Using the Zoho CRM API to Create or Update Contacts (with Python examples)
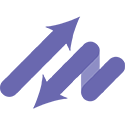
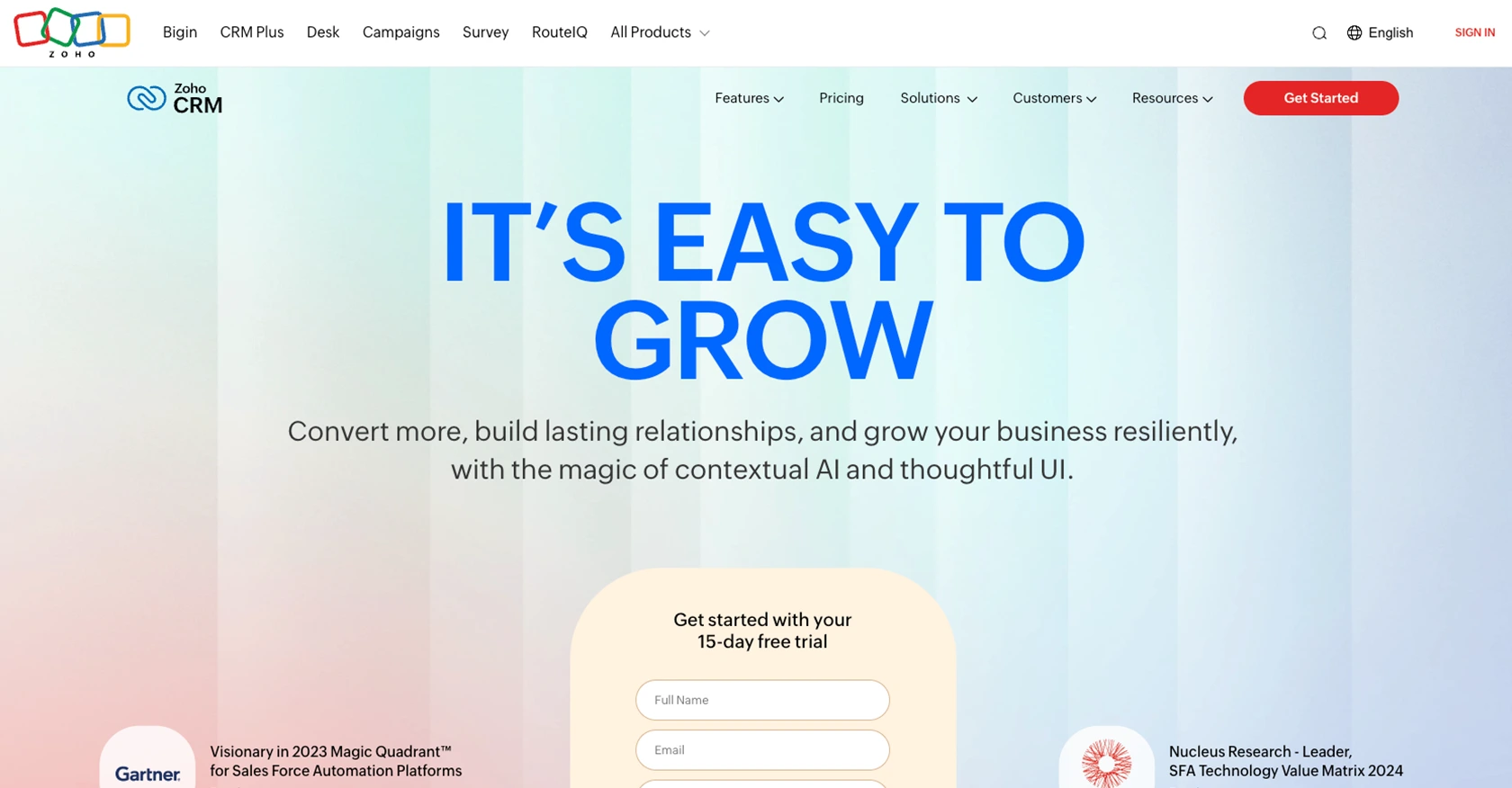
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in one unified system. Known for its flexibility and scalability, Zoho CRM is a popular choice for businesses looking to enhance their customer interactions and streamline operations.
Developers often integrate with Zoho CRM's API to automate and enhance customer data management processes. For example, using the Zoho CRM API, a developer can create or update contact information directly from a custom application, ensuring that the CRM data remains accurate and up-to-date without manual intervention.
This article will guide you through using Python to interact with the Zoho CRM API, focusing on creating and updating contacts. By following this tutorial, you can efficiently manage contact data within Zoho CRM, leveraging Python's capabilities to automate these tasks.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start integrating with the Zoho CRM API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Step 1: Register for a Zoho CRM Account
- Visit the Zoho CRM sign-up page.
- Fill in the required details to create a free account.
- Once registered, log in to your Zoho CRM account.
Step 2: Create a Zoho CRM Sandbox
The sandbox environment in Zoho CRM allows you to test API integrations without impacting your production data.
- Navigate to the Setup section in your Zoho CRM dashboard.
- Under Data Administration, select Sandbox.
- Follow the prompts to create a new sandbox environment.
Step 3: Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for secure API authentication. You'll need to register your application to obtain the necessary credentials.
- Go to the Zoho API Console.
- Click on Add Client and select Server-based Applications.
- Fill in the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Step 4: Generate Access and Refresh Tokens
With your client credentials, you can now generate access and refresh tokens to authenticate API requests.
import requests
# Define the token endpoint and payload
token_url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
"grant_type": "authorization_code",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"redirect_uri": "YOUR_REDIRECT_URI",
"code": "AUTHORIZATION_CODE"
}
# Make a POST request to obtain tokens
response = requests.post(token_url, data=payload)
tokens = response.json()
print(tokens)
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, and AUTHORIZATION_CODE
with your actual values.
Step 5: Set Up Scopes for API Access
Ensure your application has the necessary scopes to access Zoho CRM modules.
- In the API Console, navigate to your application and click on Edit.
- Under Scopes, select the required permissions such as
ZohoCRM.modules.contacts.ALL
. - Save your changes to update the scopes.
With your Zoho CRM sandbox and OAuth setup complete, you're ready to start making API calls to create or update contacts using Python.
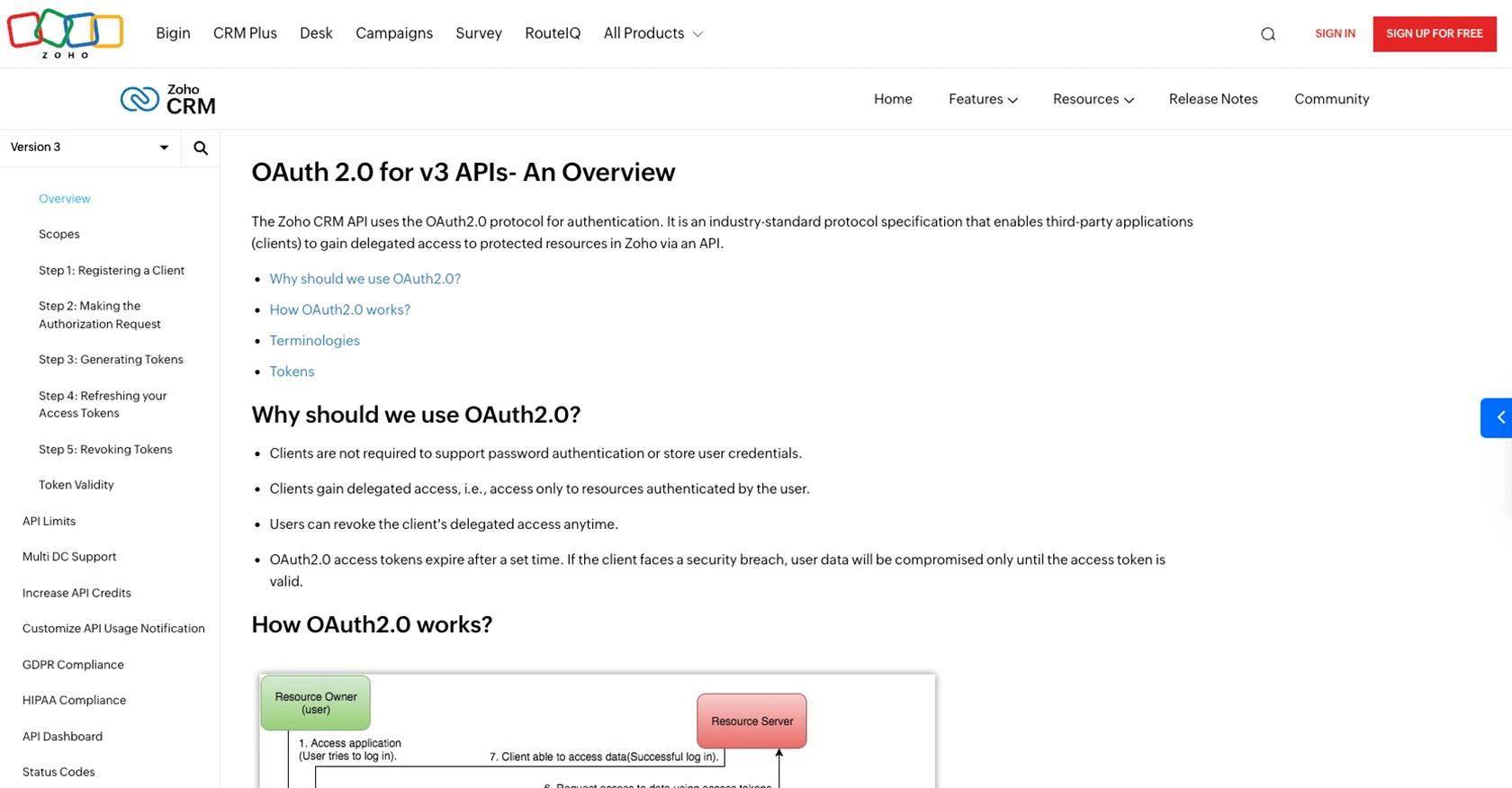
sbb-itb-96038d7
Making API Calls to Zoho CRM Using Python
With your Zoho CRM account and OAuth setup ready, you can now proceed to make API calls to create or update contacts. This section will guide you through the process using Python, ensuring you have the right tools and code to interact with Zoho CRM's API effectively.
Python Environment Setup for Zoho CRM API Integration
Before making API calls, ensure your Python environment is correctly set up. You'll need Python 3.11.1 and the requests
library to handle HTTP requests.
- Install Python 3.11.1 if it's not already installed on your machine.
- Use the following command to install the
requests
library:
pip install requests
Creating Contacts in Zoho CRM Using Python
To create a new contact in Zoho CRM, you'll need to make a POST request to the Zoho CRM API endpoint. Here's a step-by-step guide with example code:
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/crm/v3/Contacts"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the contact data
contact_data = {
"data": [
{
"First_Name": "John",
"Last_Name": "Doe",
"Email": "john.doe@example.com",
"Phone": "1234567890"
}
]
}
# Make the POST request to create a contact
response = requests.post(url, headers=headers, json=contact_data)
# Check the response
if response.status_code == 201:
print("Contact created successfully:", response.json())
else:
print("Failed to create contact:", response.json())
Replace YOUR_ACCESS_TOKEN
with your actual access token. The contact data can be customized as needed.
Updating Contacts in Zoho CRM Using Python
To update an existing contact, you'll need to know the contact's unique ID. Here's how you can update a contact's information:
import requests
# Define the API endpoint and headers
contact_id = "CONTACT_ID"
url = f"https://www.zohoapis.com/crm/v3/Contacts/{contact_id}"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated contact data
updated_data = {
"data": [
{
"Email": "new.email@example.com",
"Phone": "0987654321"
}
]
}
# Make the PUT request to update the contact
response = requests.put(url, headers=headers, json=updated_data)
# Check the response
if response.status_code == 200:
print("Contact updated successfully:", response.json())
else:
print("Failed to update contact:", response.json())
Replace CONTACT_ID
and YOUR_ACCESS_TOKEN
with the appropriate values. Modify the updated_data
dictionary to include the fields you wish to update.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors effectively. Zoho CRM API responses include status codes and messages that indicate success or failure. Here are some common status codes:
- 201 Created: The contact was successfully created.
- 200 OK: The contact was successfully updated.
- 400 Bad Request: The request was invalid, often due to incorrect data.
- 401 Unauthorized: Authentication failed, possibly due to an invalid access token.
Always check the response and handle errors gracefully to ensure a robust integration with Zoho CRM.
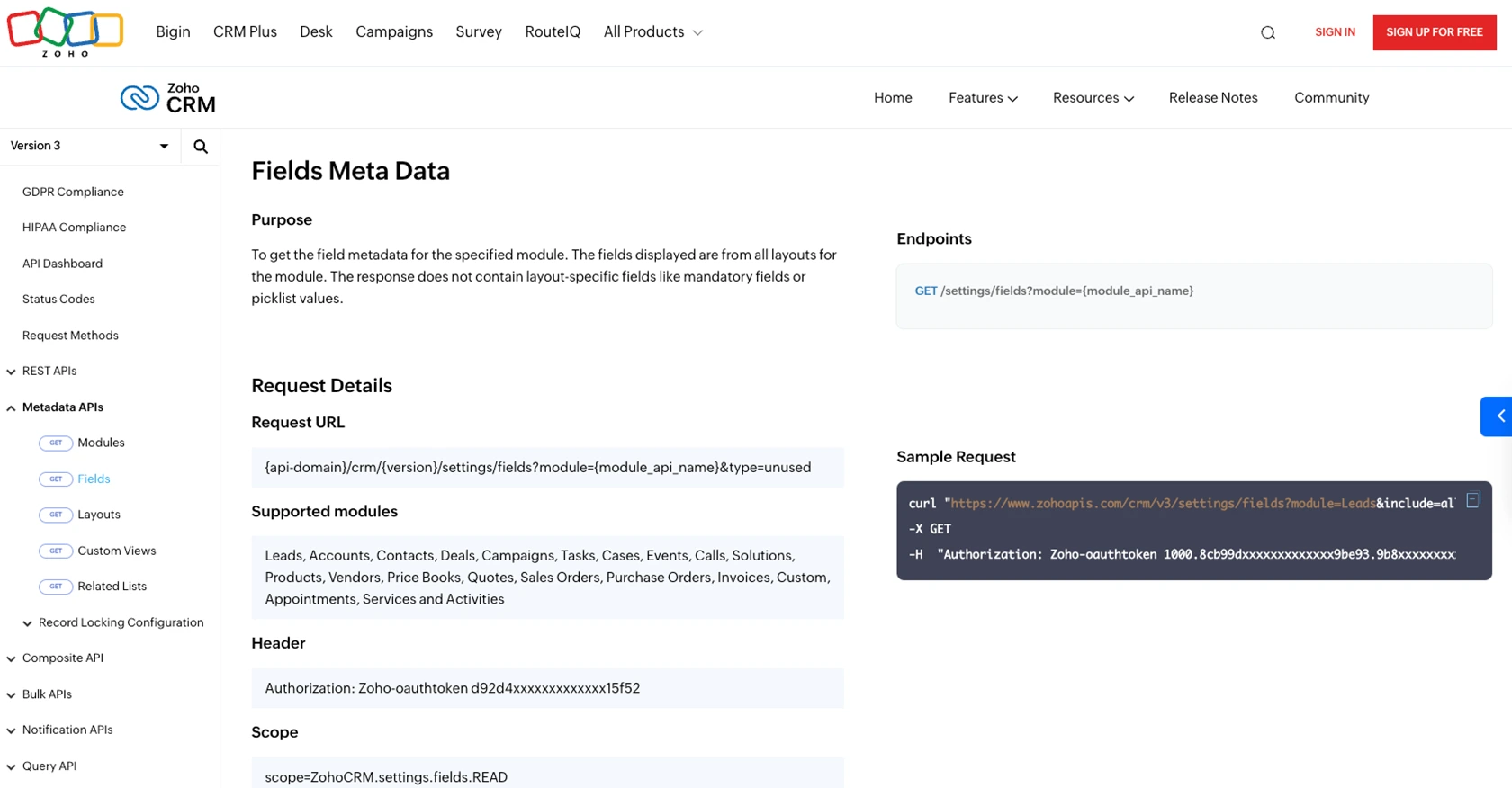
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using Python provides a powerful way to automate and enhance your customer relationship management processes. By following the steps outlined in this guide, you can efficiently create and update contacts, ensuring your CRM data remains accurate and up-to-date.
Here are some best practices to consider when working with the Zoho CRM API:
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Ensure your application handles these limits gracefully by implementing retry logic and monitoring your API usage. For more details, refer to the Zoho CRM API limits documentation.
- Validate API Responses: Always check the API responses for success or failure. Implement error handling to manage different status codes and provide meaningful feedback to users.
- Data Standardization: Ensure that the data you send to Zoho CRM is standardized and validated to prevent errors and maintain data integrity.
By adhering to these best practices, you can build robust integrations that enhance your business operations and improve customer interactions.
For developers looking to streamline their integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?