Using the Salesloft API to Create or Update Accounts in PHP
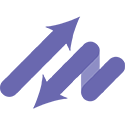
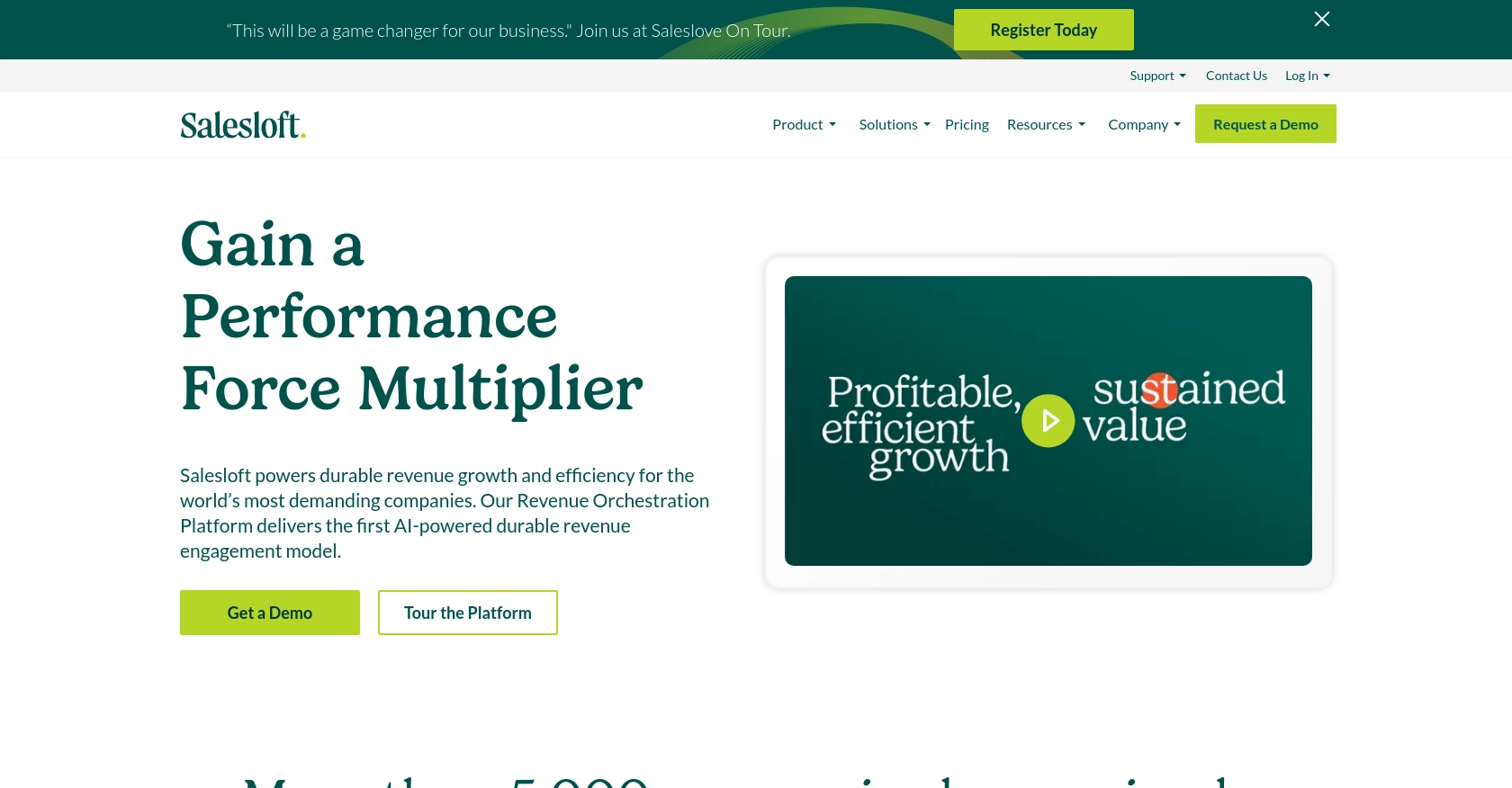
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform that enables sales teams to enhance their communication and streamline their workflows. With tools designed to improve prospecting, pipeline management, and customer engagement, Salesloft is a preferred choice for modern sales organizations looking to boost efficiency and effectiveness.
Integrating with the Salesloft API allows developers to automate and manage sales processes, such as creating or updating accounts. For example, a developer might use the Salesloft API to automatically update account information from an external CRM, ensuring that sales teams always have the most current data at their fingertips.
Setting Up Your Salesloft Test Account for API Integration
Before you can start integrating with the Salesloft API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesloft provides a sandbox environment where developers can test their applications.
Creating a Salesloft Sandbox Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This will give you access to the necessary tools and features to begin your integration work.
- Visit the Salesloft website and sign up for a free trial.
- Follow the on-screen instructions to complete your account setup.
- Once your account is created, log in to access the Salesloft dashboard.
Creating an OAuth App in Salesloft
Salesloft uses OAuth 2.0 for authentication, which requires you to create an OAuth app. This app will provide you with the credentials needed to authenticate API requests.
- Navigate to Your Applications in your Salesloft account.
- Select OAuth Applications and click on Create New.
- Fill in the required fields and click Save.
- After saving, you'll receive your Application Id (Client Id), Secret (Client Secret), and Redirect URI (Callback URL).
These credentials are essential for the next steps in the authorization process.
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you'll need to obtain an authorization code and exchange it for access and refresh tokens.
- Generate a request to the authorization endpoint using your Client Id and Redirect URI:
- Authorize the application when prompted. Upon approval, you'll receive a code in the redirect URI.
- Exchange this code for access and refresh tokens by making a POST request:
- Store the received access_token and refresh_token securely for future API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "YOUR_AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With these tokens, you can now authenticate your API requests to Salesloft.
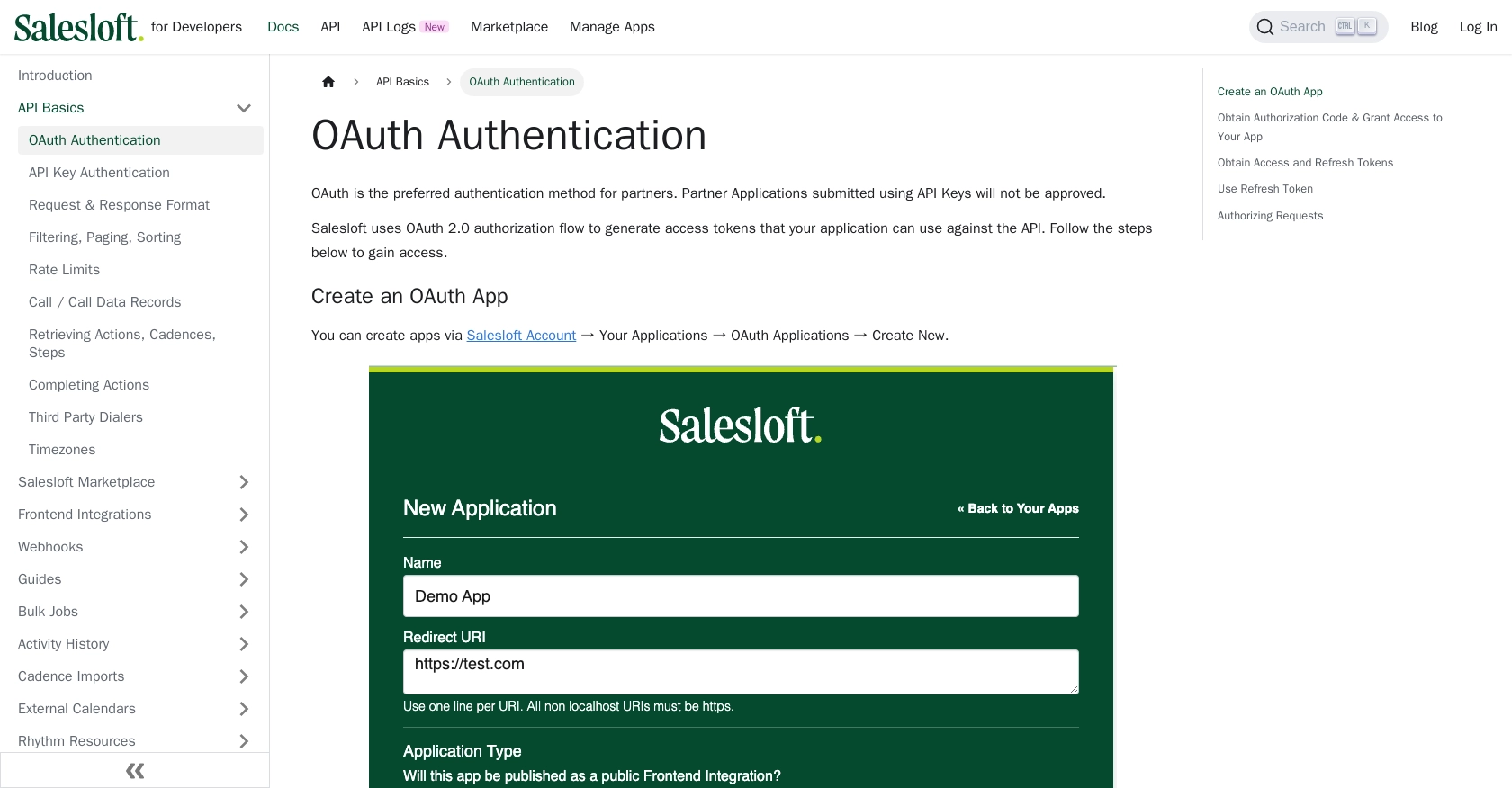
sbb-itb-96038d7
Making API Calls to Salesloft with PHP
To interact with the Salesloft API using PHP, you'll need to set up your environment and write the necessary code to create or update accounts. This section will guide you through the process, including setting up PHP, installing dependencies, and making API requests.
Setting Up PHP Environment for Salesloft API Integration
Ensure you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need Composer, a dependency manager for PHP, which you can get from here.
Once PHP and Composer are installed, create a new project directory and navigate to it in your terminal. Run the following command to initialize a new Composer project:
composer init
Next, install the Guzzle HTTP client, which will be used to make HTTP requests to the Salesloft API:
composer require guzzlehttp/guzzle
Creating or Updating Accounts with Salesloft API in PHP
With your environment set up, you can now write the PHP code to interact with the Salesloft API. Below is an example of how to create or update an account using the API.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$response = $client->post('https://api.salesloft.com/v2/accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json'
],
'json' => [
'name' => 'New Account Name',
'domain' => 'example.com',
'description' => 'Description of the account',
'phone' => '1234567890',
'website' => 'https://example.com',
'linkedin_url' => 'https://www.linkedin.com/company/example',
'twitter_handle' => '@example'
]
]);
if ($response->getStatusCode() == 200) {
echo "Account created or updated successfully.";
} else {
echo "Failed to create or update account.";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth process. The JSON payload includes fields such as name
, domain
, and description
, which you can customize based on your requirements.
Verifying API Request Success in Salesloft
After running the PHP script, you should verify that the account was created or updated successfully. You can do this by checking the Salesloft dashboard in your sandbox account. If the account appears with the specified details, the API call was successful.
Handling Errors and Salesloft API Error Codes
When making API requests, it's crucial to handle potential errors. The Salesloft API may return various HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 403 Forbidden: The request was valid, but the server is refusing action.
- 404 Not Found: The requested resource could not be found.
- 422 Unprocessable Entity: The request was well-formed but was unable to be followed due to semantic errors.
Ensure your code checks for these status codes and handles them appropriately, such as logging errors or retrying requests.
For more detailed information on error handling, refer to the Salesloft API documentation.
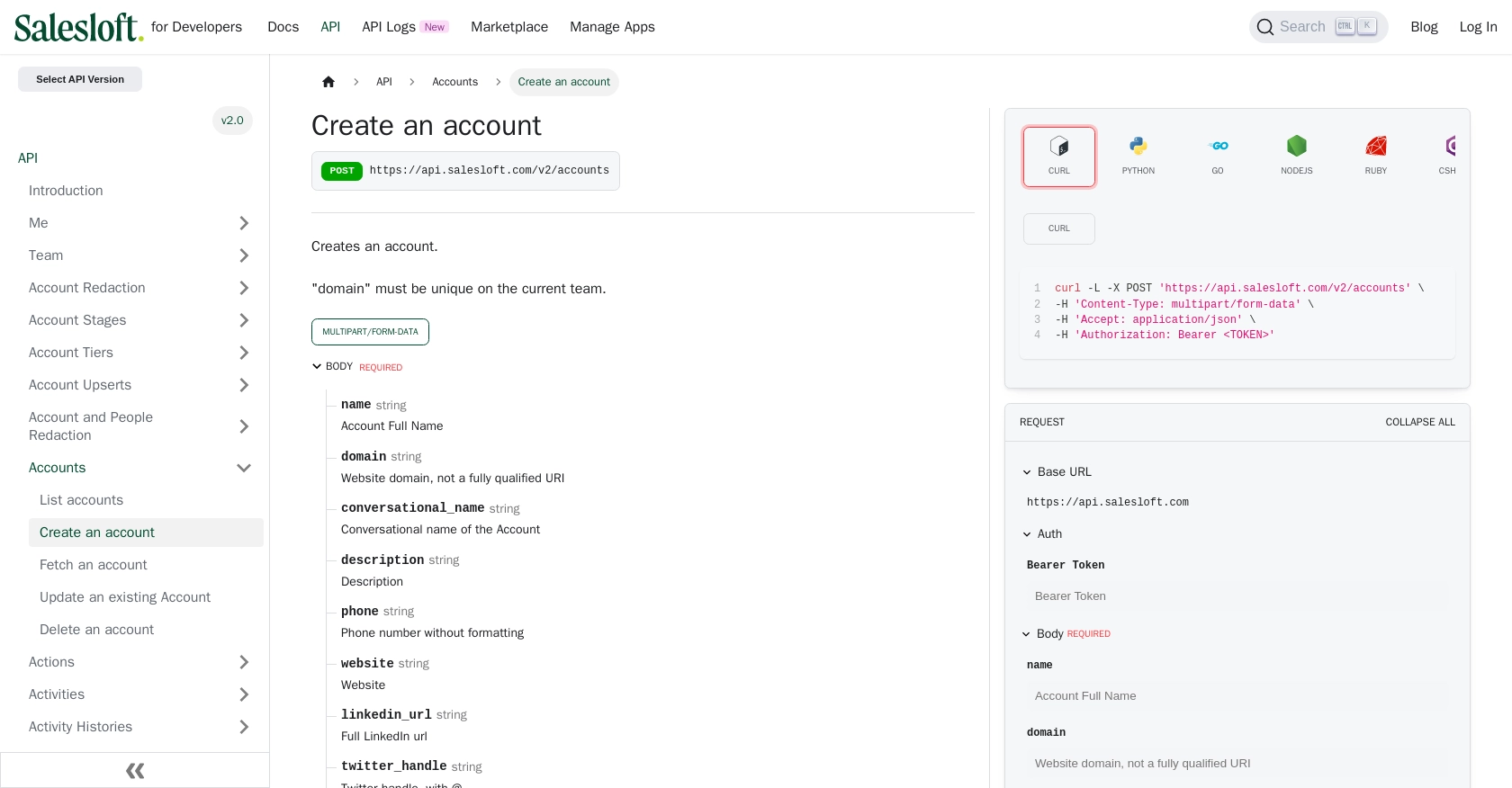
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using PHP allows developers to automate and streamline sales processes, enhancing the efficiency of sales teams. By following the steps outlined in this guide, you can successfully create or update accounts within Salesloft, ensuring that your data remains current and accessible.
Best Practices for Secure and Efficient Salesloft API Usage
- Secure Storage of Credentials: Always store your access and refresh tokens securely. Consider using environment variables or secure vaults to keep your credentials safe.
- Handling Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 cost per minute. Implement logic to handle rate limit responses and consider using a backoff strategy to retry requests. For more details, refer to the Salesloft API rate limits documentation.
- Data Transformation and Standardization: Ensure that data fields are consistently formatted and standardized before sending them to Salesloft. This helps maintain data integrity and reduces errors.
- Error Handling: Implement robust error handling in your code to manage different HTTP status codes and API errors effectively. This will improve the reliability of your integration.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Salesloft, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can benefit your development workflow.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/accounts-create/
Ready to get started?