Using the Sugar Market API to Create Tasks in Python
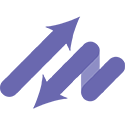
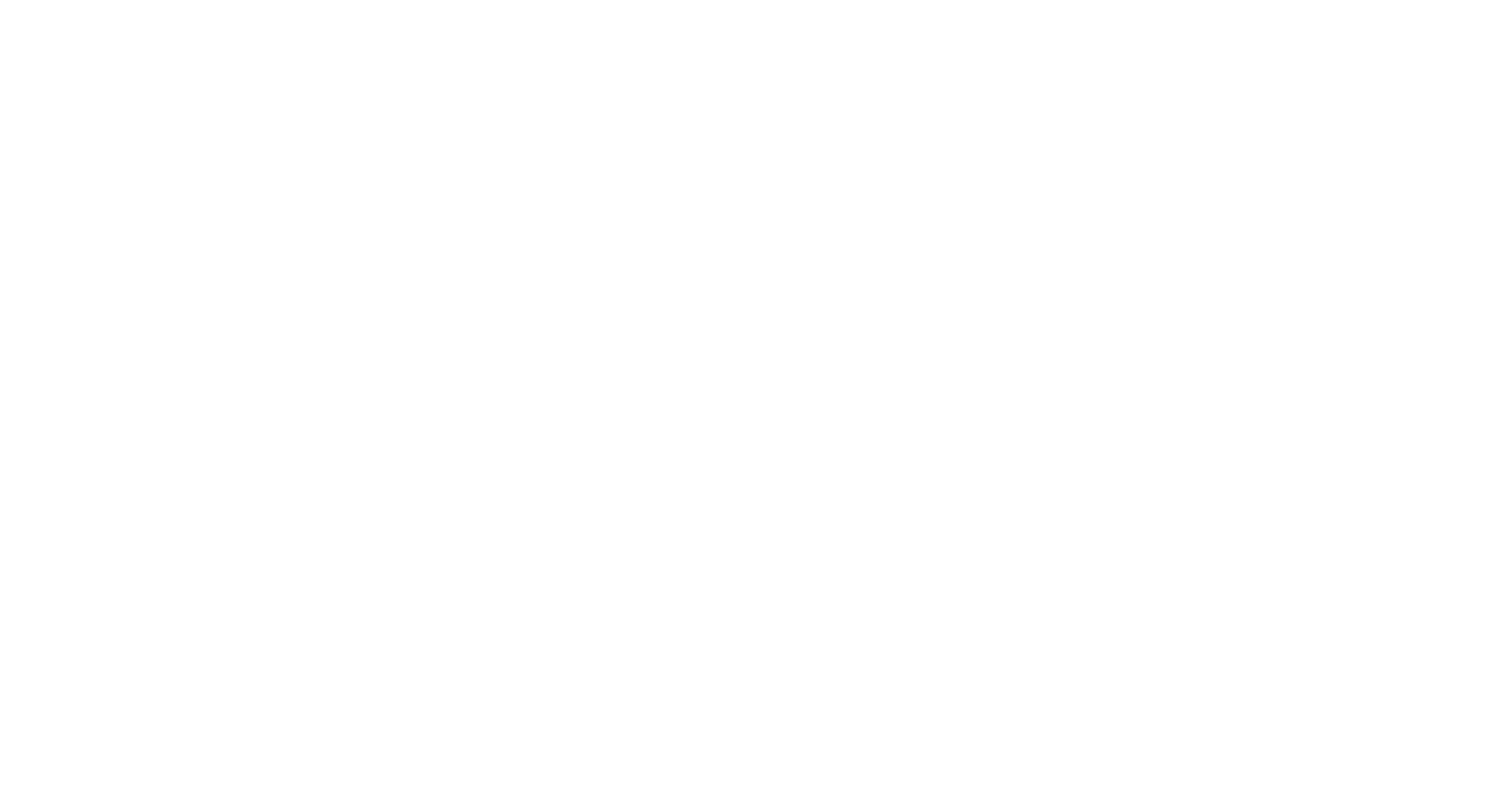
Introduction to Sugar Market
Sugar Market is a robust marketing automation platform designed to enhance the marketing efforts of B2B businesses. It offers a comprehensive suite of tools that include email marketing, lead nurturing, and analytics, all aimed at driving engagement and improving conversion rates.
Developers may want to integrate with Sugar Market's API to automate and streamline various marketing tasks. For example, using the Sugar Market API, a developer can create tasks programmatically, enabling seamless integration with other business systems and improving workflow efficiency.
Setting Up a Sugar Market Test/Sandbox Account
Before you can start integrating with the Sugar Market API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. This will give you access to the platform's features and allow you to test API interactions.
- Visit the Sugar Market website and navigate to the sign-up page.
- Follow the instructions to create your account, providing the necessary information such as your name, email, and company details.
- Once your account is set up, log in to access the Sugar Market dashboard.
Generating API Credentials for Sugar Market
To interact with the Sugar Market API, you'll need to generate API credentials. This involves creating an app within your Sugar Market account to obtain the necessary authentication details.
- Log in to your Sugar Market account and navigate to the API settings section.
- Create a new app by providing a name and description for your application.
- Once the app is created, you'll receive a client ID and client secret. These credentials are essential for authenticating your API requests.
- Store these credentials securely, as you'll need them to authorize API calls.
Understanding Sugar Market's Custom Authentication
Sugar Market uses a custom authentication method for its API. Ensure you understand how to implement this authentication in your Python code to successfully interact with the API.
Refer to the official Sugar Market API documentation for detailed instructions on setting up authentication and handling API requests.
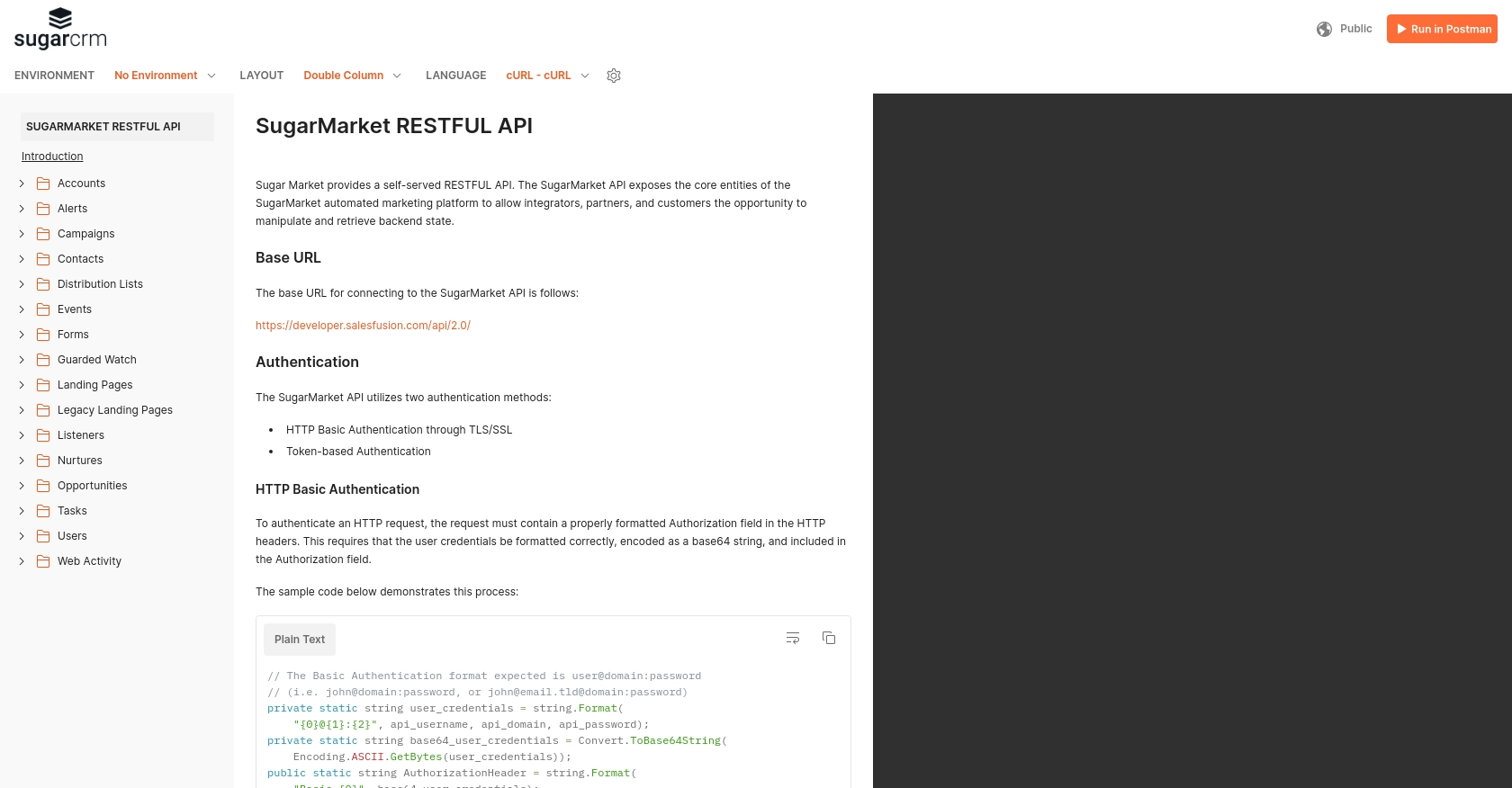
sbb-itb-96038d7
Making API Calls to Sugar Market Using Python
To interact with the Sugar Market API and create tasks programmatically, you'll need to set up your Python environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you have the right tools and knowledge to execute API calls effectively.
Setting Up Your Python Environment for Sugar Market API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1, so make sure your version is up to date. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Creating a Task in Sugar Market Using Python
With your environment set up, you can now write a Python script to create tasks in Sugar Market. The following example demonstrates how to authenticate and make a POST request to the Sugar Market API.
import requests
# Define the API endpoint for creating tasks
url = "https://api.sugarmarket.com/v1/tasks"
# Set up the headers with your API credentials
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the task data
task_data = {
"title": "Follow-up with Lead",
"due_date": "2023-12-01",
"priority": "High",
"description": "Contact the lead to discuss potential collaboration."
}
# Make the POST request to create a task
response = requests.post(url, json=task_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Task created successfully!")
else:
print("Failed to create task:", response.status_code, response.text)
Replace Your_Access_Token
with the token obtained from your Sugar Market account. This script sets up the necessary headers and task data, then sends a POST request to the API endpoint. If successful, you'll see a confirmation message.
Verifying Task Creation in Sugar Market
After running the script, log in to your Sugar Market account and navigate to the tasks section. You should see the newly created task listed there. This confirms that the API call was successful.
Handling Errors and Troubleshooting API Calls
When making API calls, it's essential to handle potential errors gracefully. The Sugar Market API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Always check the response status and handle errors accordingly.
For more detailed information on error codes, refer to the official Sugar Market API documentation.
Conclusion and Best Practices for Using Sugar Market API
Integrating with the Sugar Market API allows developers to automate and enhance marketing workflows, creating a seamless connection between various business systems. By following the steps outlined in this guide, you can efficiently create tasks in Sugar Market using Python, ensuring a streamlined process for managing marketing activities.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to manage sensitive information like client IDs and access tokens.
- Handling Rate Limits: Be aware of any rate limits imposed by the Sugar Market API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across different systems to facilitate smooth data integration and reporting.
- Error Handling: Implement robust error handling in your code to manage API response errors effectively. Log errors for monitoring and debugging purposes.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Sugar Market can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including Sugar Market.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With its intuitive integration experience, Endgrate enables you to build once for each use case, reducing the need for multiple custom integrations.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover how it can streamline your development process.
Read More
Ready to get started?