Using the Zoho Books API to Get Customers (with Javascript examples)
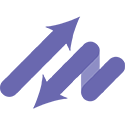
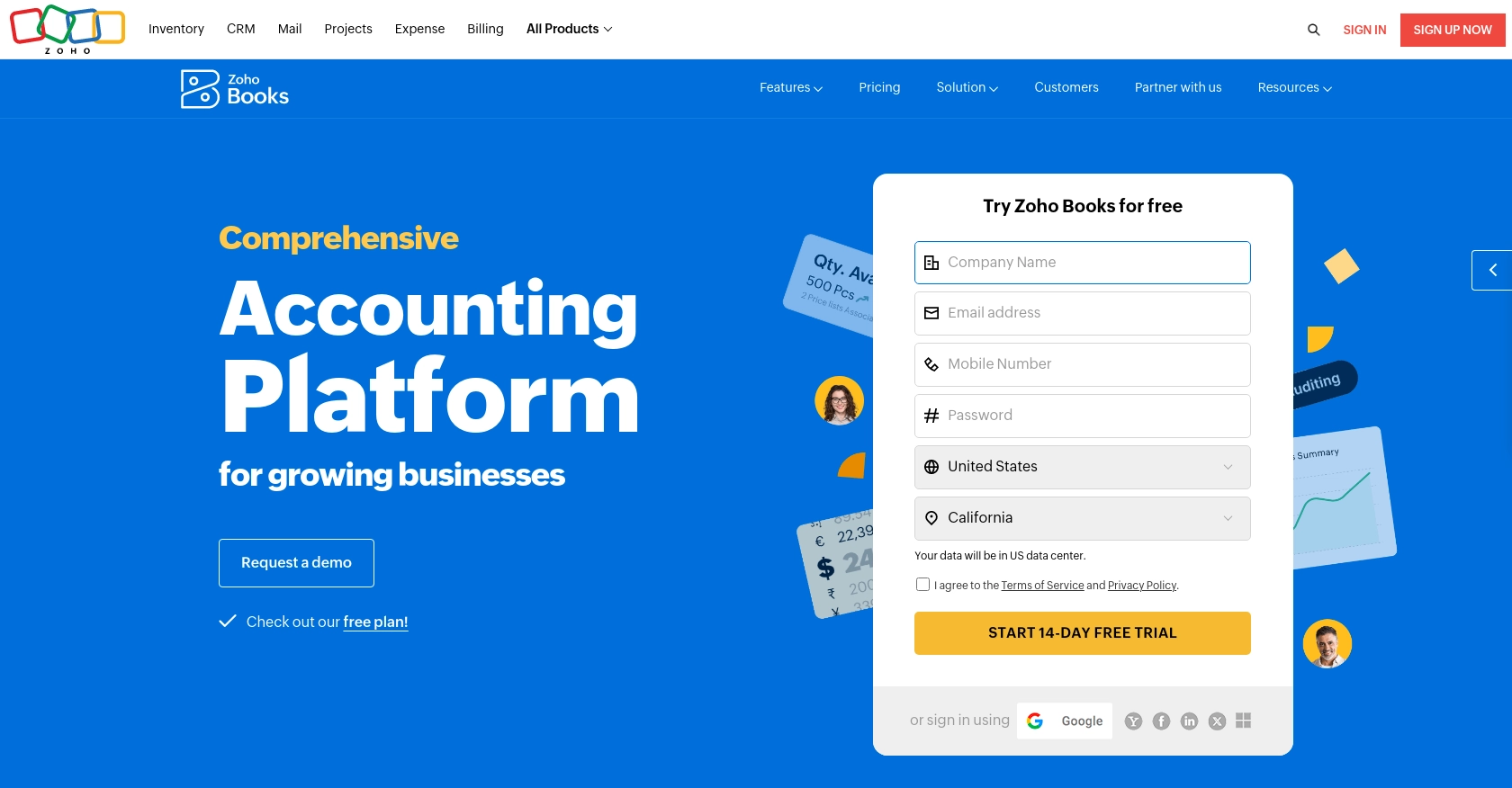
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to streamline financial management for businesses of all sizes. It offers a wide range of features, including invoicing, expense tracking, and financial reporting, making it an essential tool for managing business finances efficiently.
Developers may want to integrate with the Zoho Books API to automate and enhance their financial processes. For example, using the API, developers can retrieve customer data to generate detailed financial reports or automate billing processes. This can significantly reduce manual effort and improve accuracy in financial operations.
In this article, we'll explore how to use JavaScript to interact with the Zoho Books API, specifically focusing on retrieving customer information. This integration can be particularly useful for businesses looking to automate customer data management and improve their financial workflows.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start interacting with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Zoho Books offers a free trial that you can use to access the API and test your integration.
Creating a Zoho Books Account
To get started, visit the Zoho Books signup page and create a new account. You can choose the free trial option to explore the features and functionalities Zoho Books offers. Follow the on-screen instructions to complete the registration process.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for secure API authentication. Follow these steps to register your application and obtain the necessary credentials:
- Go to the Zoho Developer Console and sign in with your Zoho account.
- Click on Add Client ID to register a new application.
- Fill in the required details, such as the application name, homepage URL, and authorized redirect URIs.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API authentication.
Generating OAuth Tokens
With your Client ID and Client Secret, you can now generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL to obtain a grant token:
- After the user consents, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- The response will include an access_token and a refresh_token. Use the access token in your API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.contacts.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
Managing API Rate Limits
Zoho Books imposes rate limits on API requests to ensure fair usage. You can make up to 100 requests per minute per organization. Be mindful of these limits to avoid disruptions in your integration. For more details, refer to the API Call Limit documentation.
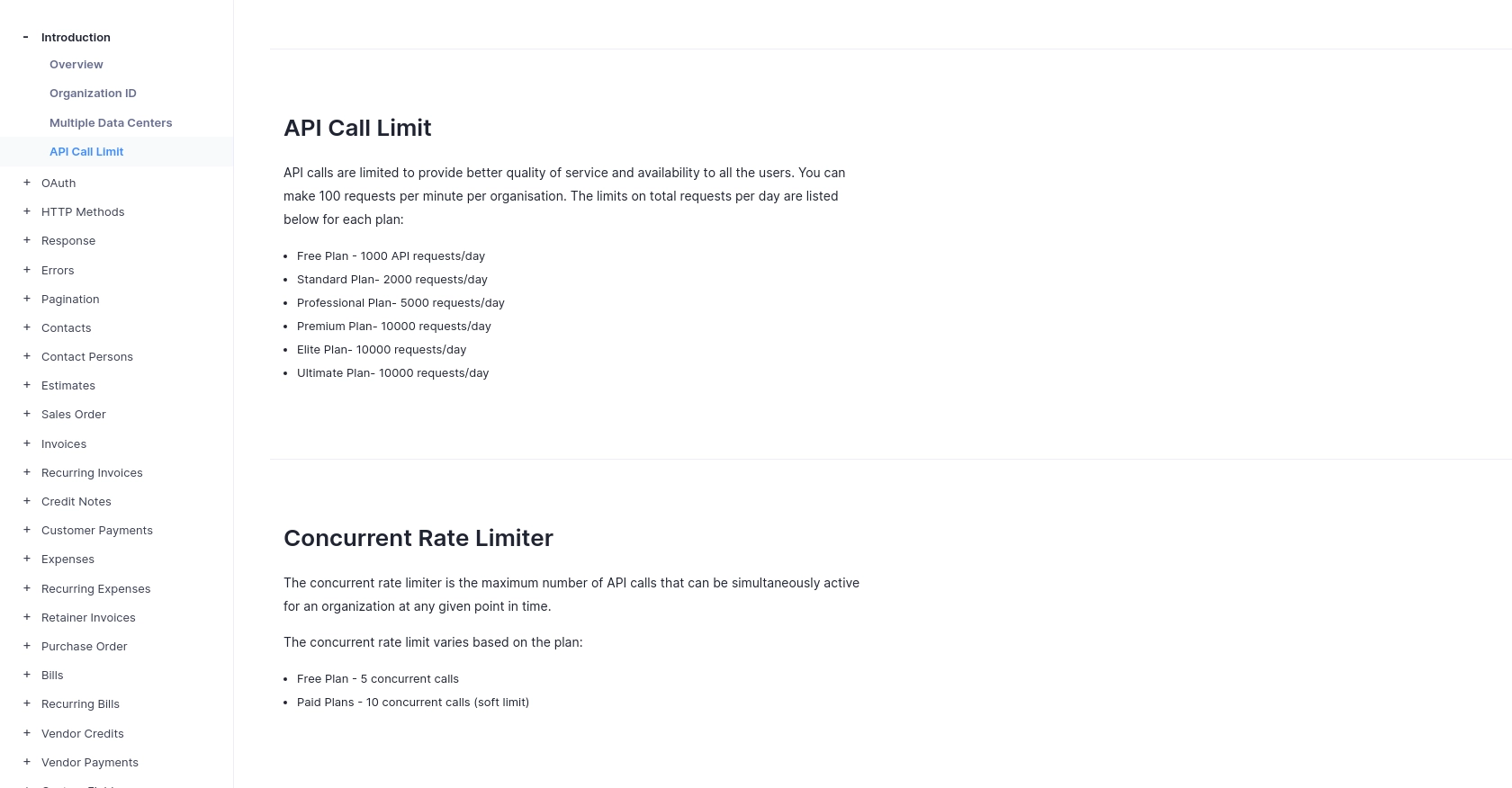
sbb-itb-96038d7
Making API Calls to Retrieve Customers from Zoho Books Using JavaScript
To interact with the Zoho Books API and retrieve customer data, you'll need to set up your JavaScript environment and make HTTP requests to the API endpoints. This section will guide you through the process of making API calls to fetch customer information using JavaScript.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside a browser. You can download and install Node.js from the official website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the necessary packages. For this tutorial, we'll use the node-fetch
package to make HTTP requests. Run the following command in your terminal to install it:
npm install node-fetch
Fetching Customer Data from Zoho Books API
With your environment set up, you can now write a script to fetch customer data from Zoho Books. The API endpoint for listing contacts is /contacts
, and you can filter by contact_type
to retrieve only customers.
Here's an example script to get customer data:
const fetch = require('node-fetch');
const getCustomers = async () => {
const url = 'https://www.zohoapis.com/books/v3/contacts?organization_id=YOUR_ORG_ID&contact_type=customer';
const options = {
method: 'GET',
headers: {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
}
};
try {
const response = await fetch(url, options);
if (response.ok) {
const data = await response.json();
console.log('Customers:', data.contacts);
} else {
console.error('Error:', response.status, response.statusText);
}
} catch (error) {
console.error('Fetch error:', error);
}
};
getCustomers();
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. This script makes a GET request to the Zoho Books API to retrieve a list of customers and logs the response to the console.
Handling API Responses and Errors
After making the API call, you should verify the response to ensure the request was successful. The Zoho Books API uses HTTP status codes to indicate the result of an API call:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 404 Not Found: The requested resource was not found.
- 429 Rate Limit Exceeded: Too many requests were made in a short period.
For more details on error codes, refer to the Zoho Books API Error Documentation.
By checking the response status and handling errors appropriately, you can ensure a robust integration with the Zoho Books API.
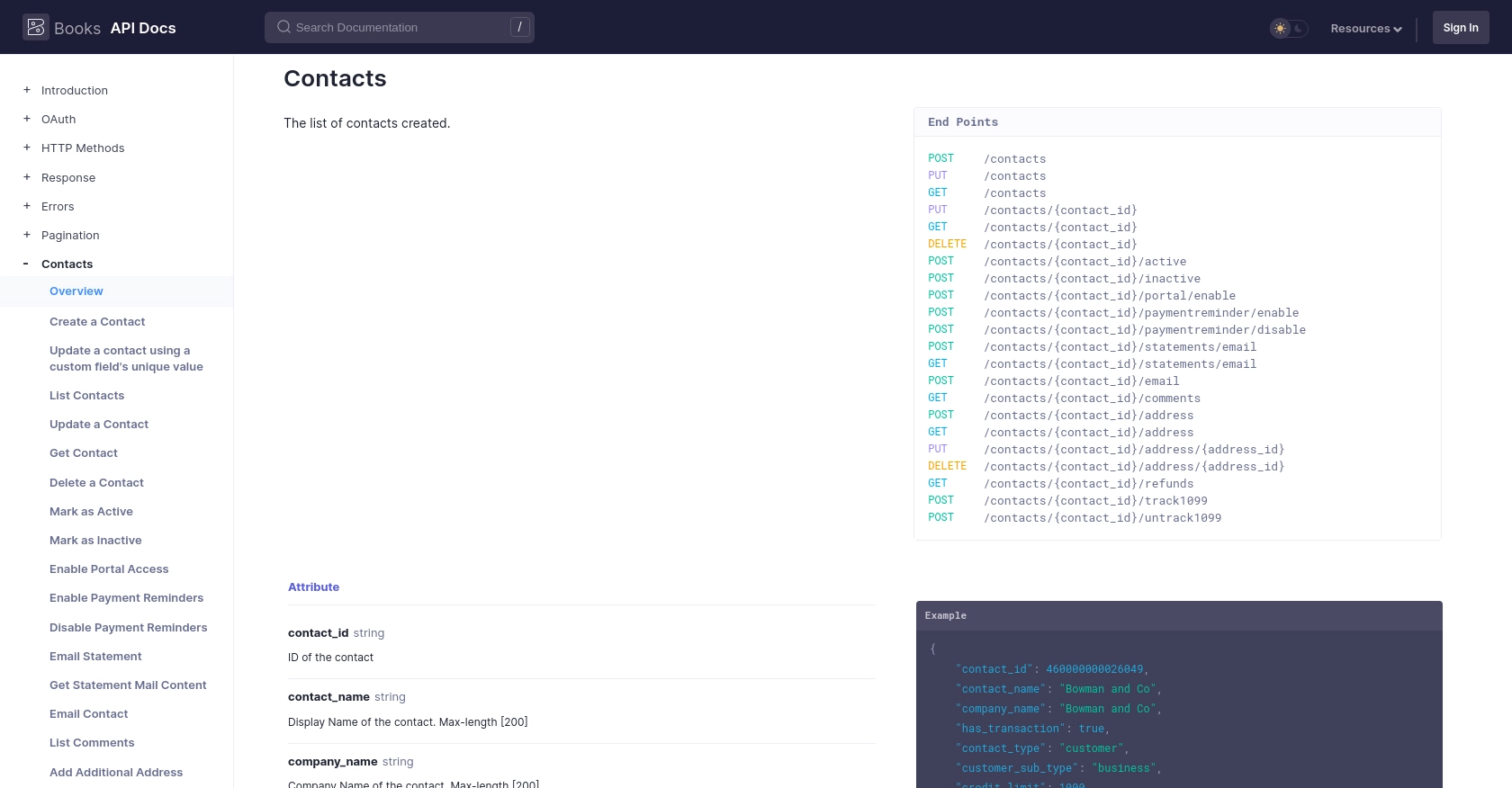
Conclusion and Best Practices for Integrating with Zoho Books API
Integrating with the Zoho Books API using JavaScript can significantly enhance your business's financial operations by automating customer data management and streamlining workflows. By following the steps outlined in this guide, you can efficiently retrieve customer information and handle API responses effectively.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always keep your Client ID, Client Secret, and access tokens secure. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Zoho Books' API rate limits, which allow up to 100 requests per minute per organization. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the API Call Limit documentation.
- Standardize Data Fields: Ensure that the data retrieved from Zoho Books is standardized and transformed as needed for your application. This will help maintain consistency and accuracy across your systems.
- Error Handling: Implement robust error handling by checking HTTP status codes and using try-catch blocks to manage exceptions. Refer to the Zoho Books API Error Documentation for guidance on handling specific error codes.
Streamline Your Integrations with Endgrate
While integrating with Zoho Books API can be a powerful way to enhance your financial processes, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Zoho Books.
By leveraging Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. Focus on your core product while providing an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?