Using the Xero API to Get Invoices in Javascript
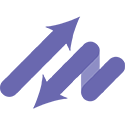
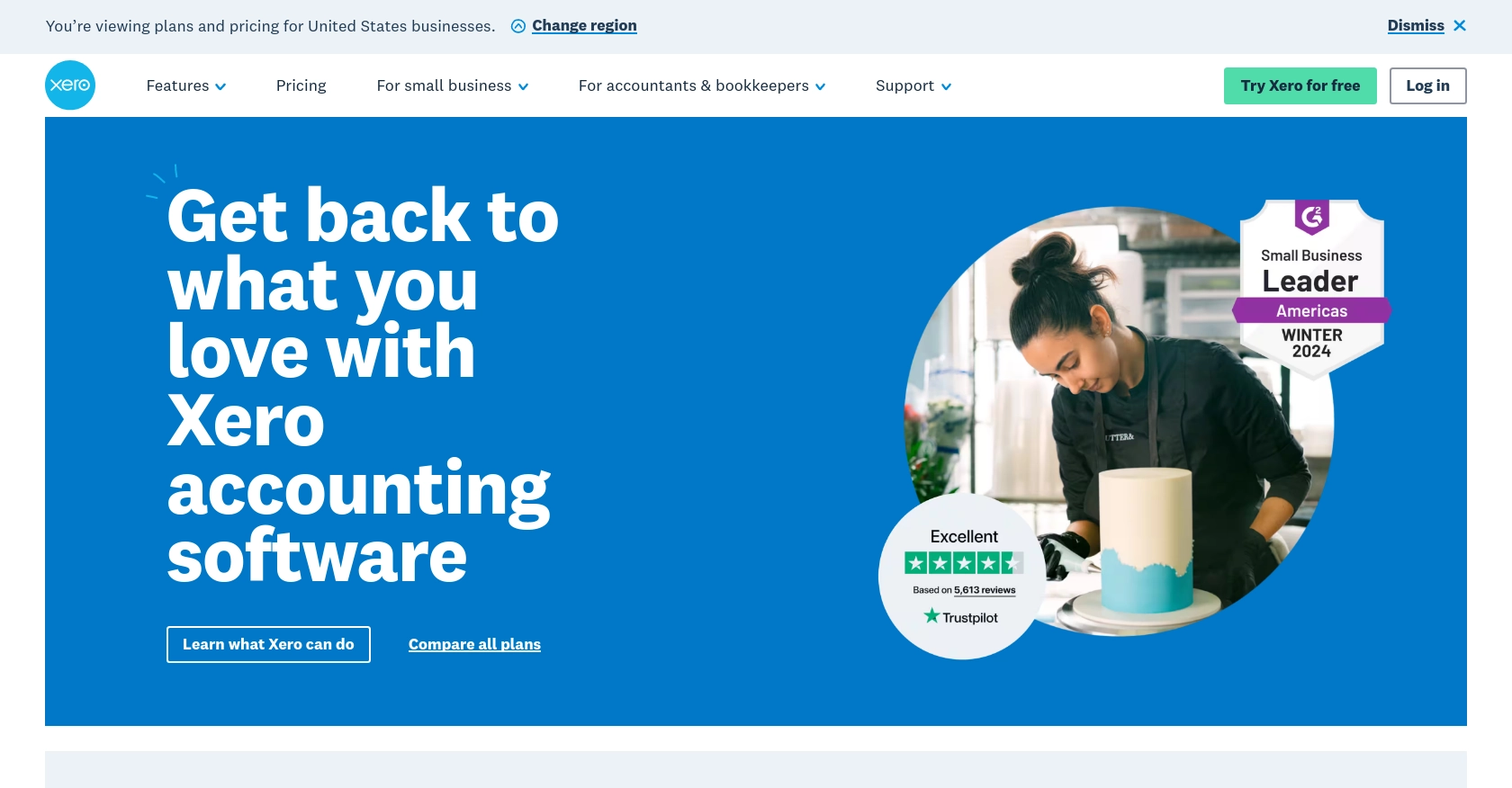
Introduction to Xero API for Invoice Management
Xero is a powerful cloud-based accounting software platform designed to help businesses manage their finances efficiently. It offers a wide range of features, including invoicing, bank reconciliation, and financial reporting, making it a popular choice for small to medium-sized enterprises.
For developers, integrating with Xero's API can streamline financial processes by automating tasks such as retrieving and managing invoices. For example, a developer might use the Xero API to automatically fetch invoice data and integrate it into a custom dashboard, providing real-time financial insights for business decision-makers.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with Xero's API to manage invoices, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Xero provides a free trial and sandbox environment specifically for developers to experiment with API calls.
Creating a Xero Developer Account
To begin, you'll need to create a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your developer account.
Setting Up a Xero Sandbox Organization
After creating your developer account, set up a sandbox organization to test your API interactions:
- Navigate to the "My Apps" section in the Xero Developer Portal.
- Click on "Create a new app" and provide the necessary details such as app name and company URL.
- Select "Web App" as the app type and ensure you have a valid redirect URI for OAuth 2.0 authentication.
- Once your app is created, you'll be provided with a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Configuring OAuth 2.0 Authentication for Xero API
Xero uses OAuth 2.0 for secure API authentication. Follow these steps to configure it:
- In the app settings, navigate to the "OAuth 2.0" section.
- Ensure that you have set the correct scopes for accessing invoices. You can find more information on scopes in the Xero OAuth 2.0 Scopes Documentation.
- Use the client ID and client secret to initiate the OAuth 2.0 authorization flow, as detailed in the Xero OAuth 2.0 Authorization Flow Guide.
With your sandbox account and OAuth 2.0 authentication configured, you're ready to start making API calls to retrieve invoices using JavaScript.
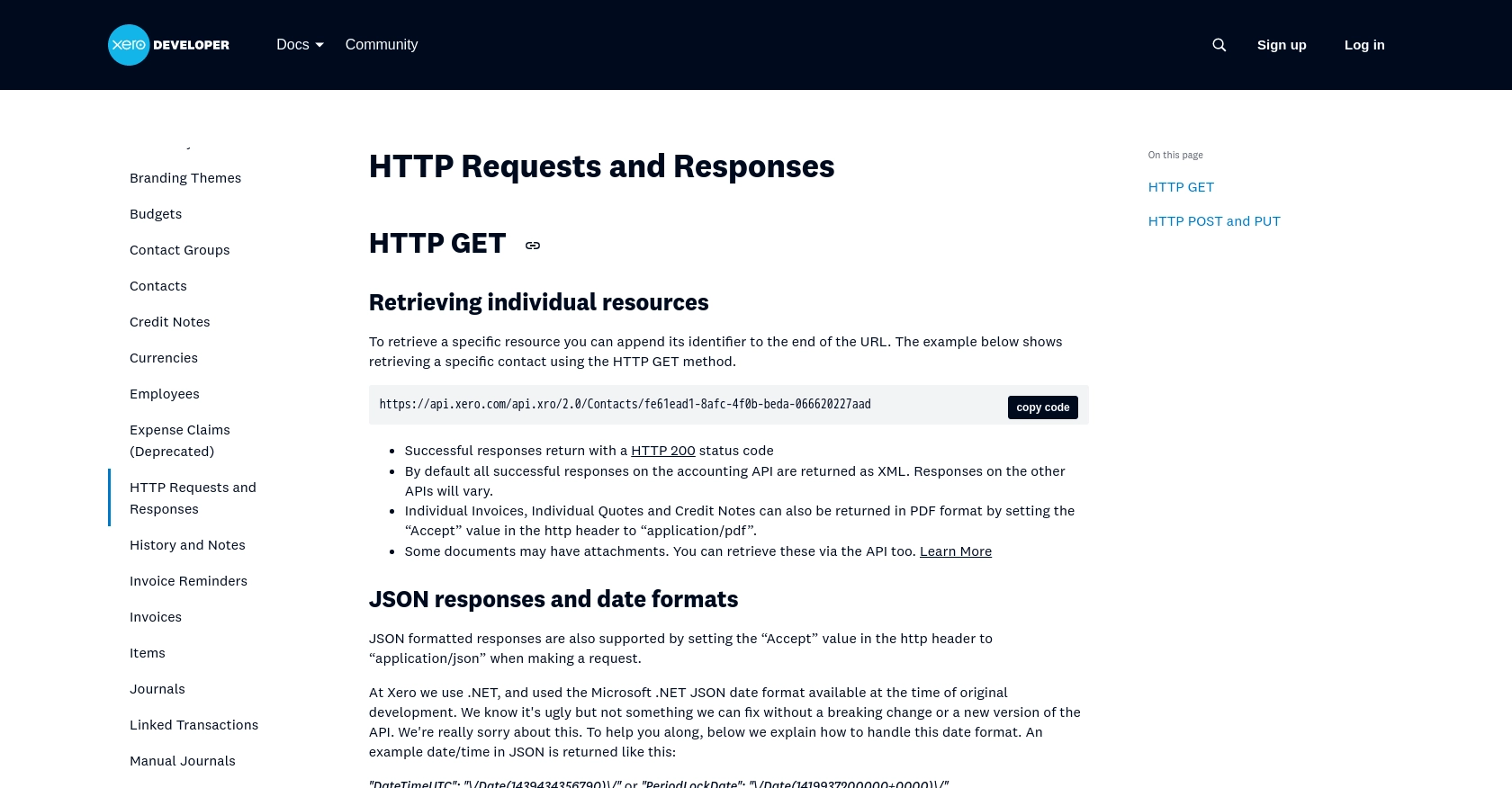
sbb-itb-96038d7
Making API Calls to Retrieve Xero Invoices Using JavaScript
With your Xero sandbox account and OAuth 2.0 authentication set up, you can now proceed to make API calls to retrieve invoices using JavaScript. This section will guide you through the necessary steps, including setting up your development environment and writing the code to interact with the Xero API.
Setting Up Your JavaScript Environment for Xero API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have these installed, create a new directory for your project and initialize it with the following command:
npm init -y
Next, install the Axios library, which will be used to make HTTP requests to the Xero API:
npm install axios
Writing JavaScript Code to Fetch Invoices from Xero
Create a new file named getXeroInvoices.js
and add the following code to it:
const axios = require('axios');
// Replace with your actual client ID, client secret, and access token
const clientId = 'Your_Client_ID';
const clientSecret = 'Your_Client_Secret';
const accessToken = 'Your_Access_Token';
// Set the API endpoint for retrieving invoices
const endpoint = 'https://api.xero.com/api.xro/2.0/Invoices';
// Set the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Function to fetch invoices
async function fetchInvoices() {
try {
const response = await axios.get(endpoint, { headers });
const invoices = response.data.Invoices;
console.log('Invoices:', invoices);
} catch (error) {
console.error('Error fetching invoices:', error.response.data);
}
}
// Call the function to fetch invoices
fetchInvoices();
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Access_Token
with the credentials obtained from your Xero app setup.
Running the JavaScript Code to Retrieve Xero Invoices
To execute the code and fetch invoices from Xero, run the following command in your terminal:
node getXeroInvoices.js
If successful, you should see a list of invoices printed in the console. This confirms that your API call was successful and that you have retrieved invoice data from Xero.
Handling Errors and Verifying API Call Success
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log any errors that occur during the API request. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 403 Forbidden: Verify that your app has the necessary scopes to access invoices.
- 429 Too Many Requests: Xero's API has rate limits, so ensure you are not exceeding them. For more details, refer to the Xero API Rate Limits Documentation.
By following these steps, you can effectively interact with the Xero API to retrieve invoices using JavaScript, enabling seamless integration with your financial systems.
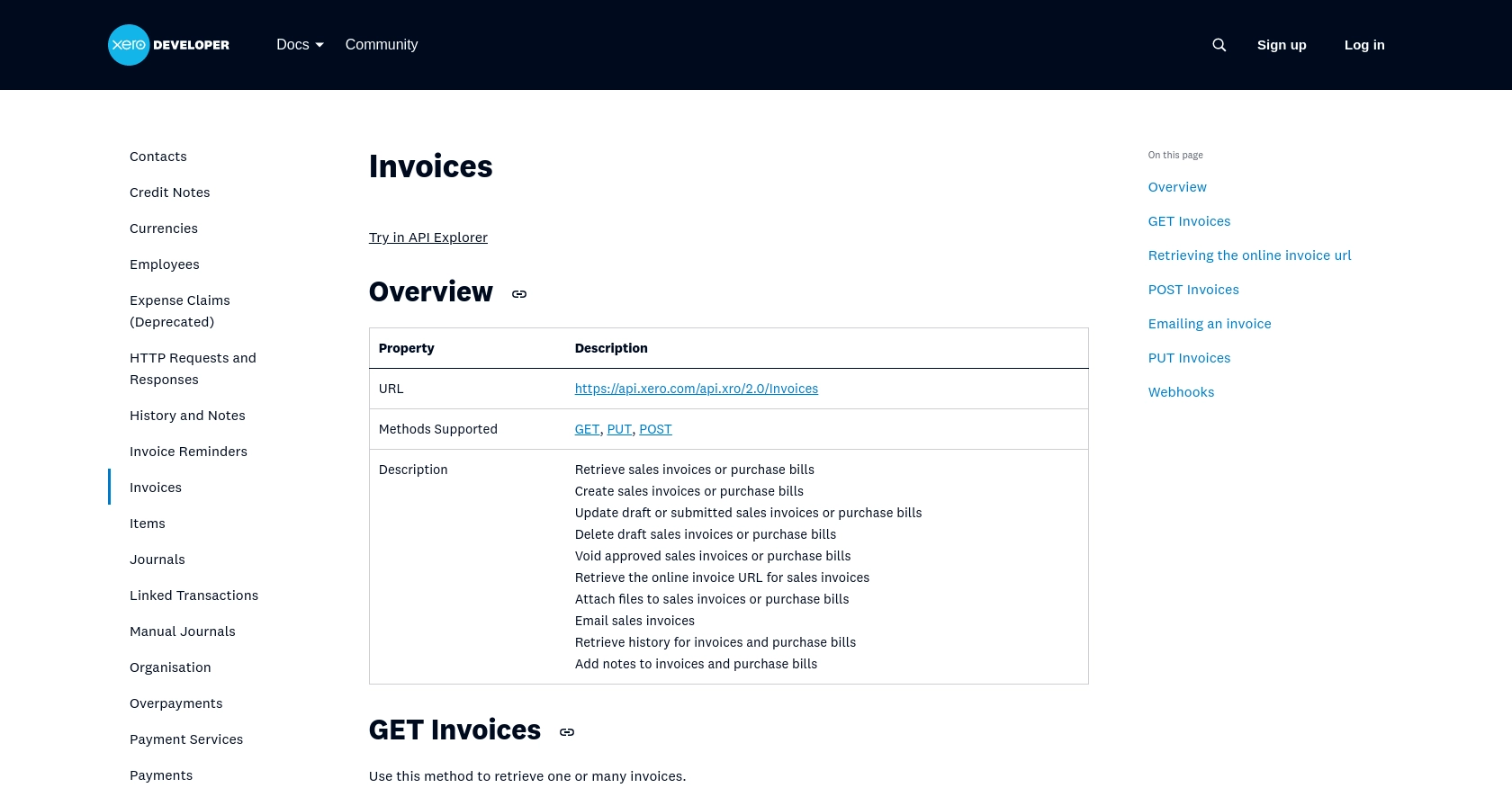
Conclusion and Best Practices for Xero API Integration Using JavaScript
Integrating with the Xero API to retrieve invoices using JavaScript can significantly enhance your financial management capabilities by automating data retrieval and integration processes. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth 2.0, and make API calls to access invoice data.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Ensure that your client ID, client secret, and access tokens are stored securely and not hard-coded in your application. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Xero's API rate limits to avoid disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Refer to the Xero API Rate Limits Documentation for more information.
- Data Transformation: Standardize and transform invoice data as needed to ensure compatibility with your systems. This can help maintain data integrity and consistency across platforms.
Streamlining Integrations with Endgrate
While integrating with Xero is a powerful way to enhance your financial processes, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this by providing a unified API endpoint that connects to various platforms, including Xero. By using Endgrate, you can save time and resources, allowing you to focus on your core product development while offering an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover how it can help you build once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/invoices
Ready to get started?