Using the Sugar Market API to Create Opportunities (with PHP examples)
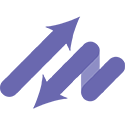
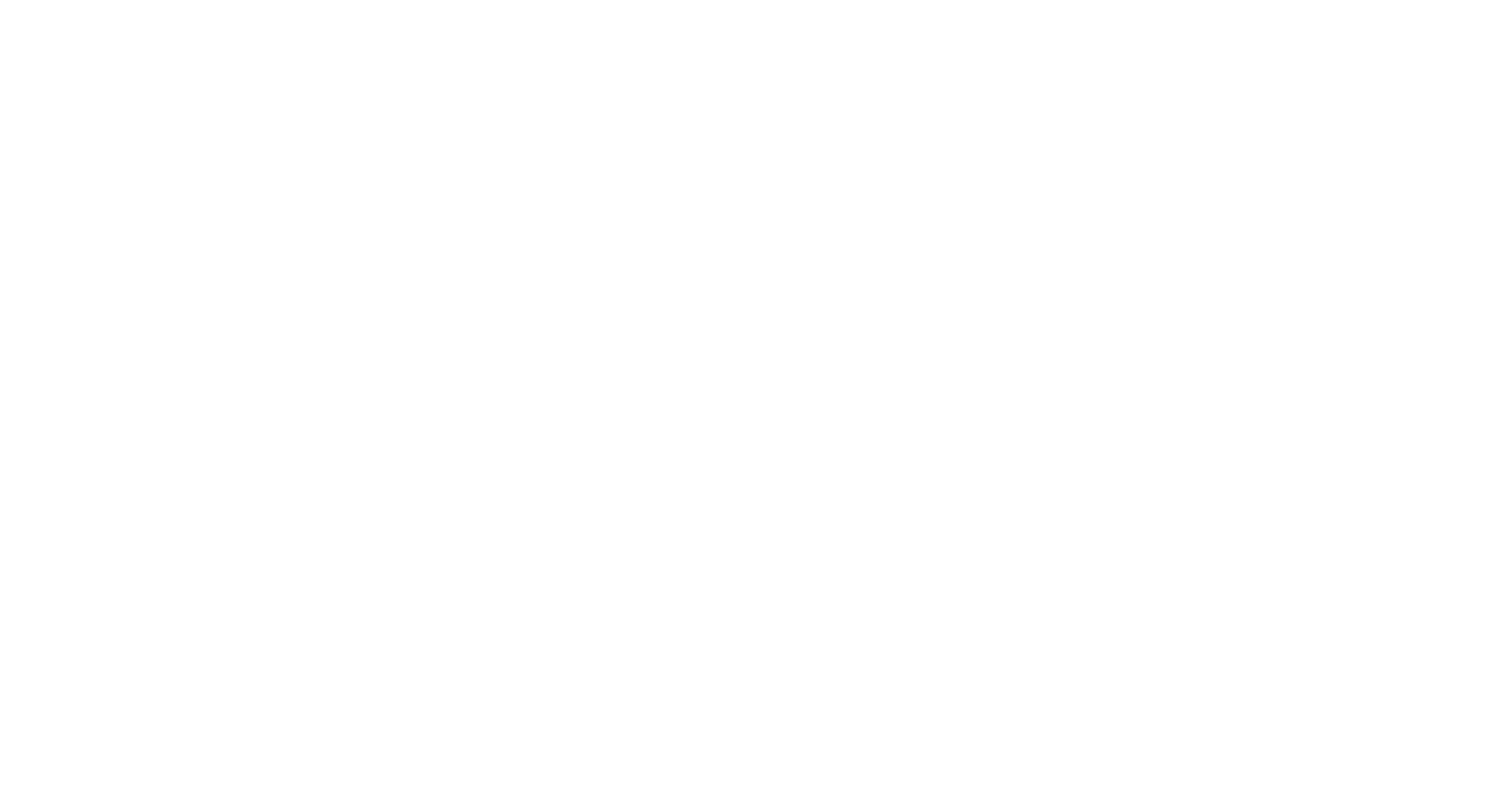
Introduction to Sugar Market
Sugar Market is a robust marketing automation platform that empowers businesses to streamline their marketing efforts and drive growth. With features like email marketing, lead nurturing, and analytics, Sugar Market provides a comprehensive solution for managing marketing campaigns and engaging with prospects.
Developers may want to integrate with the Sugar Market API to automate and enhance marketing processes. For example, creating opportunities directly through the API can help synchronize sales and marketing efforts, ensuring that potential leads are efficiently tracked and managed within the system.
This article will guide you through using PHP to interact with the Sugar Market API, focusing on creating opportunities to optimize your marketing strategy.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start creating opportunities using the Sugar Market API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the Sugar Market website. This will give you access to the necessary tools and features to begin your integration.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the account or developer settings.
- Create a new API application by providing the required details such as application name and description.
- Once the application is created, you will receive a client ID and client secret. Keep these credentials secure as they will be used to authenticate your API requests.
Configuring OAuth for Sugar Market API
To interact with the Sugar Market API, you may need to configure OAuth settings:
- In the API settings, locate the OAuth configuration section.
- Set up the redirect URI, which is the URL to which users will be redirected after authentication.
- Ensure that the necessary scopes are selected to allow your application to create opportunities.
For more detailed information on authentication, refer to the Sugar Market API documentation.
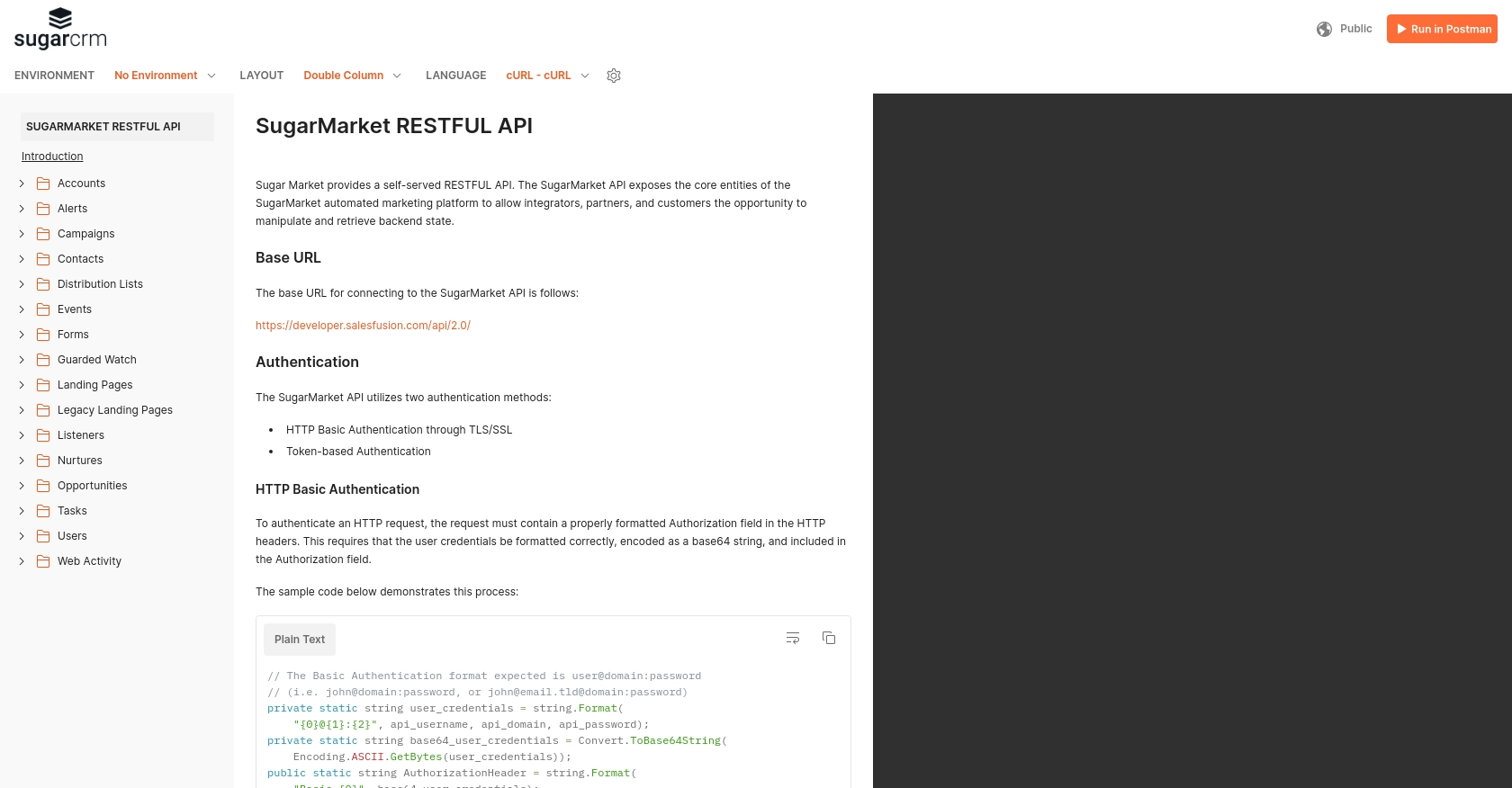
sbb-itb-96038d7
Making API Calls to Create Opportunities in Sugar Market Using PHP
To interact with the Sugar Market API and create opportunities, you'll need to set up your PHP environment and make HTTP requests to the appropriate endpoints. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to create opportunities.
Setting Up Your PHP Environment for Sugar Market API Integration
Before making API calls, ensure that your development environment is ready. You'll need PHP installed on your machine, along with the Composer package manager to handle dependencies.
Installing Required PHP Libraries for Sugar Market API
To make HTTP requests, you'll need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Create Opportunities in Sugar Market
With your environment set up, you can now write the PHP code to create opportunities using the Sugar Market API. Below is an example script:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiUrl = 'https://api.sugarmarket.com/opportunities';
$clientId = 'Your_Client_ID';
$clientSecret = 'Your_Client_Secret';
// Set up the request headers
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer ' . base64_encode("$clientId:$clientSecret")
];
// Define the opportunity data
$opportunityData = [
'name' => 'New Business Opportunity',
'amount' => 10000,
'stage' => 'Prospecting'
];
try {
// Make the POST request to create an opportunity
$response = $client->post($apiUrl, [
'headers' => $headers,
'json' => $opportunityData
]);
if ($response->getStatusCode() == 201) {
echo "Opportunity created successfully.";
} else {
echo "Failed to create opportunity.";
}
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace Your_Client_ID
and Your_Client_Secret
with your actual API credentials.
Verifying the Success of Your Sugar Market API Request
After running the script, check your Sugar Market sandbox account to verify that the opportunity has been created. If successful, the new opportunity should appear in your account.
Handling Errors and Troubleshooting Sugar Market API Calls
When making API calls, it's crucial to handle potential errors. The script above includes basic error handling using a try-catch block. If an error occurs, the exception message will be displayed, helping you diagnose issues.
For more detailed error information, refer to the Sugar Market API documentation.
Best Practices for Using the Sugar Market API
When integrating with the Sugar Market API, it's essential to follow best practices to ensure a smooth and secure implementation. Here are some recommendations:
- Securely Store API Credentials: Always store your client ID and client secret securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that the data fields you send and receive are standardized to maintain consistency across your application.
- Implement Error Handling: Use robust error handling to manage API call failures. Log errors and provide meaningful messages to help with troubleshooting.
Enhance Your Integration Strategy with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sugar Market. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts and enhance your marketing automation strategy by visiting Endgrate.
Read More
Ready to get started?