Using the Zoho Books API to Get Items in Javascript
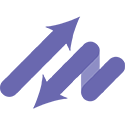
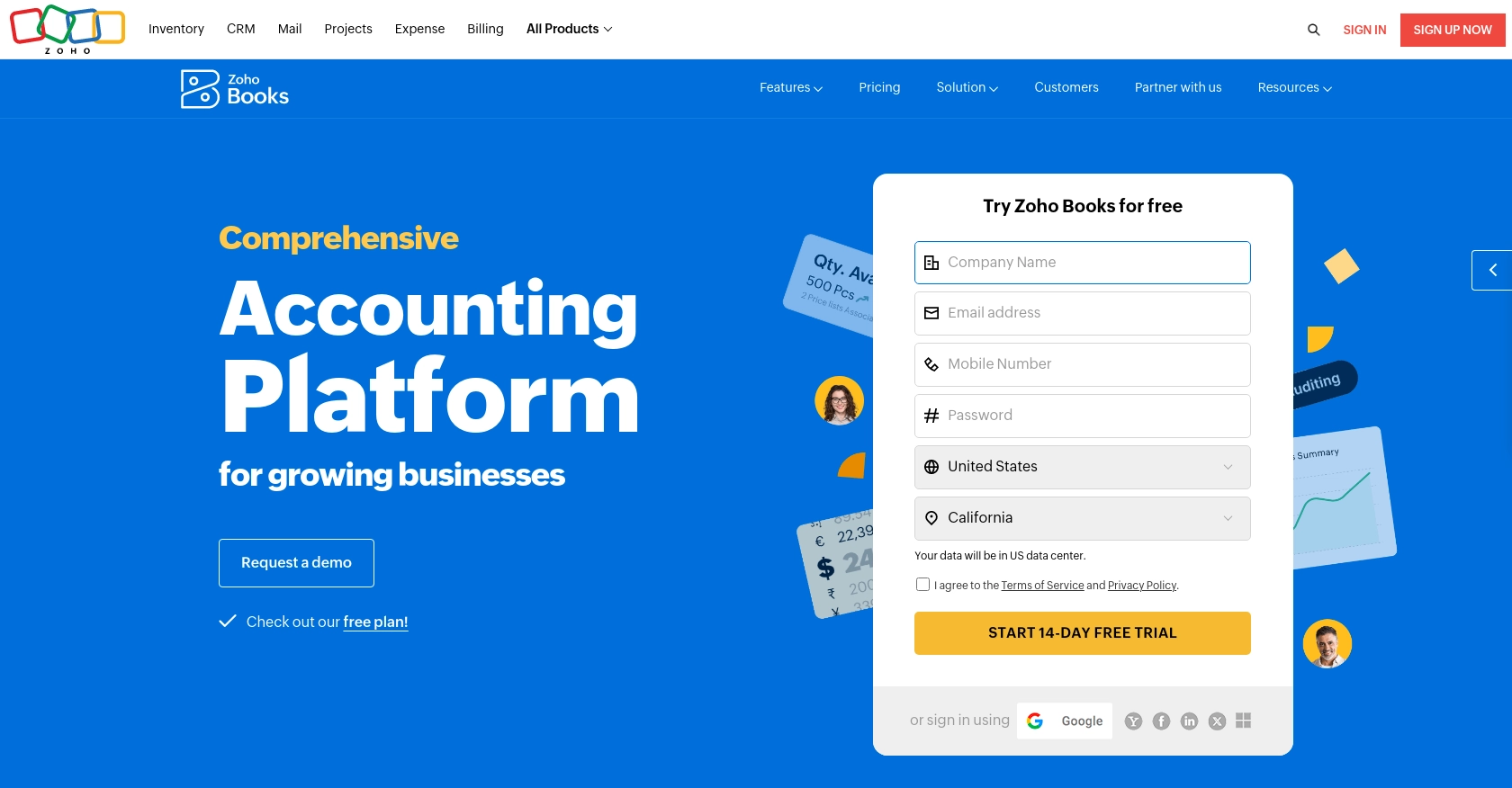
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to seamlessly interact with various financial data and automate accounting tasks. For example, using the Zoho Books API, a developer can retrieve a list of items in the inventory, enabling real-time updates and management of product data within a business application.
This article will guide you through the process of using JavaScript to interact with the Zoho Books API, specifically focusing on retrieving items from your inventory. By the end of this tutorial, you'll be able to efficiently access and manage your inventory data using JavaScript.
Setting Up Your Zoho Books Test Account for API Integration
Before diving into the Zoho Books API, you'll need to set up a test account to experiment with API calls without affecting your live data. Zoho Books offers a sandbox environment that allows developers to test their integrations safely.
Creating a Zoho Books Sandbox Account
To get started, follow these steps to create a Zoho Books sandbox account:
- Visit the Zoho Developer Console and sign in with your Zoho account credentials.
- Navigate to the "Sandbox" section and click on "Create Sandbox" to set up a new testing environment.
- Fill in the necessary details such as organization name and other relevant information to create your sandbox account.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication, ensuring secure access to your data. Here's how to register your application:
- In the Zoho Developer Console, click on "Add Client ID" to register your application.
- Provide the required details, including the redirect URI, which is crucial for OAuth authentication.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for generating access tokens.
Generating OAuth Tokens for Zoho Books API Access
With your application registered, you can now generate OAuth tokens:
- Redirect users to the following authorization URL to obtain a grant token:
- After user consent, Zoho will redirect to your specified URI with a code parameter. Use this code to request an access token:
- In the response, you'll receive an access token and a refresh token. The access token is used for API calls, while the refresh token is used to obtain new access tokens when the current one expires.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
By following these steps, you will have a fully functional sandbox account and the necessary credentials to interact with the Zoho Books API using OAuth 2.0 authentication. This setup ensures that you can safely test and develop your integration with Zoho Books.
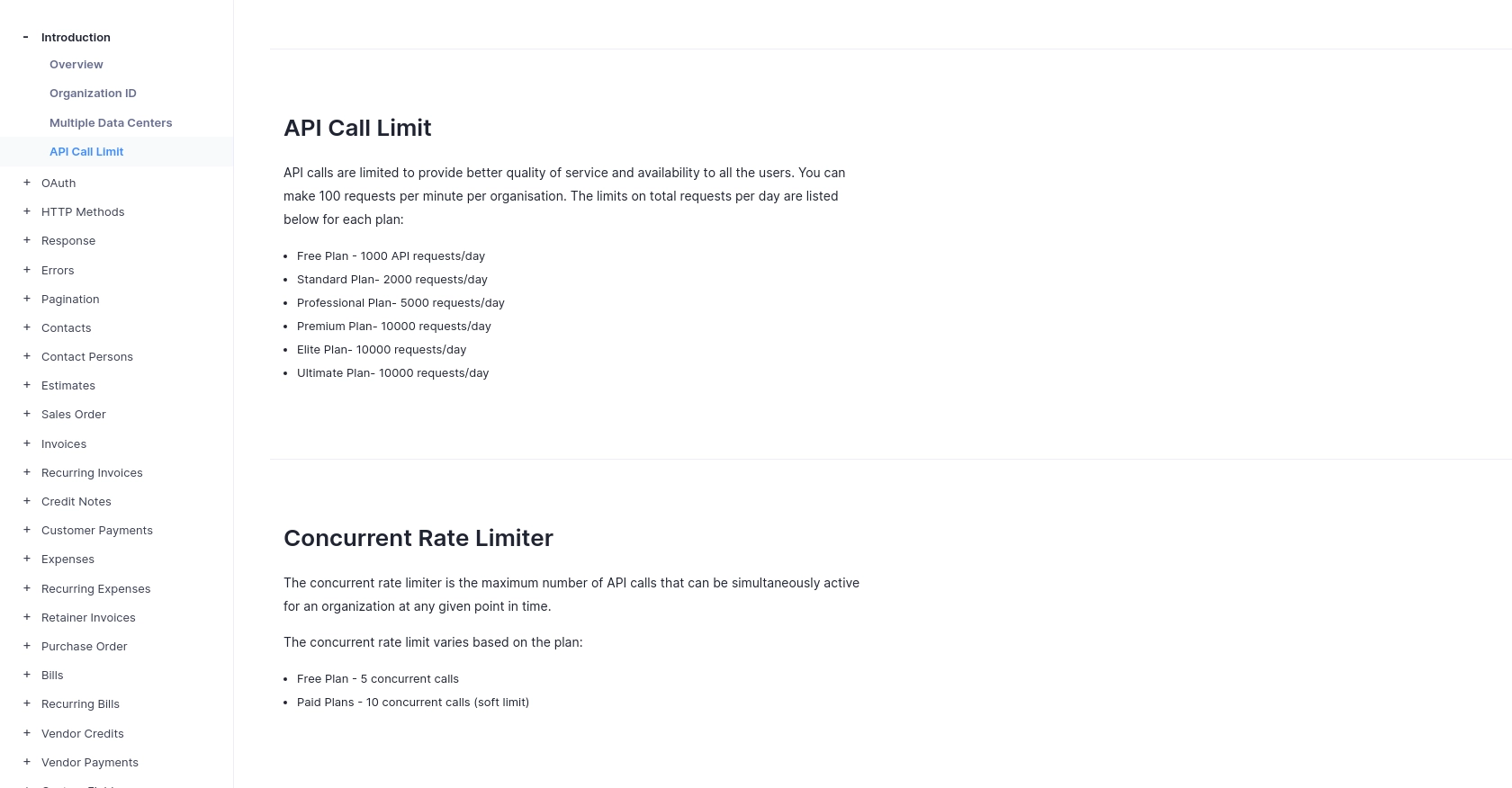
sbb-itb-96038d7
Making API Calls to Retrieve Items from Zoho Books Using JavaScript
Now that you have set up your Zoho Books sandbox account and obtained the necessary OAuth credentials, it's time to make API calls to retrieve items from your inventory using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Zoho Books API Integration
Before making API calls, ensure that you have a JavaScript environment ready. You can use Node.js or any modern web browser for this purpose. Additionally, you'll need to use the Fetch API or any HTTP client library like Axios to make HTTP requests.
Dependencies and JavaScript Version
Ensure you are using a modern version of JavaScript (ES6+) to take advantage of features like promises and async/await. If you're using Node.js, you may need to install the node-fetch
package:
npm install node-fetch
Example Code to Fetch Items from Zoho Books
Below is an example of how to retrieve items from Zoho Books using JavaScript:
const fetch = require('node-fetch');
async function getItems() {
const url = 'https://www.zohoapis.com/books/v3/items?organization_id=YOUR_ORG_ID';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
};
try {
const response = await fetch(url, { headers });
if (response.ok) {
const data = await response.json();
console.log('Items:', data.items);
} else {
console.error('Error:', response.status, response.statusText);
}
} catch (error) {
console.error('Fetch error:', error);
}
}
getItems();
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token obtained from the OAuth process.
Understanding the API Response and Handling Errors
When you make the API call, the response will include a list of items in JSON format. You can access the items using data.items
. It's crucial to handle errors gracefully by checking the response status and logging any issues.
Common HTTP status codes you might encounter include:
- 200 OK: The request was successful, and the items are returned.
- 401 Unauthorized: The access token is invalid or expired.
- 429 Rate Limit Exceeded: You've exceeded the API call limit. Refer to the API call limit documentation for more details.
Verifying Successful API Requests in Zoho Books Sandbox
To verify that your API requests are successful, check the returned data against your sandbox account. If you're retrieving items, ensure the data matches the items listed in your Zoho Books sandbox environment.
By following these steps, you can effectively retrieve and manage inventory data from Zoho Books using JavaScript, enabling seamless integration with your business applications.
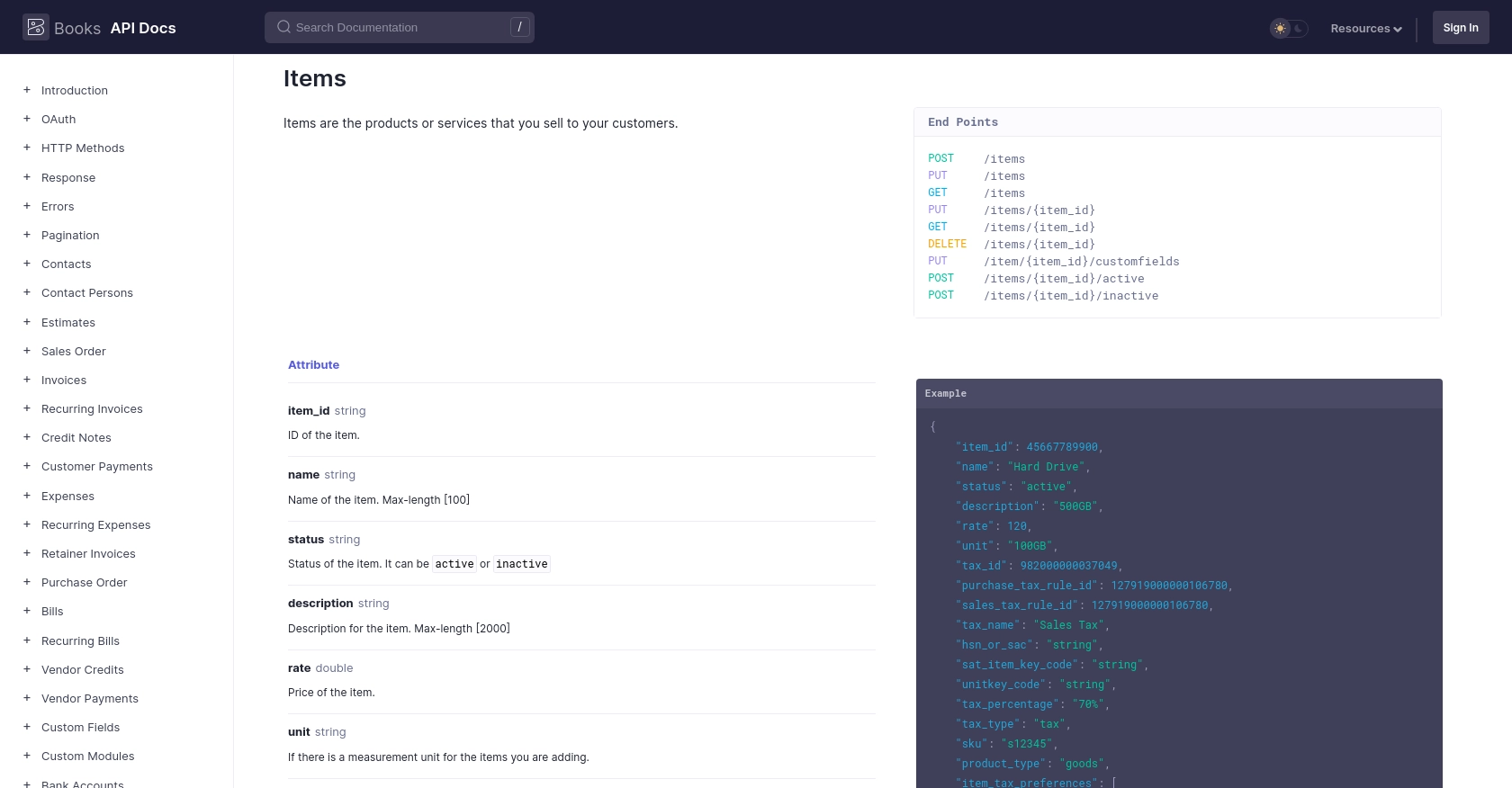
Conclusion and Best Practices for Zoho Books API Integration Using JavaScript
Integrating with the Zoho Books API using JavaScript provides a powerful way to manage your business's financial data programmatically. By following the steps outlined in this guide, you can efficiently retrieve and manage inventory items, enhancing your application's capabilities.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as Client ID, Client Secret, and access tokens, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors gracefully and retry requests as needed. For more details, refer to the API call limit documentation.
- Refresh Tokens Regularly: Access tokens expire after a certain period. Use the refresh token to obtain new access tokens without requiring user intervention.
- Validate API Responses: Always check the response status and handle errors appropriately. Refer to the error documentation for guidance on handling specific error codes.
- Optimize Data Handling: Use pagination to manage large datasets efficiently. Adjust the
per_page
parameter to control the number of records returned in each API call.
Streamline Your Integration Process with Endgrate
While integrating with Zoho Books API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Zoho Books. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/items/#overview
Ready to get started?