Using the Recruitee API to Create or Update Candidates in Javascript
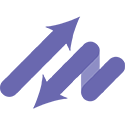
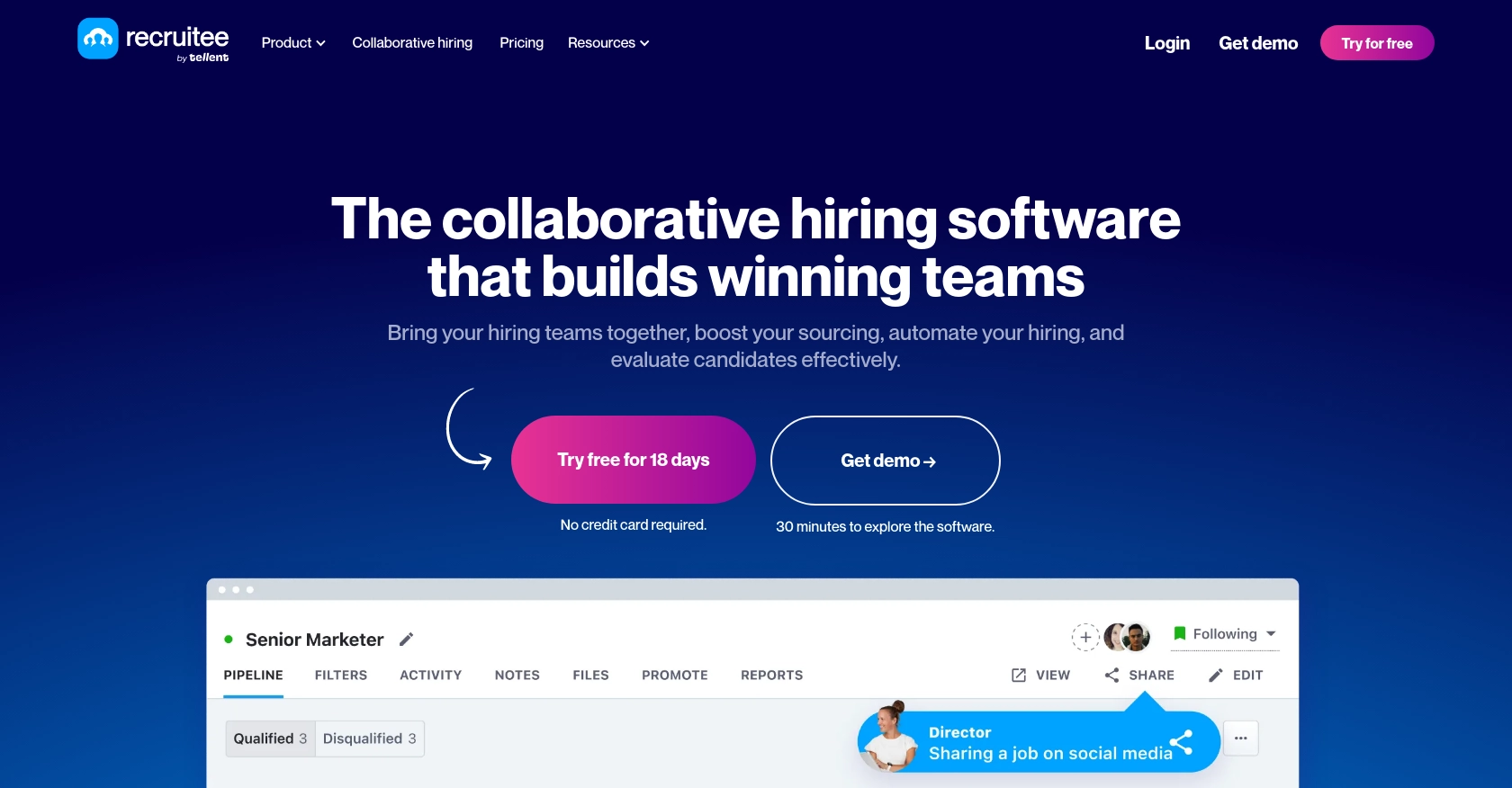
Introduction to Recruitee API
Recruitee is a powerful applicant tracking system (ATS) designed to streamline the recruitment process for businesses of all sizes. It offers a comprehensive suite of tools to manage job postings, track candidates, and collaborate with hiring teams, making it a popular choice for modern HR departments.
Integrating with Recruitee's API allows developers to automate and enhance recruitment workflows. For example, you can use the Recruitee API to programmatically create or update candidate profiles directly from your application, ensuring that your recruitment data is always up-to-date and synchronized across platforms.
This article will guide you through using JavaScript to interact with the Recruitee API, focusing on creating and updating candidate information. By the end of this tutorial, you'll be able to seamlessly integrate Recruitee's capabilities into your own applications, enhancing your recruitment processes.
Setting Up Your Recruitee API Test Account
Before you can start integrating with the Recruitee API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Recruitee provides a straightforward way to generate an API token, which is essential for authenticating your requests.
Creating a Recruitee Account
If you don't already have a Recruitee account, you can sign up for a free trial on their website. This will give you access to the necessary features to test the API integration.
- Visit the Recruitee website and click on the "Sign Up" button.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating a Personal API Token in Recruitee
To interact with the Recruitee API, you'll need a personal API token. This token will authenticate your API requests and ensure secure access to your account data.
- Navigate to Settings in the Recruitee dashboard.
- Select Apps and plugins from the menu.
- Click on Personal API tokens.
- Click + New token in the top-right corner to generate a new token.
- Copy the generated token and store it securely, as you'll need it to authenticate your API calls.
Note: The personal API token does not expire unless you revoke it, providing a stable authentication method for your integration.
For more detailed information on generating API tokens, refer to the Recruitee API documentation.
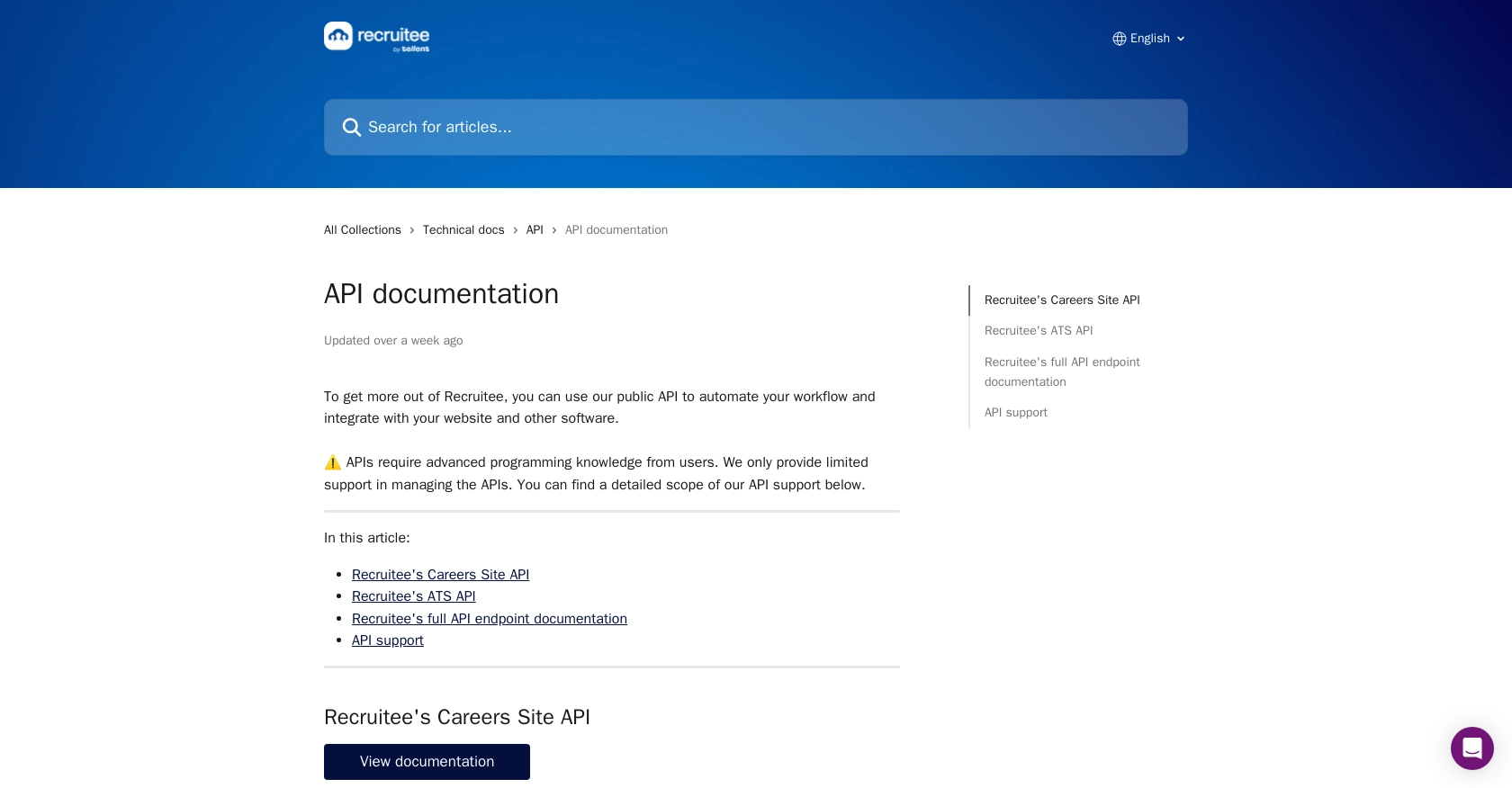
sbb-itb-96038d7
Making API Calls to Recruitee Using JavaScript
To interact with the Recruitee API using JavaScript, you'll need to set up your development environment and write code to perform API requests. This section will guide you through the process of creating and updating candidate profiles using Recruitee's ATS API.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a web browser. You can download it from the official Node.js website.
Additionally, you'll need to install the axios
library, which simplifies making HTTP requests. Run the following command in your terminal to install it:
npm install axios
Creating a Candidate with Recruitee API
To create a candidate in Recruitee, you'll use the POST
method to send candidate data to the API endpoint. Here's how you can do it:
const axios = require('axios');
// Replace with your company ID and API token
const companyId = 'your_company_id';
const apiToken = 'your_api_token';
// API endpoint for creating a candidate
const endpoint = `https://api.recruitee.com/c/${companyId}/candidates`;
// Candidate data
const candidateData = {
candidate: {
name: 'John Doe',
email: 'johndoe@example.com',
phone: '+1234567890'
}
};
// Headers for the request
const headers = {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiToken}`
};
// Function to create a candidate
async function createCandidate() {
try {
const response = await axios.post(endpoint, candidateData, { headers });
console.log('Candidate created successfully:', response.data);
} catch (error) {
console.error('Error creating candidate:', error.response.data);
}
}
createCandidate();
Replace your_company_id
and your_api_token
with your actual Recruitee company ID and API token. This script sends a request to create a candidate with the specified details. If successful, it logs the response data; otherwise, it logs the error.
Updating a Candidate with Recruitee API
To update an existing candidate, use the PATCH
method. You'll need the candidate's ID to perform the update. Here's an example:
const candidateId = 'existing_candidate_id';
// API endpoint for updating a candidate
const updateEndpoint = `https://api.recruitee.com/c/${companyId}/candidates/${candidateId}`;
// Updated candidate data
const updatedData = {
candidate: {
name: 'Jane Doe',
email: 'janedoe@example.com'
}
};
// Function to update a candidate
async function updateCandidate() {
try {
const response = await axios.patch(updateEndpoint, updatedData, { headers });
console.log('Candidate updated successfully:', response.data);
} catch (error) {
console.error('Error updating candidate:', error.response.data);
}
}
updateCandidate();
Replace existing_candidate_id
with the ID of the candidate you wish to update. This script updates the candidate's information and logs the result.
For more details on the API endpoints, refer to the Recruitee API documentation for creating candidates and the Recruitee API documentation for updating candidates.
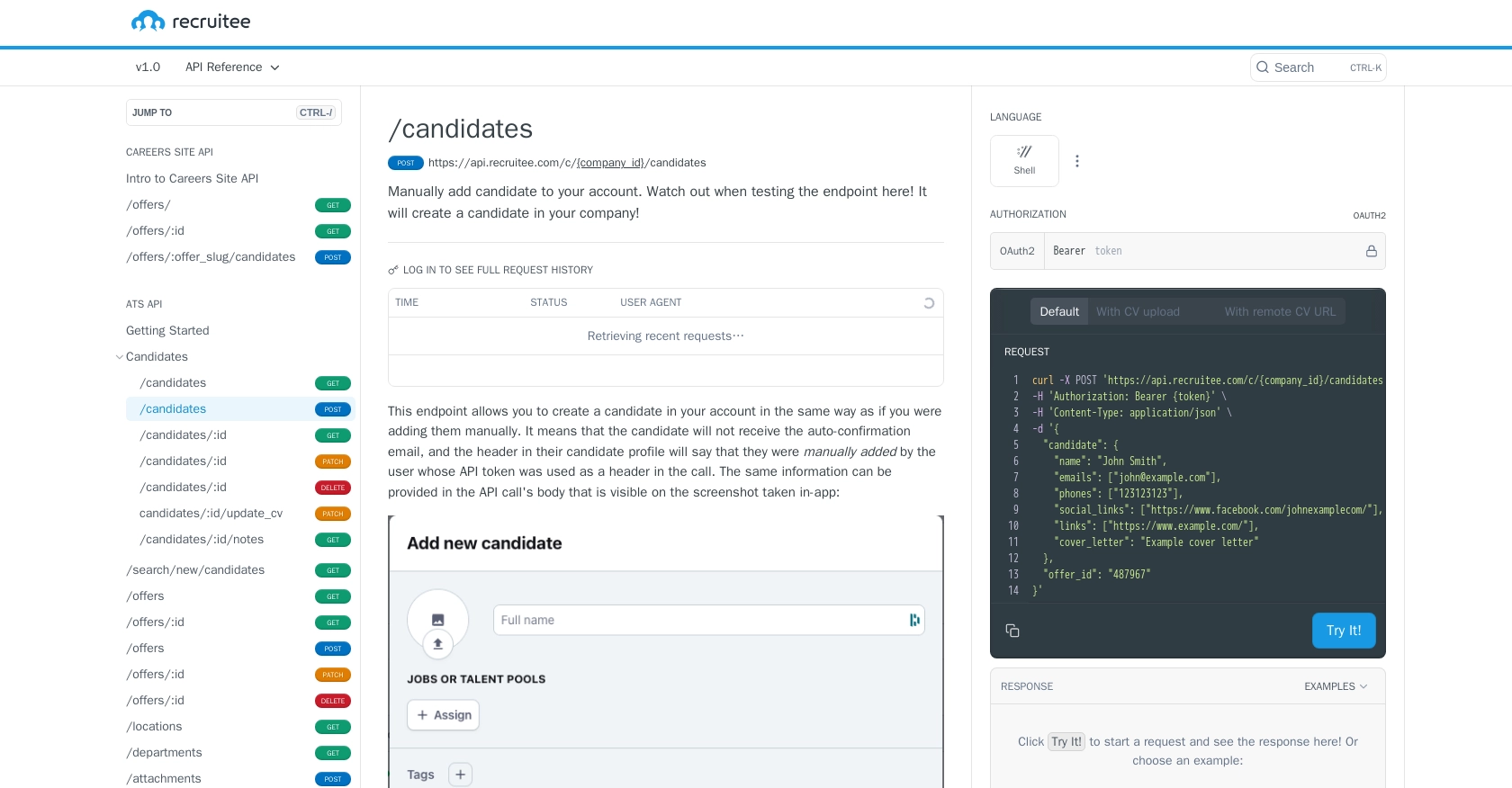
Conclusion and Best Practices for Using Recruitee API in JavaScript
Integrating with the Recruitee API using JavaScript allows developers to efficiently manage candidate data, enhancing recruitment workflows. By following the steps outlined in this article, you can create and update candidate profiles seamlessly, ensuring your recruitment data remains synchronized across platforms.
Best Practices for Secure and Efficient API Integration with Recruitee
- Securely Store API Tokens: Always store your API tokens securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Errors Gracefully: Implement error handling to manage API call failures, such as network issues or invalid data, and provide meaningful feedback to users.
- Respect Rate Limits: Be mindful of any rate limits imposed by Recruitee to avoid throttling. Implement retry logic with exponential backoff if necessary.
- Data Validation: Validate candidate data before making API calls to ensure data integrity and prevent errors.
Enhance Your Integration Strategy with Endgrate
While integrating with Recruitee's API can significantly improve your recruitment processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Recruitee. This streamlined approach saves time and resources, providing an intuitive integration experience for your team and customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website today.
Read More
Ready to get started?