Using the Teamleader API to Get Contacts (with Javascript examples)
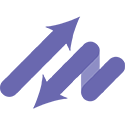
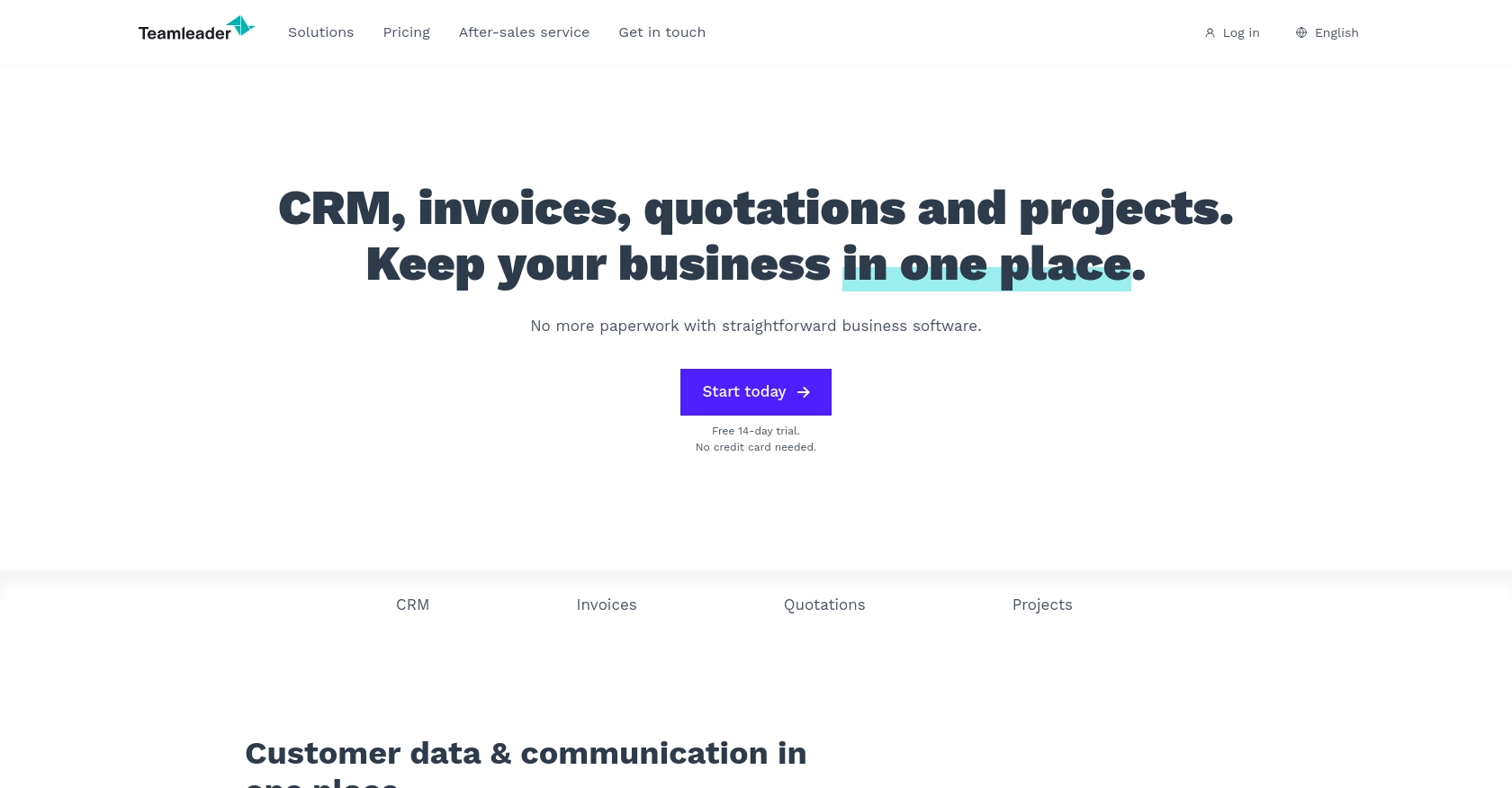
Introduction to Teamleader API
Teamleader is a versatile CRM and project management platform designed to help businesses streamline their operations. It offers a comprehensive suite of tools for managing contacts, projects, and invoices, making it an essential solution for businesses looking to enhance productivity and organization.
Integrating with the Teamleader API allows developers to automate and optimize various business processes. For example, by accessing contact information through the API, developers can synchronize customer data across different platforms, ensuring consistency and accuracy in customer interactions.
This article will guide you through using JavaScript to interact with the Teamleader API, specifically focusing on retrieving contact information. By following this tutorial, you'll learn how to efficiently access and manage contacts within the Teamleader platform.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start interacting with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Teamleader Account
If you don't already have a Teamleader account, you can sign up for a free trial on the Teamleader website. Follow the instructions to create your account. Once your account is set up, you'll have access to the Teamleader platform.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth for authentication, which requires you to create an app within your Teamleader account. This app will provide you with the necessary credentials to authenticate API requests.
- Log in to your Teamleader account.
- Navigate to the "Integrations" section from the dashboard.
- Select "Create a new app" and fill in the required details such as app name and description.
- Under the "Scopes" section, ensure you select the appropriate permissions for accessing contacts.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as you'll need them for authentication.
Generating Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate your API requests.
- Use the OAuth 2.0 authorization flow to obtain an access token. This typically involves redirecting users to a Teamleader authorization URL where they can grant permissions.
- Once authorized, you'll receive an authorization code, which you can exchange for an access token by making a POST request to the Teamleader token endpoint.
- Store the access token securely, as it will be used in the headers of your API requests to authenticate them.
For more detailed information on OAuth authentication, refer to the Teamleader API Documentation.
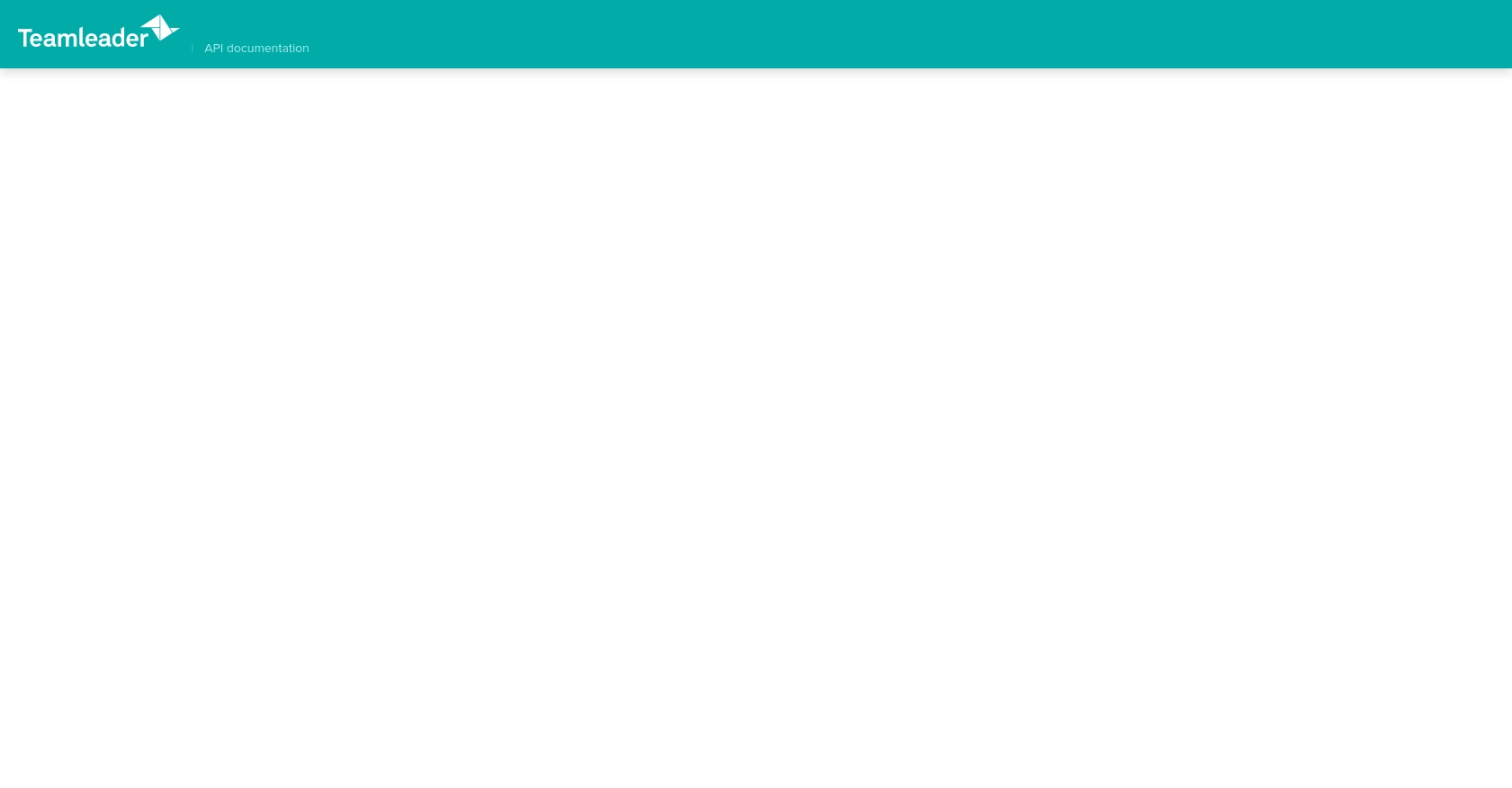
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Teamleader Using JavaScript
To interact with the Teamleader API and retrieve contact information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Teamleader API Integration
Before making API calls, ensure that you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside of a browser.
- Download and install Node.js from the official website.
- Once installed, open your terminal or command prompt and verify the installation by running
node -v
andnpm -v
. - Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library, which simplifies making HTTP requests, by running
npm install axios
.
Writing JavaScript Code to Fetch Contacts from Teamleader API
With your environment set up, you can now write the JavaScript code to make a GET request to the Teamleader API and retrieve contact information.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.teamleader.eu/contacts.list';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to fetch contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.data;
console.log('Contacts retrieved successfully:', contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function to fetch contacts
getContacts();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth authentication process. This code uses Axios to send a GET request to the Teamleader API endpoint for contacts. If successful, it logs the retrieved contacts to the console.
Verifying Successful API Requests and Handling Errors
After running the code, you should see the list of contacts printed in your console. To verify the request's success, you can cross-check the data with your Teamleader sandbox account.
In case of errors, the code includes error handling to log the error message. Common issues might include invalid access tokens or insufficient permissions. Ensure your access token is valid and that the necessary scopes are granted.
For more detailed error information, refer to the Teamleader API Documentation.
Conclusion and Best Practices for Using Teamleader API with JavaScript
Integrating with the Teamleader API using JavaScript provides a powerful way to automate and streamline your business processes. By following the steps outlined in this article, you can efficiently retrieve contact information and ensure data consistency across platforms.
Best Practices for Secure and Efficient API Integration with Teamleader
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Teamleader API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Teamleader is transformed and standardized to match your application's data structures. This will help maintain consistency and improve data integrity.
Enhancing Integration Capabilities with Endgrate
If managing multiple integrations is becoming a challenge, consider using a tool like Endgrate. Endgrate simplifies the integration process by providing a single API endpoint that connects to multiple platforms, including Teamleader. This allows you to focus on your core product while outsourcing the complexities of integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
Ready to get started?