Using the Zendesk Sell API to Get Leads in PHP
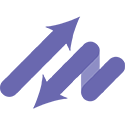
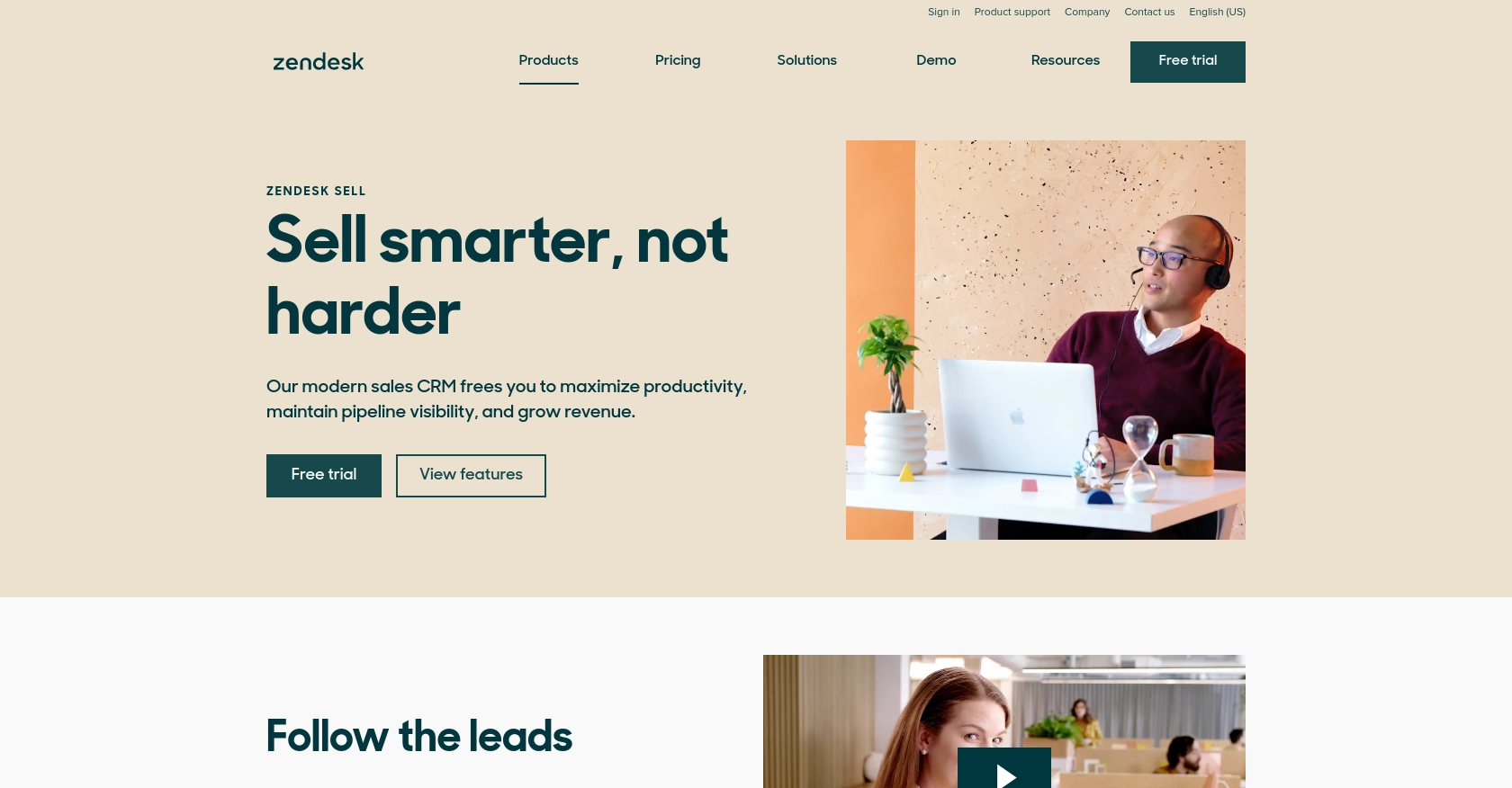
Introduction to Zendesk Sell API
Zendesk Sell is a powerful sales CRM platform designed to enhance productivity and streamline sales processes for businesses. It offers a comprehensive suite of tools that help sales teams manage leads, track communication, and close deals more efficiently.
Integrating with the Zendesk Sell API allows developers to automate and optimize sales workflows. For example, you can use the API to retrieve leads and integrate them into your custom sales dashboard, providing real-time insights and improving decision-making processes.
Setting Up Your Zendesk Sell Test Account
Before you can start integrating with the Zendesk Sell API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Zendesk Sell Sandbox Account
If you don't already have a Zendesk Sell account, you can sign up for a free trial or a sandbox account on the Zendesk Sell website. Follow these steps to get started:
- Visit the Zendesk Sell website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once your account is created, you'll receive a confirmation email. Follow the instructions in the email to activate your account.
Setting Up OAuth Authentication for Zendesk Sell API
The Zendesk Sell API uses OAuth 2.0 for authentication. Follow these steps to create an app and obtain the necessary credentials:
- Log in to your Zendesk Sell account and navigate to the "Settings" section.
- Under "Integrations," select "API & Apps" and then click on "Create App."
- Fill in the required information for your app, such as the app name and description.
- After creating the app, you'll receive a Client ID and Client Secret. Keep these credentials secure as you'll need them for API authentication.
Generating Access Tokens
To interact with the Zendesk Sell API, you'll need to generate an access token. Here's how:
- Use the Authorization Code Flow to obtain an authorization code. Direct users to the following URL, replacing
$CLIENT_ID
and$CLIENT_REDIRECT_URI
with your app's details: - Once the user authorizes the app, they'll be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- The response will include an access token that you can use to authenticate API requests.
https://api.getbase.com/oauth2/authorize?client_id=$CLIENT_ID&response_type=code&redirect_uri=$CLIENT_REDIRECT_URI
curl -X POST https://api.getbase.com/oauth2/token \
-u "$CLIENT_ID:$CLIENT_SECRET" \
-d "grant_type=authorization_code" \
-d "code=$AUTHORIZATION_CODE" \
-d "redirect_uri=$CLIENT_REDIRECT_URI"
With your Zendesk Sell test account and OAuth setup complete, you're ready to start making API calls to retrieve leads and integrate them into your applications.
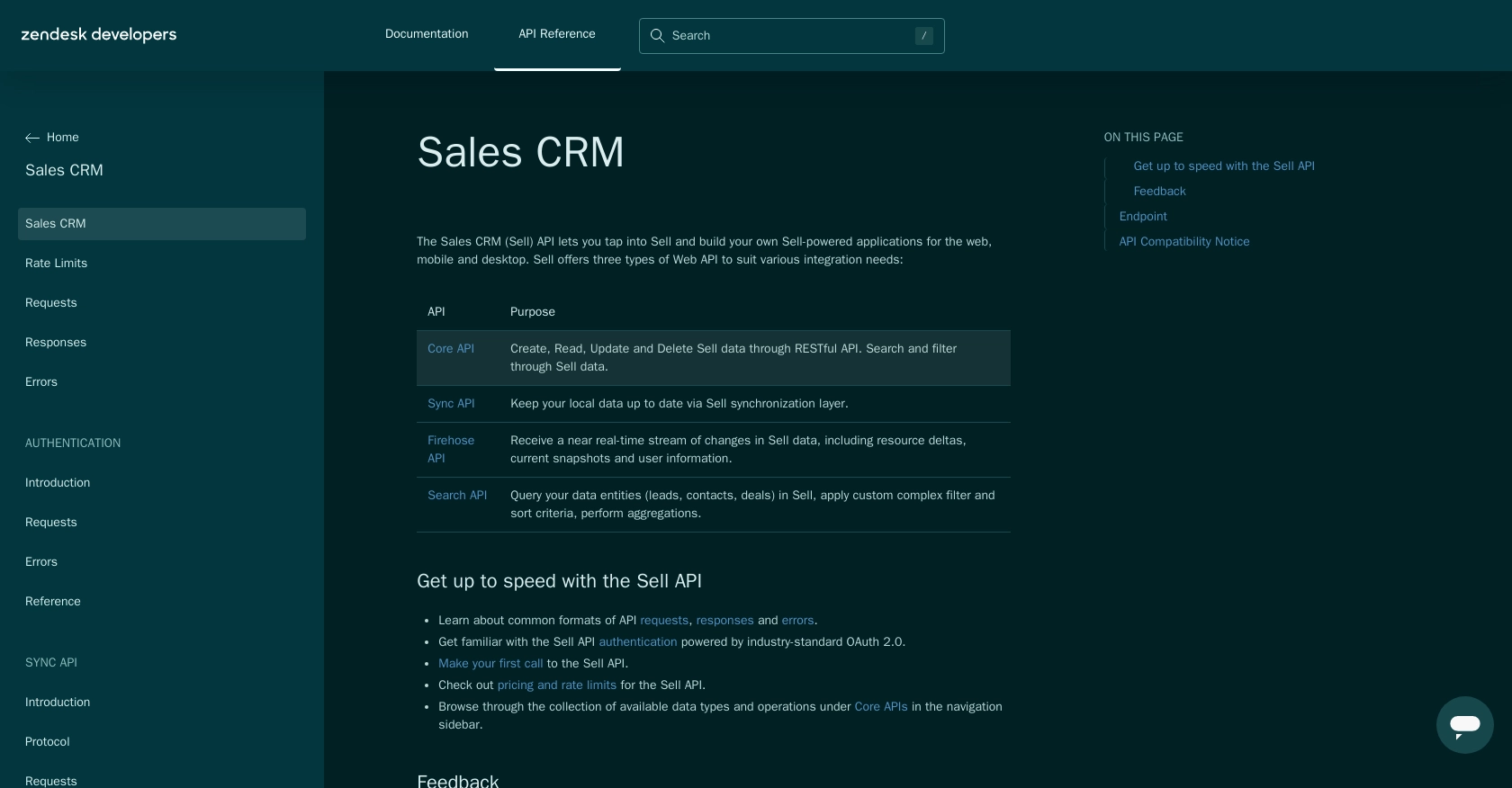
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Zendesk Sell Using PHP
To interact with the Zendesk Sell API and retrieve leads, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Zendesk Sell API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- cURL extension for PHP
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Retrieving Leads from Zendesk Sell API Using PHP
Once your environment is set up, you can proceed to make an API call to retrieve leads. Here's a step-by-step guide:
- Create a new PHP file named
get_zendesk_leads.php
and add the following code: - Replace
Your_Access_Token
with the access token you obtained during the OAuth setup. - Run the PHP script from the command line or a web server to see the list of leads.
<?php
$accessToken = 'Your_Access_Token';
$url = 'https://api.getbase.com/v2/leads';
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $accessToken,
'Accept: application/json'
]);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode JSON response
$data = json_decode($response, true);
// Display leads
foreach ($data['items'] as $lead) {
echo 'Lead ID: ' . $lead['data']['id'] . '<br>';
echo 'Name: ' . $lead['data']['first_name'] . ' ' . $lead['data']['last_name'] . '<br>';
echo 'Email: ' . $lead['data']['email'] . '<br><br>';
}
}
// Close cURL session
curl_close($ch);
?>
Handling Zendesk Sell API Response and Errors
After executing the API call, you should verify the response to ensure it succeeded. The Zendesk Sell API returns a JSON object containing the leads data. If the request fails, handle the errors appropriately:
- Check for HTTP status codes. A successful request returns a
200 OK
status. - Handle error codes such as
401 Unauthorized
for invalid tokens or429 Too Many Requests
if the rate limit is exceeded.
For more details on error handling, refer to the Zendesk Sell API error documentation.
Verifying API Call Success in Zendesk Sell
To confirm that your API call was successful, log in to your Zendesk Sell account and check the leads section. The leads retrieved by your script should match those displayed in the Zendesk Sell dashboard.
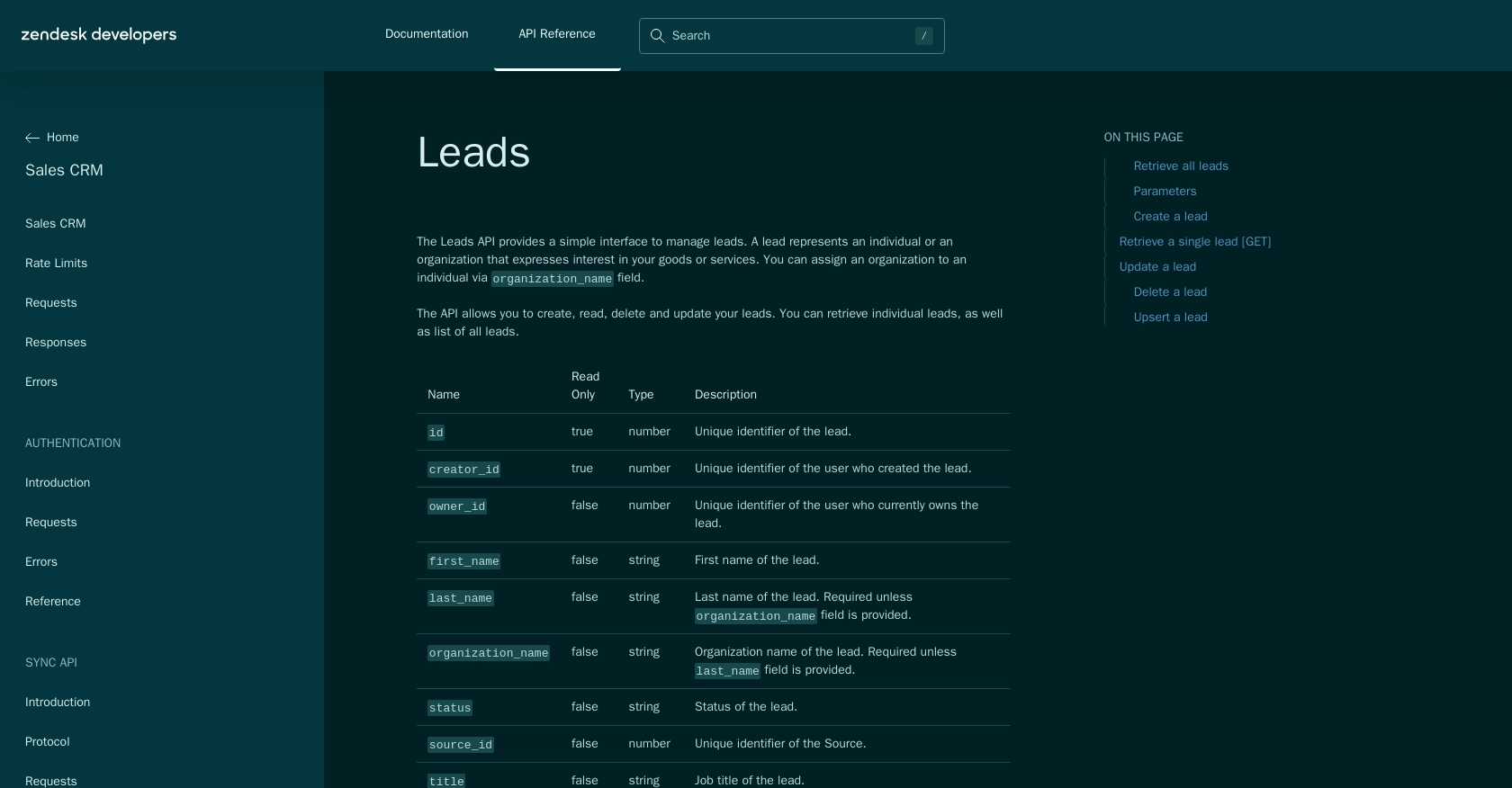
Conclusion and Best Practices for Using Zendesk Sell API with PHP
Integrating with the Zendesk Sell API using PHP allows developers to efficiently manage leads and streamline sales processes. By following the steps outlined in this guide, you can set up OAuth authentication, make API calls to retrieve leads, and handle responses effectively.
Best Practices for Secure and Efficient Zendesk Sell API Integration
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: The Zendesk Sell API allows up to 36,000 requests per hour (10 requests/token/second). Implement logic to handle the
429 Too Many Requests
error by retrying after a delay. For more details, refer to the Zendesk Sell API rate limits documentation. - Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application and Zendesk Sell.
- Error Handling: Implement robust error handling to manage different HTTP status codes and error messages. This will improve the reliability of your integration.
Streamlining Integration Development with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including Zendesk Sell.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can enhance your integration experience by visiting Endgrate's website.
With these best practices and tools, you can create a seamless and efficient integration with Zendesk Sell, enhancing your sales processes and driving business success.
Read More
- https://endgrate.com/provider/zendesksell
- https://developer.zendesk.com/api-reference/sales-crm/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/rate-limits/
- https://developer.zendesk.com/api-reference/sales-crm/requests/
- https://developer.zendesk.com/api-reference/sales-crm/errors/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/requests/#client-authentication
- https://developer.zendesk.com/api-reference/sales-crm/resources/leads/
Ready to get started?