How to Create or Update Companies with the Copper API in PHP
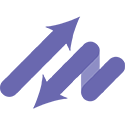
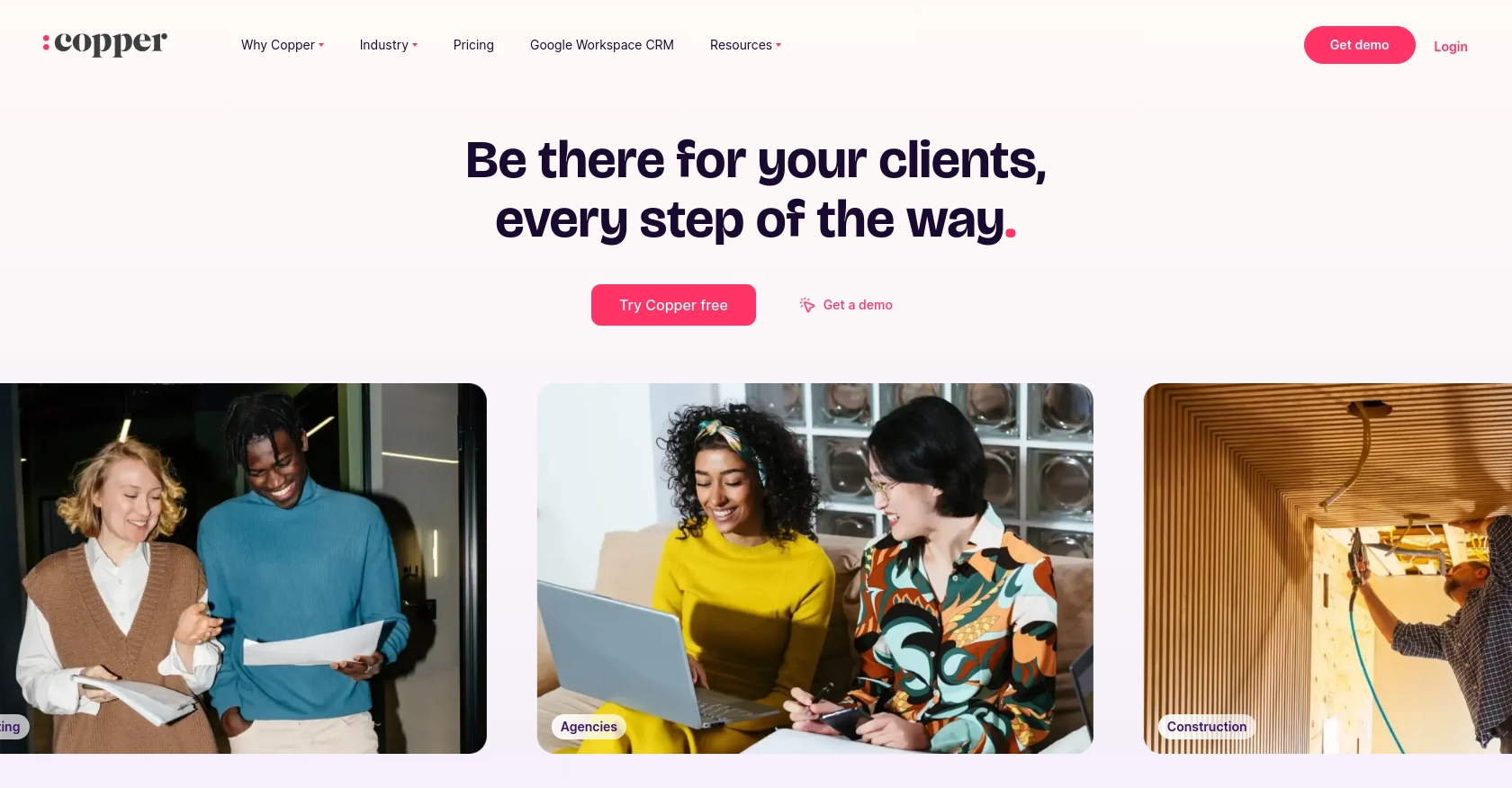
Introduction to Copper API for Company Management
Copper is a robust CRM platform tailored for businesses seeking to enhance their customer relationship management processes. It seamlessly integrates with Google Workspace, offering tools for managing contacts, leads, and sales pipelines directly within Gmail and other Google apps.
Developers often turn to Copper's API to automate and streamline business operations. By integrating with the Copper API, developers can efficiently manage company data, such as creating or updating company records. This capability is particularly useful for businesses that need to synchronize data between Copper and other platforms, ensuring that company information is always up-to-date and consistent across systems.
For example, a developer might use the Copper API to automatically update company details in Copper whenever changes occur in an external database, reducing manual data entry and minimizing errors.
Setting Up a Copper Test or Sandbox Account for API Integration
Before you can begin integrating with the Copper API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls and manage company data without affecting your live data.
Creating a Copper Account
If you don't already have a Copper account, you can sign up for a free trial on the Copper website. This trial will give you access to all the features necessary for testing API integrations.
- Visit the Copper website and click on the "Try Free" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Copper dashboard.
Generating API Keys for Copper Integration
To interact with the Copper API, you'll need to generate API keys. These keys will authenticate your requests and allow you to perform operations such as creating or updating company records.
- Navigate to the "Settings" section in your Copper dashboard.
- Select "API Keys" from the menu.
- Click on "Generate API Key" and provide a name for your key.
- Copy the generated API key and secret. Store them securely, as you'll need them for authentication in your PHP code.
Configuring OAuth for Secure Access
Copper uses OAuth 2.0 for secure API access. Follow these steps to configure OAuth:
- In the "API Keys" section, click on "Create OAuth App."
- Fill in the required fields, such as the application name and redirect URI.
- Save the app to receive your client ID and client secret.
- Use these credentials to authenticate your API requests.
Testing API Calls in the Copper Sandbox Environment
With your API keys and OAuth configuration in place, you can start testing API calls. Use the Copper sandbox environment to ensure your integration works as expected without impacting live data.
Refer to the Copper API documentation for detailed information on available endpoints and request formats.
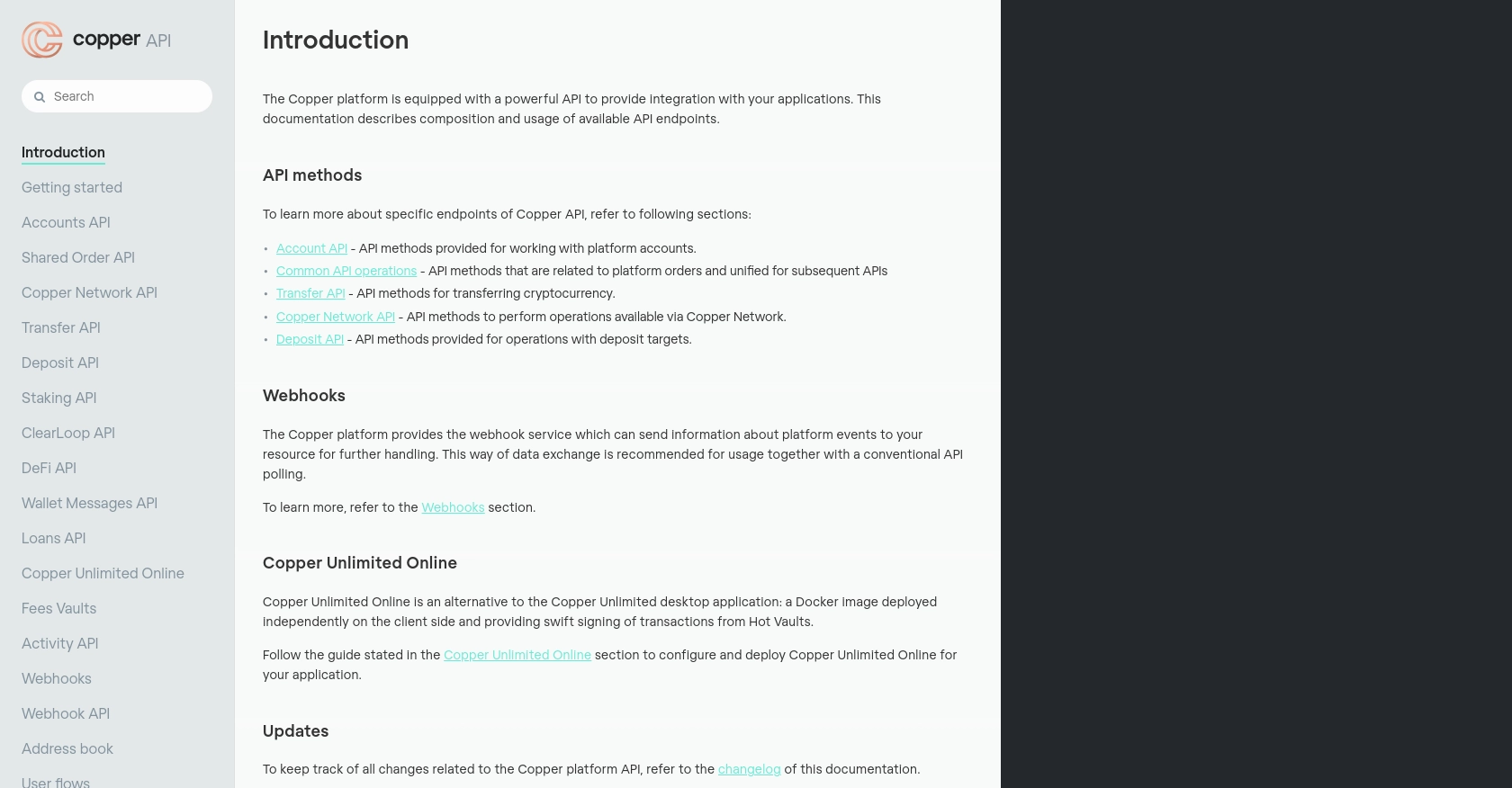
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Copper API in PHP
To interact with the Copper API for creating or updating company records, you'll need to set up your PHP environment and make authenticated API calls. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to perform the API operations.
Setting Up Your PHP Environment for Copper API Integration
Ensure you have PHP installed on your system. It's recommended to use PHP 7.4 or later for compatibility with modern libraries and security features.
- Download and install PHP from the official PHP website.
- Verify the installation by running
php -v
in your terminal.
Installing Required PHP Libraries for Copper API
You'll need the GuzzleHTTP
library to make HTTP requests to the Copper API. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Companies Using Copper API
With your environment set up, you can now write the PHP code to interact with the Copper API. Below is a sample script to create or update a company record:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'your_api_key';
$apiSecret = 'your_api_secret';
$timestamp = time() * 1000;
$method = 'POST';
$path = '/v1/companies';
$body = json_encode([
'name' => 'New Company',
'details' => 'Company details here'
]);
$signature = hash_hmac('sha256', $timestamp . $method . $path . $body, $apiSecret);
$response = $client->request($method, 'https://api.copper.co' . $path, [
'headers' => [
'Authorization' => 'ApiKey ' . $apiKey,
'X-Signature' => $signature,
'X-Timestamp' => $timestamp,
'Content-Type' => 'application/json'
],
'body' => $body
]);
echo $response->getBody();
Replace your_api_key
and your_api_secret
with the API key and secret you generated earlier. This script constructs a request to the Copper API to create or update a company, using the GuzzleHTTP
client for HTTP communication.
Verifying Successful API Requests in Copper
After executing your PHP script, verify that the company record was created or updated successfully by checking the Copper dashboard. If the request was successful, the new or updated company should appear in your Copper account.
Handling Errors and Common Copper API Error Codes
It's important to handle potential errors when making API calls. Copper API may return various error codes, such as:
- 400 Bad Request: Validation errors or missing required fields.
- 401 Unauthorized: Invalid API key or signature.
- 429 Too Many Requests: Rate limit exceeded.
Implement error handling in your PHP code to manage these responses and ensure robust integration.
Best Practices for Copper API Integration in PHP
When integrating with the Copper API, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Secure API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Copper API imposes rate limits to prevent abuse. Implement logic to handle HTTP 429 errors by retrying requests after a delay. This ensures your application remains compliant with Copper's usage policies.
- Data Transformation: Ensure that data fields are standardized and transformed as needed before sending requests to the Copper API. This helps maintain consistency across integrated systems.
- Error Handling: Implement comprehensive error handling to manage different response codes and error messages. This will help in diagnosing issues quickly and maintaining a robust integration.
Enhancing Integration Efficiency with Endgrate
While integrating with Copper API can be straightforward, managing multiple integrations can become complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Copper. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management, allowing your team to focus on core product development.
- Build once for each use case, reducing the need for multiple integrations for different platforms.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
Ready to get started?