Using the Younium API to Create or Update Payments (with Python examples)
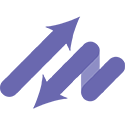
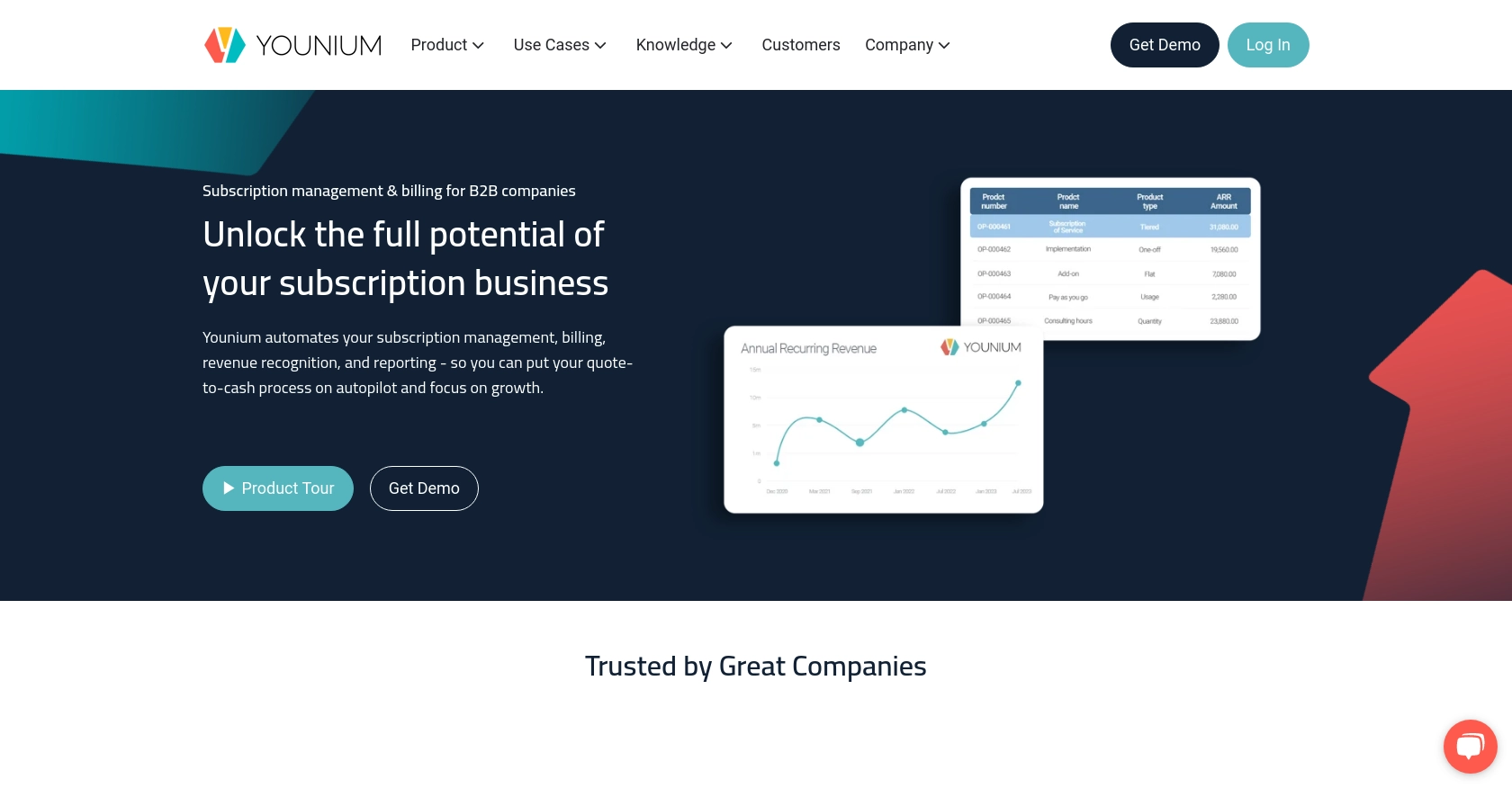
Introduction to Younium API for Payment Management
Younium is a comprehensive subscription management platform designed to streamline billing and financial operations for B2B SaaS companies. It offers a robust API that allows developers to automate and manage various financial processes, including invoicing, payments, and subscription management.
Integrating with the Younium API can significantly enhance a developer's ability to manage payments efficiently. For example, you can automate the creation or updating of payment records directly from your application, ensuring that financial data is always up-to-date and accurate.
This article will guide you through using Python to interact with the Younium API for creating or updating payments, providing a step-by-step approach to streamline your financial operations.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start interacting with the Younium API, it's essential to set up a sandbox account. This environment allows you to test API calls without affecting your production data, ensuring a safe and controlled development process.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps:
- Visit the Younium Developer Portal.
- Sign up for a sandbox account by following the on-screen instructions.
- Once your account is created, log in to access the sandbox environment.
Generating API Credentials for Younium
With your sandbox account ready, the next step is to generate the necessary API credentials. These credentials will allow you to authenticate your API requests securely.
- Navigate to your user profile by clicking your name in the top right corner and select “Privacy & Security”.
- In the left panel, click on “Personal Tokens” and then “Generate Token”.
- Provide a relevant description for your token and click “Create”.
- Copy the generated Client ID and Secret Key. These will be used to obtain a JWT access token.
Acquiring a JWT Access Token
To authenticate your API requests, you'll need a JWT access token. Here's how to acquire it:
import requests
# Define the endpoint and headers
url = "https://api.sandbox.younium.com/auth/token"
headers = {"Content-Type": "application/json"}
# Set the request body with your client credentials
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make the POST request to get the JWT token
response = requests.post(url, headers=headers, json=body)
# Parse the response to get the access token
token_data = response.json()
access_token = token_data.get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. The access token is valid for 24 hours, after which you'll need to request a new one.
For more details on authentication, refer to the Younium Authentication Documentation.
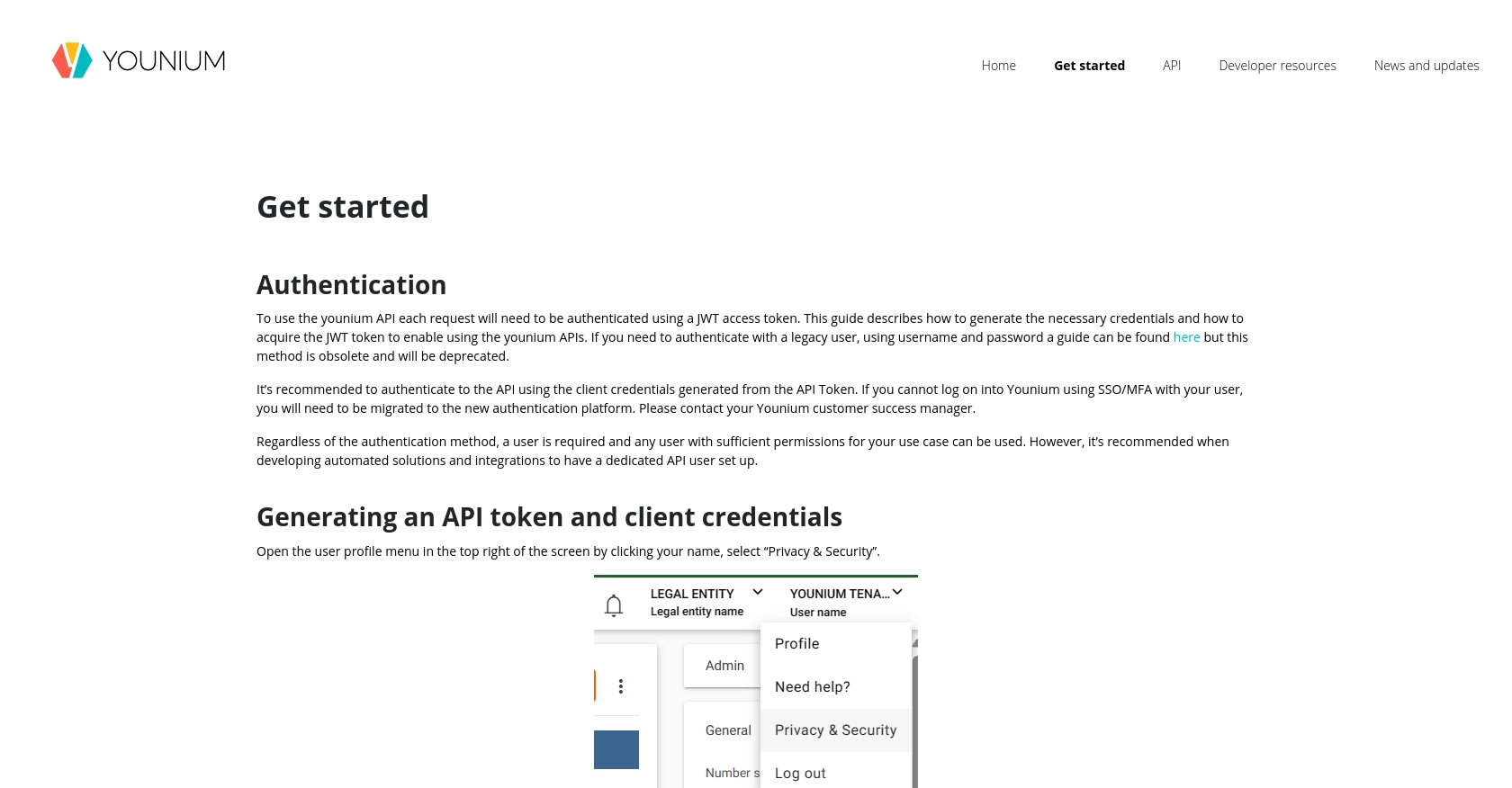
sbb-itb-96038d7
Making API Calls to Younium for Payment Management Using Python
To interact with the Younium API for creating or updating payments, you'll need to make authenticated API calls using Python. This section will guide you through the process, including setting up your Python environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Younium API Integration
Before making API calls, ensure you have Python 3.x installed on your machine along with the necessary dependencies. You'll need the requests
library to handle HTTP requests.
pip install requests
Creating or Updating Payments with the Younium API
Once your environment is set up, you can proceed to create or update payments using the Younium API. Below is a sample Python script to demonstrate how to perform these operations.
import requests
# Define the API endpoint and headers
url = "https://api.sandbox.younium.com/payments"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Define the payment data
payment_data = {
"amount": 100.00,
"currency": "USD",
"paymentDate": "2023-10-01",
"account": "A-000001",
"description": "Payment for Invoice #12345"
}
# Make the POST request to create or update a payment
response = requests.post(url, headers=headers, json=payment_data)
# Check if the request was successful
if response.status_code == 201:
print("Payment created successfully:", response.json())
else:
print("Failed to create payment:", response.status_code, response.json())
Replace Your_Access_Token
with the JWT token obtained earlier. The payment_data
dictionary should be customized with the relevant payment details.
Verifying API Call Success and Handling Errors
After executing the script, verify the success of the API call by checking the response status code. A status code of 201
indicates successful creation or update of the payment. If the request fails, the response will include an error message with a status code such as 400
(Bad Request) or 401
(Unauthorized).
For more detailed error handling, refer to the Younium API Documentation.
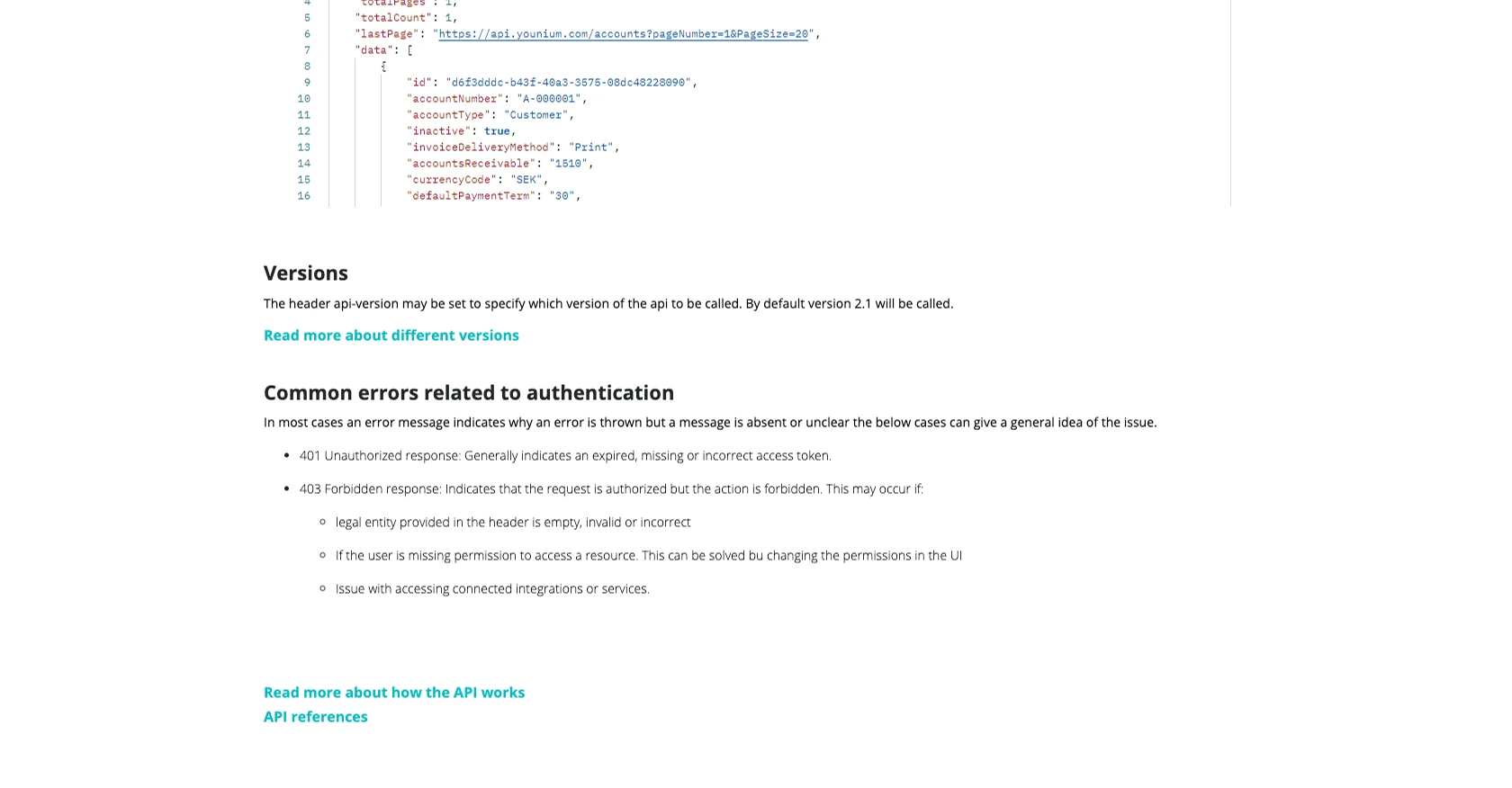
Conclusion and Best Practices for Using Younium API in Python
Integrating with the Younium API for payment management offers a powerful way to automate and streamline financial operations within your B2B SaaS applications. By following the steps outlined in this guide, you can efficiently create or update payments using Python, ensuring your financial data remains accurate and up-to-date.
Best Practices for Secure and Efficient Younium API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to manage sensitive information like Client IDs and Secret Keys.
- Handling Rate Limits: Be mindful of any rate limits imposed by the Younium API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across your applications. This includes using consistent currency codes and date formats.
- Error Handling: Implement robust error handling to manage different response codes effectively. Log errors for monitoring and debugging purposes.
Streamlining Integrations with Endgrate
While integrating with the Younium API can enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing an intuitive experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?