and monitoring API usage. Refer to the QuickBooks API Documentation for specific rate limit details.
- Implement Error Handling: Properly handle errors by checking response codes and implementing fallback mechanisms. This ensures your application remains robust and user-friendly.
- Data Transformation and Standardization: Ensure that data sent to and received from QuickBooks is transformed and standardized to match your application's requirements, maintaining consistency across systems.
Streamline Your Integration Process with Endgrate
While integrating with QuickBooks API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including QuickBooks.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/item
Ready to get started?