Using the Sage 100 API to Create or Update Sales Orders (with Javascript examples)
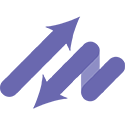
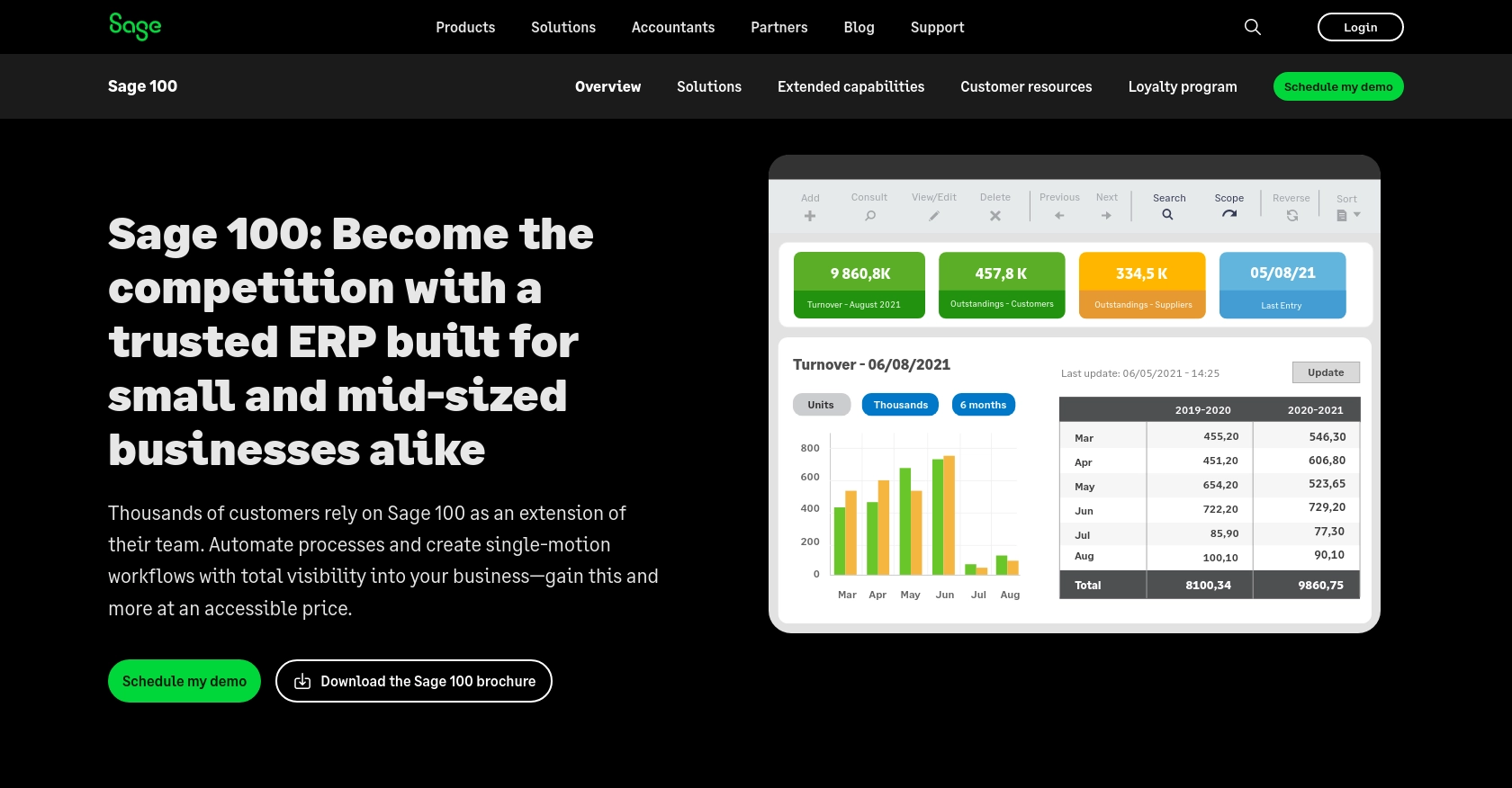
Introduction to Sage 100 API for Sales Order Management
Sage 100 is a comprehensive ERP solution that offers robust tools for managing various business operations, including accounting, inventory, and sales. It is widely used by small to medium-sized businesses to streamline their processes and improve efficiency.
Integrating with the Sage 100 API allows developers to automate and enhance sales order management. By connecting to this API, developers can create or update sales orders programmatically, reducing manual entry and minimizing errors. For example, a developer might use the Sage 100 API to automatically update sales orders from an e-commerce platform, ensuring that inventory levels and sales data are always current.
Setting Up Your Sage 100 Test/Sandbox Account for API Integration
Before you can start creating or updating sales orders using the Sage 100 API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with the API without affecting your live data.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, ensure that the Sage 100 ODBC driver is installed and properly configured on your system. Follow these steps:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
- Ensure the client/server ODBC driver is set up to run as an application or service. For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Sandbox Account
If you don't already have a Sage 100 sandbox account, you may need to contact Sage support or your account representative to set one up. This account will provide a safe environment to test API interactions.
Configuring the Sage 100 API Access
Once your sandbox account is ready, configure the API access:
- Log in to your Sage 100 account and navigate to the API settings.
- Ensure that the necessary permissions are granted for creating and updating sales orders.
- Generate any required API keys or tokens that will be used for authentication in your API calls.
Testing the ODBC Connection
After configuring the ODBC driver and API access, test the connection to ensure everything is set up correctly:
- Open the ODBC Data Source Administrator and locate your Sage 100 DSN.
- Use the "Test Connection" feature to verify that the connection to the Sage 100 database is successful.
If you encounter any issues, consult the Sage 100 ODBC Driver Configuration Guide for troubleshooting tips.
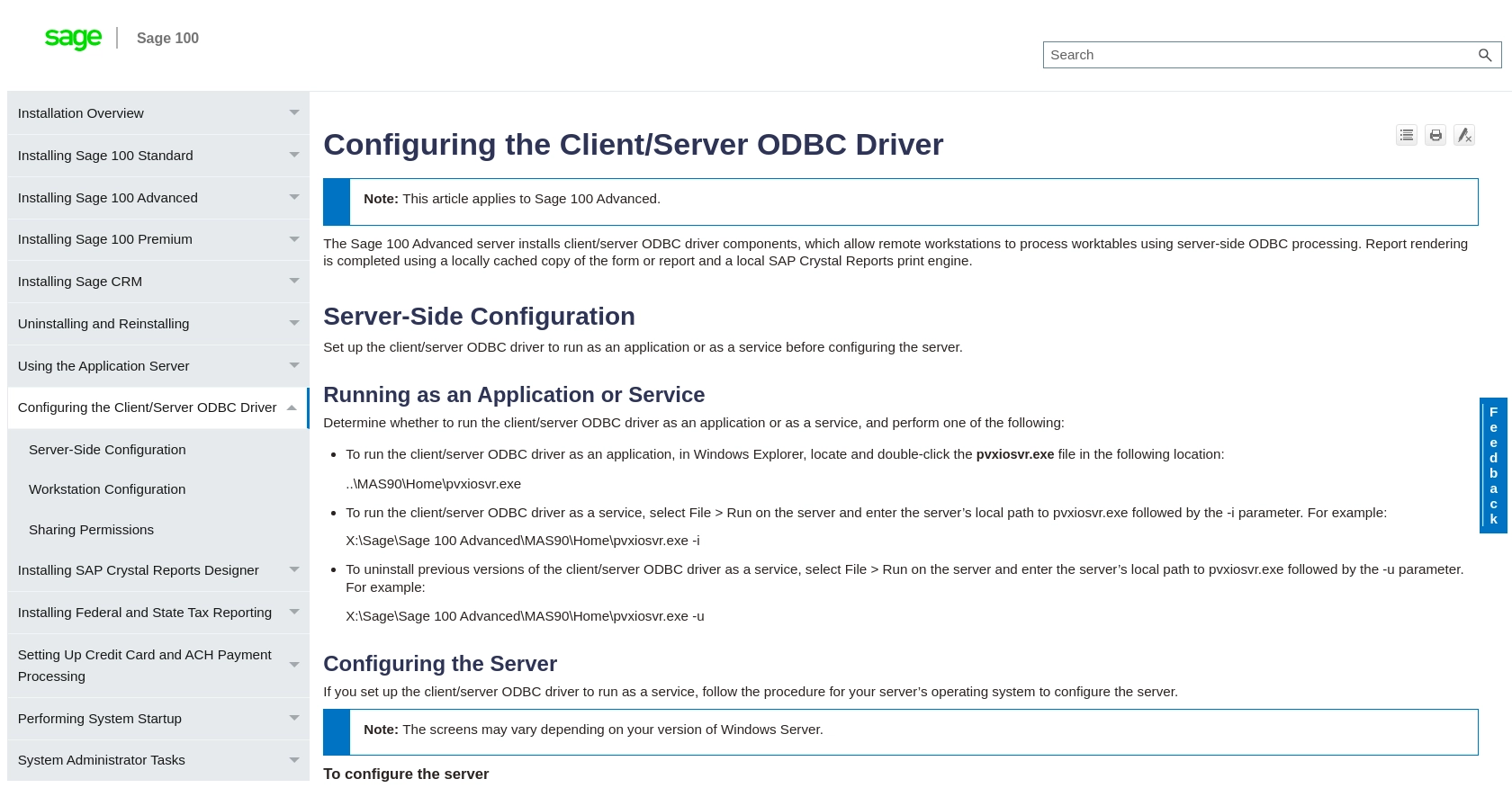
sbb-itb-96038d7
Making API Calls to Sage 100 for Sales Order Management Using JavaScript
To interact with the Sage 100 API for creating or updating sales orders, you'll need to use JavaScript to make HTTP requests. This section will guide you through setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Sage 100 API Integration
Before making API calls, ensure you have Node.js installed on your system, as it provides the runtime environment for executing JavaScript code outside a browser. Additionally, you'll need to install the axios
library to simplify HTTP requests.
npm install axios
Creating or Updating Sales Orders with Sage 100 API
Once your environment is ready, you can start writing the JavaScript code to interact with the Sage 100 API. Below is an example of how to create or update sales orders.
const axios = require('axios');
// Set up the API endpoint and headers
const endpoint = 'https://api.sage100.com/salesorders';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_API_Token'
};
// Define the sales order data
const salesOrderData = {
orderNumber: '12345',
customerID: 'CUST001',
orderDate: '2023-10-01',
items: [
{ itemID: 'ITEM001', quantity: 10, price: 100 },
{ itemID: 'ITEM002', quantity: 5, price: 50 }
]
};
// Function to create or update a sales order
async function createOrUpdateSalesOrder() {
try {
const response = await axios.post(endpoint, salesOrderData, { headers });
console.log('Sales Order Created/Updated Successfully:', response.data);
} catch (error) {
console.error('Error Creating/Updating Sales Order:', error.response ? error.response.data : error.message);
}
}
// Execute the function
createOrUpdateSalesOrder();
Verifying Successful API Requests in Sage 100
After executing the code, you can verify the success of your API requests by checking the sales orders in your Sage 100 sandbox account. Ensure that the newly created or updated sales orders appear as expected.
Handling Errors and Troubleshooting Sage 100 API Calls
When making API calls, it's crucial to handle potential errors gracefully. The example code above includes a try-catch
block to catch and log any errors that occur during the request. Common issues might include network errors, authentication failures, or invalid data formats.
For more detailed error information, refer to the Sage 100 API documentation: Sales Order Header and Sales Order Detail.
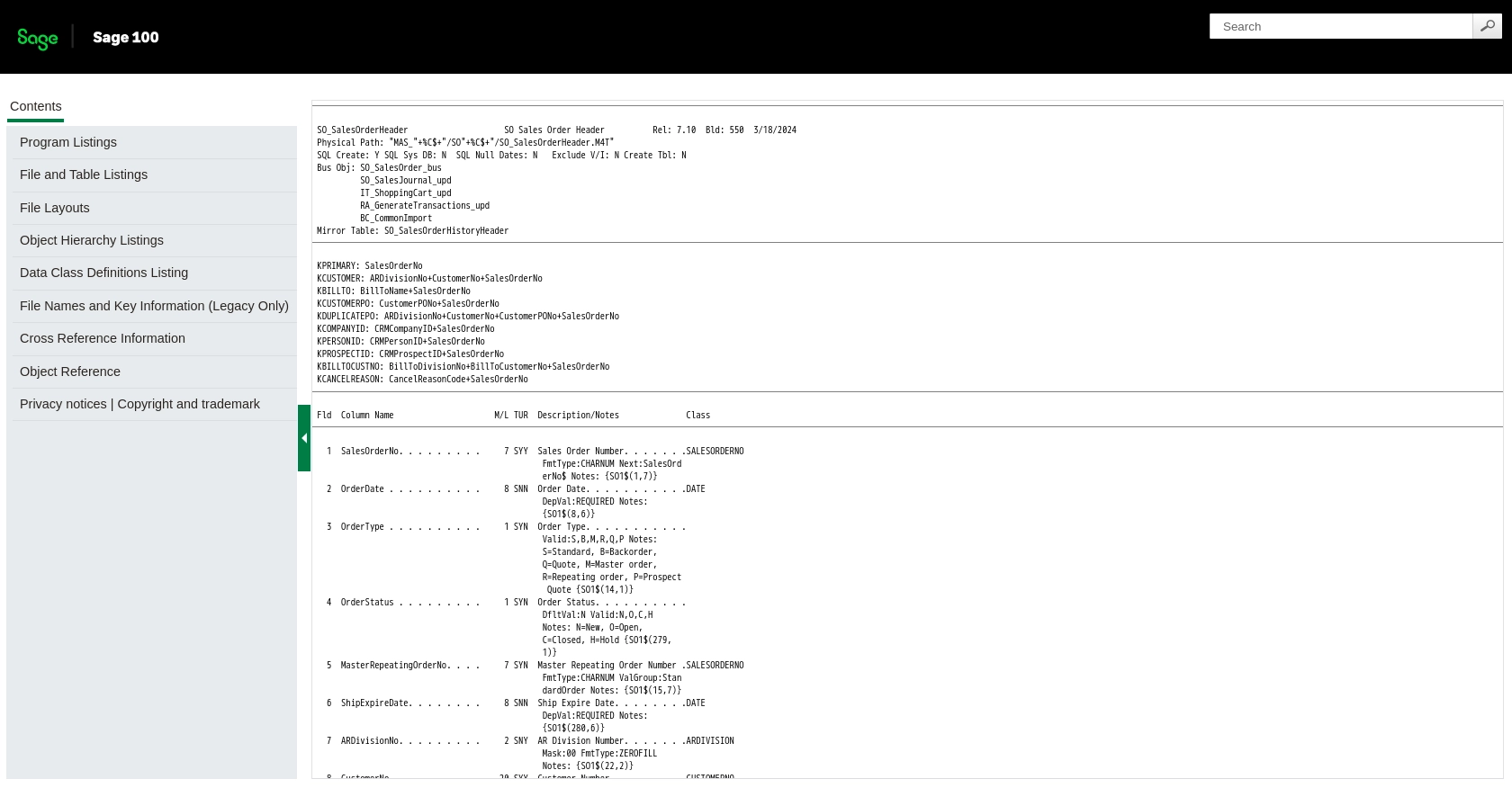
Best Practices for Sage 100 API Integration and Sales Order Management
Successfully integrating with the Sage 100 API requires adherence to best practices that ensure efficiency, security, and scalability. Here are some key recommendations:
Securely Storing Sage 100 API Credentials
Always store your API credentials securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Sage 100 API Rate Limiting
Be mindful of any rate limits imposed by the Sage 100 API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a specified delay. Check the Sage 100 API documentation for specific rate limit details.
Standardizing and Transforming Data for Sage 100 API
Ensure that the data you send to the Sage 100 API is standardized and validated. This includes formatting dates, numbers, and strings according to the API's requirements to prevent errors and ensure data integrity.
Utilizing Endgrate for Streamlined Integration
Consider using Endgrate to simplify your integration process. Endgrate provides a unified API endpoint that connects to multiple platforms, including Sage 100, allowing you to manage integrations more efficiently. By leveraging Endgrate, you can focus on your core product while outsourcing complex integration tasks.
For more information on how Endgrate can enhance your integration experience, visit Endgrate.
Conclusion: Enhancing Business Operations with Sage 100 API
Integrating with the Sage 100 API for sales order management can significantly enhance your business operations by automating processes and reducing manual errors. By following the steps outlined in this guide and adhering to best practices, you can ensure a smooth and efficient integration experience.
Whether you're updating sales orders from an e-commerce platform or managing inventory levels, the Sage 100 API provides the tools necessary to streamline your workflows. Embrace the power of automation and integration to drive your business forward.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderHeader.htm?Highlight=SO_SalesOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderDetail.htm?Highlight=SO_SalesOrderDetail
Ready to get started?