Using the Zoho Books API to Create or Update Invoices (with PHP examples)
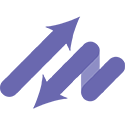
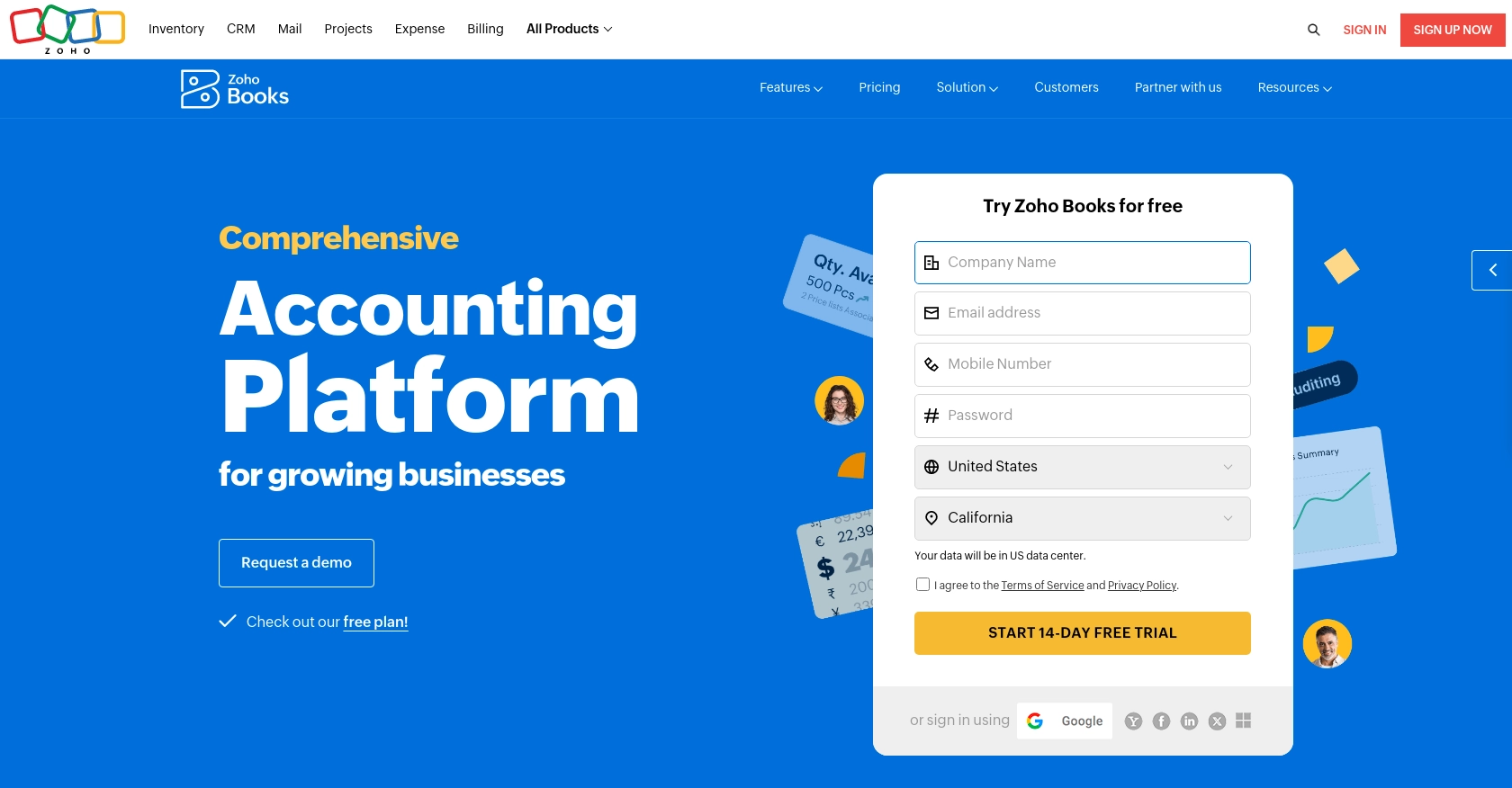
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It is a part of the Zoho suite of applications, known for its robust features and user-friendly interface.
Integrating with the Zoho Books API allows developers to automate financial operations, such as creating or updating invoices. For example, a developer might use the API to automatically generate invoices from sales data collected in another system, streamlining the billing process and reducing manual entry errors.
Setting Up Your Zoho Books Test or Sandbox Account
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account. Once your account is set up, you can log in to access the dashboard.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication. Follow these steps to register your application and obtain the necessary credentials:
- Go to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details, such as the client name, redirect URI, and authorized domains.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Generating OAuth Tokens
With your Client ID and Client Secret, you can generate OAuth tokens to authenticate API requests:
- Redirect users to the following authorization URL to obtain a grant token:
- After the user consents, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- The response will include an access_token and a refresh_token. Use the access token for API calls. Refresh tokens can be used to obtain new access tokens when the current one expires.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.invoices.CREATE,ZohoBooks.invoices.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
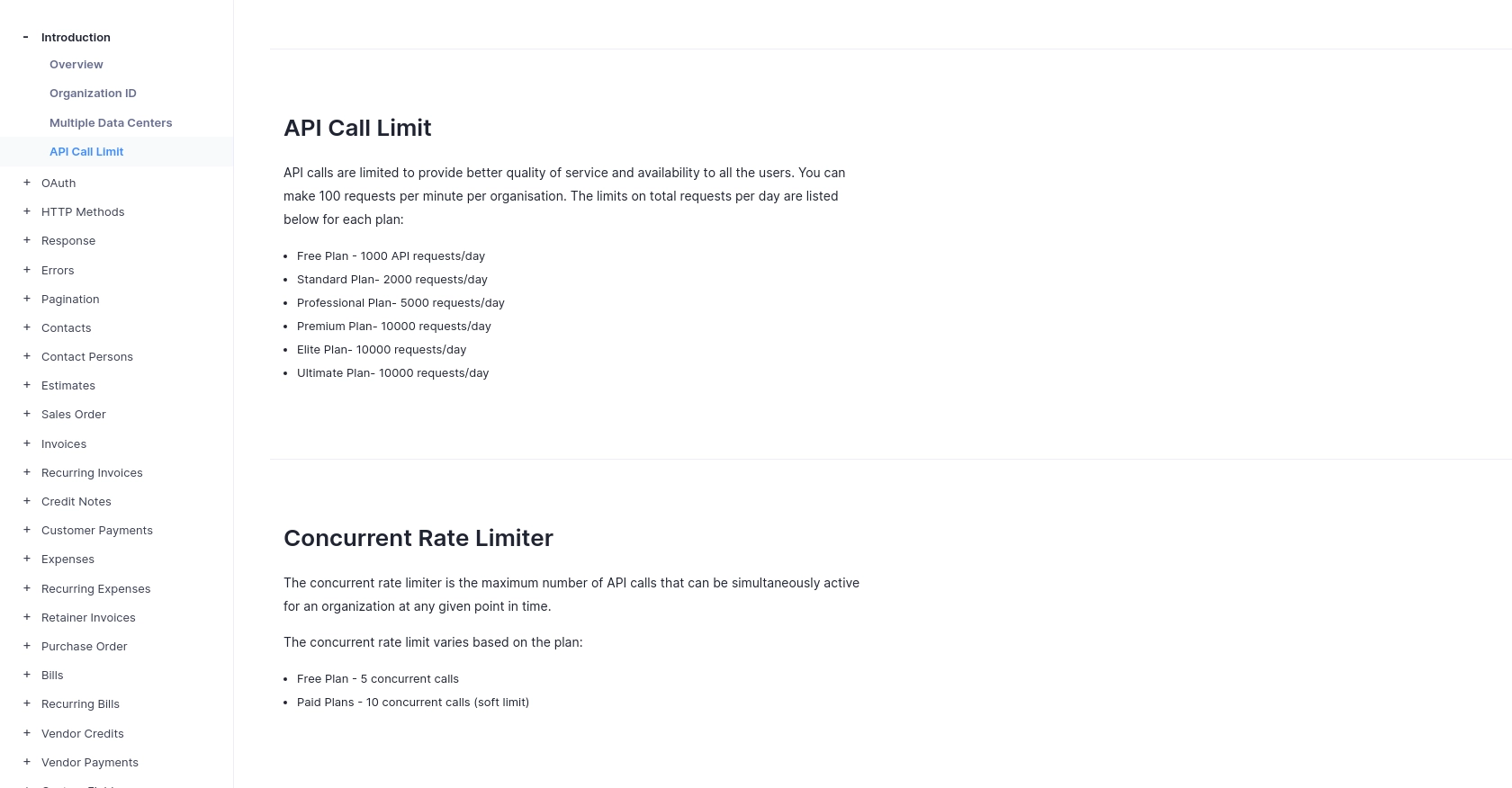
sbb-itb-96038d7
Making API Calls to Zoho Books for Invoice Management Using PHP
To interact with the Zoho Books API for creating or updating invoices, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Zoho Books API Integration
Before you begin, ensure that you have the following prerequisites installed on your system:
- PHP 7.4 or higher
- Composer for managing dependencies
You'll also need the GuzzleHTTP
library to make HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Creating an Invoice with Zoho Books API Using PHP
To create an invoice, you'll need to make a POST request to the Zoho Books API endpoint. Here's a step-by-step guide:
- Set up the API endpoint and headers:
- Prepare the invoice data:
- Make the API call:
use GuzzleHttp\Client;
$client = new Client();
$endpoint = 'https://www.zohoapis.com/books/v3/invoices?organization_id=YOUR_ORG_ID';
$headers = [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
];
$data = [
'customer_id' => 'CUSTOMER_ID',
'line_items' => [
[
'item_id' => 'ITEM_ID',
'quantity' => 1,
'rate' => 100
]
],
'invoice_number' => 'INV-00001',
'date' => '2023-10-01',
'due_date' => '2023-10-15'
];
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $data
]);
$result = json_decode($response->getBody(), true);
echo 'Invoice Created: ' . $result['invoice']['invoice_number'];
Updating an Existing Invoice in Zoho Books Using PHP
To update an invoice, you'll need to make a PUT request. Follow these steps:
- Set up the API endpoint for updating:
- Prepare the updated data:
- Make the PUT request:
$invoiceId = 'INVOICE_ID';
$updateEndpoint = "https://www.zohoapis.com/books/v3/invoices/$invoiceId?organization_id=YOUR_ORG_ID";
$updateData = [
'line_items' => [
[
'item_id' => 'ITEM_ID',
'quantity' => 2,
'rate' => 150
]
]
];
$updateResponse = $client->put($updateEndpoint, [
'headers' => $headers,
'json' => $updateData
]);
$updateResult = json_decode($updateResponse->getBody(), true);
echo 'Invoice Updated: ' . $updateResult['invoice']['invoice_number'];
Handling API Responses and Errors for Zoho Books
After making API calls, it's crucial to handle responses and errors properly:
- Check the HTTP status code to determine if the request was successful (e.g., 200 for success, 400 for bad request).
- Parse the JSON response to access the data returned by the API.
- Handle errors by checking the response code and message. For example, a 401 status code indicates an unauthorized request due to an invalid token.
For detailed error codes and their meanings, refer to the Zoho Books API error documentation.
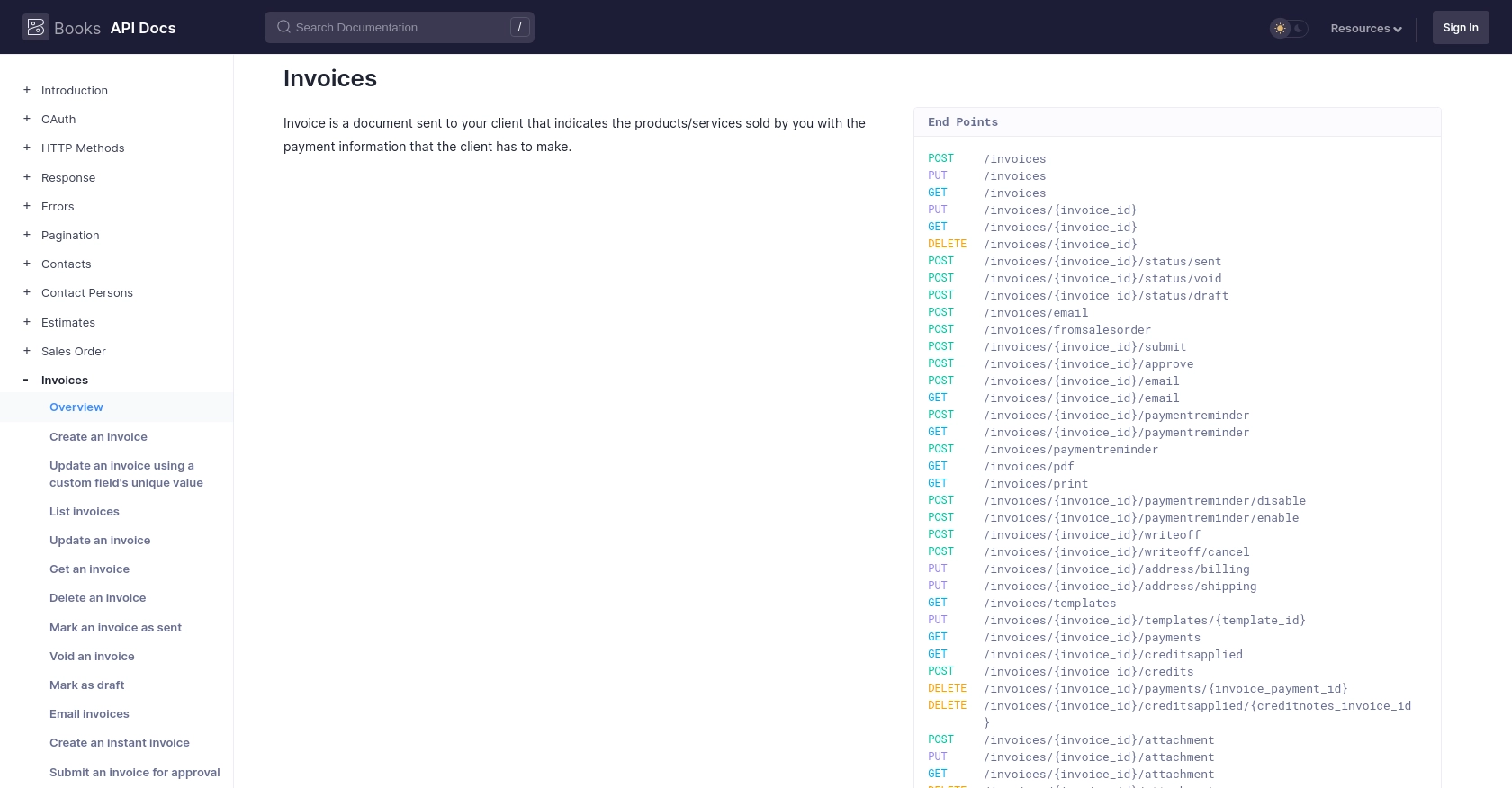
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using PHP can significantly streamline your financial operations by automating invoice management. By following the steps outlined in this guide, you can efficiently create and update invoices, reducing manual errors and saving time.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store Credentials: Always keep your OAuth credentials, such as Client ID and Client Secret, secure. Avoid hardcoding them in your source code. Use environment variables or secure vaults to store sensitive information.
- Handle Rate Limits: Zoho Books API has rate limits of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the API call limit documentation.
- Error Handling: Implement robust error handling by checking HTTP status codes and parsing error messages. This ensures that your application can respond appropriately to issues such as authentication failures or invalid data.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your systems. This can help prevent errors and improve data integrity.
Enhance Your Integration Strategy with Endgrate
While integrating with Zoho Books API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Zoho Books. By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/invoices/#overview
Ready to get started?