Using the Sage 100 API to Get Tax Schedules in Javascript
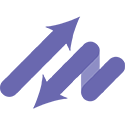
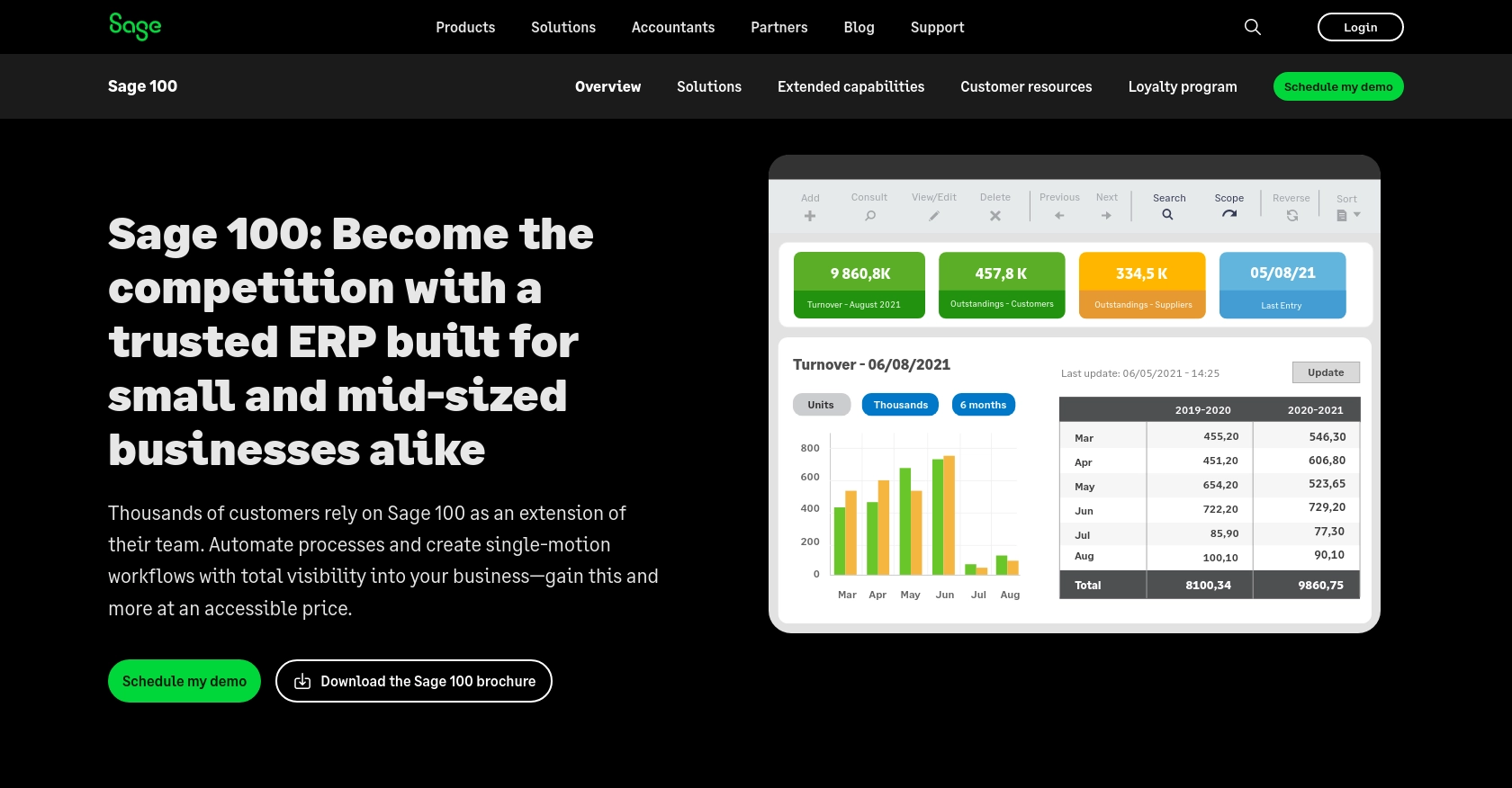
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution tailored for small to medium-sized businesses, offering robust features for accounting, inventory management, and customer relationship management. Its flexibility and scalability make it a preferred choice for businesses aiming to streamline their operations.
Integrating with the Sage 100 API allows developers to access and manipulate various data points within the system, such as tax schedules. This can be particularly useful for automating tax calculations and ensuring compliance with local tax regulations. For example, a developer might use the Sage 100 API to retrieve tax schedules and integrate them into a custom invoicing application, enhancing accuracy and efficiency.
Setting Up Your Sage 100 Test Environment
Before diving into the integration process, it's essential to set up a test environment for Sage 100. This will allow you to safely experiment with the API without affecting live data. Follow these steps to configure your Sage 100 test account:
Install and Configure the Sage 100 ODBC Driver
To interact with the Sage 100 API, you'll need to install and configure the Sage 100 ODBC driver. This driver facilitates the connection between your application and the Sage 100 database. Here's how to set it up:
- Access the ODBC Data Source Administrator on your system.
- Create a new Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Refer to the official Sage 100 documentation for detailed instructions on configuring the ODBC driver.
Create a Sage 100 Test Account
To begin testing, you'll need access to a Sage 100 test account. If you don't have one, follow these steps:
- Contact your Sage 100 administrator to request access to a sandbox environment.
- Ensure that the test account has the necessary permissions to access tax schedules and other relevant data.
Configure Sage 100 for API Access
Once your test account is ready, configure Sage 100 to allow API access:
- Log in to the Sage 100 interface using your test account credentials.
- Navigate to the Library Master module and select Setup > System Configuration.
- Enable the Client/Server ODBC Driver by checking the appropriate box under the ODBC Driver tab.
- Enter the server name or IP address where the ODBC application or service is running.
Test the ODBC Connection
Before proceeding, ensure that the ODBC connection is functioning correctly:
- Open the ODBC Data Source Administrator and locate your Sage 100 DSN.
- Click on the Debug tab and select Test Connection.
- If the connection is successful, you're ready to start interacting with the Sage 100 API.
By following these steps, you'll have a fully configured test environment for Sage 100, allowing you to safely develop and test your API integrations.
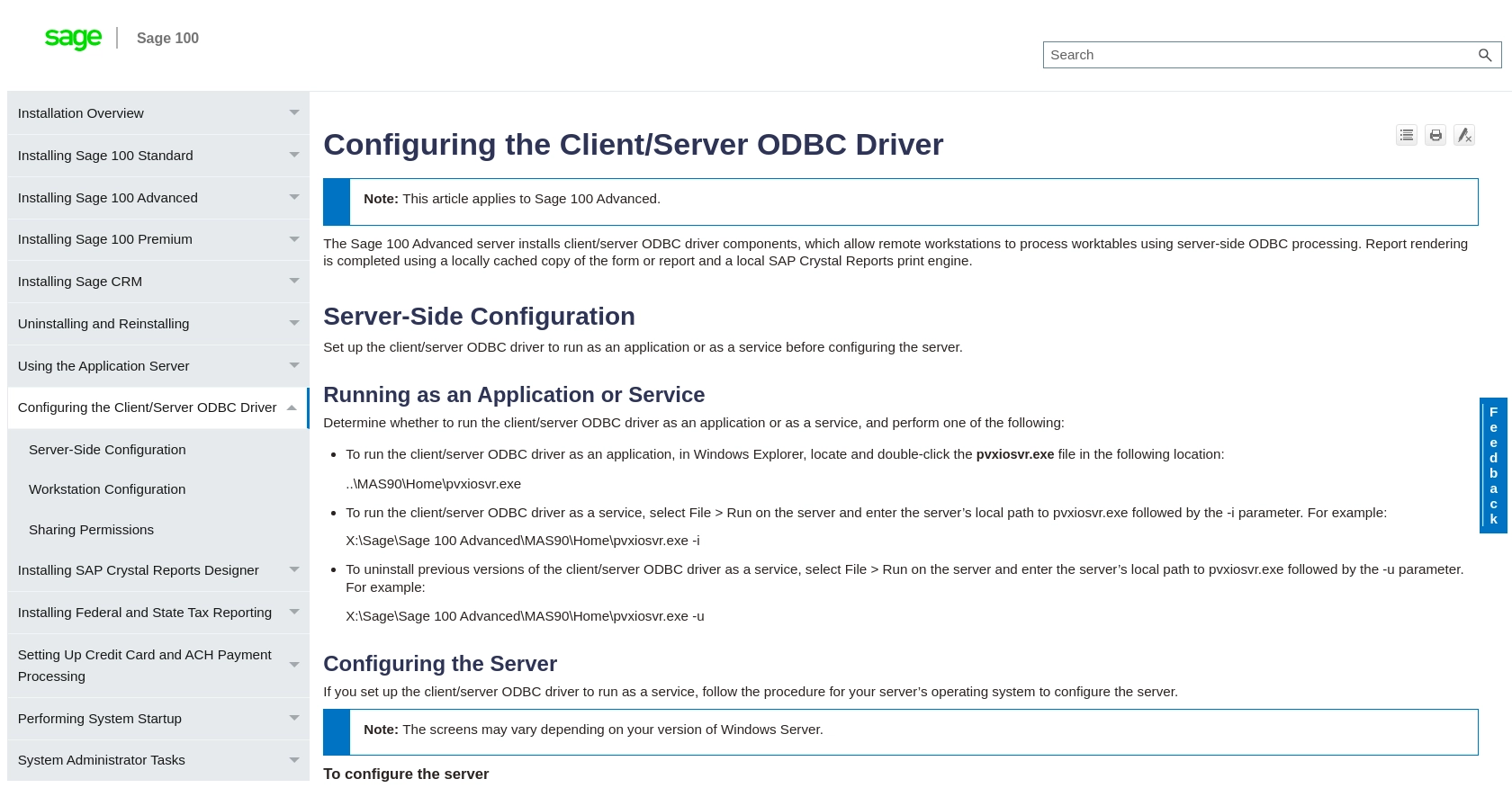
sbb-itb-96038d7
Executing API Calls to Retrieve Tax Schedules from Sage 100 Using JavaScript
To interact with the Sage 100 API and retrieve tax schedules, you'll need to set up your JavaScript environment and make the necessary API calls. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Sage 100 API
Before making API calls, ensure your JavaScript environment is ready:
- Ensure you have Node.js installed on your system. This will allow you to run JavaScript outside the browser.
- Use npm (Node Package Manager) to install any required packages, such as
axios
for making HTTP requests.
npm install axios
Writing JavaScript Code to Access Sage 100 Tax Schedules
With your environment set up, you can now write the JavaScript code to interact with the Sage 100 API:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://your-sage100-api-endpoint.com/tax-schedules';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Function to get tax schedules
async function getTaxSchedules() {
try {
const response = await axios.get(endpoint, { headers });
const taxSchedules = response.data;
console.log('Tax Schedules:', taxSchedules);
} catch (error) {
console.error('Error fetching tax schedules:', error);
}
}
// Execute the function
getTaxSchedules();
Replace Your_Token
with the token obtained from your Sage 100 setup. The code uses axios
to send a GET request to the Sage 100 API endpoint for tax schedules. If successful, it logs the retrieved tax schedules to the console.
Verifying API Call Success and Handling Errors
After running your JavaScript code, verify the success of your API call:
- Check the console output to ensure the tax schedules are displayed as expected.
- If you encounter errors, review the error messages for details. Common issues may include incorrect endpoint URLs or authentication failures.
Handle errors gracefully by using try-catch blocks, as shown in the code. This will help you manage exceptions and provide meaningful error messages.
By following these steps, you can effectively interact with the Sage 100 API using JavaScript to retrieve tax schedules, enhancing your application's functionality and efficiency.
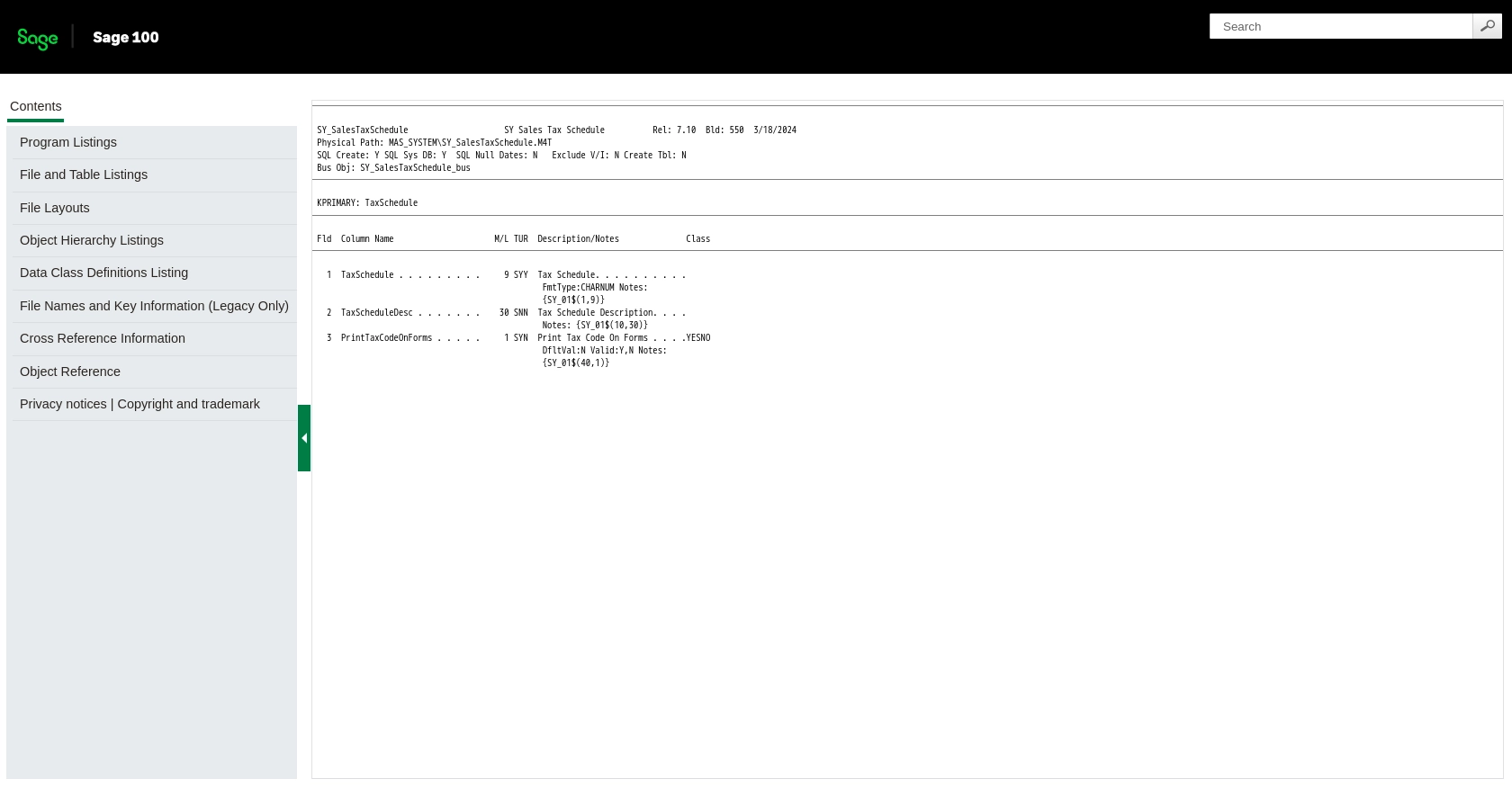
Conclusion and Best Practices for Using Sage 100 API with JavaScript
Integrating with the Sage 100 API using JavaScript provides a powerful way to automate and streamline your business processes, particularly in managing tax schedules. By following the steps outlined in this guide, you can efficiently set up your environment, execute API calls, and handle any potential errors.
Best Practices for Secure and Efficient API Integration with Sage 100
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to protect sensitive information like API tokens.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements. This will help maintain data consistency across systems.
- Error Handling: Implement robust error handling to manage exceptions and provide meaningful feedback to users. This includes logging errors and implementing fallback mechanisms where necessary.
Enhancing Integration Efficiency with Endgrate
For developers looking to streamline their integration processes further, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate offers a unified API endpoint that connects to multiple platforms, including Sage 100, providing an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxSchedule.htm?Highlight=SY_SalesTaxSchedule
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxScheduleDetail.htm?Highlight=SY_SalesTaxScheduleDetail
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxCodeDetail.htm?Highlight=SY_SalesTaxCodeDetail
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Library_Master/SY_SalesTaxCode.htm?Highlight=SY_SalesTaxCode
Ready to get started?