Using the Sage Accounting API to Create or Update Vendors (with Javascript examples)
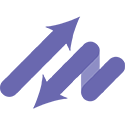
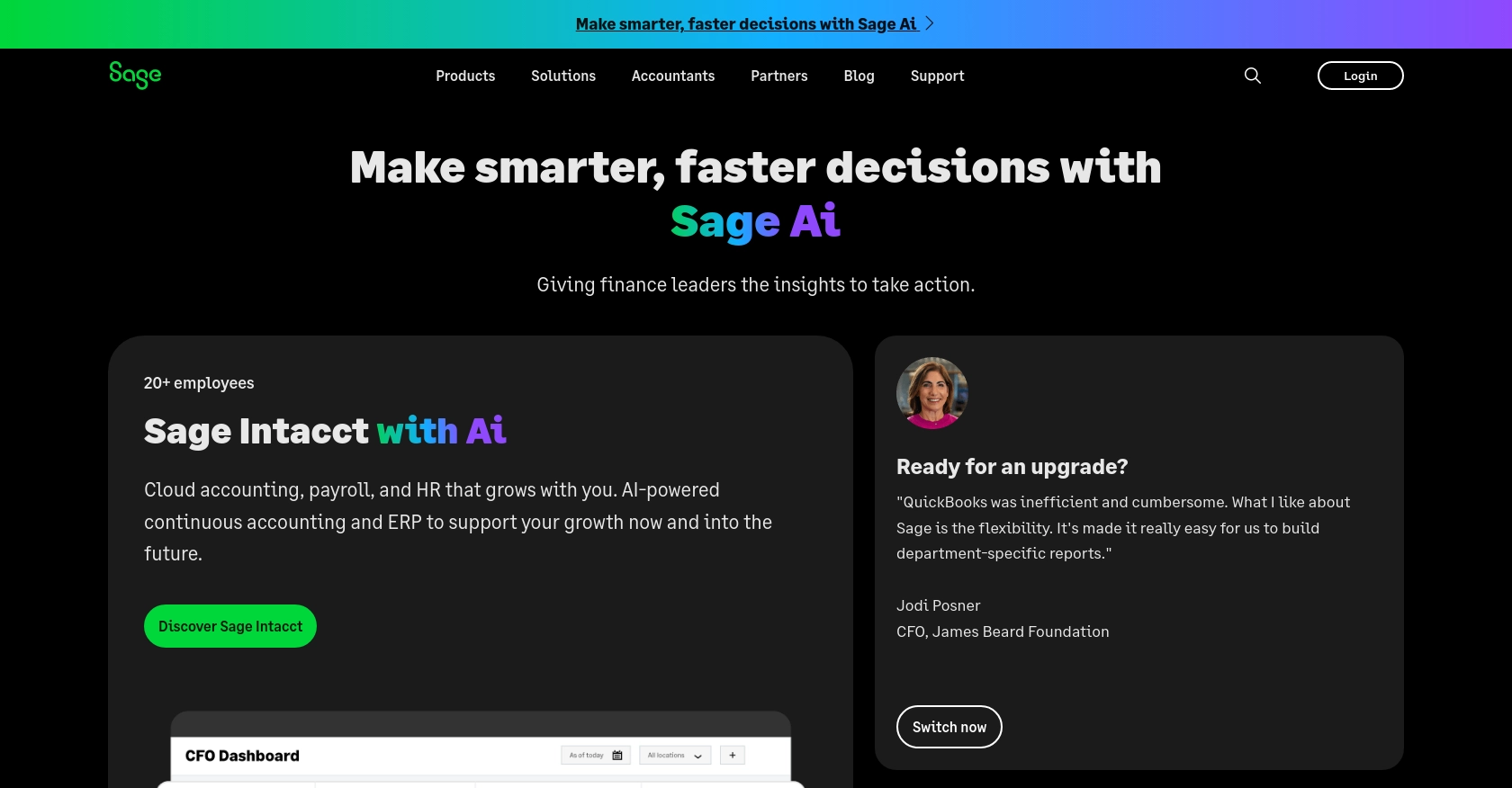
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage finances, track expenses, and streamline accounting processes. With its user-friendly interface and powerful features, Sage Accounting is a popular choice for businesses looking to enhance their financial management capabilities.
Integrating with the Sage Accounting API allows developers to automate and optimize various accounting tasks. For example, you can create or update vendor information directly from your application, ensuring that your financial data is always up-to-date and accurate. This integration can significantly reduce manual data entry and improve the efficiency of your accounting workflows.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting any live data. Sage provides developers with the ability to create trial accounts for development purposes, which can be used to test various features and functionalities.
Step 1: Sign Up for a Sage Developer Account
To begin, you'll need a Sage Developer account. This account will allow you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the instructions to complete the registration process.
Step 2: Create a Trial Business Account
Once you have a developer account, you can create a trial business account for testing purposes. Sage offers trial accounts for all supported regions and subscription tiers.
- Navigate to the Set up the Basics page.
- Select the appropriate region and subscription tier for your trial account.
- Follow the prompts to create your trial business account.
Step 3: Register Your Application
With your trial account set up, the next step is to create an application within the Sage Developer Portal. This will provide you with the necessary credentials to authenticate API requests.
- Log in to your Sage Developer account.
- Click on "Create App" and fill in the required details, including the app name and callback URL.
- Save your application to generate a Client ID and Client Secret.
- Refer to the Create an App guide for more information.
Step 4: Upgrade to a Developer Account
To fully utilize the Sage Accounting API, you may need to upgrade your trial account to a developer account. This provides extended access for testing your integration.
- Submit a request through the Upgrade Your Account page.
- Provide the necessary details, including your app name and Client ID.
- Wait for confirmation from the Sage team, which typically takes 3-5 working days.
Once you have completed these steps, you'll be ready to start making API calls to the Sage Accounting platform, allowing you to create or update vendor information seamlessly.
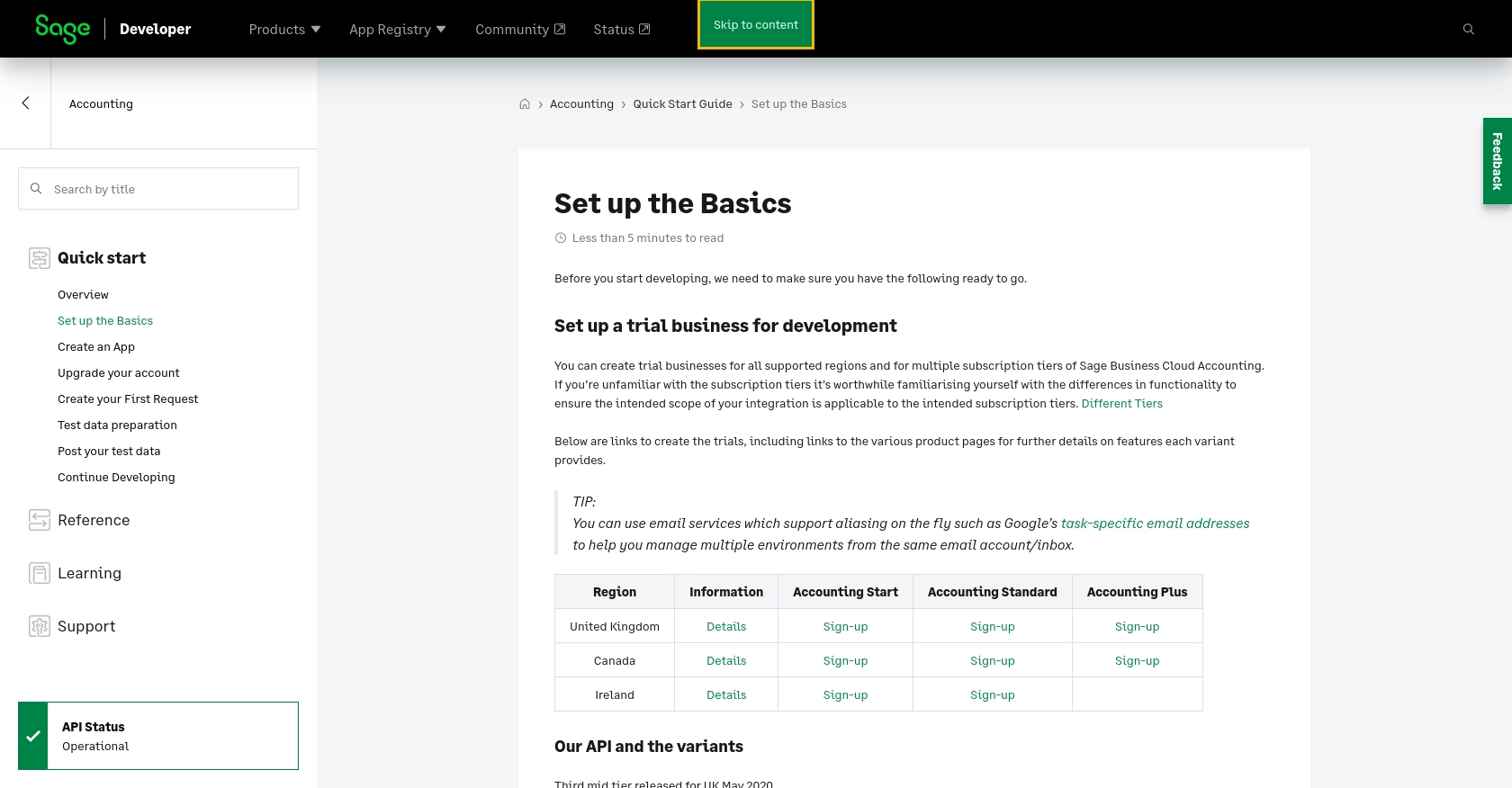
sbb-itb-96038d7
Making API Calls to Sage Accounting for Vendor Management Using JavaScript
To interact with the Sage Accounting API for creating or updating vendors, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Prerequisites for Using JavaScript with Sage Accounting API
Before you begin, ensure you have the following installed on your machine:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
You'll also need to install the Axios library, which simplifies making HTTP requests. Run the following command in your terminal:
npm install axios
Creating a Vendor with Sage Accounting API Using JavaScript
Let's start by writing a script to create a new vendor in Sage Accounting. Create a file named createVendor.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.accounting.sage.com/v3.1/contacts';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Define the vendor data
const vendorData = {
contact: {
name: 'New Vendor',
contact_type_ids: ['vendor'],
reference: 'Vendor123',
main_address: {
address_line_1: '123 Main St',
city: 'Anytown',
postal_code: '12345',
country_id: 'US'
}
}
};
// Make a POST request to create the vendor
axios.post(endpoint, vendorData, { headers })
.then(response => {
console.log('Vendor Created:', response.data);
})
.catch(error => {
console.error('Error Creating Vendor:', error.response.data);
});
Replace Your_Token
with the token obtained from your Sage Developer account. This script sends a POST request to the Sage Accounting API to create a new vendor with the specified details.
Updating a Vendor with Sage Accounting API Using JavaScript
To update an existing vendor, modify the script as follows. Create a file named updateVendor.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const vendorId = 'Existing_Vendor_ID';
const endpoint = `https://api.accounting.sage.com/v3.1/contacts/${vendorId}`;
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Define the updated vendor data
const updatedVendorData = {
contact: {
name: 'Updated Vendor Name',
reference: 'UpdatedReference'
}
};
// Make a PUT request to update the vendor
axios.put(endpoint, updatedVendorData, { headers })
.then(response => {
console.log('Vendor Updated:', response.data);
})
.catch(error => {
console.error('Error Updating Vendor:', error.response.data);
});
Replace Existing_Vendor_ID
with the ID of the vendor you wish to update. This script sends a PUT request to update the vendor's information.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. The above examples log the response data to the console, which includes the details of the created or updated vendor. If an error occurs, the error message is logged, helping you diagnose issues.
For more detailed error handling, you can inspect error.response.status
and error.response.data
to understand the nature of the error and take appropriate actions.
By following these steps, you can efficiently manage vendor information in Sage Accounting using JavaScript, streamlining your accounting processes and reducing manual data entry.
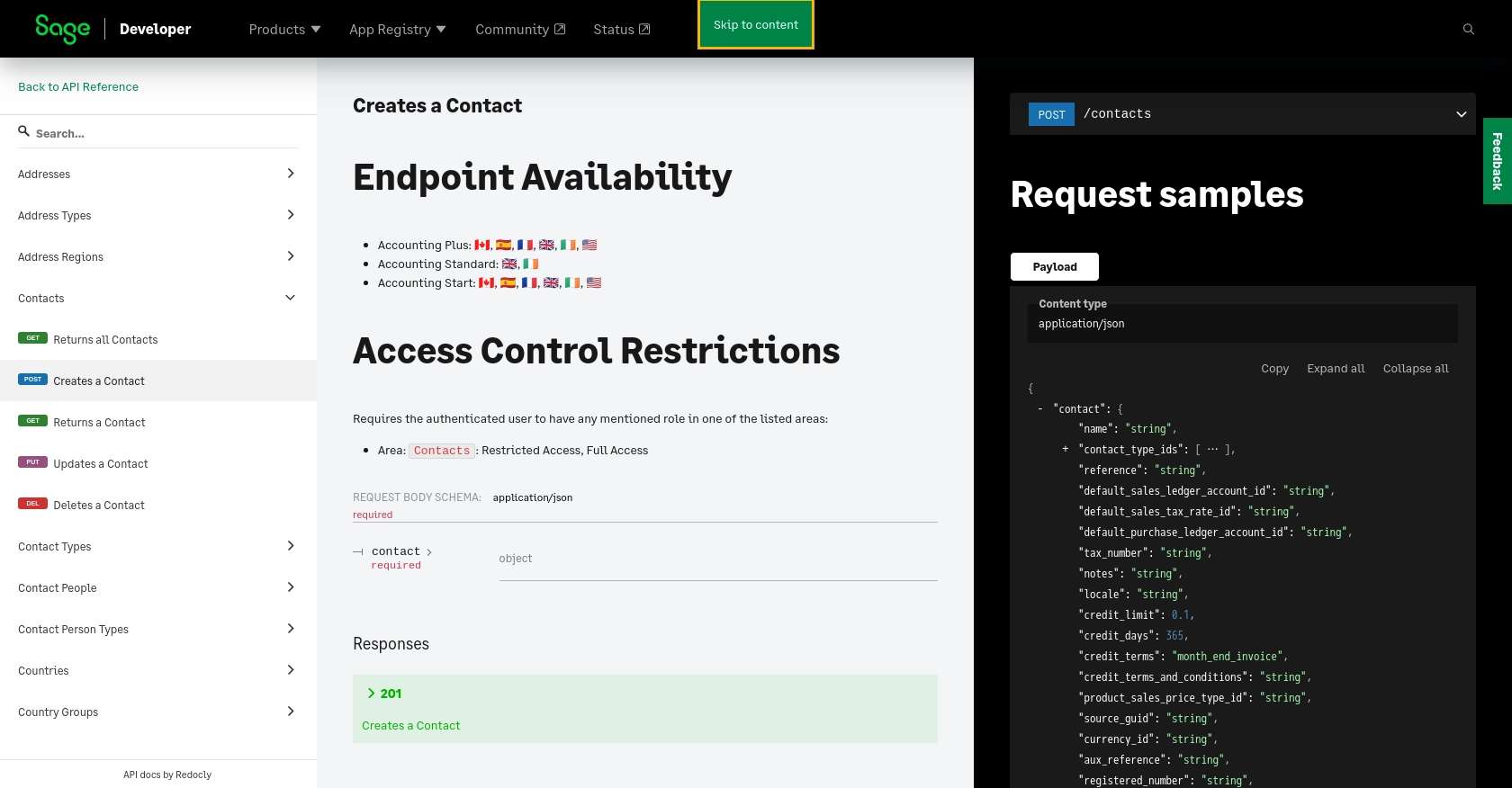
Conclusion and Best Practices for Using Sage Accounting API with JavaScript
Integrating with the Sage Accounting API using JavaScript provides a powerful way to automate vendor management and streamline accounting processes. By following the steps outlined in this guide, you can efficiently create and update vendor information, reducing manual data entry and enhancing data accuracy.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your API credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Validate API Responses: Always validate the responses from the API to ensure data integrity. Implement error handling to manage different HTTP status codes effectively.
- Standardize Data Formats: Ensure that data formats are consistent across your application to prevent discrepancies in vendor information.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Sage Accounting, allowing you to build once and deploy across multiple services. This approach saves time and resources, enabling you to focus on your core product development.
Visit Endgrate to learn more about how you can enhance your integration capabilities and provide a seamless experience for your users.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/postContacts
Ready to get started?