Using the Recruit CRM API to Get Contacts (with Javascript examples)
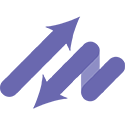
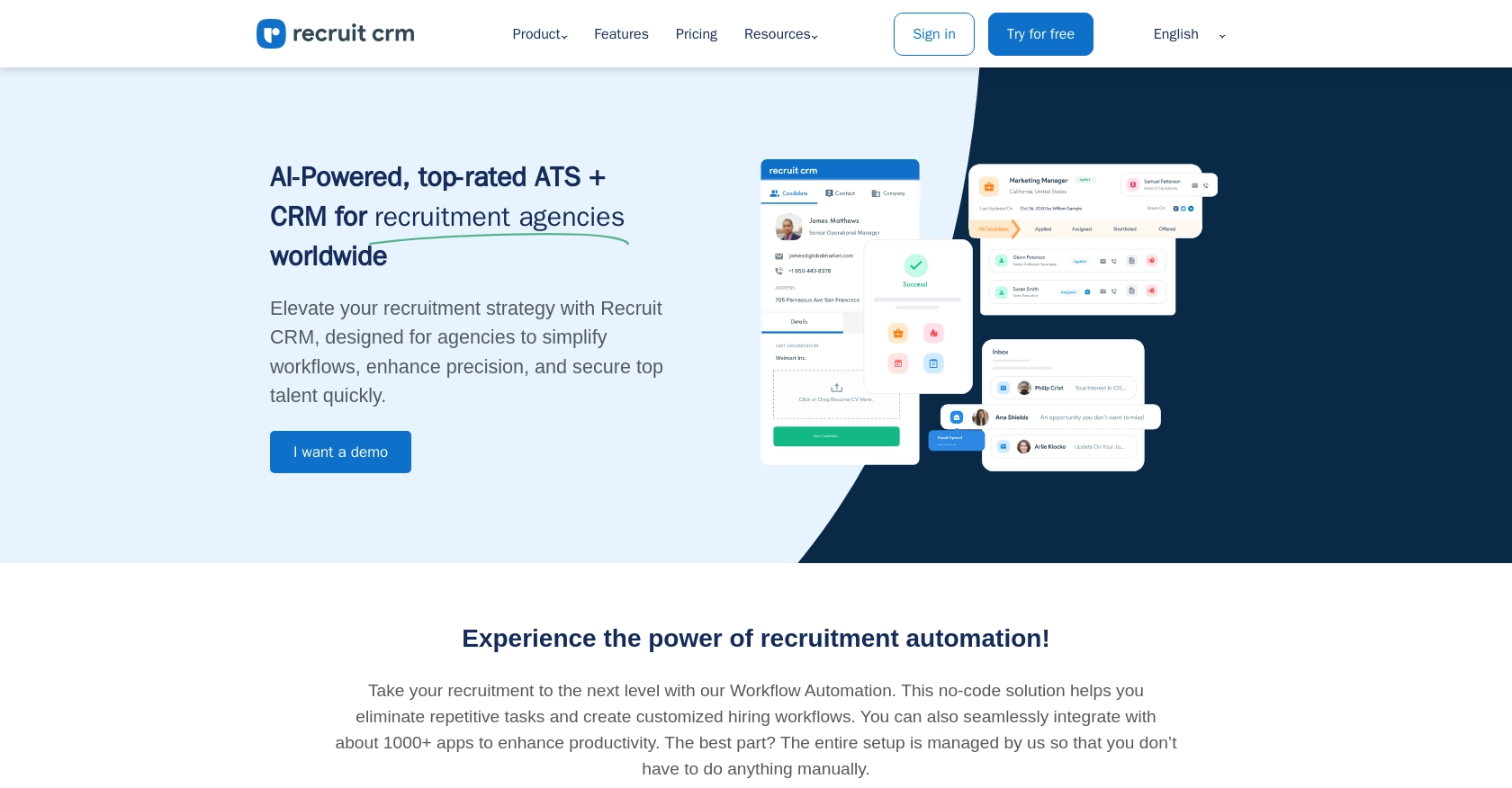
Introduction to Recruit CRM
Recruit CRM is a powerful recruitment software platform designed to enhance the efficiency of recruitment businesses. It offers a comprehensive suite of tools for managing candidates, companies, contacts, and job data, making it an essential tool for recruitment professionals.
Developers may want to integrate with Recruit CRM to streamline their recruitment processes by automating data retrieval and management. For example, using the Recruit CRM API, a developer can programmatically access contact information to update or synchronize it with other systems, ensuring that recruitment teams have the most up-to-date data at their fingertips.
Setting Up Your Recruit CRM Account for API Access
Before you can start using the Recruit CRM API to manage contacts, you'll need to set up your account and obtain the necessary API token. This token will allow you to authenticate your API requests securely.
Creating a Recruit CRM Account
If you don't already have a Recruit CRM account, you'll need to sign up for one. Visit the Recruit CRM website and follow the instructions to create an account. Note that API access requires a Business Plan subscription.
Generating Your API Token in Recruit CRM
Once your account is set up, you'll need to generate an API token. This token is essential for authenticating your API requests.
- Log in to your Recruit CRM account.
- Navigate to Admin Setting in the dashboard.
- Select Account Management.
- Locate the section for API Token management.
- Generate a new API token and ensure you store it securely. Avoid sharing it or storing it in publicly accessible areas.
Important Security Considerations
Your API token carries significant privileges, so it's crucial to keep it secure. All API requests must be made over HTTPS to ensure data security. Requests made without authentication or over plain HTTP will fail.
For more detailed information on authentication, you can refer to the Recruit CRM documentation.
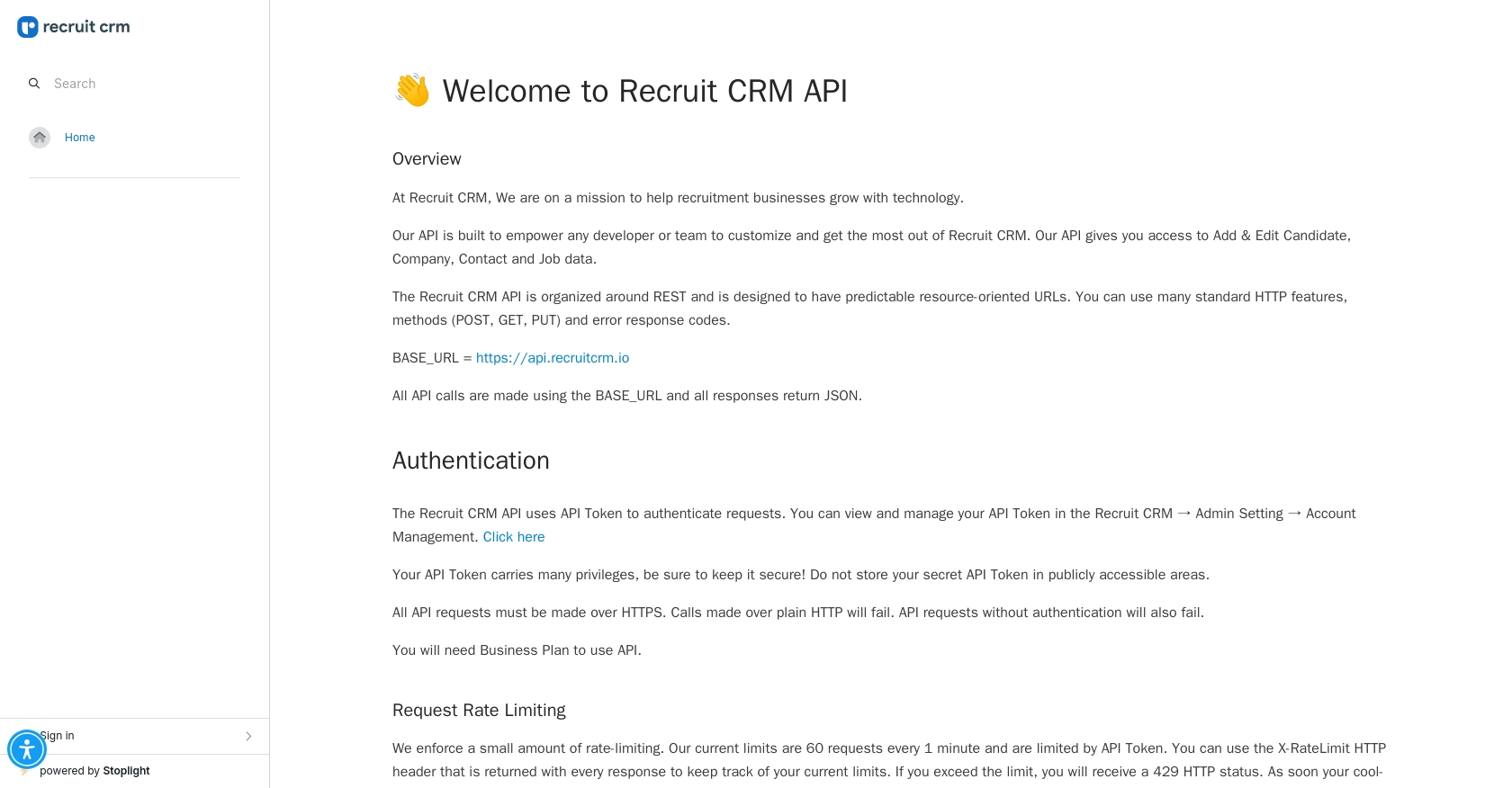
sbb-itb-96038d7
Making API Calls to Recruit CRM Using JavaScript
To interact with the Recruit CRM API and retrieve contact information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment
Before you begin coding, ensure you have the following prerequisites:
- A modern web browser or a Node.js environment for running JavaScript code.
- An API token from Recruit CRM, as described in the previous section.
Installing Required Dependencies
If you're using Node.js, you'll need to install the axios
library to make HTTP requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Retrieve Contacts from Recruit CRM
Create a JavaScript file named getContacts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.recruitcrm.io/v1/contacts';
const headers = {
'Authorization': 'Bearer Your_API_Token',
'Accept': 'application/json'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data;
// Loop through the contacts and log their information
contacts.forEach(contact => {
console.log(`Name: ${contact.name}, Email: ${contact.email}`);
});
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace Your_API_Token
with the API token you generated from Recruit CRM.
Running the JavaScript Code
To execute the code, run the following command in your terminal:
node getContacts.js
You should see a list of contacts printed in the console, each with their name and email address.
Handling Errors and Verifying API Requests
When making API calls, it's essential to handle potential errors gracefully. The code above uses a try-catch
block to catch any errors that occur during the request. If an error occurs, it logs the error message or response data.
To verify that your request succeeded, you can cross-check the returned data with the contacts listed in your Recruit CRM account.
For more detailed information on API endpoints and error codes, refer to the Recruit CRM API documentation.
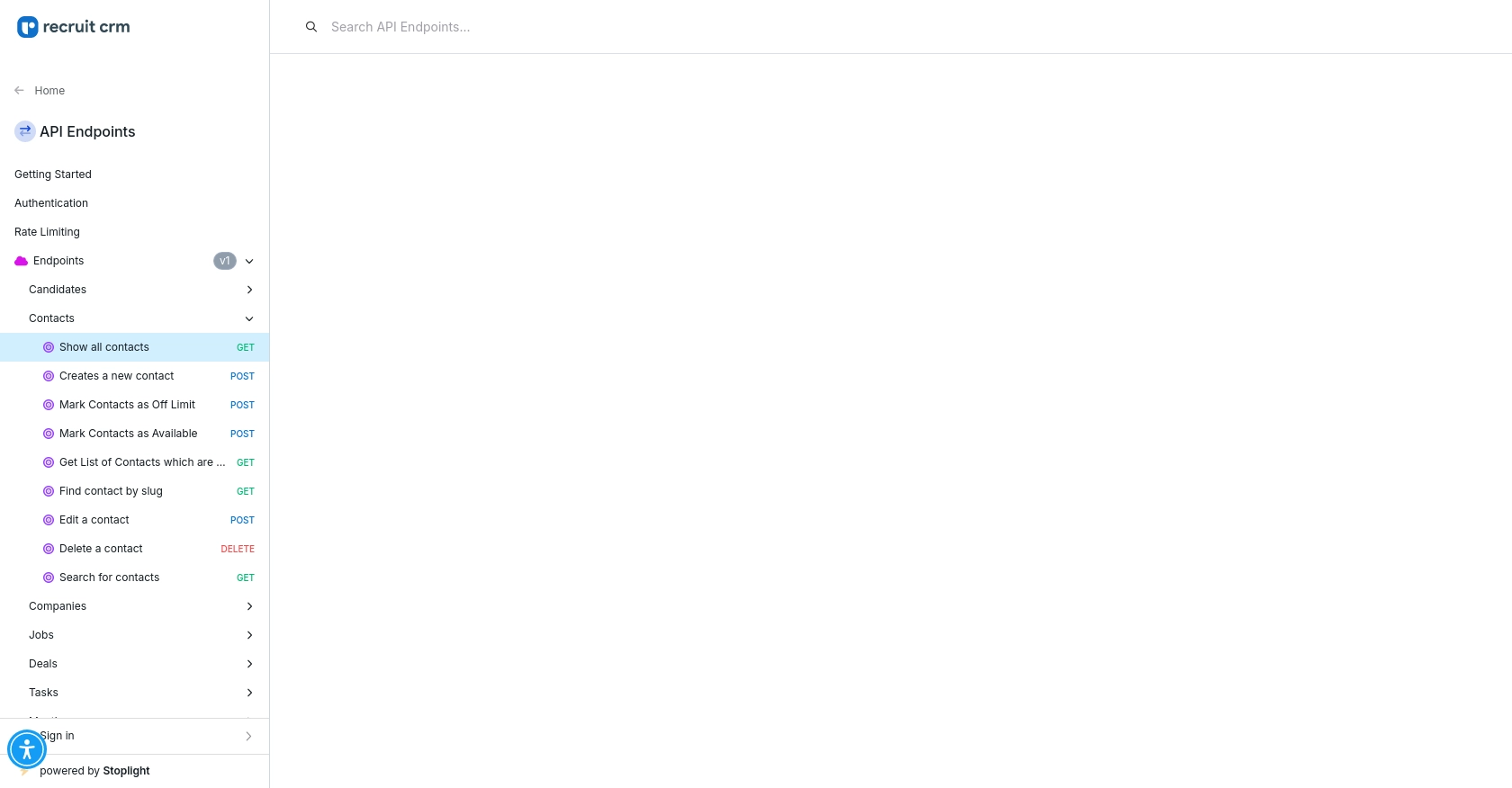
Conclusion and Best Practices for Using Recruit CRM API with JavaScript
Integrating with the Recruit CRM API using JavaScript can significantly enhance your recruitment processes by automating data retrieval and synchronization. By following the steps outlined in this guide, you can efficiently access and manage contact information, ensuring your recruitment team has the most current data available.
Best Practices for Secure and Efficient API Integration
- Secure API Tokens: Always store your API tokens securely and avoid exposing them in public repositories or client-side code.
- Handle Rate Limiting: Be mindful of Recruit CRM's rate limits, which allow 60 requests per minute. Implement logic to handle the 429 status code and retry requests after the cooldown period.
- Data Transformation: Consider transforming and standardizing data fields to ensure consistency across different systems.
- Error Handling: Use robust error handling to manage API errors gracefully, providing informative feedback to users or logs for developers.
Streamlining Integrations with Endgrate
While integrating with Recruit CRM is a powerful way to enhance your recruitment processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Recruit CRM.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, simplifying the integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate and discover the benefits of a seamless integration experience.
Read More
Ready to get started?