Using the Zoho Books API to Create or Update Vendors in Python
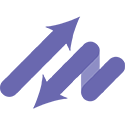
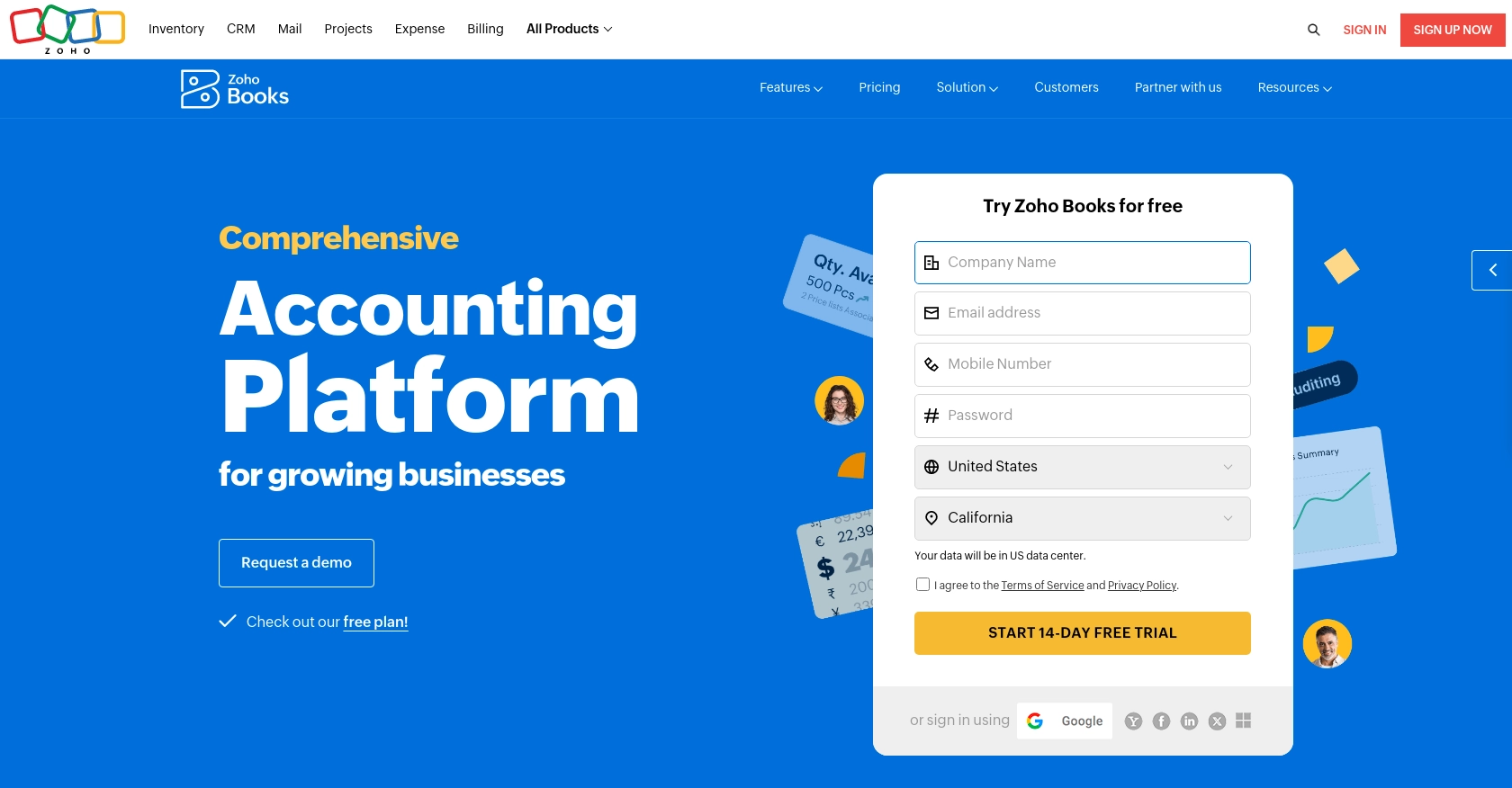
Introduction to Zoho Books API for Vendor Management
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial management for businesses. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to automate their accounting processes.
Integrating with the Zoho Books API allows developers to efficiently manage vendor data, which is crucial for maintaining accurate financial records. By using the API, developers can automate tasks such as creating or updating vendor information, ensuring that the data remains consistent and up-to-date across platforms.
For example, a developer might want to use the Zoho Books API to automatically update vendor details in response to changes in a CRM system, thereby reducing manual data entry and minimizing errors.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Zoho Books Account
- Visit the Zoho Books website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is set up, log in to access the Zoho Books dashboard.
Register a New Application in Zoho's Developer Console
To interact with the Zoho Books API, you need to register your application to obtain the necessary OAuth credentials:
- Go to the Zoho Developer Console.
- Click on Add Client ID to register a new application.
- Provide the required details, such as the application name and redirect URI.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure.
Generate OAuth Tokens for Zoho Books API Access
With your application registered, you can now generate the OAuth tokens needed to authenticate API requests:
- Redirect to the following authorization URL, replacing the placeholders with your actual values:
- Authorize the application when prompted. You will be redirected to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request to the following URL:
- In the response, you will receive an access_token and a refresh_token. Use these tokens to authenticate your API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on Zoho Books API authentication, refer to the official Zoho Books OAuth documentation.
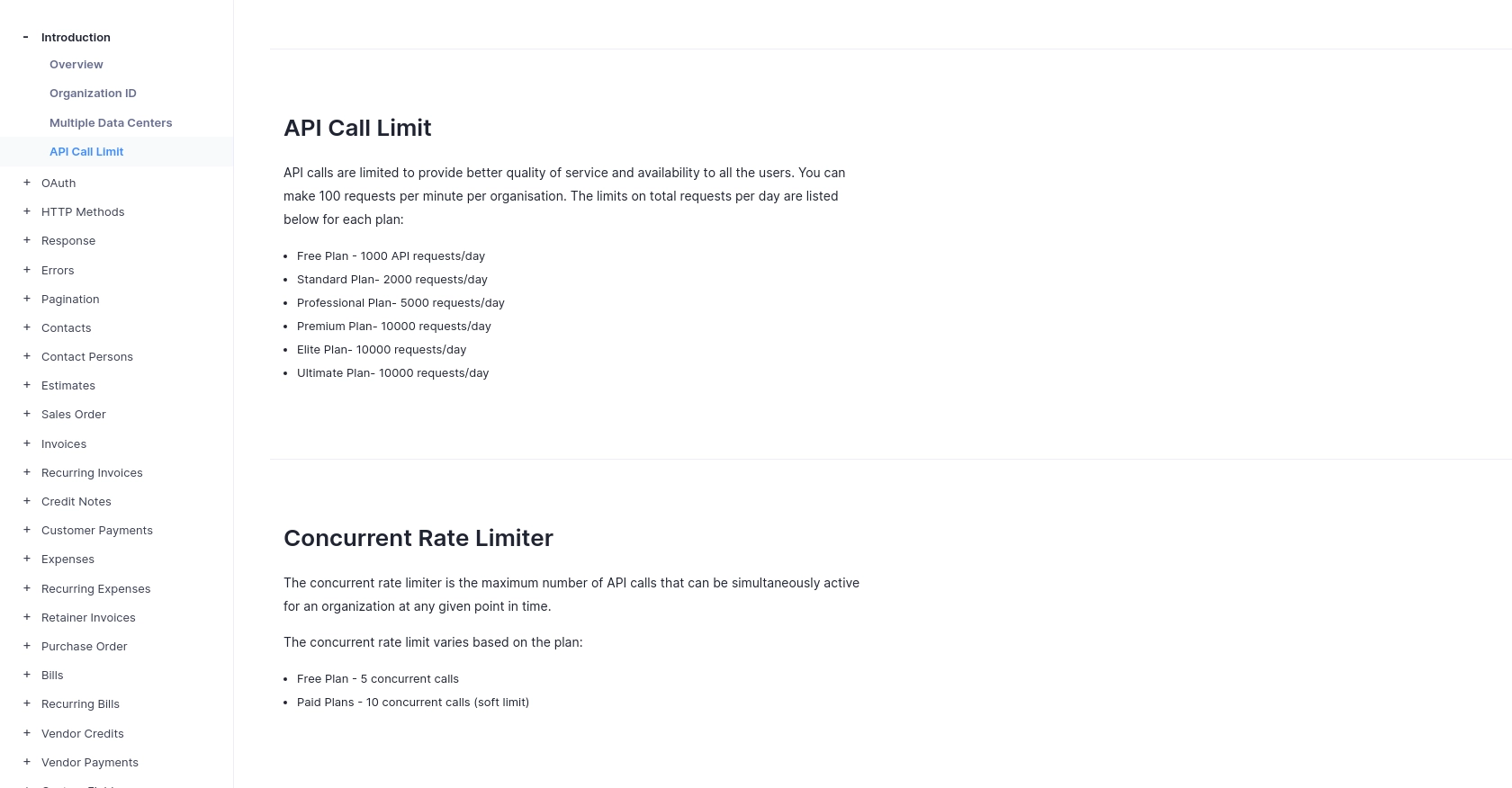
sbb-itb-96038d7
Making API Calls to Create or Update Vendors in Zoho Books Using Python
To interact with the Zoho Books API for creating or updating vendor information, you'll need to use Python. This section will guide you through setting up your Python environment and making the necessary API calls.
Setting Up Your Python Environment for Zoho Books API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating a Vendor in Zoho Books Using the API
To create a vendor in Zoho Books, you'll need to make a POST request to the API endpoint. Here's a step-by-step guide:
import requests # Define the API endpoint and headers url = "https://www.zohoapis.com/books/v3/contacts?organization_id=YOUR_ORG_ID" headers = { "Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN", "Content-Type": "application/json" } # Define the vendor data vendor_data = { "contact_name": "Vendor Name", "contact_type": "vendor", "company_name": "Vendor Company", "billing_address": { "address": "123 Vendor St", "city": "Vendor City", "state": "Vendor State", "zip": "12345", "country": "Vendor Country" } } # Make the POST request response = requests.post(url, json=vendor_data, headers=headers) # Check the response if response.status_code == 201: print("Vendor created successfully.") else: print("Failed to create vendor:", response.json())
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. If successful, the response will confirm the vendor's creation.
Updating a Vendor in Zoho Books Using the API
To update an existing vendor, use a PUT request. Here's how:
# Define the API endpoint for updating a vendor vendor_id = "VENDOR_ID" url = f"https://www.zohoapis.com/books/v3/contacts/{vendor_id}?organization_id=YOUR_ORG_ID" # Define the updated vendor data updated_vendor_data = { "contact_name": "Updated Vendor Name", "company_name": "Updated Vendor Company" } # Make the PUT request response = requests.put(url, json=updated_vendor_data, headers=headers) # Check the response if response.status_code == 200: print("Vendor updated successfully.") else: print("Failed to update vendor:", response.json())
Replace VENDOR_ID
with the ID of the vendor you wish to update. The response will indicate whether the update was successful.
Handling Errors and Verifying API Requests in Zoho Books
It's important to handle potential errors when making API calls. Zoho Books uses HTTP status codes to indicate success or failure:
- 200: OK
- 201: Created
- 400: Bad Request
- 401: Unauthorized
- 404: Not Found
- 429: Rate Limit Exceeded
- 500: Internal Server Error
For more detailed error information, refer to the Zoho Books API error documentation.
To verify the success of your requests, check the Zoho Books dashboard to ensure that the vendor data reflects the changes made through the API.
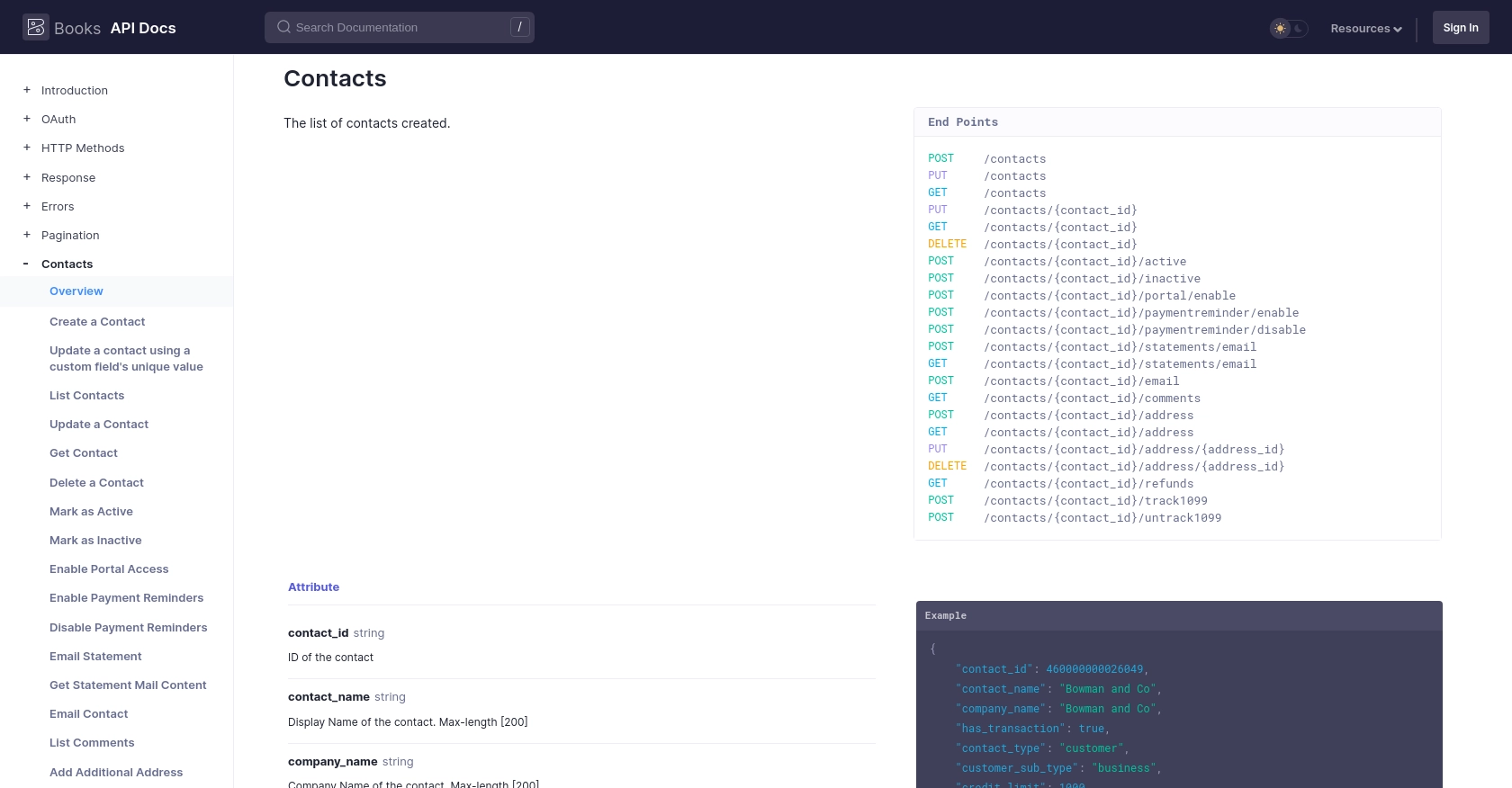
Best Practices for Zoho Books API Integration
When integrating with the Zoho Books API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP status code 429) by pausing requests and retrying after a delay. For more details, refer to the API call limit documentation.
- Data Standardization: Ensure that the data you send and receive is standardized and consistent with your application's data models. This helps maintain data integrity across systems.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes. Log errors for debugging and provide user-friendly messages for operational issues.
Streamlining Vendor Management with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Books. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while outsourcing integration complexities to Endgrate.
- Build Once, Use Everywhere: Develop integration logic once and apply it across multiple platforms, reducing redundancy.
- Enhance Customer Experience: Offer seamless and intuitive integration experiences to your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?