Using the Sage Accounting API to Create or Update Item (with Javascript examples)
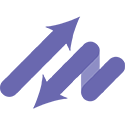
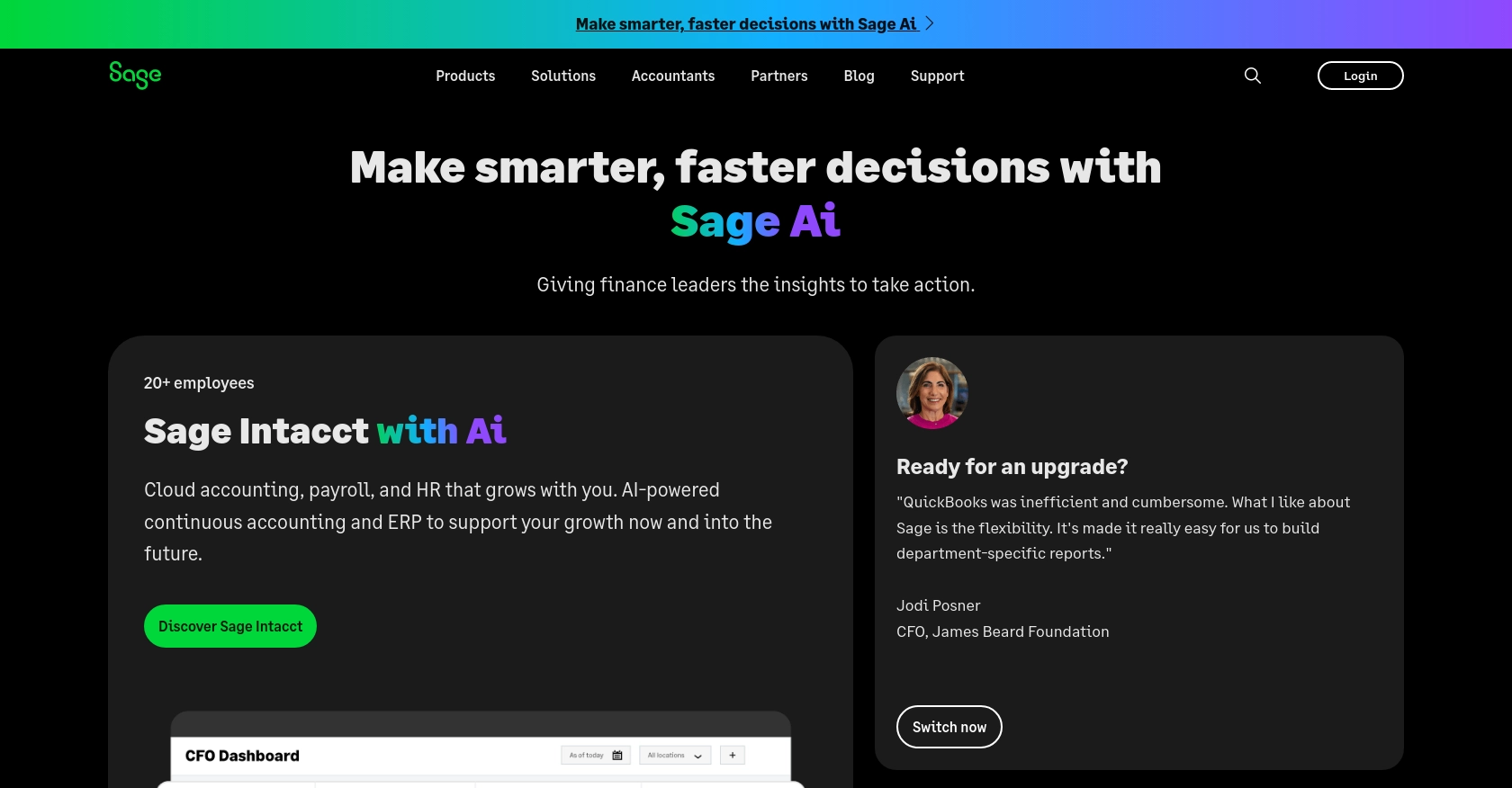
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage finances, including invoicing, expense tracking, and financial reporting. Sage Accounting's flexibility and user-friendly interface make it a preferred choice for businesses aiming to streamline their financial operations.
Developers may want to integrate with the Sage Accounting API to automate and enhance financial processes within their applications. For example, using the API, developers can create or update items in the Sage Accounting system, allowing for seamless inventory management and real-time data synchronization. This integration can significantly improve efficiency by reducing manual data entry and ensuring that financial records are always up-to-date.
Setting Up a Sage Accounting Test/Sandbox Account
Before you can start integrating with the Sage Accounting API, it's essential to set up a test or sandbox account. This allows you to safely test your API interactions without affecting live data. Sage provides a trial business account specifically for development purposes.
Step-by-Step Guide to Creating a Sage Accounting Trial Account
-
Sign Up for a Sage Developer Account:
Begin by signing up for a Sage Developer account. You can register using your GitHub account or an email address. This account will allow you to manage your applications and obtain necessary credentials.
Visit the Sage Developer Portal to get started.
-
Create a Trial Business:
Once your developer account is set up, create a trial business for development. Sage offers trial businesses for all supported regions and subscription tiers. Choose the variant that best suits your integration needs.
Refer to the Sage Quick Start Guide for detailed instructions.
-
Register Your Application:
With your trial business ready, the next step is to register your application. This process generates the client credentials needed for API access.
Log in to your Sage Developer account, navigate to the "Create App" section, and provide the required details such as the app name and callback URL. For more information, visit the Create an App Guide.
-
Upgrade to a Developer Account:
To fully utilize the API, request an upgrade to a developer account. This upgrade grants you 12 months of free access to your Sage Accounting business for testing purposes.
Submit your upgrade request through the Upgrade Your Account page.
Setting Up OAuth Authentication for Sage Accounting API
The Sage Accounting API uses OAuth for authentication. Follow these steps to configure OAuth for your application:
-
Obtain Client ID and Client Secret:
After registering your application, you will receive a Client ID and Client Secret. These credentials are crucial for authenticating API requests.
-
Configure OAuth Settings:
Ensure your application is configured with the correct OAuth settings, including the callback URL specified during app registration.
-
Authorize API Requests:
Use the Client ID and Client Secret to obtain an access token. This token will be used to authorize API requests to the Sage Accounting system.
For troubleshooting and additional guidance, refer to the Sage Authentication Documentation.
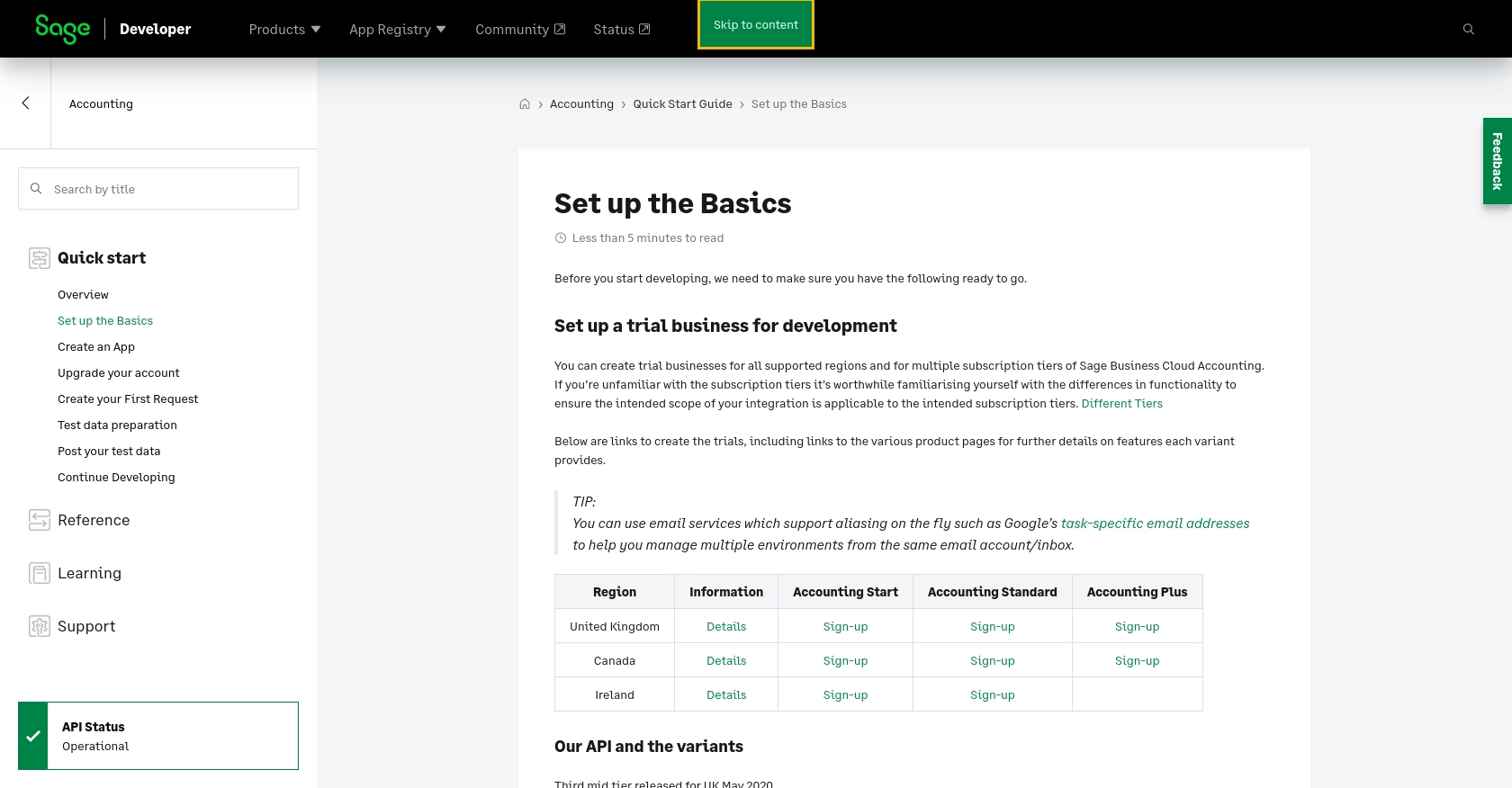
sbb-itb-96038d7
Making API Calls to Create or Update Items in Sage Accounting Using JavaScript
To interact with the Sage Accounting API for creating or updating items, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Sage Accounting API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
- The
axios
library for making HTTP requests.
Install the axios
library using npm:
npm install axios
Writing JavaScript Code to Create or Update Items in Sage Accounting
Below is an example of how to create or update an item using the Sage Accounting API with JavaScript:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.accounting.sage.com/v3.1/products';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the item data
const itemData = {
product: {
description: 'New Product Description',
sales_ledger_account_id: '12345',
purchase_ledger_account_id: '67890',
item_code: 'NP001',
notes: 'This is a new product',
sales_tax_rate_id: 'tax123',
purchase_tax_rate_id: 'tax456',
cost_price: 100.00,
active: true
}
};
// Function to create or update an item
async function createOrUpdateItem() {
try {
const response = await axios.post(endpoint, itemData, { headers });
console.log('Item created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating item:', error.response ? error.response.data : error.message);
}
}
// Execute the function
createOrUpdateItem();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during OAuth authentication.
Verifying API Call Success in Sage Accounting
After executing the code, verify the success of your API call by checking the Sage Accounting dashboard. The newly created or updated item should appear in your product list.
Handling Errors and Common Error Codes in Sage Accounting API
When making API calls, it's essential to handle potential errors. Common error codes include:
- 400 Bad Request: The request was invalid. Check your input data.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The specified resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Sage Accounting API documentation.
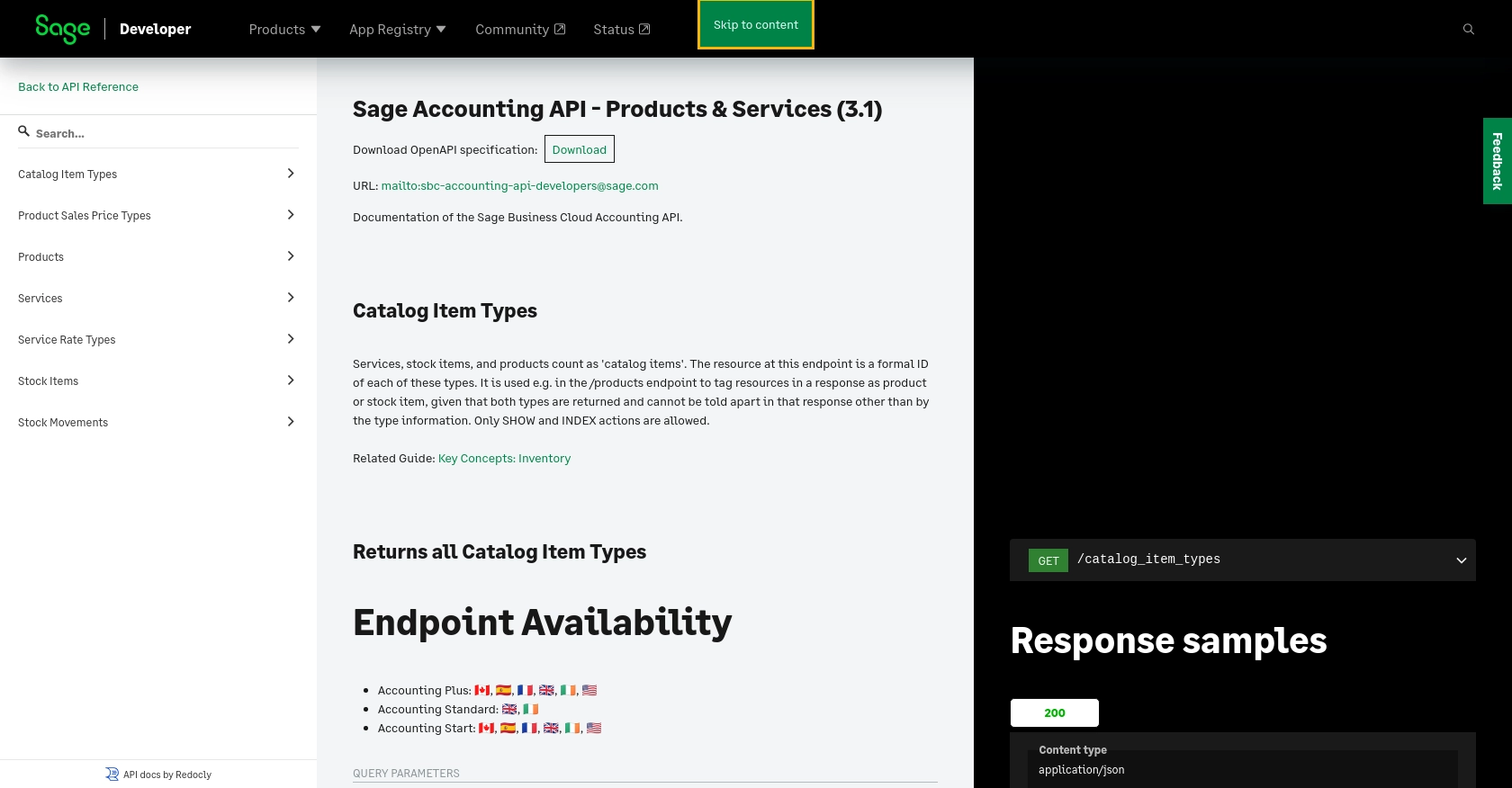
Best Practices for Using the Sage Accounting API
When integrating with the Sage Accounting API, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during API interactions.
- Implement Error Handling: Use robust error handling to manage API errors effectively. Log errors for debugging and provide meaningful feedback to users.
Enhancing Integration Efficiency with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate offers a streamlined solution to simplify this process. By using Endgrate, you can:
- Focus on Core Product Development: Outsource integrations to Endgrate and allocate more resources to enhancing your core product.
- Build Once, Use Multiple Times: Create a single integration for each use case and leverage it across multiple platforms, reducing redundancy.
- Provide a Seamless Experience: Offer your customers an intuitive and consistent integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/products-services/
Ready to get started?