Using the Zoom API to Get Meetings in Javascript
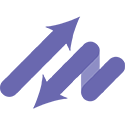
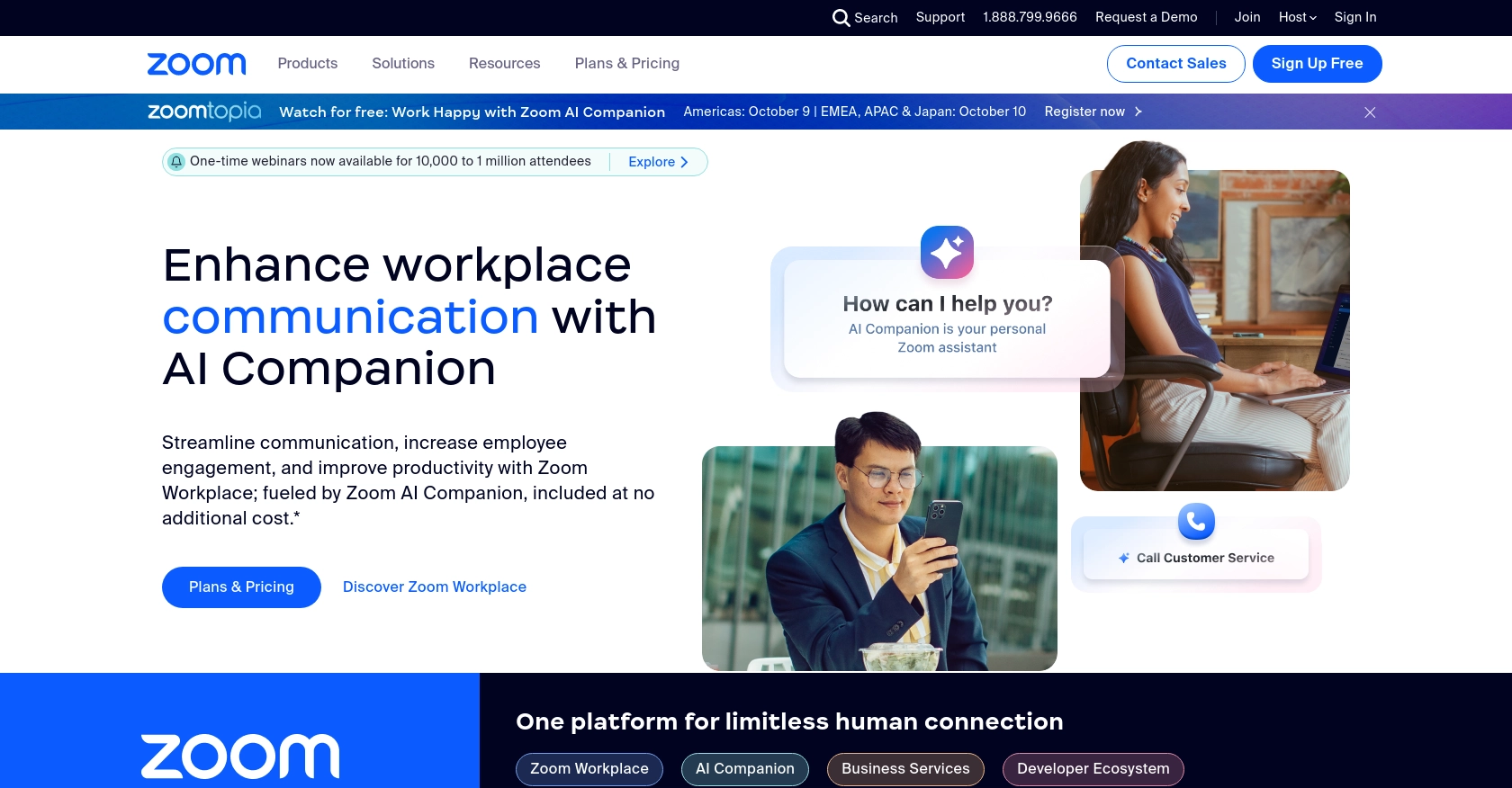
Introduction to Zoom API Integration
Zoom is a widely-used video conferencing platform that offers a range of features for virtual meetings, webinars, and collaboration. Its robust API allows developers to integrate Zoom's functionalities into their applications, enabling seamless communication and interaction.
Integrating with the Zoom API can be highly beneficial for developers looking to automate meeting management and enhance user experiences. For example, you might want to retrieve a list of scheduled meetings to display on a dashboard, allowing users to quickly access meeting details and join links.
This article will guide you through using JavaScript to interact with the Zoom API, specifically focusing on retrieving meeting information. By the end of this tutorial, you'll be equipped to efficiently manage Zoom meetings within your application.
Setting Up Your Zoom Developer Account for API Integration
Before you can start interacting with the Zoom API using JavaScript, you'll need to set up a Zoom developer account and create an app to obtain the necessary credentials for authentication. This process involves creating a sandbox environment where you can safely test your API calls without affecting live data.
Creating a Zoom Developer Account
If you don't already have a Zoom developer account, follow these steps to create one:
- Visit the Zoom App Marketplace and sign up for a developer account.
- Once registered, log in to your account to access the developer dashboard.
Setting Up an OAuth App for Zoom API Access
Zoom uses OAuth 2.0 for authentication, which requires you to create an app to obtain client credentials. Follow these steps to set up your OAuth app:
- In the Zoom App Marketplace, navigate to the "Develop" dropdown and select "Build App."
- Choose the "OAuth" app type and click "Create."
- Fill in the required information, such as the app name and description.
- Under the "App Credentials" tab, note down your Client ID and Client Secret. These will be used for authentication.
- In the "Redirect URL for OAuth" field, enter a valid URL where users will be redirected after authorization.
Configuring Scopes and Permissions for Zoom API
To interact with the Zoom API, you need to define the scopes that your app will request access to:
- Navigate to the "Scopes" tab in your app settings.
- Add the necessary scopes for accessing meeting information, such as
meeting:read
andmeeting:write
. - Save your changes to update the app configuration.
Generating an Access Token for Zoom API Calls
With your app set up, you can now generate an access token to authenticate your API requests:
- Direct users to the authorization URL provided in your app settings to obtain an authorization code.
- Exchange the authorization code for an access token by making a POST request to Zoom's token endpoint:
var request = require('request');
var options = {
method: 'POST',
url: 'https://zoom.us/oauth/token',
qs: {
grant_type: 'authorization_code',
code: 'YOUR_AUTHORIZATION_CODE',
redirect_uri: 'YOUR_REDIRECT_URI'
},
headers: {
Authorization: 'Basic ' + Buffer.from('YOUR_CLIENT_ID:YOUR_CLIENT_SECRET').toString('base64')
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
Replace YOUR_AUTHORIZATION_CODE
, YOUR_REDIRECT_URI
, YOUR_CLIENT_ID
, and YOUR_CLIENT_SECRET
with your actual values. This will return an access token that you can use for subsequent API calls.
With your Zoom developer account and OAuth app configured, you're now ready to start making API calls to retrieve meeting information using JavaScript.
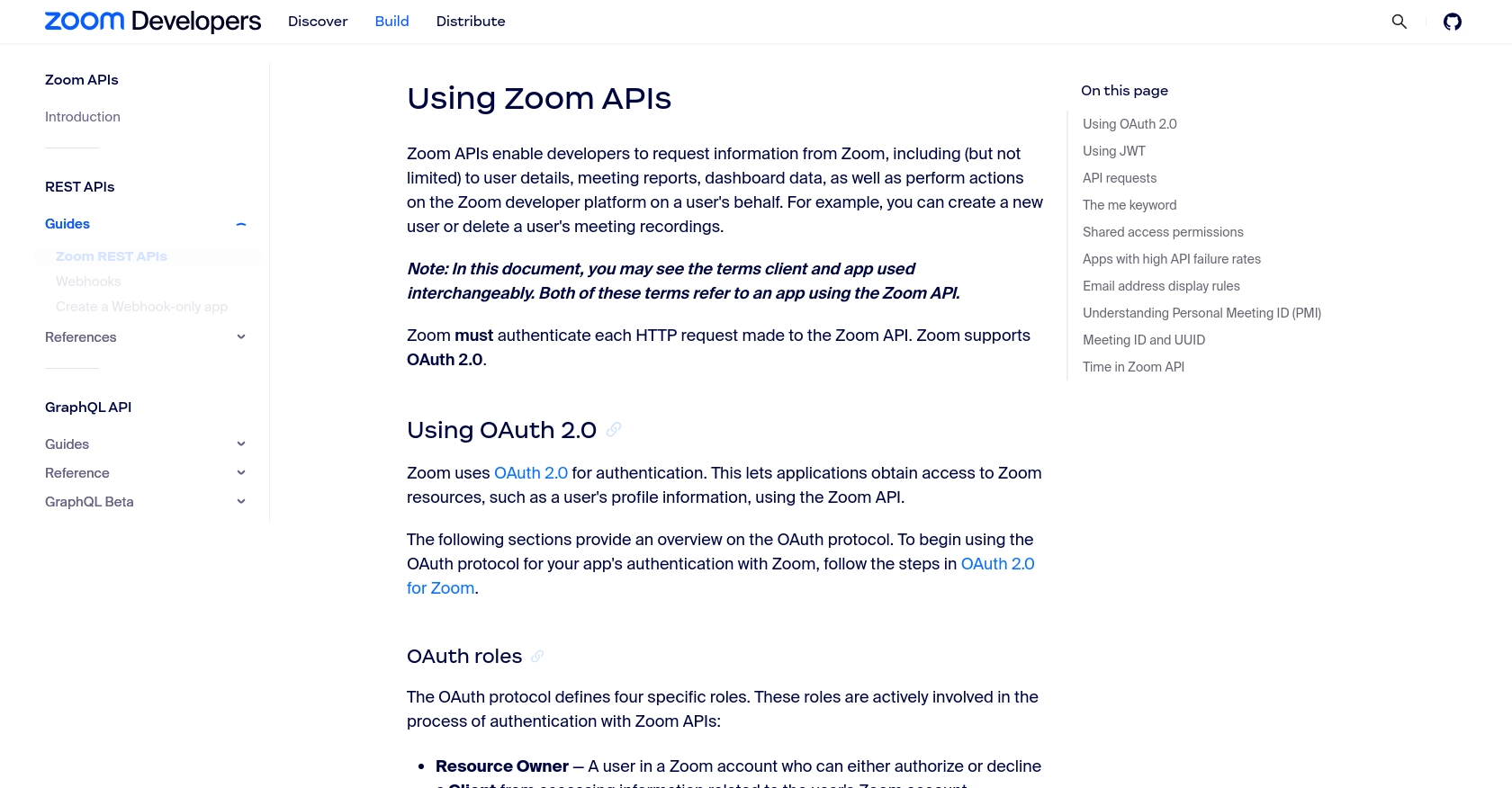
sbb-itb-96038d7
Making API Calls to Retrieve Zoom Meetings Using JavaScript
With your Zoom OAuth app set up and access token in hand, you can now proceed to make API calls to retrieve meeting information. This section will guide you through the process of using JavaScript to interact with the Zoom API and fetch meeting details.
Prerequisites for Zoom API Integration with JavaScript
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- The
request
library for making HTTP requests. Install it using the command:
npm install request
Fetching Zoom Meetings with JavaScript
To retrieve a list of meetings, you'll need to make a GET request to the Zoom API endpoint. Here's how you can do it using JavaScript:
var request = require('request');
var options = {
method: 'GET',
url: 'https://api.zoom.us/v2/users/me/meetings',
headers: {
authorization: 'Bearer YOUR_ACCESS_TOKEN'
},
qs: {
page_size: 30,
page_number: 1
}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
Replace YOUR_ACCESS_TOKEN
with the access token obtained from the OAuth process. This code will fetch a list of meetings associated with the authenticated user.
Understanding the API Response and Pagination
The API response will include meeting details such as meeting ID, topic, start time, and more. If there are multiple pages of results, use the next_page_token
to fetch additional pages:
var options = {
method: 'GET',
url: 'https://api.zoom.us/v2/users/me/meetings',
headers: {
authorization: 'Bearer YOUR_ACCESS_TOKEN'
},
qs: {
page_size: 30,
next_page_token: 'YOUR_NEXT_PAGE_TOKEN'
}
};
Ensure to handle pagination by checking for the next_page_token
in the response and using it in subsequent requests.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the HTTP status code and handle any errors appropriately. Common error codes include:
- 400 Bad Request: Invalid or missing data.
- 401 Unauthorized: Invalid or missing credentials.
- 403 Forbidden: Insufficient permissions.
- 429 Too Many Requests: Rate limit exceeded.
Refer to the Zoom API Error Definitions for more details on handling errors.
Verifying Retrieved Meeting Data in Zoom
After successfully retrieving meeting data, verify the results by cross-checking with your Zoom account. Ensure that the meeting details match the expected data in your Zoom dashboard.
By following these steps, you can efficiently retrieve and manage Zoom meetings using JavaScript, enhancing your application's functionality and user experience.
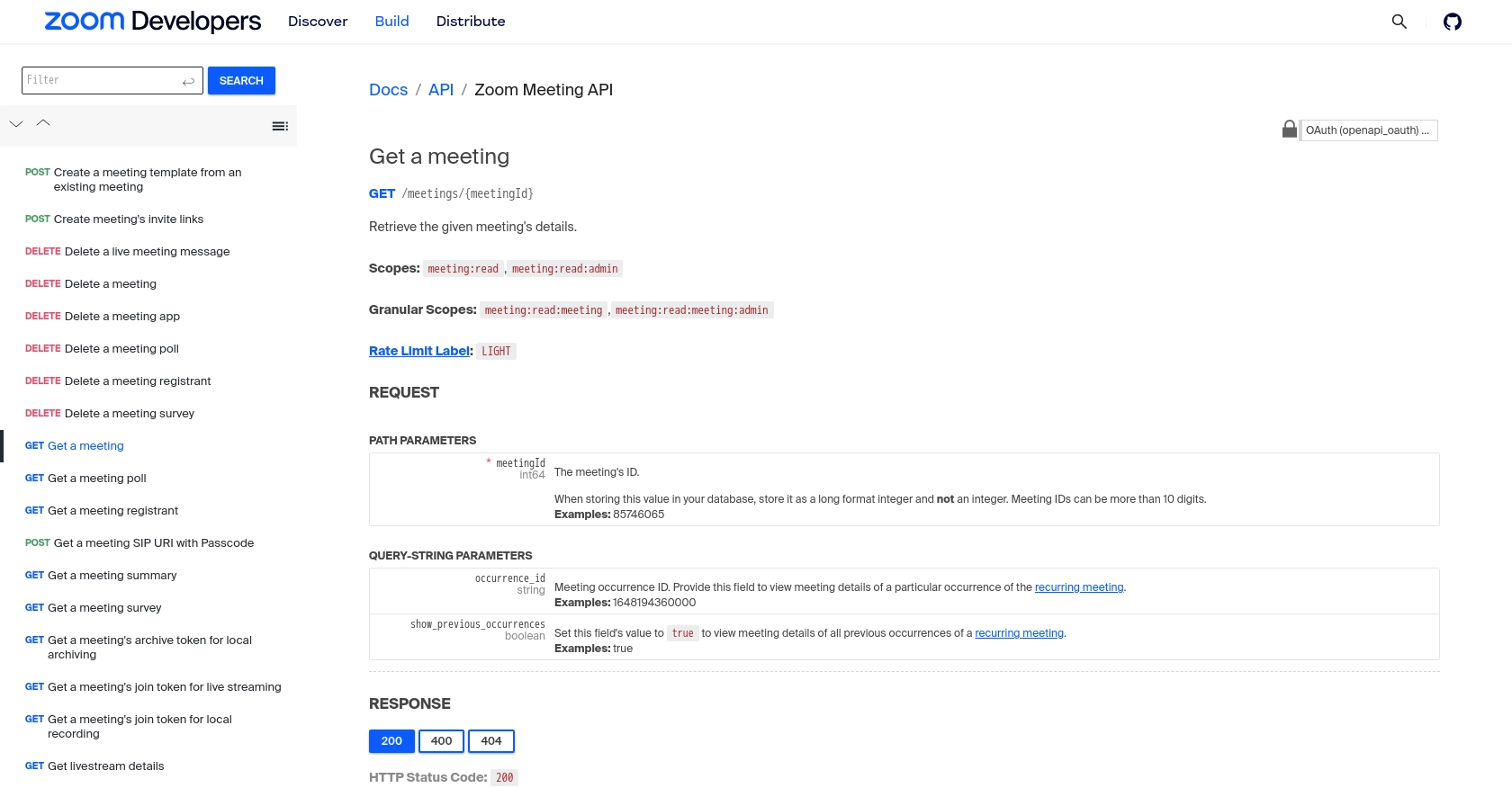
Conclusion and Best Practices for Zoom API Integration with JavaScript
Integrating with the Zoom API using JavaScript provides a powerful way to automate meeting management and enhance user experiences within your application. By following the steps outlined in this guide, you can efficiently retrieve and manage Zoom meetings, ensuring seamless communication and collaboration for your users.
Best Practices for Secure and Efficient Zoom API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Zoom's rate limits to avoid disruptions. Implement retry mechanisms and exponential backoff strategies when you encounter HTTP 429 errors. For more details, refer to the Zoom API Rate Limits documentation.
- Implement Error Handling: Ensure robust error handling by checking HTTP status codes and handling errors gracefully. Refer to the Zoom API Error Definitions for guidance on managing different error scenarios.
- Optimize Data Handling: Use pagination effectively to manage large datasets and reduce the load on your application. Utilize the
next_page_token
to fetch additional pages of data as needed.
Enhancing Your Integration Experience with Endgrate
While integrating with the Zoom API can significantly enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Zoom.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration process by visiting Endgrate.
Read More
- https://endgrate.com/provider/zoom
- https://developers.zoom.us/docs/api/rest/using-zoom-apis/
- https://developers.zoom.us/docs/api/rest/pagination/
- https://developers.zoom.us/docs/api/rest/error-definitions/
- https://developers.zoom.us/docs/api/rest/rate-limits/
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/meeting
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/pastMeetingParticipants
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/recordingsList
Ready to get started?