Using the Zoho Recruit API to Create or Update Candidates in PHP
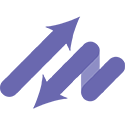
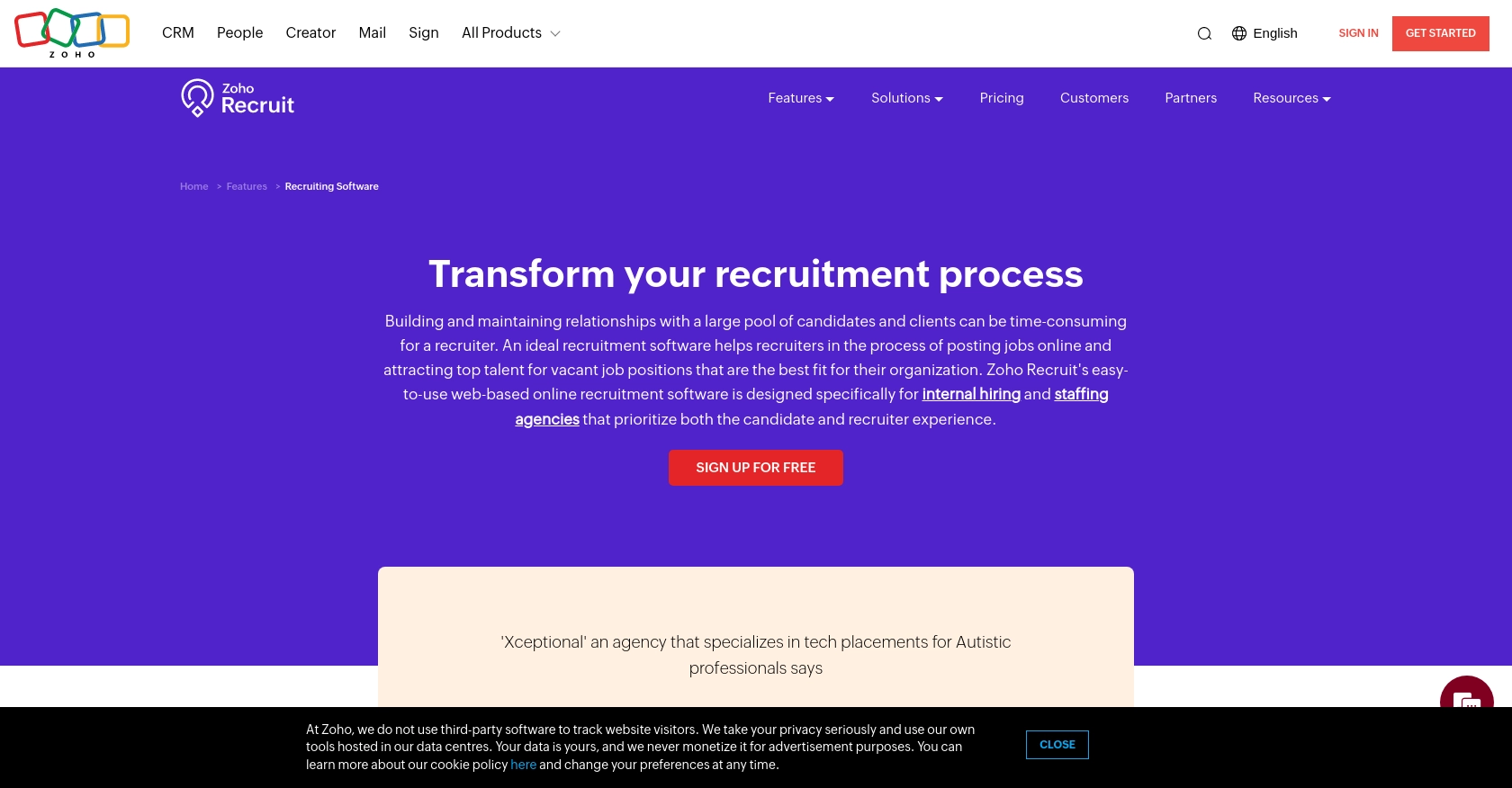
Introduction to Zoho Recruit API
Zoho Recruit is a comprehensive recruitment software solution designed to streamline the hiring process for businesses of all sizes. It offers a range of features, including candidate sourcing, resume management, and interview scheduling, making it a popular choice for staffing agencies and corporate HR departments.
Integrating with Zoho Recruit's API allows developers to automate and enhance recruitment workflows. For example, you can use the API to create or update candidate records directly from your application, ensuring that your recruitment database is always up-to-date and reducing manual data entry.
This article will guide you through using PHP to interact with the Zoho Recruit API, specifically focusing on creating or updating candidate records. By following this tutorial, you'll be able to efficiently manage candidate data within Zoho Recruit using your PHP applications.
Setting Up Your Zoho Recruit Test Account
Before you can start integrating with the Zoho Recruit API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Zoho Recruit offers a free trial that you can use to access the API and test its features.
Creating a Zoho Recruit Account
- Visit the Zoho Recruit website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process. Once registered, you'll have access to the Zoho Recruit dashboard.
Registering Your Application for OAuth Authentication
Zoho Recruit uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials.
- Go to the Zoho Developer Console.
- Click on "Add Client" and choose the client type that suits your application (e.g., Web-Based or Self Client).
- Fill in the required details:
- Client Name: The name of your application.
- Homepage URL: The URL of your application's homepage.
- Authorized Redirect URIs: The URL where Zoho will redirect after successful authentication.
- Click "Create" to register your application. You will receive a Client ID and Client Secret.
Generating Access and Refresh Tokens
With your Client ID and Client Secret, you can now generate access and refresh tokens to authenticate API requests.
- Direct your users to the Zoho authorization URL to obtain an authorization code:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoRecruit.modules.ALL&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
- Exchange the authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint:
https://accounts.zoho.com/oauth/v2/token?grant_type=authorization_code&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&code=AUTHORIZATION_CODE
- Store the access and refresh tokens securely, as they will be used to authenticate your API requests.
For more detailed information on OAuth setup, refer to the Zoho Recruit OAuth Overview and Register Client with Zoho documentation.
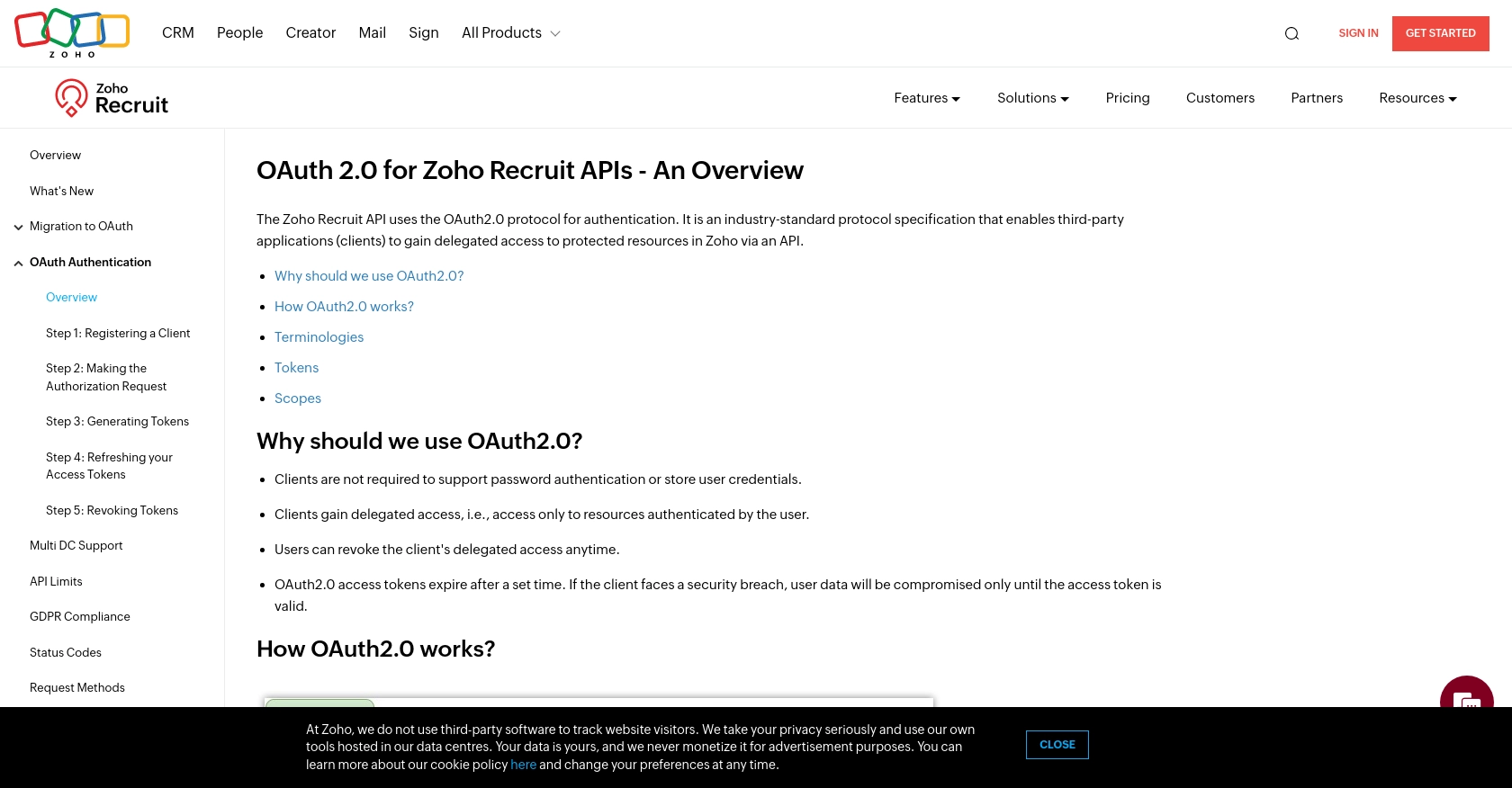
sbb-itb-96038d7
Making API Calls to Zoho Recruit Using PHP
To interact with the Zoho Recruit API using PHP, you'll need to set up your environment and write code to create or update candidate records. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
Install the required dependencies using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Candidates with Zoho Recruit API
With your environment set up, you can now write PHP code to interact with the Zoho Recruit API. The following example demonstrates how to create or update candidate records using the API.
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$apiUrl = 'https://recruit.zoho.com/recruit/v2/Candidates/upsert';
$data = [
'data' => [
[
'First_Name' => 'John',
'Last_Name' => 'Doe',
'Email' => 'john.doe@example.com'
]
],
'duplicate_check_fields' => ['Email']
];
$response = $client->post($apiUrl, [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $data
]);
if ($response->getStatusCode() == 201) {
echo "Candidate created or updated successfully.";
} else {
echo "Failed to create or update candidate.";
}
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup. This code uses the Guzzle HTTP client to send a POST request to the Zoho Recruit API, specifying the candidate data and duplicate check fields.
Verifying API Call Success in Zoho Recruit
After executing the code, verify the success of your API call by checking the Zoho Recruit dashboard. The candidate record should appear in the Candidates module. If the record already exists, it will be updated with the new information.
Handling Errors and Status Codes
It's crucial to handle potential errors when making API calls. Zoho Recruit provides various status codes to indicate the result of your request:
- 200 OK: The request was successful.
- 201 Created: The candidate was successfully created or updated.
- 400 Bad Request: The request was invalid, possibly due to incorrect parameters.
- 401 Authorization Error: Invalid API key or access token.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed information on status codes, refer to the Zoho Recruit Status Codes documentation.
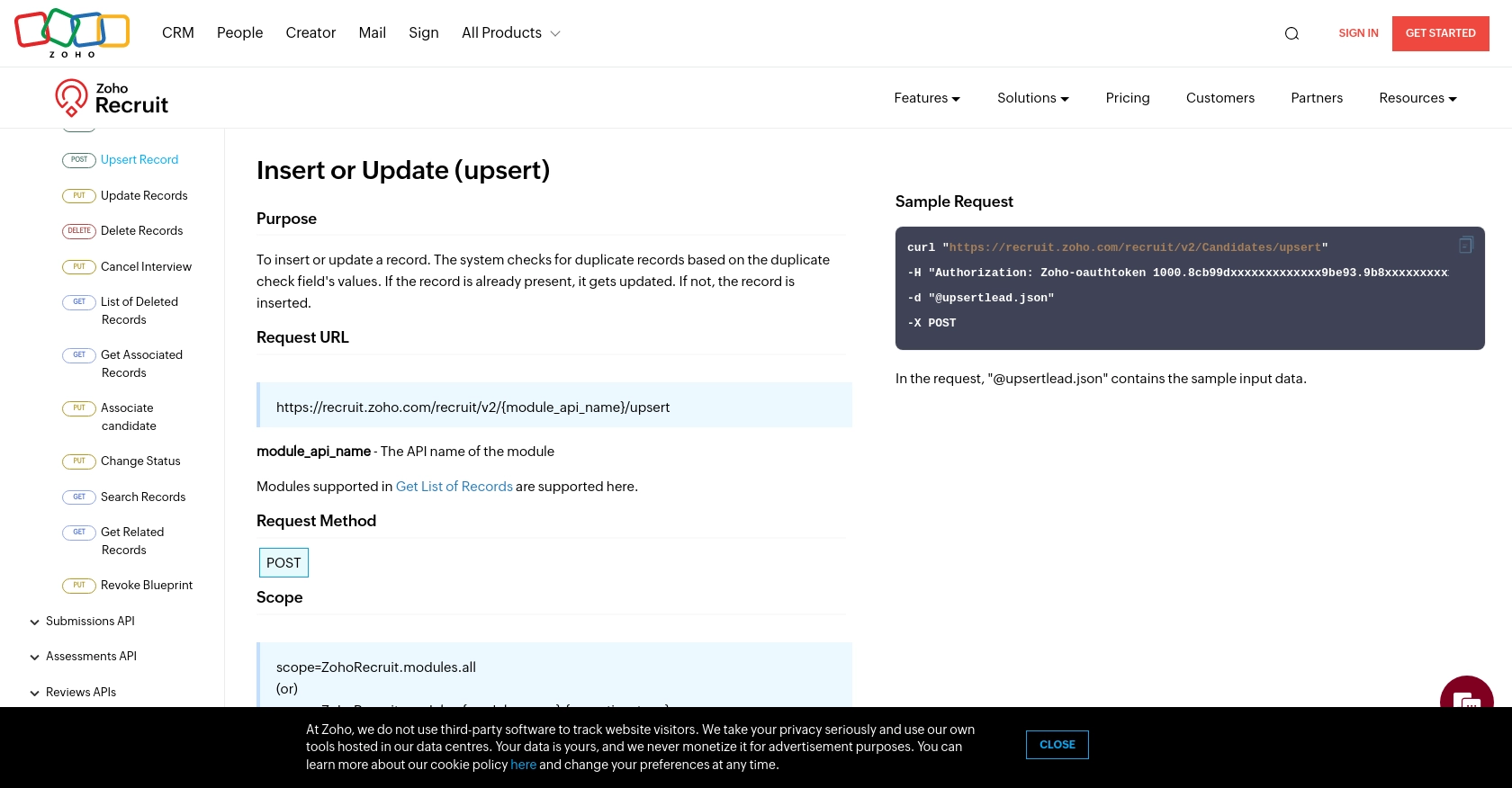
Best Practices for Using Zoho Recruit API in PHP Applications
When integrating with the Zoho Recruit API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle API Rate Limits: Zoho Recruit imposes rate limits on API requests. Monitor your application's API usage and implement retry logic with exponential backoff to handle
429 Too Many Requests
errors. For more details, refer to the Zoho Recruit API Limits documentation. - Data Validation and Error Handling: Validate data before sending it to the API to prevent errors. Implement comprehensive error handling to manage different status codes and error messages effectively.
- Token Management: Regularly refresh your access tokens using the refresh token to maintain uninterrupted access to the API. Implement logic to handle token expiration gracefully.
- Optimize API Calls: Batch multiple operations into a single API call where possible to reduce the number of requests and improve performance.
Enhancing Recruitment Workflows with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Recruit. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohorecruit
- https://www.zoho.com/recruit/developer-guide/apiv2/oauth-overview.html
- https://www.zoho.com/recruit/developer-guide/apiv2/register-client.html
- https://www.zoho.com/recruit/developer-guide/apiv2/limits.html
- https://www.zoho.com/recruit/developer-guide/apiv2/status-codes.html
- https://www.zoho.com/recruit/developer-guide/apiv2/upsert-records.html
Ready to get started?