Using the Salesloft API to Get Users (with PHP examples)
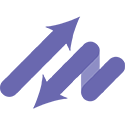
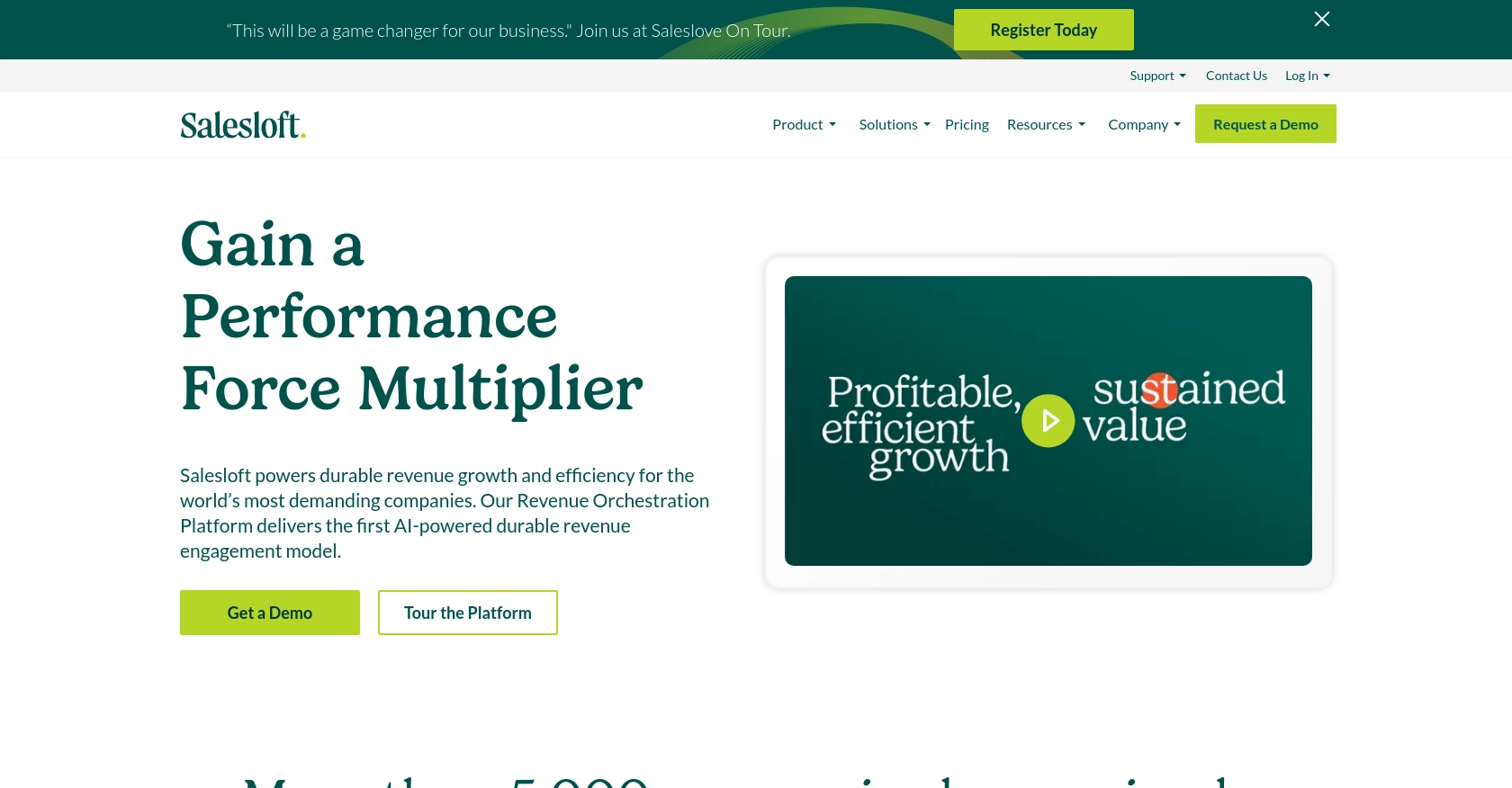
Introduction to Salesloft API Integration
Salesloft is a premier sales engagement platform designed to enhance the efficiency and effectiveness of sales teams. By providing tools for communication, automation, and analytics, Salesloft empowers sales professionals to connect with prospects and close deals more effectively.
Integrating with the Salesloft API allows developers to access and manage user data, enabling seamless integration with other business systems. For example, a developer might use the Salesloft API to retrieve user information and synchronize it with a CRM system, ensuring that sales teams have up-to-date data across platforms.
Setting Up Your Salesloft Test Account
Before you can start using the Salesloft API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesloft provides a sandbox environment for developers to test their integrations.
Creating a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This will give you access to the necessary tools and features to begin testing the API.
Generating OAuth Credentials for Salesloft API
Since the Salesloft API uses OAuth 2.0 for authentication, you'll need to create an OAuth application to obtain the necessary credentials. Follow these steps to set up your OAuth app:
- Log in to your Salesloft account.
- Navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New.
- Fill in the required fields, such as the application name and redirect URI, then click Save.
- Once saved, you'll receive your Client ID and Client Secret. Keep these credentials secure as you'll need them for API authentication.
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you'll need to obtain an access token. Here's how you can do it:
- Direct your users to the following authorization URL, replacing
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
with your app's credentials: - Once the user authorizes the application, you'll receive an authorization code in the redirect URI.
- Exchange this authorization code for an access token by making a POST request to the token endpoint:
- The response will include an
access_token
and arefresh_token
. Use the access token to authenticate your API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
For more detailed information, refer to the Salesloft OAuth Authentication documentation.
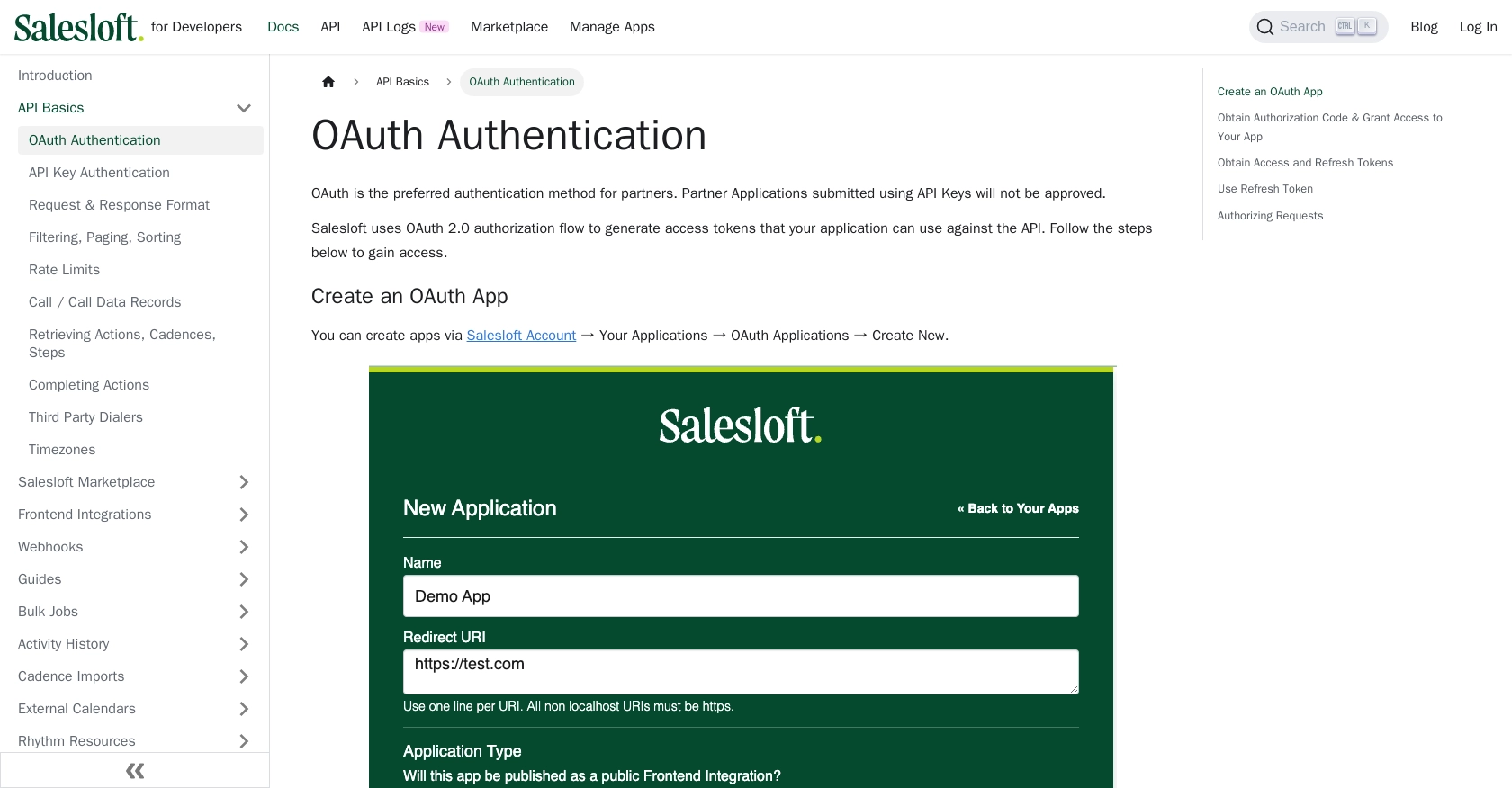
sbb-itb-96038d7
Making API Calls to Retrieve Users from Salesloft Using PHP
To interact with the Salesloft API and retrieve user data, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Salesloft API Integration
Before you begin, ensure that you have PHP installed on your machine. You will also need the cURL
extension enabled, as it allows you to make HTTP requests in PHP.
To check if cURL
is enabled, you can create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL
section in the output. If it's not enabled, you may need to install or enable it in your PHP configuration.
Writing PHP Code to Fetch Users from Salesloft API
Once your environment is ready, you can proceed with writing the PHP code to make an API call to Salesloft and retrieve user data. Here's a step-by-step guide:
- Create a new PHP file named
get_salesloft_users.php
and open it in your preferred code editor. - Add the following code to set up the API request:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$url = 'https://api.salesloft.com/v2/users';
// Initialize cURL session
$ch = curl_init($url);
// Set the required headers
$headers = [
'Authorization: Bearer ' . $accessToken,
'Accept: application/json'
];
// Configure cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display user information
foreach ($data['data'] as $user) {
echo 'ID: ' . $user['id'] . '<br>';
echo 'Name: ' . $user['name'] . '<br>';
echo 'Email: ' . $user['email'] . '<br><br>';
}
}
// Close the cURL session
curl_close($ch);
?>
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth authentication process.
Understanding the Salesloft API Response
When you run the script, it will make a GET request to the Salesloft API to fetch user data. The response will be in JSON format, containing user details such as ID, name, and email.
If the request is successful, the script will output the user information. If there's an error, it will display the error message.
Handling Errors and Verifying API Requests
It's crucial to handle errors gracefully when making API calls. The Salesloft API may return various HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 403 Forbidden: You don't have permission to access the resource.
- 404 Not Found: The requested resource could not be found.
- 422 Unprocessable Entity: There were validation errors in the request.
For more details on error handling, refer to the Salesloft Request & Response Format documentation.
After running the script, you can verify the retrieved users in your Salesloft sandbox account to ensure the data matches the API response.
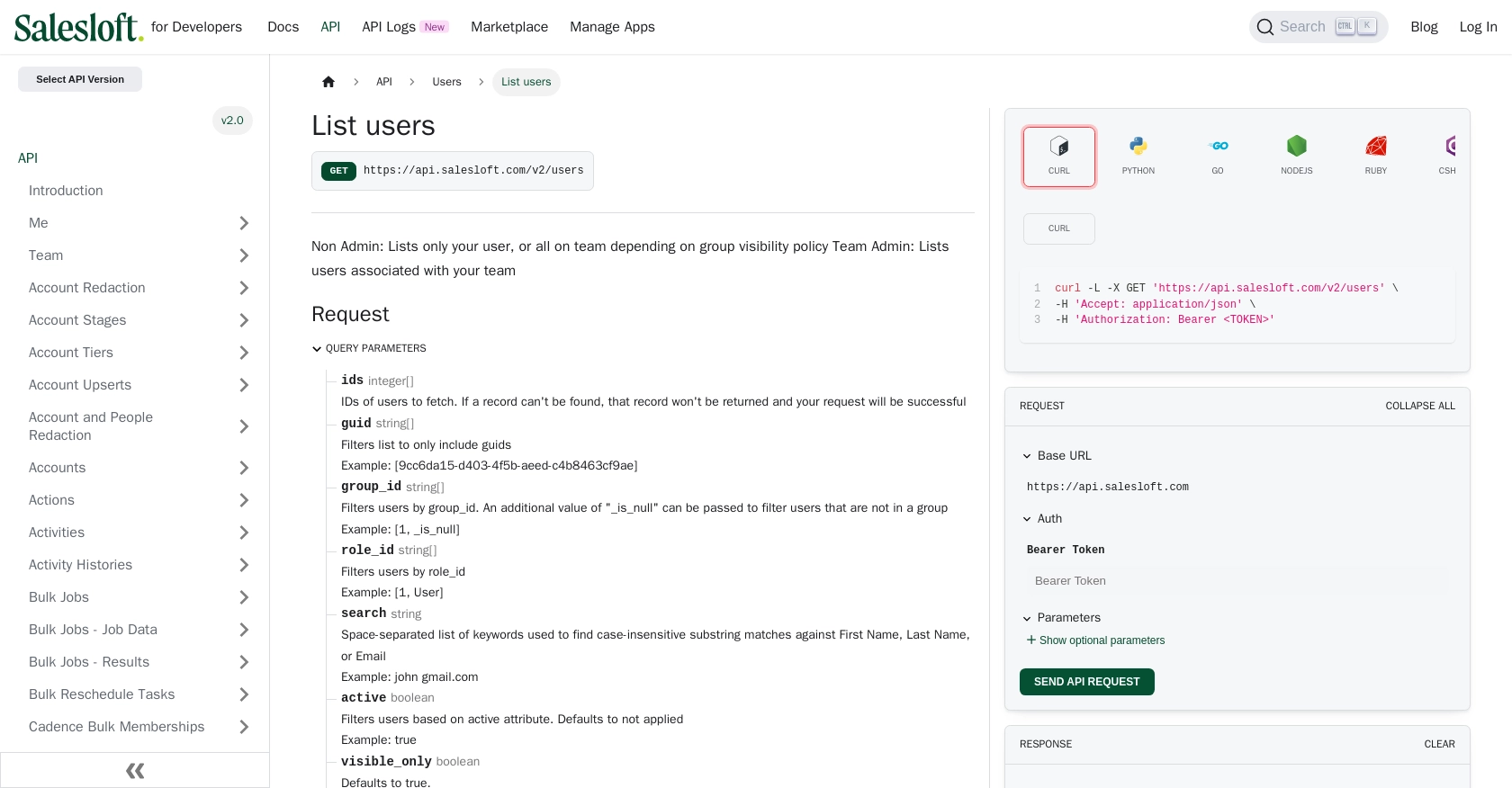
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using PHP provides a powerful way to manage user data and enhance your sales engagement processes. By following the steps outlined in this guide, you can efficiently retrieve user information and synchronize it with other business systems.
Best Practices for Secure and Efficient Salesloft API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as the client ID, client secret, and access tokens, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handling Rate Limits: Be mindful of the Salesloft API's rate limits, which are set at 600 cost per minute. Implement logic to handle rate limit responses and consider using a backoff strategy to retry requests when limits are reached. For more details, refer to the Salesloft Rate Limits documentation.
- Data Transformation and Standardization: When integrating data from Salesloft with other systems, ensure that data fields are transformed and standardized to match the target system's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging purposes.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including Salesloft, allowing you to build once for each use case and focus on your core product. Explore how Endgrate can save you time and resources by visiting Endgrate.
By following these best practices and leveraging tools like Endgrate, you can ensure a seamless and efficient integration experience with the Salesloft API.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/users-index/
Ready to get started?