Using the Xero API to Create or Update vendors in Javascript
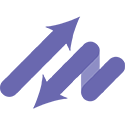
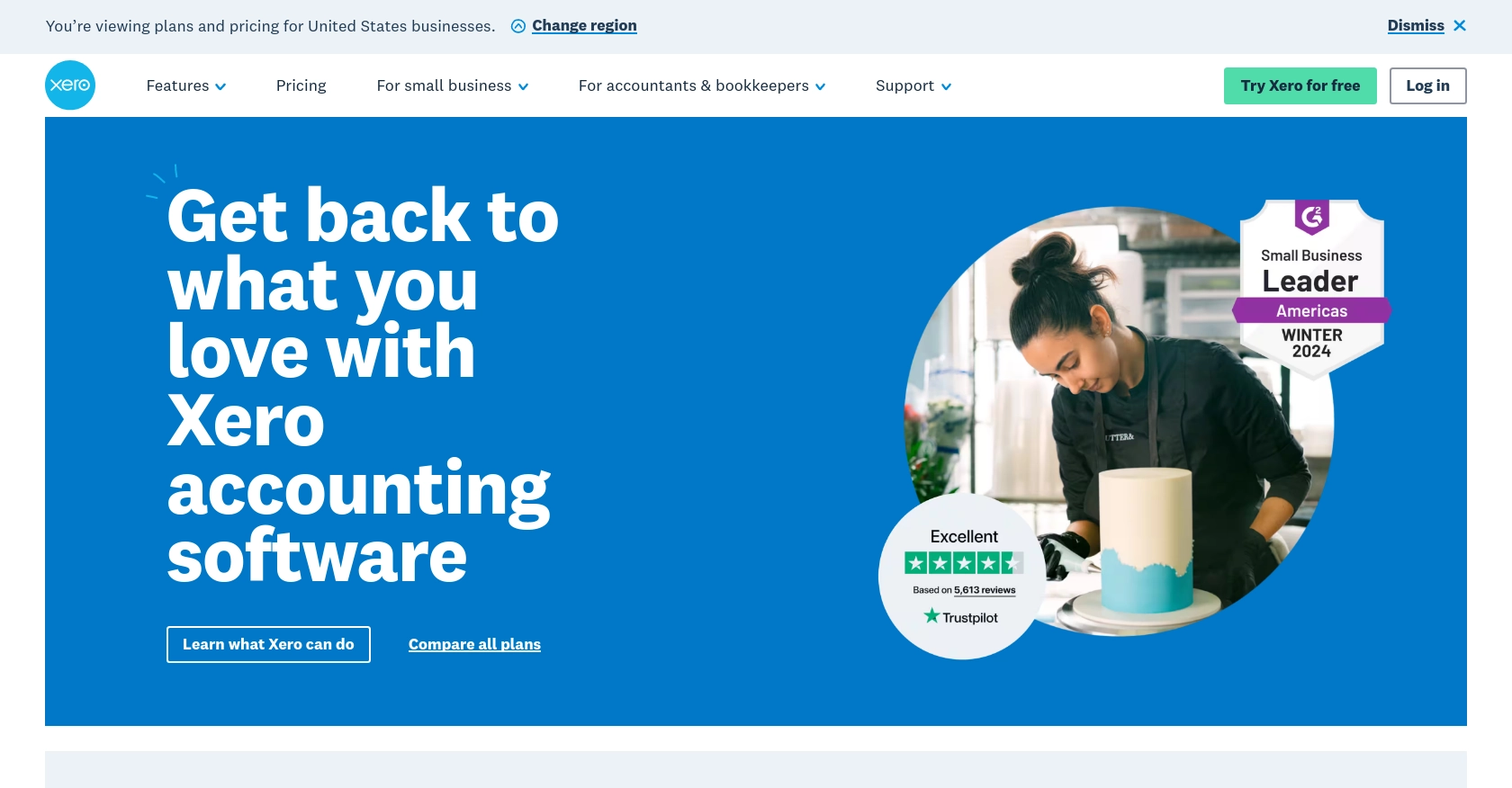
Introduction to Xero API for Vendor Management
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances with ease. It offers a wide range of features, including invoicing, payroll, and expense tracking, making it a popular choice for businesses looking to streamline their financial operations.
For developers, integrating with Xero's API can unlock the potential to automate and enhance financial processes. By connecting with the Xero API, developers can create or update vendor information programmatically, ensuring that financial data remains accurate and up-to-date. For example, a developer might use the Xero API to automatically update vendor details when changes occur in an external CRM system, reducing manual data entry and minimizing errors.
Setting Up Your Xero Sandbox Account for API Integration
Before you begin integrating with the Xero API, it's essential to set up a sandbox account. This allows you to test API interactions without affecting live data. Xero provides a free demo company that you can use for this purpose.
Creating a Xero Developer Account
To start, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for a developer account.
- Once registered, log in to your account.
Accessing the Xero Demo Company
Xero offers a demo company that you can use to test API calls:
- Log in to your Xero account and navigate to the "My Xero" tab.
- Select "Try the Demo Company" to access a sandbox environment.
Setting Up OAuth 2.0 Authentication
The Xero API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth 2.0:
- In the Xero Developer Portal, create a new app by navigating to "My Apps" and clicking "New App."
- Fill in the required details, including the app name and company URL.
- Set the redirect URI to a valid endpoint where you can receive authorization codes.
- Once the app is created, note down the client ID and client secret, which you'll use for authentication.
For more information on OAuth 2.0, refer to the Xero OAuth 2.0 documentation.
Configuring Scopes and Permissions
To interact with vendor data, you'll need to configure the appropriate scopes:
- In your app settings, navigate to the "Scopes" section.
- Select the necessary scopes for managing contacts, such as
accounting.contacts
.
For a detailed guide on scopes, visit the Xero Scopes documentation.
Generating an Access Token
With your app set up, you can now generate an access token:
- Use the standard OAuth 2.0 authorization code flow to obtain an authorization code.
- Exchange the authorization code for an access token using your client ID and client secret.
Refer to the Xero Authorization Flow documentation for detailed steps.
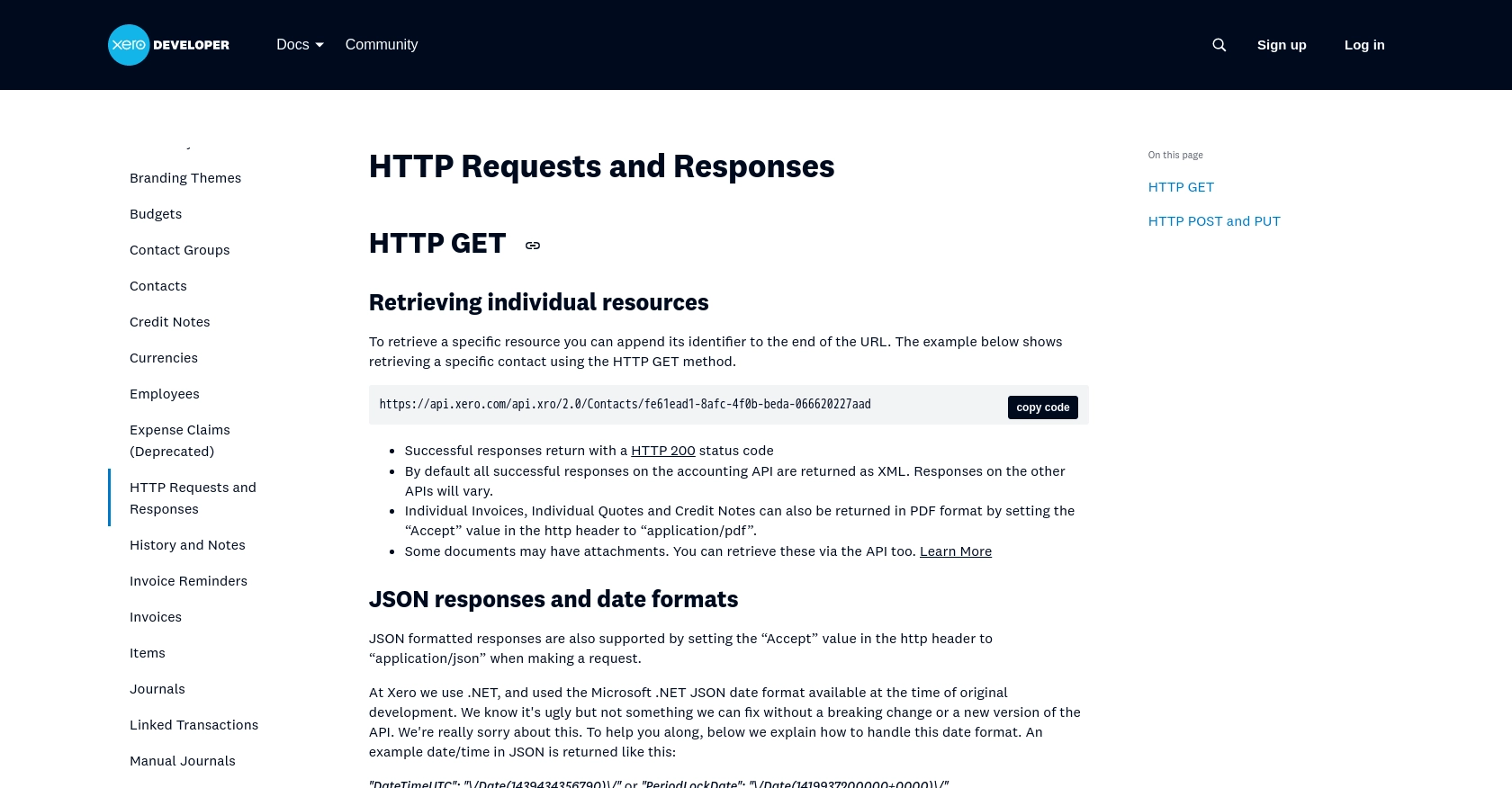
sbb-itb-96038d7
Making API Calls to Xero for Vendor Management Using JavaScript
Now that your Xero sandbox account is set up and authenticated, you can proceed to make API calls to create or update vendor information. This section will guide you through the process using JavaScript, ensuring you have the right tools and code to interact with the Xero API effectively.
Setting Up Your JavaScript Environment
Before making API calls, ensure your development environment is ready. You'll need:
- Node.js installed on your machine. You can download it from the official Node.js website.
- The
axios
library for making HTTP requests. Install it using npm:
npm install axios
Creating or Updating Vendors with the Xero API
To interact with the Xero API, you'll use the axios
library to send HTTP requests. Below is an example of how to create or update a vendor in Xero using JavaScript:
const axios = require('axios');
// Set the API endpoint
const endpoint = 'https://api.xero.com/api.xro/2.0/Contacts';
// Set the access token obtained from the OAuth flow
const accessToken = 'Your_Access_Token';
// Define the vendor data
const vendorData = {
"Contacts": [
{
"Name": "New Vendor",
"EmailAddress": "vendor@example.com",
"ContactNumber": "123456789",
"AccountNumber": "VEND001"
}
]
};
// Configure the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Make the POST request to create or update the vendor
axios.post(endpoint, vendorData, { headers })
.then(response => {
console.log('Vendor created/updated successfully:', response.data);
})
.catch(error => {
console.error('Error creating/updating vendor:', error.response.data);
});
Replace Your_Access_Token
with the access token you generated earlier. This script sends a POST request to the Xero API to create or update a vendor. The response will confirm the success of the operation or provide error details if something goes wrong.
Verifying API Call Success in Xero Sandbox
After running the script, you can verify the success of your API call by checking the Xero demo company:
- Log in to your Xero account and navigate to the "Contacts" section.
- Look for the vendor you created or updated to ensure the changes are reflected.
Handling Errors and Understanding Xero API Response Codes
When interacting with the Xero API, it's crucial to handle potential errors gracefully. Common response codes include:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid, often due to incorrect data.
- 401 Unauthorized: Authentication failed, possibly due to an expired token.
- 429 Too Many Requests: Rate limit exceeded. Refer to the Xero API limits documentation for more details.
Implement error handling in your code to manage these scenarios and ensure a robust integration.
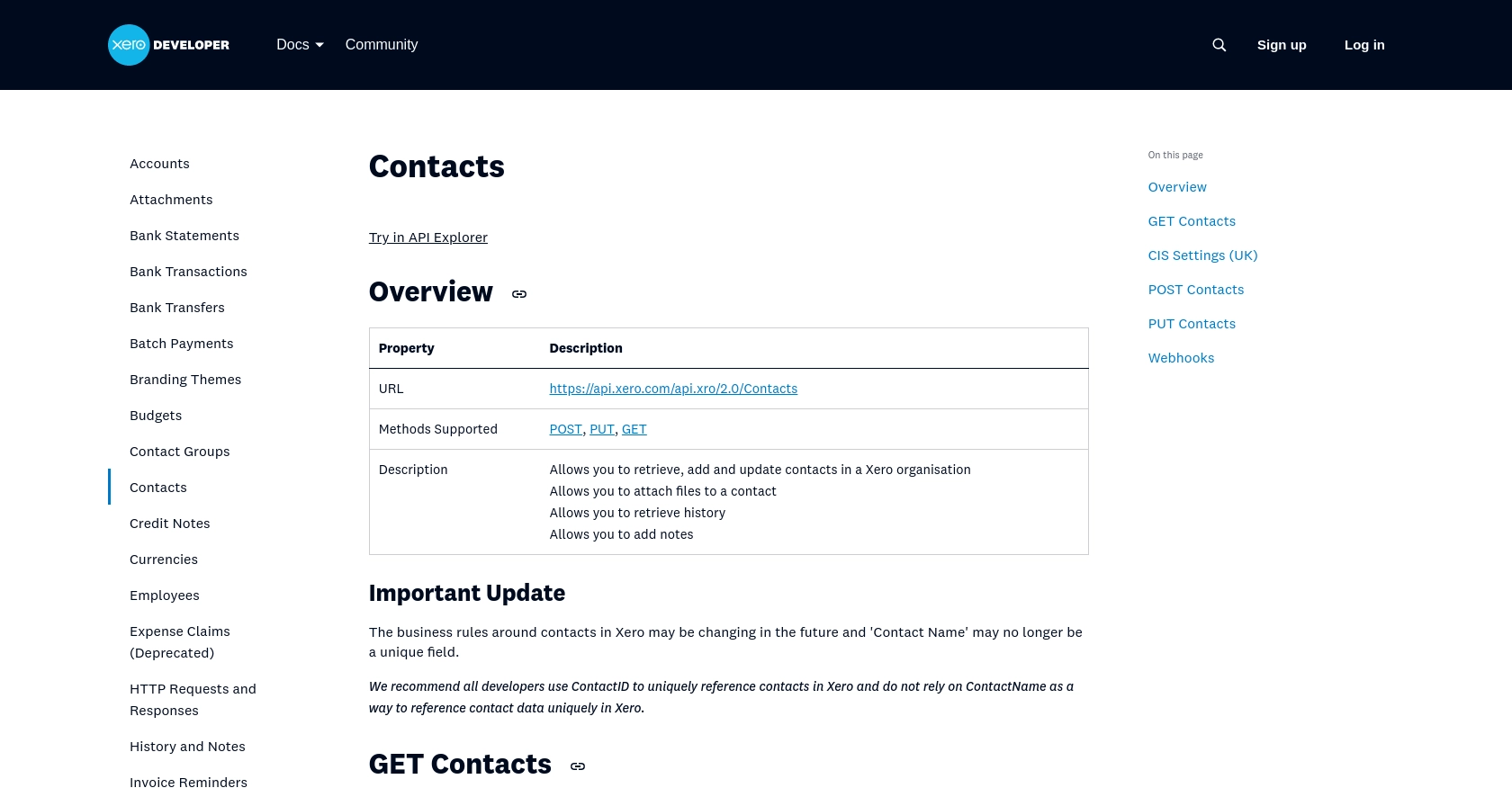
Conclusion: Best Practices for Xero API Integration and Vendor Management
Integrating with the Xero API to manage vendor information can significantly enhance your business's financial operations by automating data entry and ensuring accuracy. To maximize the benefits of this integration, consider the following best practices:
Securely Storing Xero API Credentials
Ensure that your client ID, client secret, and access tokens are stored securely. Use environment variables or secure vaults to prevent unauthorized access and protect sensitive information.
Handling Xero API Rate Limits
Be mindful of Xero's API rate limits to avoid disruptions. Implement logic to handle 429 Too Many Requests
errors by retrying requests after a delay. For more details, refer to the Xero API limits documentation.
Standardizing Vendor Data Formats
Ensure that vendor data is consistently formatted before sending it to the Xero API. This reduces the risk of errors and ensures that data is processed correctly.
Utilizing Endgrate for Seamless Integrations
Consider using Endgrate to simplify your integration processes. Endgrate provides a unified API endpoint that connects to multiple platforms, including Xero, allowing you to focus on your core product while outsourcing complex integrations. Learn more about how Endgrate can streamline your integration efforts by visiting Endgrate.
By following these best practices, you can ensure a robust and efficient integration with the Xero API, enhancing your vendor management processes and overall business operations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/contacts
Ready to get started?