Using the Wrike API to Get Tasks (with PHP examples)
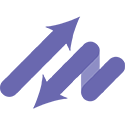
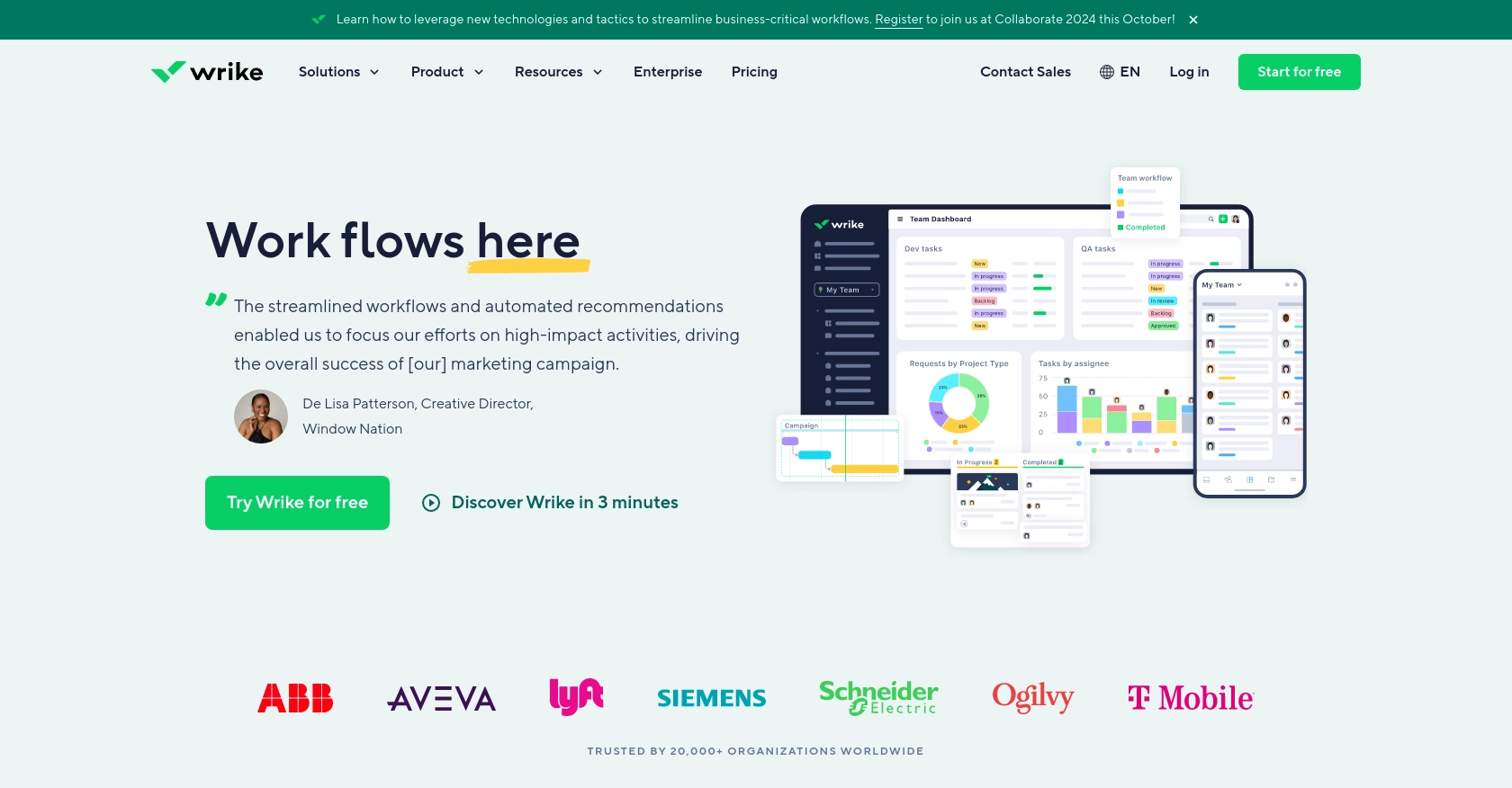
Introduction to Wrike API for Task Management
Wrike is a powerful project management and collaboration platform that enables teams to streamline their workflows and enhance productivity. With features like task management, time tracking, and reporting, Wrike is a popular choice for businesses looking to optimize their project management processes.
Integrating with Wrike's API allows developers to automate and customize task management workflows. For example, a developer might use the Wrike API to retrieve tasks and integrate them into a custom dashboard, providing team members with real-time updates and insights.
Setting Up Your Wrike Test/Sandbox Account for API Integration
Before diving into the Wrike API for task management, it's essential to set up a test or sandbox account. This allows developers to safely experiment with API calls without affecting live data. Wrike offers a comprehensive environment for developers to explore its features and capabilities.
Creating a Wrike Developer Account
To begin, you'll need to create a Wrike developer account. Visit the Wrike Developer Portal and sign up for an account. This will grant you access to the necessary tools and documentation to start building your integration.
Setting Up OAuth 2.0 Authentication
Wrike uses OAuth 2.0 for secure API authentication. Follow these steps to set up OAuth 2.0:
- Log in to your Wrike developer account and navigate to the "Apps" section.
- Click on "Create New App" and fill in the required details, such as the app name and description.
- Specify the redirect URI, which is the URL to which users will be redirected after authentication.
- Once the app is created, note down the client ID and client secret. These credentials are crucial for authenticating API requests.
Generating Access Tokens
With your app set up, you can now generate access tokens to authenticate API requests:
- Direct users to the Wrike authorization URL, including your client ID and redirect URI.
- Upon user consent, Wrike will redirect to your specified URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Wrike's token endpoint, including your client ID, client secret, and authorization code.
For detailed steps, refer to the Wrike OAuth 2.0 Authorization Guide.
Testing API Calls in the Sandbox Environment
With your access token, you can start making API calls to the Wrike sandbox environment. This allows you to test and validate your integration without impacting production data. Ensure that your API requests include the access token in the authorization header.
By setting up a test account and configuring OAuth 2.0, you're now ready to explore the powerful capabilities of the Wrike API for task management.
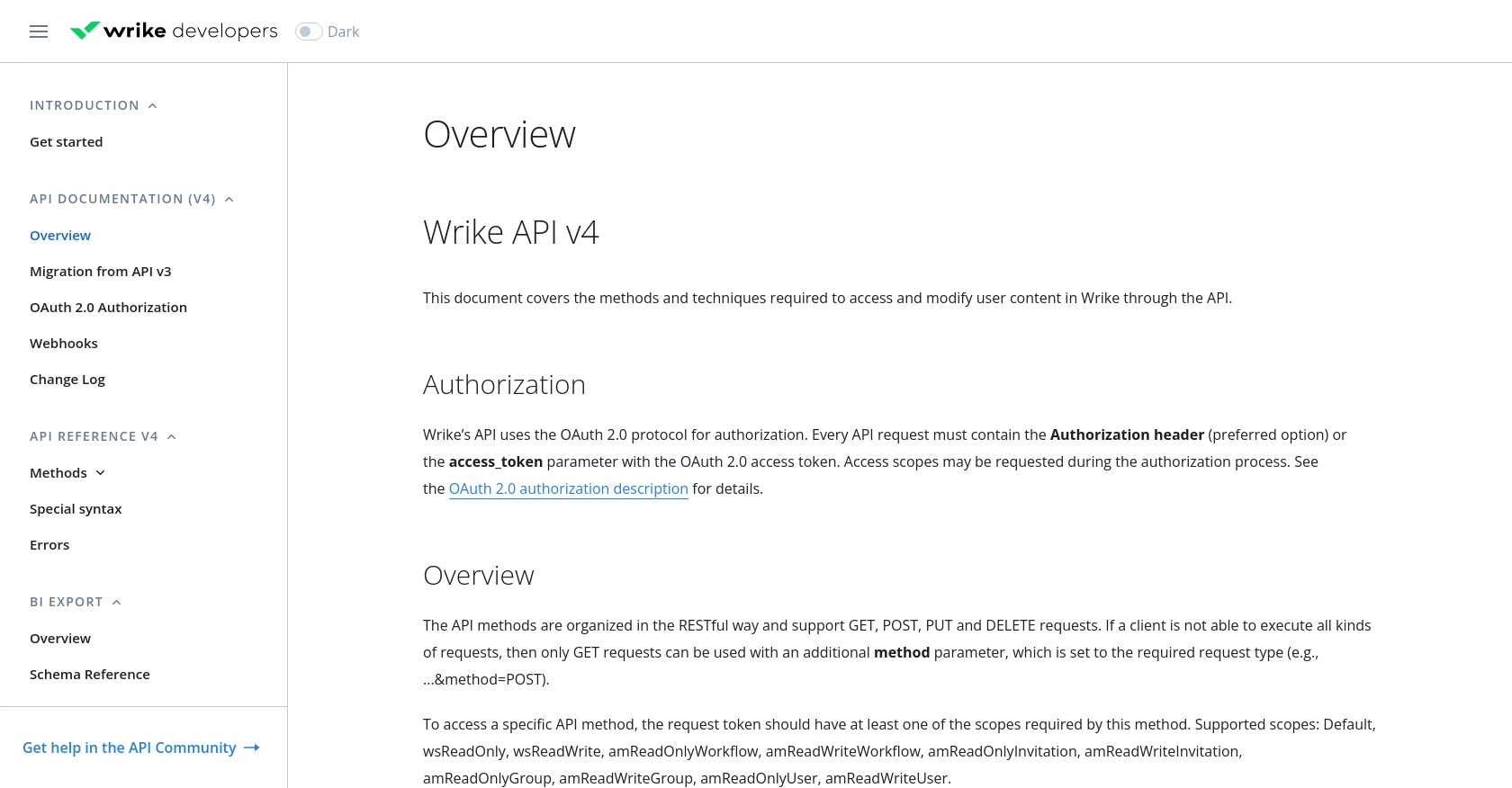
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Wrike Using PHP
To interact with the Wrike API and retrieve tasks, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to fetch tasks, and handling potential errors.
Setting Up Your PHP Environment for Wrike API Integration
Before you begin, ensure that you have PHP installed on your machine. You can verify this by running php -v
in your terminal. Additionally, you'll need the cURL
extension enabled to make HTTP requests.
To install any missing dependencies, you can use the following command:
sudo apt-get install php-curl
Writing PHP Code to Fetch Tasks from Wrike
Once your environment is set up, you can proceed to write the PHP code to interact with the Wrike API. Create a new PHP file named get_wrike_tasks.php
and add the following code:
<?php
$accessToken = 'Your_Access_Token';
$endpoint = 'https://www.wrike.com/api/v4/tasks';
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $accessToken
]);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode JSON response
$tasks = json_decode($response, true);
foreach ($tasks['data'] as $task) {
echo 'Task Title: ' . $task['title'] . '<br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Access_Token
with the access token you obtained during the OAuth 2.0 setup.
Understanding the API Response and Handling Errors
After executing the PHP script, you should see a list of task titles from your Wrike account. If the request fails, the script will output the error message. It's crucial to handle errors gracefully to ensure a robust integration.
For more information on error codes and handling, refer to the Wrike API Errors Documentation.
Verifying API Call Success in the Wrike Sandbox
To confirm that your API call is successful, log in to your Wrike sandbox account and verify that the tasks retrieved match those in your account. This ensures that your integration is working as expected.
By following these steps, you can efficiently retrieve tasks from Wrike using PHP, allowing you to integrate task data into your applications seamlessly.
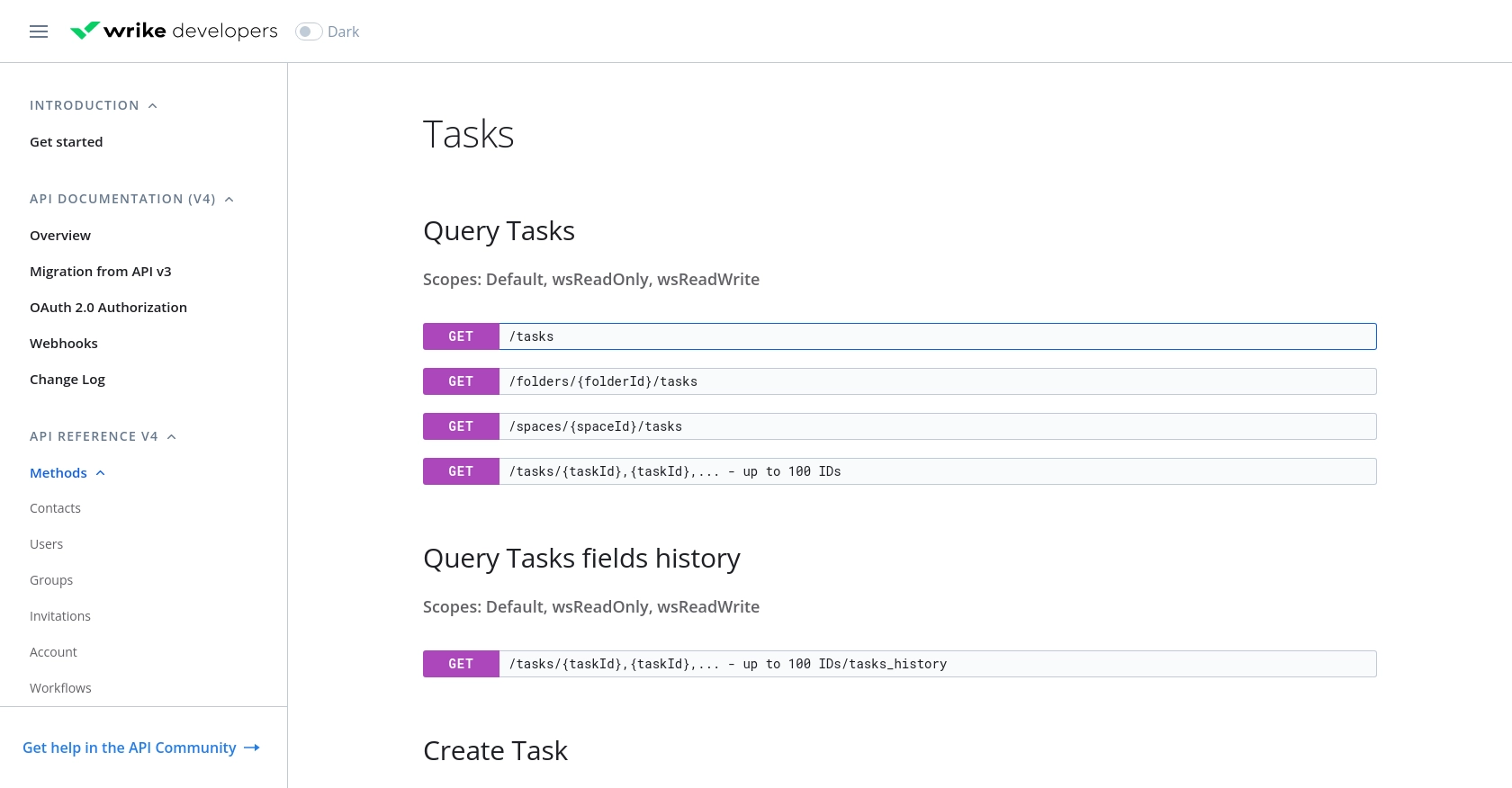
Conclusion and Best Practices for Wrike API Integration with PHP
Integrating with the Wrike API using PHP offers a powerful way to automate and enhance task management workflows. By following the steps outlined in this guide, you can efficiently retrieve tasks and incorporate them into your applications, providing real-time insights and updates for your team.
Best Practices for Secure and Efficient Wrike API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as client ID, client secret, and access tokens, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of Wrike's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage API request limits effectively. For more details, refer to the Wrike API Overview.
- Standardize Data Fields: When integrating task data, ensure that fields are standardized and mapped correctly to your application's data structure. This helps maintain consistency and accuracy.
- Error Handling: Implement robust error handling to gracefully manage API errors. Utilize Wrike's error codes to diagnose and resolve issues promptly. For more information, see the Wrike API Errors Documentation.
Streamlining Integrations with Endgrate
While integrating with Wrike's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can simplify your integration process.
Endgrate offers a unified API endpoint that connects to multiple platforms, including Wrike, allowing you to build once for each use case rather than multiple times for different integrations. By outsourcing integrations to Endgrate, you can save time and resources, enabling your team to focus on core product development and deliver an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?