Using the Recruitee API to Create or Update Candidates (with Python examples)
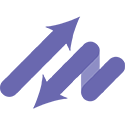
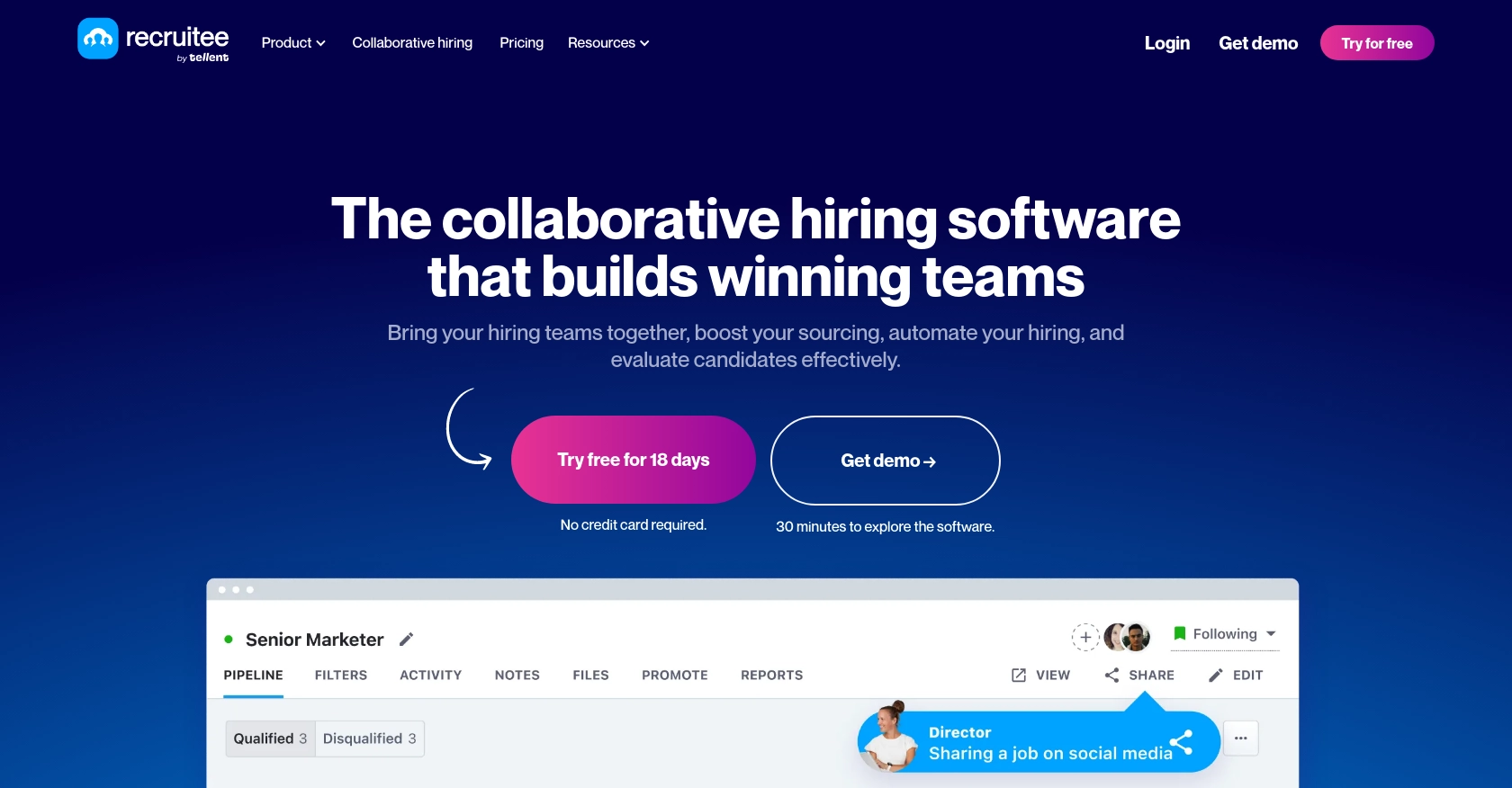
Introduction to Recruitee API Integration
Recruitee is a powerful applicant tracking system (ATS) designed to streamline the recruitment process for businesses of all sizes. It offers a comprehensive suite of tools to manage job postings, candidate applications, and team collaboration, making it a popular choice for HR teams looking to enhance their hiring efficiency.
Integrating with Recruitee's API allows developers to automate and optimize recruitment workflows. For example, you can programmatically create or update candidate profiles directly from your custom application, ensuring that your recruitment data is always up-to-date and accessible.
This article will guide you through using Python to interact with the Recruitee API, specifically focusing on creating and updating candidate information. By the end of this tutorial, you'll be equipped to seamlessly integrate Recruitee's capabilities into your software solutions.
Setting Up Your Recruitee Test Account for API Integration
Before you can start integrating with the Recruitee API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Recruitee provides a straightforward way to generate API tokens, which are essential for authenticating your requests.
Creating a Recruitee Account
If you don't already have a Recruitee account, you can sign up for a free trial on the Recruitee website. This will give you access to the necessary features to test the API integration.
- Visit the Recruitee website and click on the "Sign Up" button.
- Follow the prompts to create your account, providing the required information.
- Once your account is set up, log in to access the dashboard.
Generating a Personal API Token in Recruitee
To authenticate your API requests, you'll need a personal API token. Follow these steps to generate one:
- Log in to your Recruitee account and navigate to Settings.
- Under Apps and plugins, select Personal API tokens.
- Click on + New token in the top-right corner to generate a new token.
- Copy the generated token and store it securely, as you'll need it for API authentication.
For more detailed instructions, refer to the Recruitee API documentation.
Understanding API Key Authentication
Recruitee uses API key-based authentication, which means you will include your personal API token in the headers of your HTTP requests. This token does not expire unless manually revoked, providing a stable authentication method for your integration.
With your Recruitee account and API token ready, you can now proceed to make API calls to create or update candidate information using Python.
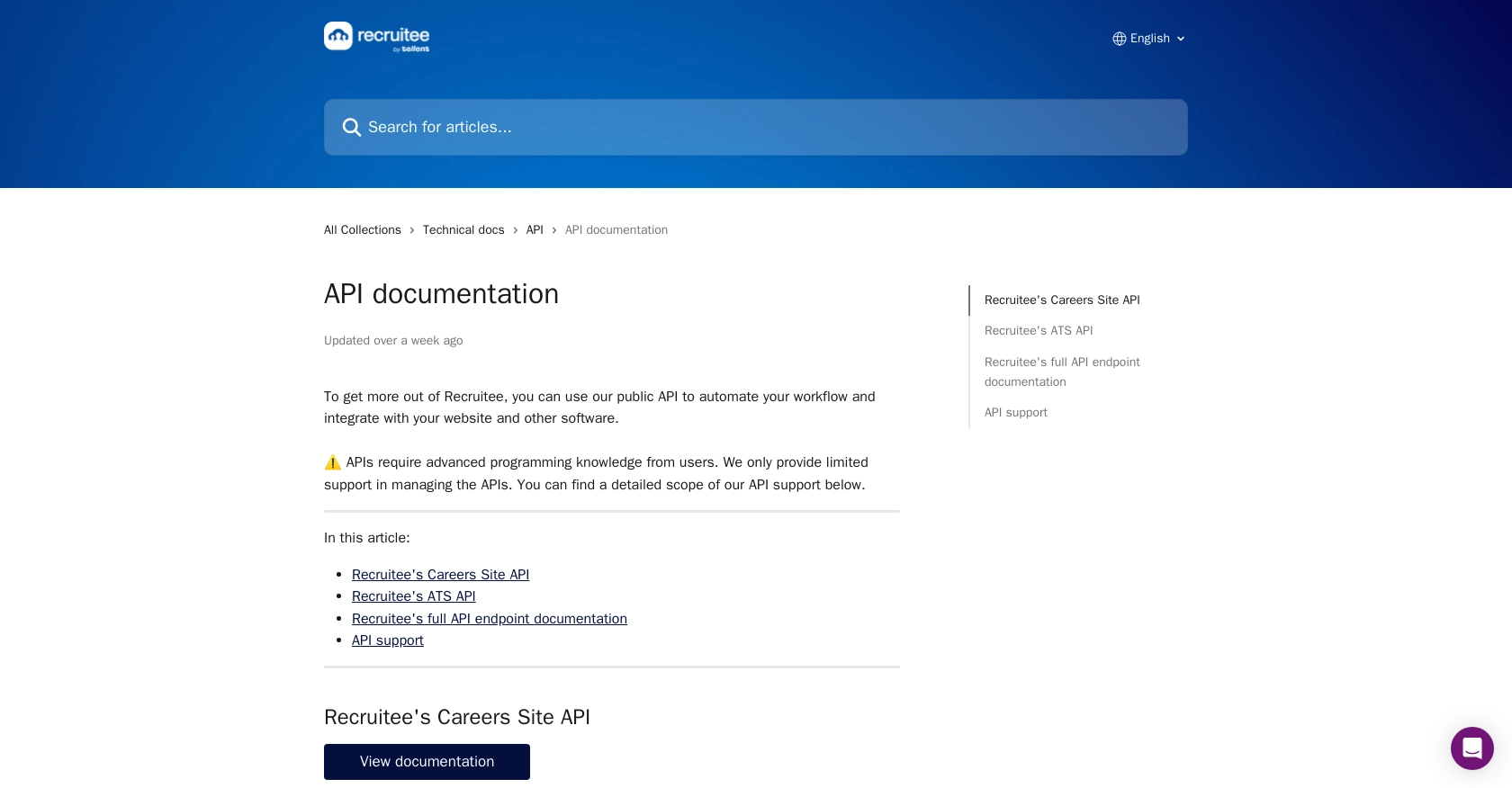
sbb-itb-96038d7
Making API Calls to Recruitee with Python
To interact with the Recruitee API for creating or updating candidate information, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Recruitee API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Creating a Candidate in Recruitee Using Python
To create a candidate in Recruitee, you'll use the POST
method. Here's how you can do it:
import requests
# Set the API endpoint and headers
company_id = "your_company_id"
url = f"https://api.recruitee.com/c/{company_id}/candidates"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer your_api_token"
}
# Define the candidate data
candidate_data = {
"name": "John Doe",
"email": "johndoe@example.com",
"phone": "+123456789",
"note": "This candidate was added via the API."
}
# Make the POST request
response = requests.post(url, json=candidate_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Candidate created successfully!")
else:
print("Failed to create candidate:", response.json())
Replace your_company_id
and your_api_token
with your actual Recruitee company ID and API token. The code sends a POST request to create a candidate, and you can verify the creation in your Recruitee dashboard.
Updating a Candidate in Recruitee Using Python
To update an existing candidate, use the PATCH
method. Here's an example:
import requests
# Set the API endpoint and headers
candidate_id = "existing_candidate_id"
url = f"https://api.recruitee.com/c/{company_id}/candidates/{candidate_id}"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer your_api_token"
}
# Define the updated candidate data
updated_data = {
"name": "John Doe Updated",
"note": "Updated candidate information via API."
}
# Make the PATCH request
response = requests.patch(url, json=updated_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Candidate updated successfully!")
else:
print("Failed to update candidate:", response.json())
Replace existing_candidate_id
with the ID of the candidate you wish to update. This code updates the candidate's information and confirms the update through the API response.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. Recruitee's API will return error codes if something goes wrong. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data and try again.
- 401 Unauthorized: Authentication failed. Verify your API token.
- 404 Not Found: The specified resource could not be found.
Always check the response status code and handle errors gracefully in your application. For more details, refer to the Recruitee API documentation.
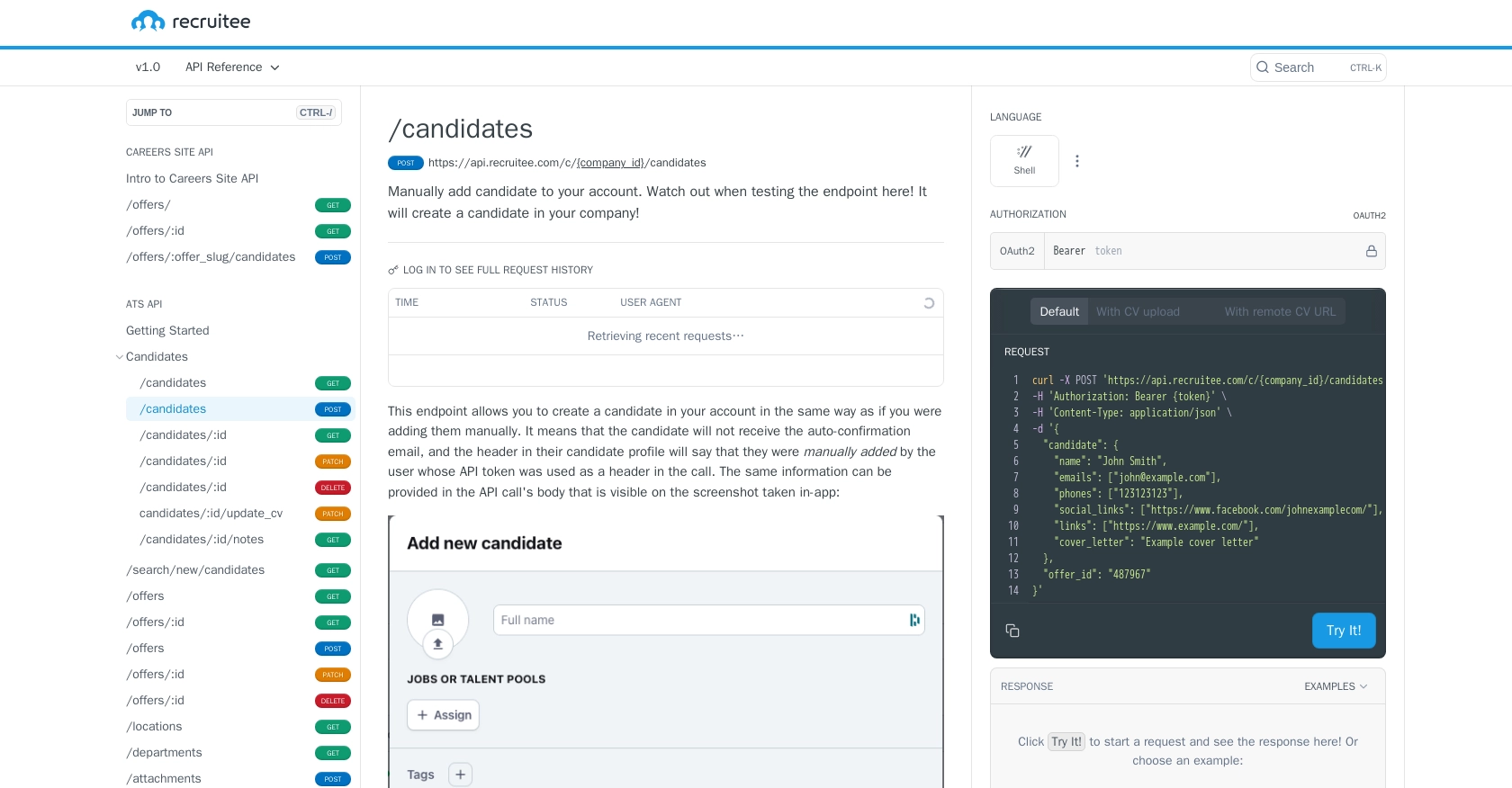
Conclusion and Best Practices for Recruitee API Integration
Integrating with the Recruitee API using Python allows developers to automate and enhance recruitment workflows by programmatically managing candidate data. By following the steps outlined in this guide, you can efficiently create and update candidate profiles, ensuring your recruitment process is both streamlined and up-to-date.
Best Practices for Secure and Efficient Recruitee API Usage
- Secure API Tokens: Always store your API tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Recruitee's API rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests.
- Data Standardization: Ensure that candidate data is standardized before sending it to the API to maintain consistency across your recruitment platform.
- Error Handling: Implement robust error handling to gracefully manage API errors and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
While integrating with Recruitee's API can significantly enhance your recruitment processes, managing multiple integrations can be complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring seamless connectivity across platforms.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?