Using the Sage 100 API to Create or Update Items (with Javascript examples)
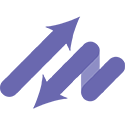
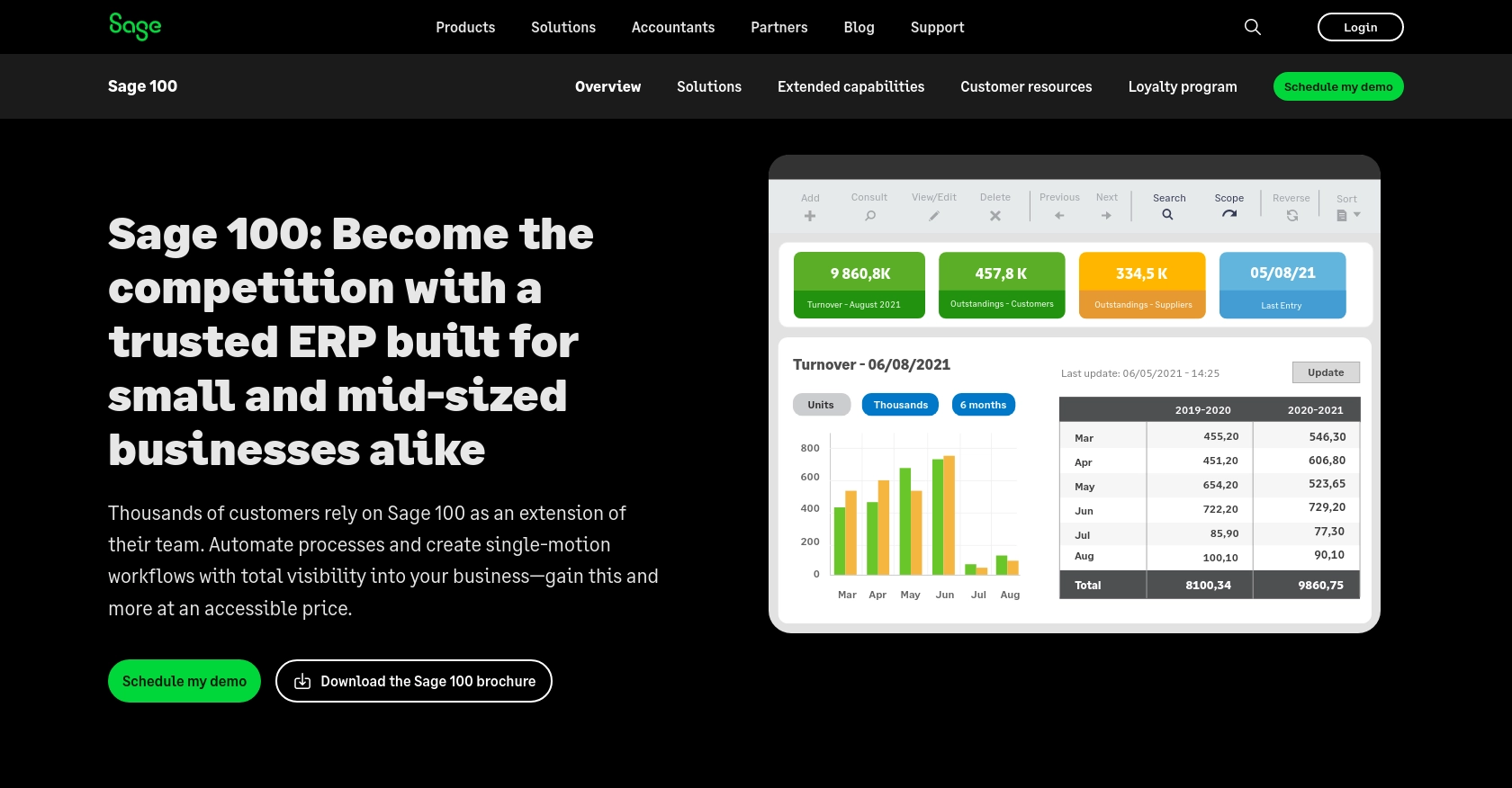
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive enterprise resource planning (ERP) solution that caters to small and medium-sized businesses. It offers a robust suite of tools for managing accounting, inventory, distribution, and manufacturing processes. With its extensive capabilities, Sage 100 helps businesses streamline operations and improve efficiency.
Integrating with the Sage 100 API allows developers to automate and enhance various business processes. For example, you can create or update inventory items programmatically, ensuring that your product data is always up-to-date across different platforms. This integration can be particularly useful for businesses that need to manage large inventories or frequently update product information.
Setting Up a Sage 100 Test/Sandbox Account for API Integration
Before you can begin integrating with the Sage 100 API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data. Follow these steps to configure your Sage 100 test account and prepare for API interactions.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you must first ensure that the Sage 100 ODBC driver is installed and configured correctly. This driver facilitates the connection to the Sage 100 database. Here's how to set it up:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Verify the connection by testing the DSN configuration.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 API Application
Once the ODBC driver is set up, you need to create an application within Sage 100 to obtain the necessary credentials for API access:
- Log in to your Sage 100 account and navigate to the API management section.
- Create a new application and note down the client ID and client secret provided.
- Configure the application permissions to allow access to inventory items.
Configuring Authentication for Sage 100 API
Sage 100 uses a custom authentication method. Follow these steps to authenticate your API requests:
- Use the client ID and client secret obtained from your application setup.
- Generate an access token by sending a request to the authentication endpoint.
- Include the access token in the header of your API requests to authenticate.
Testing the Sage 100 API Connection
After setting up your test account and configuring authentication, it's crucial to test the connection to ensure everything is working correctly:
- Use a tool like Postman or a simple script to send a test API request to Sage 100.
- Verify the response to ensure that the connection is successful and the data is returned as expected.
By following these steps, you can confidently set up a Sage 100 test account and prepare for seamless API integration.
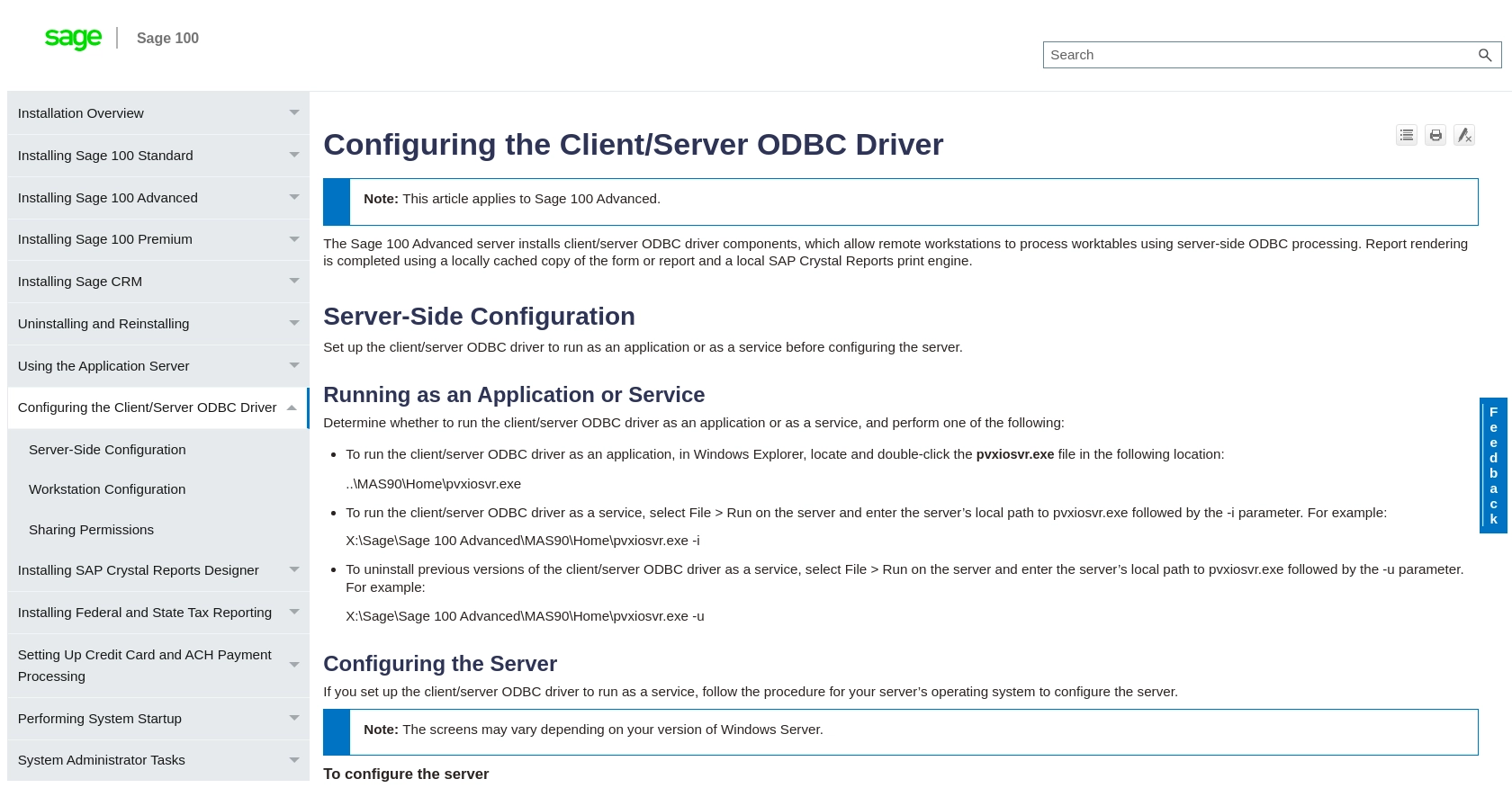
sbb-itb-96038d7
Making API Calls to Sage 100 for Creating or Updating Items Using JavaScript
To interact with the Sage 100 API for creating or updating items, you need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Sage 100 API Integration
Before you start coding, ensure you have the necessary tools and libraries installed. You'll need Node.js and npm (Node Package Manager) to manage dependencies. Follow these steps to set up your environment:
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the
axios
library for making HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Create or Update Items in Sage 100
With your environment set up, you can now write the JavaScript code to interact with the Sage 100 API. Below is an example of how to create or update an item:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.sage100.com/items';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Define the item data
const itemData = {
itemCode: 'ITEM001',
description: 'Sample Item',
price: 100.00,
quantity: 50
};
// Function to create or update an item
async function createOrUpdateItem() {
try {
const response = await axios.post(endpoint, itemData, { headers });
console.log('Item created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating item:', error.response ? error.response.data : error.message);
}
}
// Call the function
createOrUpdateItem();
Replace Your_Access_Token
with the access token obtained during authentication. This code uses the axios
library to send a POST request to the Sage 100 API, creating or updating an item with the specified data.
Verifying API Request Success and Handling Errors
After executing the API call, it's crucial to verify the success of the request and handle any errors that may occur:
- Check the response status code to ensure the request was successful (typically a 200 or 201 status code).
- Log the response data to confirm that the item was created or updated as expected.
- Implement error handling using try-catch blocks to manage issues such as network errors or invalid data.
By following these steps, you can effectively make API calls to Sage 100 to create or update items using JavaScript, ensuring your inventory data remains accurate and up-to-date.
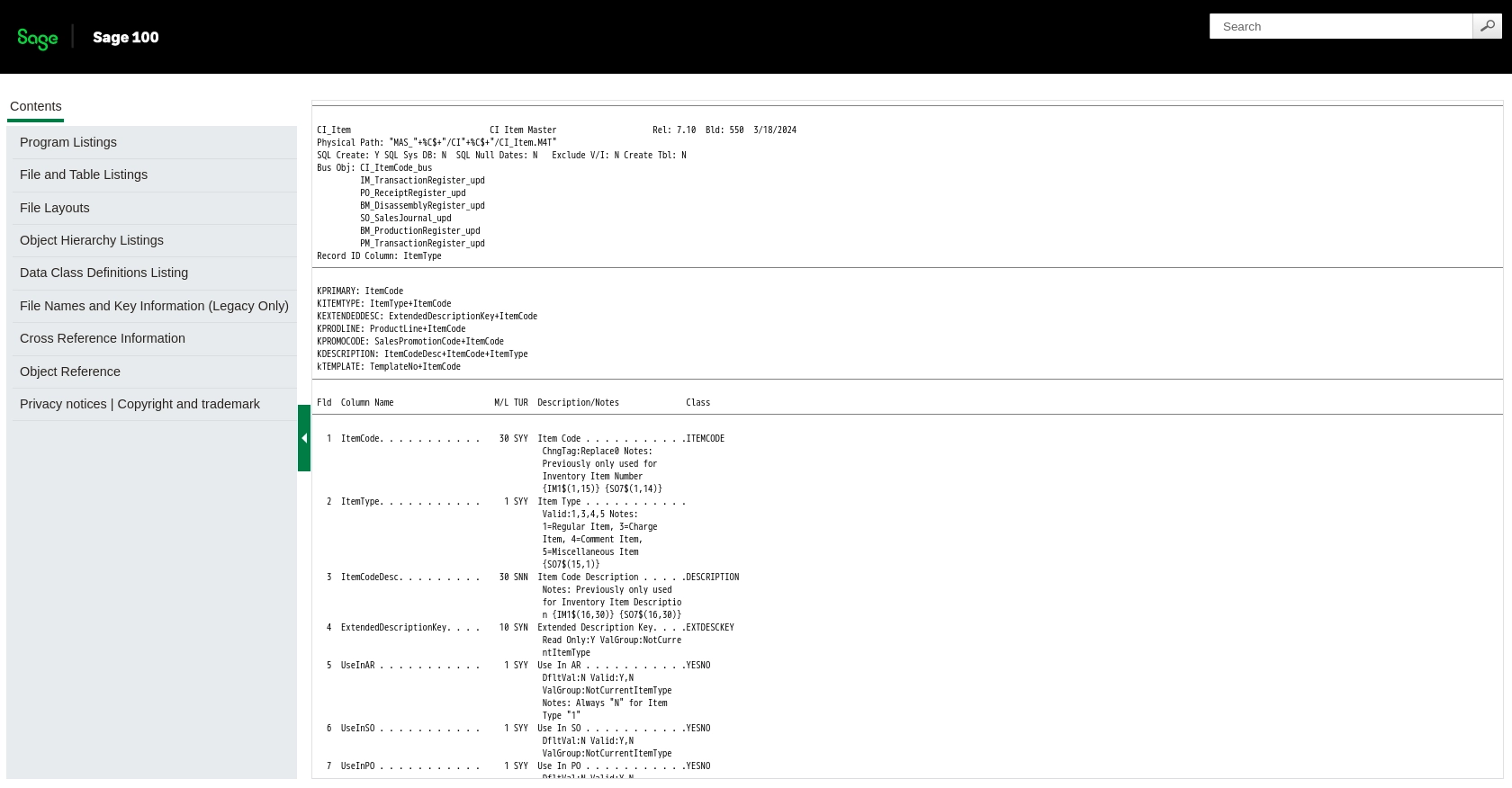
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API to create or update items using JavaScript can significantly enhance your business operations by automating inventory management and ensuring data consistency across platforms. By following the steps outlined in this guide, you can effectively set up your environment, authenticate your requests, and handle API interactions with confidence.
Best Practices for Secure and Efficient Sage 100 API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation: Ensure that the data being sent to the API is validated and sanitized to prevent errors and maintain data integrity.
- Error Handling: Implement comprehensive error handling to manage network issues, authentication failures, and invalid data responses.
Streamlining Integrations with Endgrate
While integrating with the Sage 100 API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage a seamless integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?