Using the Xero API to Get Purchase Orders in Python
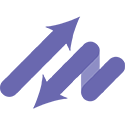
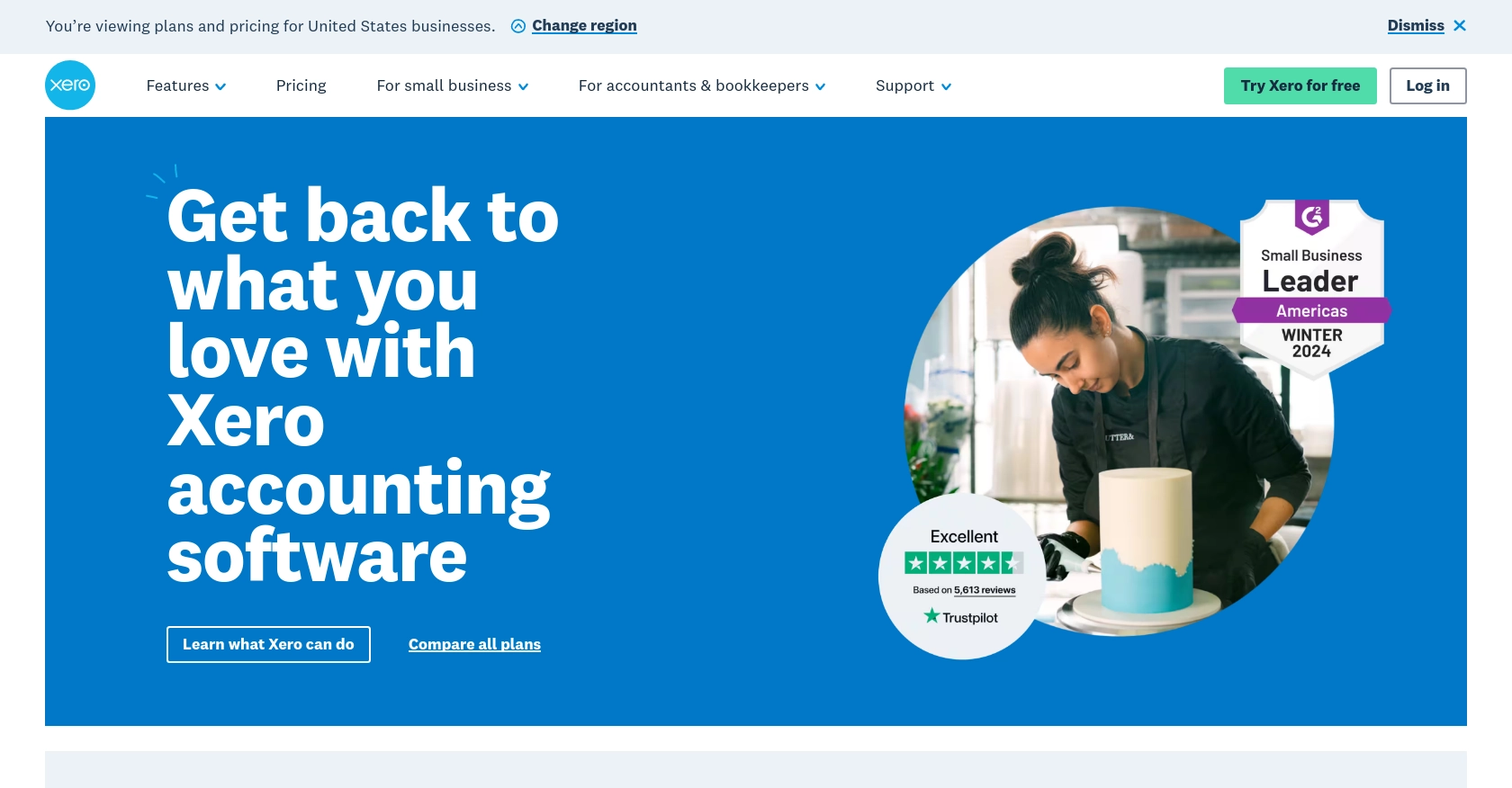
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to meet the needs of small to medium-sized businesses. It offers a comprehensive suite of tools for managing finances, including invoicing, payroll, and purchase orders. Xero's user-friendly interface and robust features make it a popular choice for businesses looking to streamline their accounting processes.
Integrating with Xero's API allows developers to automate and enhance financial workflows. For example, by using the Xero API, developers can retrieve purchase orders to analyze spending patterns or integrate with other systems for seamless financial management. This capability is particularly useful for businesses that need to synchronize their accounting data with other platforms or applications.
Setting Up Your Xero Sandbox Account for API Integration
Before diving into the Xero API, you need to set up a sandbox account. This will allow you to test your integration without affecting live data. Xero provides a demo company that you can use for this purpose, which is ideal for developers looking to experiment with API calls.
Creating a Xero Developer Account
To get started, you'll need a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal and sign up for a developer account.
- Once registered, log in to your developer account to access the dashboard.
Accessing the Xero Demo Company
With your developer account ready, you can now access the Xero demo company:
- Navigate to the "My Apps" section in your Xero developer dashboard.
- Select "Try the Demo Company" to connect to a pre-configured sandbox environment.
Setting Up OAuth 2.0 Authentication for Xero API
Xero uses OAuth 2.0 for authentication, ensuring secure access to its APIs. Follow these steps to set up OAuth 2.0:
- In your developer dashboard, go to "My Apps" and click "Add a New App."
- Fill in the required details, such as the app name and company URL.
- Under "OAuth 2.0 Redirect URI," enter the URL where users will be redirected after authentication.
- Save your app to generate a client ID and client secret.
Generating Access Tokens for Xero API
With your app created, you can now generate access tokens:
- Use the client ID and client secret to request an access token via the OAuth 2.0 authorization code flow.
- Refer to the Xero OAuth 2.0 Guide for detailed steps on obtaining tokens.
Once you have your access token, you can start making API calls to the Xero demo company to retrieve purchase orders and other financial data.
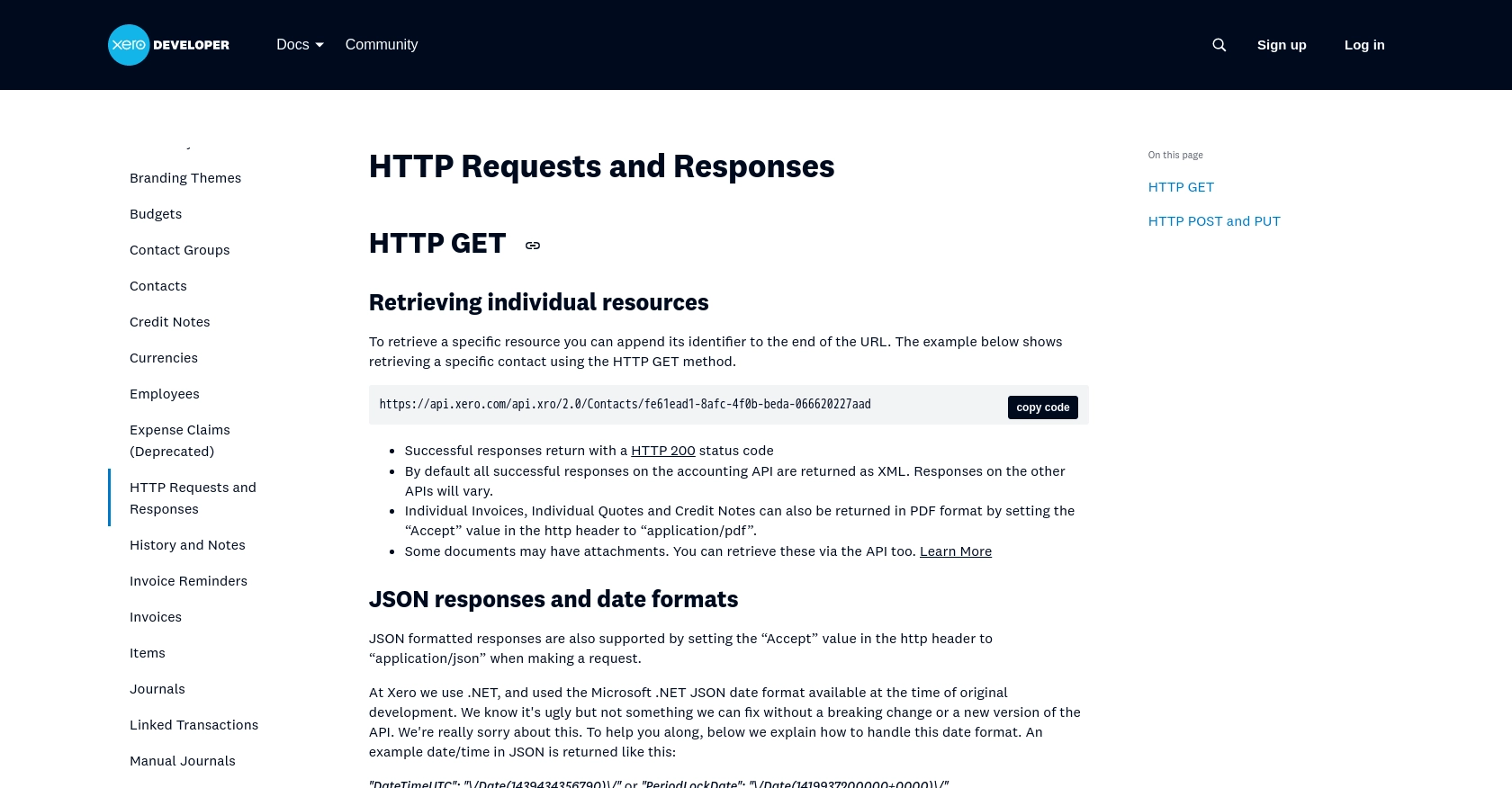
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from Xero Using Python
To interact with the Xero API and retrieve purchase orders, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your Python Environment for Xero API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Fetch Purchase Orders from Xero
With your environment set up, you can now write the Python code to make API calls to Xero and retrieve purchase orders. Create a file named get_xero_purchase_orders.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.xero.com/api.xro/2.0/PurchaseOrders"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the purchase orders and print their information
for order in data.get("PurchaseOrders", []):
print(order)
else:
print(f"Failed to retrieve purchase orders: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token you obtained during the OAuth 2.0 setup.
Running the Python Script and Verifying Xero API Responses
To execute the script and retrieve purchase orders, run the following command in your terminal:
python get_xero_purchase_orders.py
If successful, the script will output the purchase orders from your Xero demo company. Verify the data by checking the purchase orders in the Xero sandbox environment.
Handling Errors and Understanding Xero API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Xero API may return various HTTP status codes indicating the success or failure of your request. Here are some common response codes:
- 200 OK: The request was successful, and the purchase orders are returned.
- 401 Unauthorized: Authentication failed. Check your access token.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more details on handling errors, refer to the Xero API documentation.
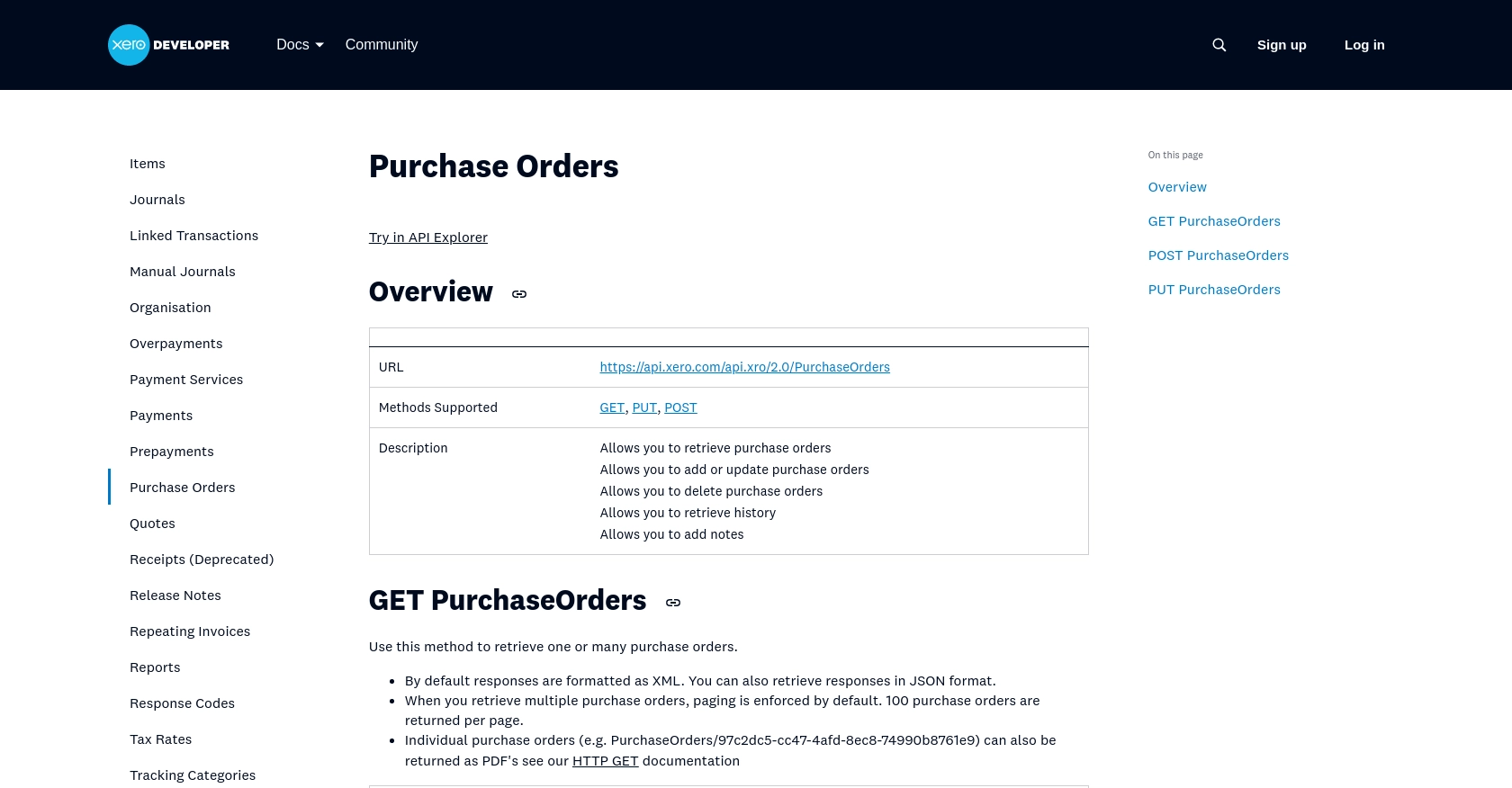
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API to retrieve purchase orders using Python can significantly enhance your financial management processes. By automating data retrieval, you can streamline operations, reduce manual errors, and ensure that your accounting data is always up-to-date.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limits: Xero imposes rate limits on API requests. Be sure to implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the Xero API rate limits documentation.
- Data Standardization: Ensure that the data retrieved from Xero is standardized and transformed as needed to integrate seamlessly with other systems.
- Error Handling: Implement robust error handling to manage different HTTP response codes effectively, ensuring that your application can recover gracefully from API errors.
Streamlining Integrations with Endgrate
While integrating with Xero's API can provide powerful capabilities, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Xero.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing an intuitive experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/purchaseorders
Ready to get started?