Using the Sugar Sell API to Create Records in Python
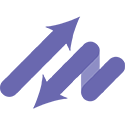
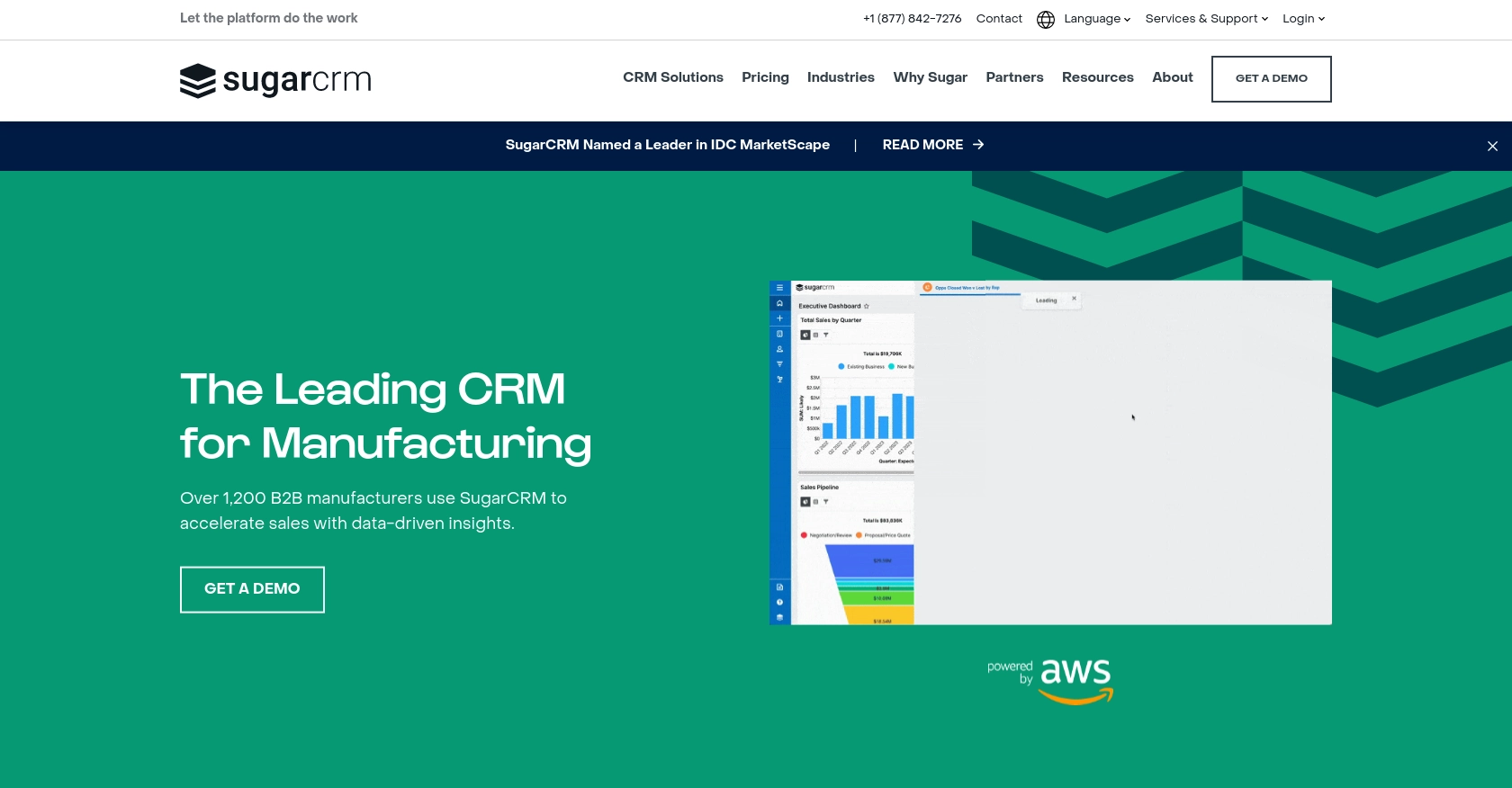
Introduction to Sugar Sell API
Sugar Sell is a powerful customer relationship management (CRM) platform designed to enhance sales processes and improve customer interactions. It offers a comprehensive suite of tools that help businesses manage leads, opportunities, and customer data efficiently.
Integrating with the Sugar Sell API allows developers to automate and streamline CRM tasks, such as creating and managing records. For example, a developer might use the Sugar Sell API to automatically create new customer records from an external data source, ensuring that the sales team has up-to-date information at their fingertips.
Setting Up Your Sugar Sell Test or Sandbox Account
Before you begin integrating with the Sugar Sell API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Sugar Sell Sandbox Account
If you don't already have a Sugar Sell account, you can sign up for a free trial or request a sandbox account through the SugarCRM website. This will provide you with a controlled environment to test your API integrations.
- Visit the SugarCRM website and navigate to the trial or sandbox request page.
- Fill out the necessary information and submit your request.
- Once your account is created, you'll receive login credentials via email.
Generate OAuth2 Credentials for Sugar Sell API
Sugar Sell uses OAuth2 for authentication, which requires you to generate specific credentials. Follow these steps to create an app and obtain the necessary tokens:
- Log in to your Sugar Sell account and navigate to the Admin section.
- Under OAuth Keys, click on Create OAuth Key.
- Fill in the required fields, such as Client Name and Redirect URI.
- Save the OAuth Key to generate your Client ID and Client Secret.
Authenticate Using OAuth2
To authenticate your API requests, you'll need to obtain an access token using the OAuth2 credentials. Here's how to do it:
import requests
# Define the token endpoint and payload
token_url = "https:///rest/v13.2/oauth2/token"
payload = {
"grant_type": "password",
"client_id": "sugar",
"client_secret": "",
"username": "",
"password": "",
"platform": "custom"
}
# Make a POST request to obtain the access token
response = requests.post(token_url, json=payload)
tokens = response.json()
# Extract the access token
access_token = tokens['access_token']
print("Access Token:", access_token)
Replace <site_url>
, <your_username>
, and <your_password>
with your actual Sugar Sell account details. The response will include an access_token
that you'll use for subsequent API calls.
For more detailed information on authentication, refer to the Sugar Sell API Authentication Documentation.
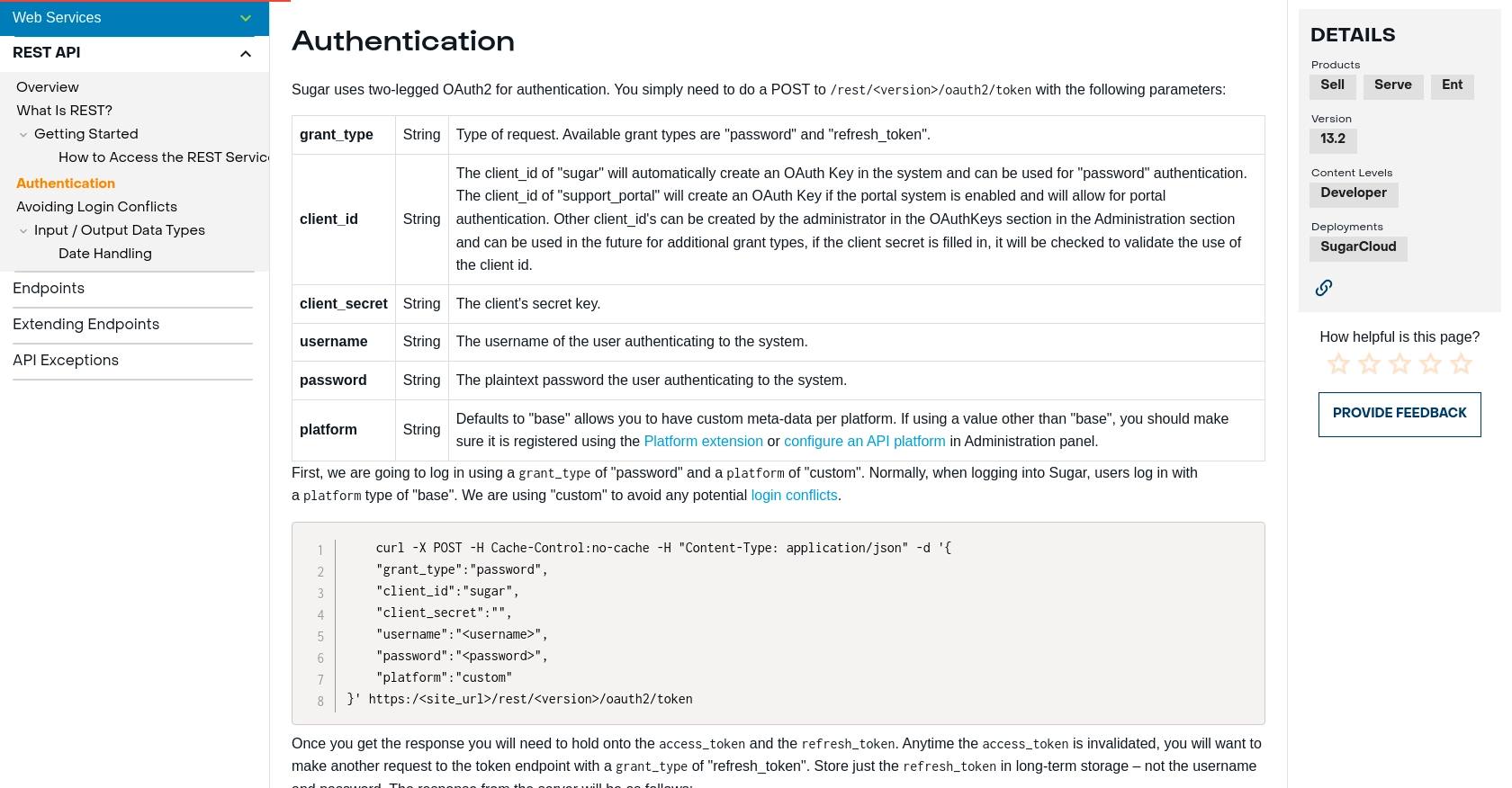
sbb-itb-96038d7
Making API Calls to Create Records in Sugar Sell Using Python
Now that you have set up your Sugar Sell account and obtained the necessary authentication tokens, you can proceed to make API calls to create records. This section will guide you through the process of using Python to interact with the Sugar Sell API and create new records efficiently.
Prerequisites for Python Integration with Sugar Sell API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Install the requests
library, which is essential for making HTTP requests:
pip install requests
Creating a Record in Sugar Sell Using Python
To create a new record in Sugar Sell, you will need to make a POST request to the appropriate endpoint. Follow these steps to create a record:
import requests
# Define the API endpoint for creating records
url = "https:///rest/v13.2/YourModule"
# Set the request headers, including the access token
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {access_token}"
}
# Define the data for the new record
data = {
"name": "New Record Name",
"description": "Description of the new record"
}
# Make the POST request to create the record
response = requests.post(url, json=data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Record created successfully:", response.json())
else:
print("Failed to create record:", response.status_code, response.text)
Replace <site_url>
with your Sugar Sell instance URL and YourModule
with the specific module you are targeting, such as Accounts
or Contacts
. The access_token
should be the token obtained from the authentication step.
Verifying Record Creation in Sugar Sell
After executing the code, verify that the record has been created by checking your Sugar Sell sandbox account. Navigate to the module where you attempted to create the record and confirm its presence.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors gracefully. Common error codes include:
- 400 Bad Request: The request was malformed or missing required fields.
- 401 Unauthorized: The access token is invalid or expired.
- 403 Forbidden: The user does not have permission to perform the action.
- 404 Not Found: The specified endpoint or resource does not exist.
For more detailed information on error handling, refer to the Sugar Sell API Endpoints Documentation.
Conclusion and Best Practices for Using Sugar Sell API
Integrating with the Sugar Sell API can significantly enhance your CRM capabilities by automating and streamlining various tasks. By following the steps outlined in this guide, you can efficiently create records in Sugar Sell using Python, ensuring your sales team has access to the most up-to-date customer information.
Best Practices for Secure and Efficient Sugar Sell API Integration
- Secure Storage of Credentials: Always store your OAuth2 credentials securely. Avoid hardcoding sensitive information directly in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent with your existing CRM data to maintain data integrity.
- Error Handling: Implement robust error handling to manage API call failures and provide meaningful feedback to users or logs.
For more detailed information on best practices, refer to the Sugar Sell API Documentation.
Streamline Your Integrations with Endgrate
While integrating with Sugar Sell API can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Sugar Sell. This allows you to build once and deploy across multiple services, saving time and resources.
Visit Endgrate to learn how you can simplify your integration process and focus on your core product development.
Read More
- https://endgrate.com/provider/sugarsell
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/#Authentication
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/endpoints/
Ready to get started?