Using the Xero API to Get Purchase Orders (with Javascript examples)
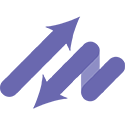
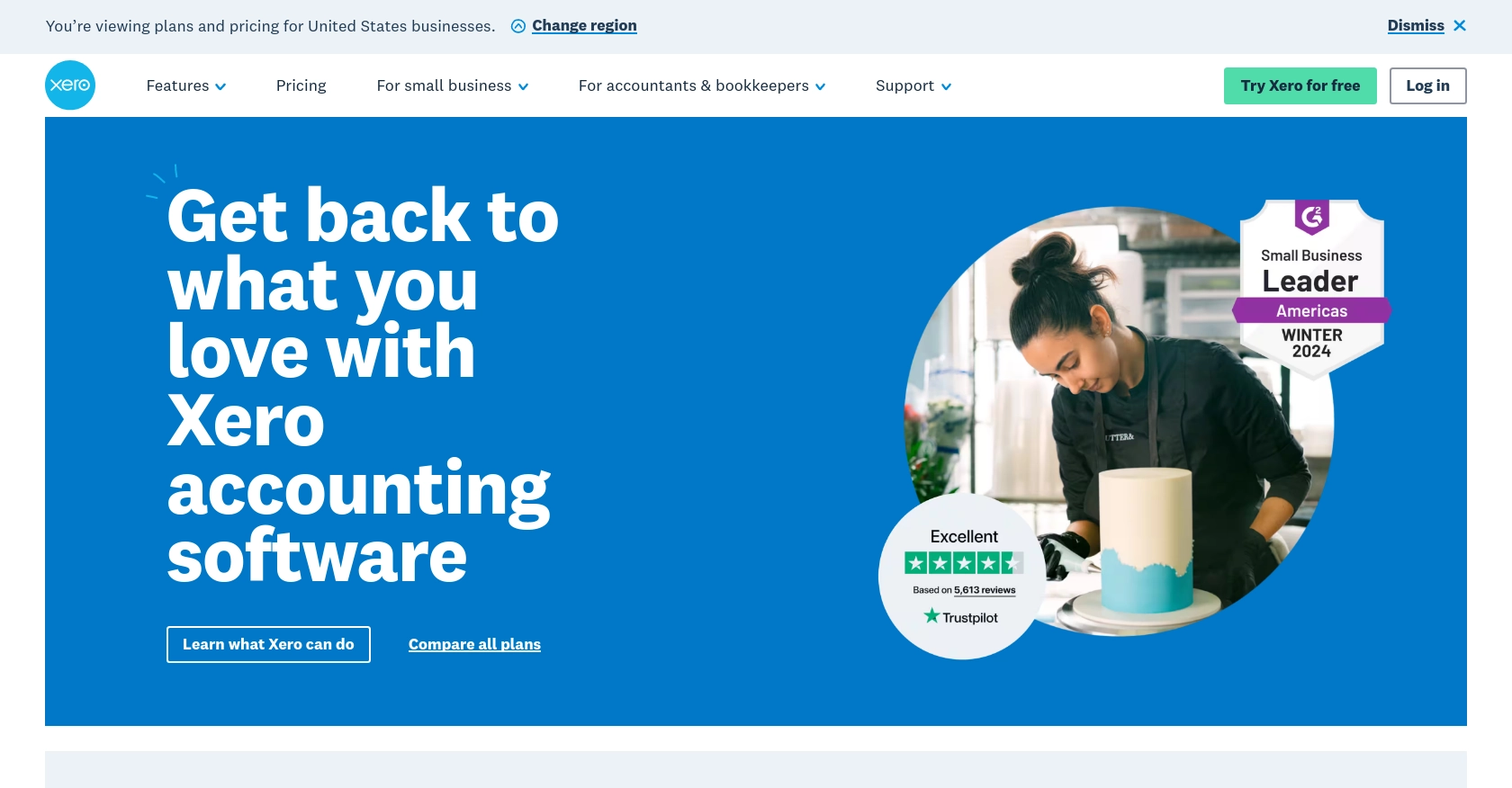
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to simplify financial management for small to medium-sized businesses. It offers a wide range of features, including invoicing, payroll, and purchase order management, making it a popular choice for businesses seeking efficient financial solutions.
Developers may want to integrate with Xero's API to automate and streamline accounting processes. For example, accessing purchase orders through the Xero API can help businesses keep track of their procurement activities, ensuring that all financial data is up-to-date and accurate.
This article will guide you through using JavaScript to interact with the Xero API, specifically focusing on retrieving purchase orders. By the end of this tutorial, you'll be equipped with the knowledge to efficiently manage purchase orders within the Xero platform using JavaScript.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start retrieving purchase orders using the Xero API, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Xero provides a demo company that you can use for this purpose.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal and sign up for a developer account.
- Once registered, log in to your developer dashboard.
Accessing the Xero Demo Company
After setting up your developer account, you can access the Xero demo company:
- Navigate to the "My Apps" section in your developer dashboard.
- Select "Try the Demo Company" to access a sandbox environment with sample data.
Creating a Xero App for OAuth 2.0 Authentication
To interact with the Xero API, you'll need to create an app to obtain the necessary credentials for OAuth 2.0 authentication:
- In the "My Apps" section, click "New App" to create a new application.
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid endpoint where you can handle OAuth callbacks.
- Save your app to generate the client ID and client secret.
Configuring OAuth 2.0 Scopes
Ensure your app has the correct scopes to access purchase orders:
- In your app settings, navigate to the "Scopes" section.
- Select the scopes related to purchase orders, such as
accounting.transactions
.
For more information on OAuth 2.0 authentication, refer to the Xero OAuth 2.0 Overview.
Generating Access Tokens
With your app set up, you can now generate access tokens:
- Use the standard OAuth 2.0 authorization code flow to obtain an access token.
- Refer to the Xero OAuth 2.0 Auth Flow for detailed steps.
Once you have your access token, you'll be ready to make API calls to retrieve purchase orders from Xero.
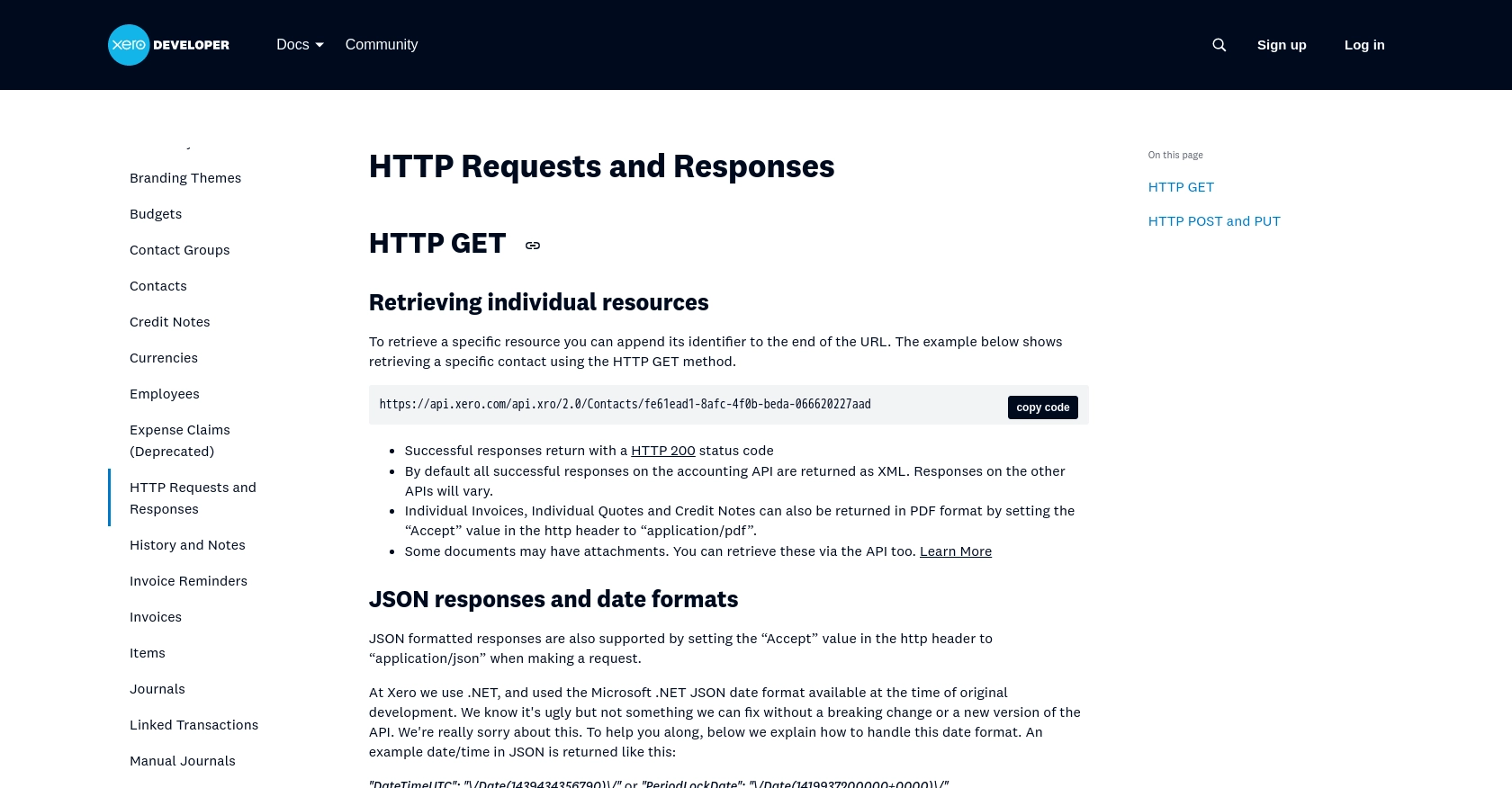
sbb-itb-96038d7
Making API Calls to Retrieve Xero Purchase Orders Using JavaScript
Once you have your access token, you can start making API calls to Xero to retrieve purchase orders. This section will guide you through the process of setting up your JavaScript environment and executing the necessary API requests.
Setting Up Your JavaScript Environment for Xero API Integration
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (preferably version 14.x or later)
- NPM (Node Package Manager)
With Node.js and NPM installed, you can set up your project:
mkdir xero-api-integration
cd xero-api-integration
npm init -y
npm install axios
The axios
library will be used to handle HTTP requests to the Xero API.
Writing JavaScript Code to Fetch Purchase Orders from Xero
Create a file named getPurchaseOrders.js
and add the following code:
const axios = require('axios');
// Replace with your access token
const accessToken = 'Your_Access_Token';
// Xero API endpoint for purchase orders
const endpoint = 'https://api.xero.com/api.xro/2.0/PurchaseOrders';
// Set up headers for the request
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Accept': 'application/json'
};
// Function to get purchase orders
async function getPurchaseOrders() {
try {
const response = await axios.get(endpoint, { headers });
const purchaseOrders = response.data.PurchaseOrders;
console.log('Purchase Orders:', purchaseOrders);
} catch (error) {
console.error('Error fetching purchase orders:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getPurchaseOrders();
In this code, you use axios
to send a GET request to the Xero API endpoint for purchase orders. The access token is included in the request headers for authentication.
Running the JavaScript Code and Handling Responses
To execute the script and retrieve purchase orders, run the following command in your terminal:
node getPurchaseOrders.js
If successful, the console will display the purchase orders retrieved from your Xero demo company. If there are any errors, they will be logged to the console.
Verifying API Call Success and Handling Errors
To ensure the API call was successful, check the response status code. A status code of 200 indicates success. If you encounter errors, refer to the error message for troubleshooting.
Common error codes include:
- 401 Unauthorized: Check if your access token is valid and has not expired.
- 403 Forbidden: Ensure your app has the correct scopes for accessing purchase orders.
- 429 Too Many Requests: Xero's API rate limit is 60 requests per minute. Implement rate limiting strategies if needed.
For more information on error codes, refer to the Xero API Requests and Responses documentation.
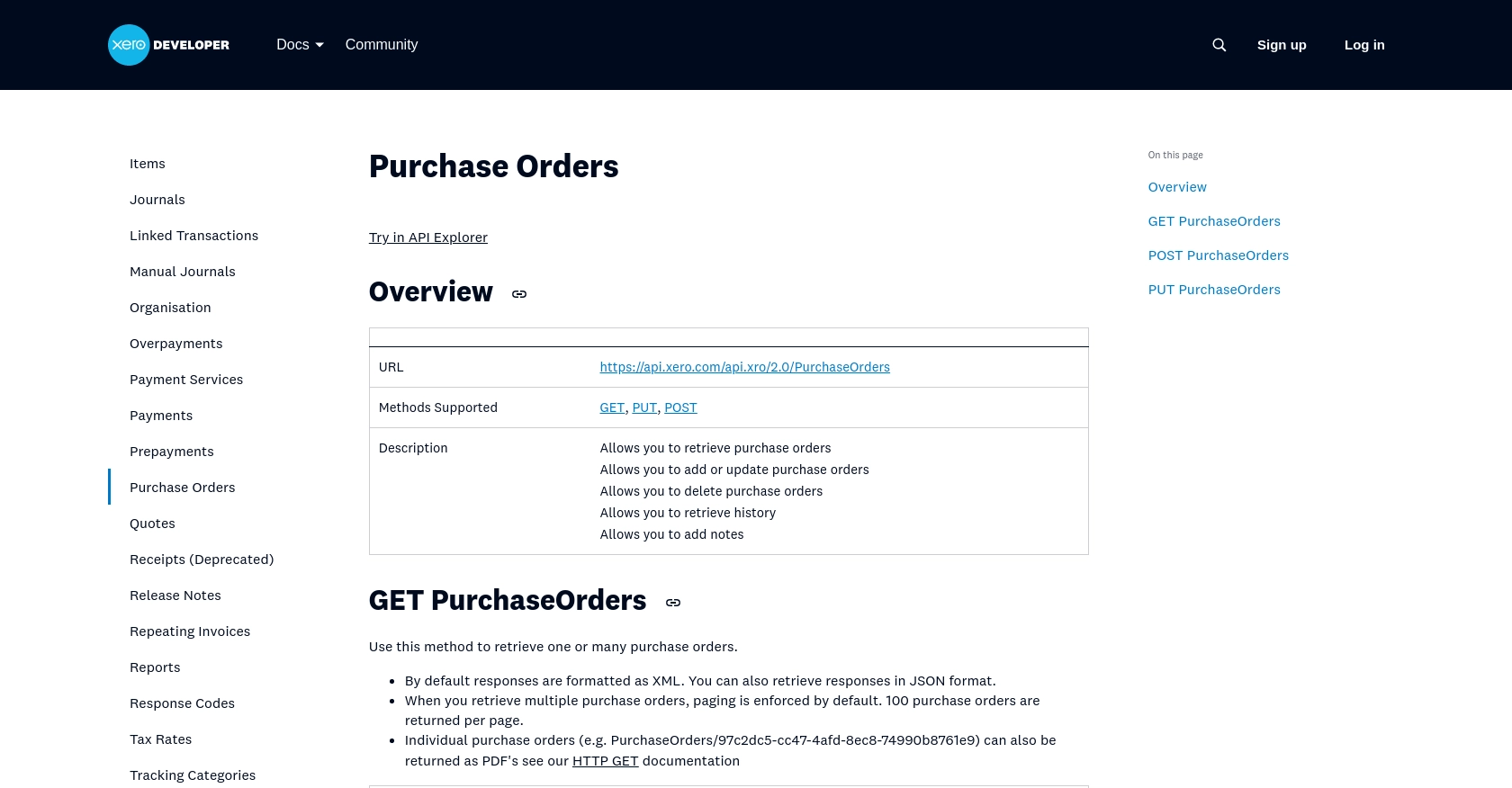
Conclusion: Best Practices for Xero API Integration and Efficient Purchase Order Management
Integrating with the Xero API to manage purchase orders can significantly enhance your business's financial operations by automating and streamlining procurement processes. By following the steps outlined in this guide, you can efficiently retrieve purchase orders using JavaScript, ensuring your financial data remains accurate and up-to-date.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of Xero's API rate limit of 60 requests per minute. Implement strategies such as exponential backoff or request queuing to manage rate limits effectively.
- Data Transformation and Standardization: Ensure that data retrieved from Xero is transformed and standardized to fit your application's requirements, maintaining consistency across systems.
Streamlining Integrations with Endgrate
While integrating with Xero is a powerful way to manage purchase orders, handling multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Xero.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover how you can streamline your business operations today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/purchaseorders
Ready to get started?