How to Get People with the Copper API in PHP
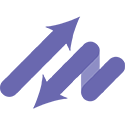
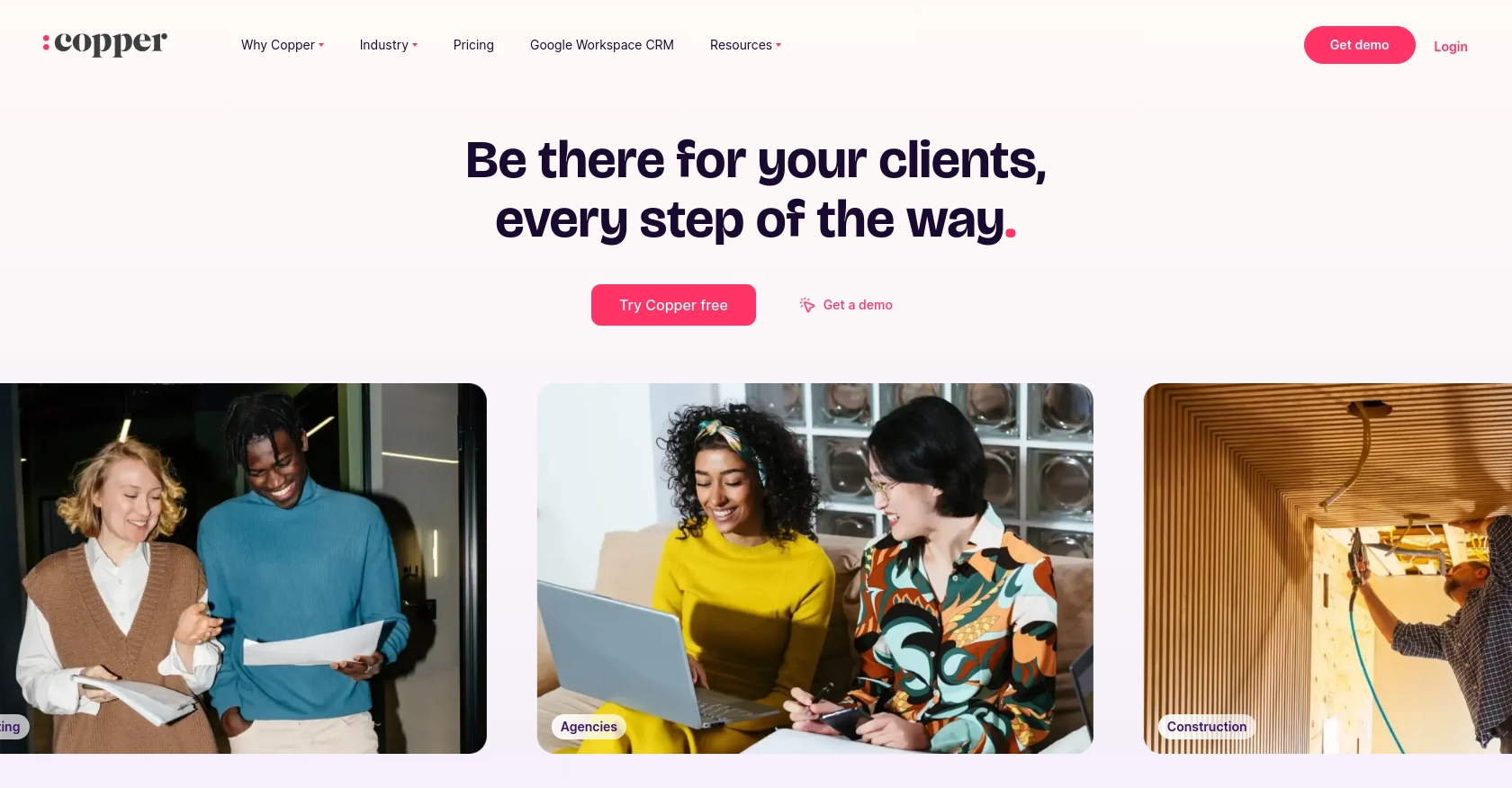
Introduction to Copper API
Copper is a comprehensive platform designed to streamline cryptocurrency management for businesses. It offers a robust API that allows developers to integrate and automate various financial operations, including trading, settlement, and asset management.
Connecting with Copper's API can significantly enhance a developer's ability to manage digital assets efficiently. For example, you might want to retrieve a list of people or contacts associated with your Copper account to facilitate better communication and transaction tracking.
This article will guide you through the process of using PHP to interact with the Copper API, specifically focusing on retrieving people-related data. By the end of this guide, you'll be equipped to integrate Copper's powerful features into your applications seamlessly.
Setting Up Your Copper API Test or Sandbox Account
Before you can start interacting with the Copper API using PHP, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Copper provides a demo environment specifically for this purpose.
Creating a Copper Demo Account
To begin, you'll need to create a Copper demo account. Follow these steps:
- Visit the Copper website and navigate to the demo environment at https://api.stage.copper.co.
- Sign up for a demo account by providing the necessary details. If you already have an account, simply log in.
- Once logged in, you will have access to the Copper platform's demo features, allowing you to test API interactions.
Generating Copper API Keys for Authentication
To authenticate your API requests, you'll need to generate API keys. Copper uses a custom authentication method involving an API key and a secret. Here’s how to obtain them:
- Navigate to the API settings within your Copper demo account.
- Generate a new API key. You will receive two strings: the API key and the API key secret.
- Store these credentials securely, as they will be used to authenticate your API requests.
Understanding Copper API Authentication Headers
When making API calls, you need to include specific headers for authentication:
- Authorization: Use the format
Authorization: ApiKey Your_API_Key
. - X-Timestamp: Include the current timestamp in milliseconds.
- X-Signature: Generate a signature using HMAC SHA256 with your API secret, timestamp, HTTP method, and request path.
Example of Creating a Signature in PHP
Here is an example of how to create a signature in PHP:
$apiKey = 'Your_API_Key';
$secret = 'Your_API_Secret';
$timestamp = round(microtime(true) * 1000);
$method = 'GET';
$path = '/platform/accounts';
$body = '';
$signature = hash_hmac('sha256', $timestamp . $method . $path . $body, $secret);
With your Copper demo account set up and your API keys generated, you are now ready to start making API calls to retrieve people-related data using PHP.
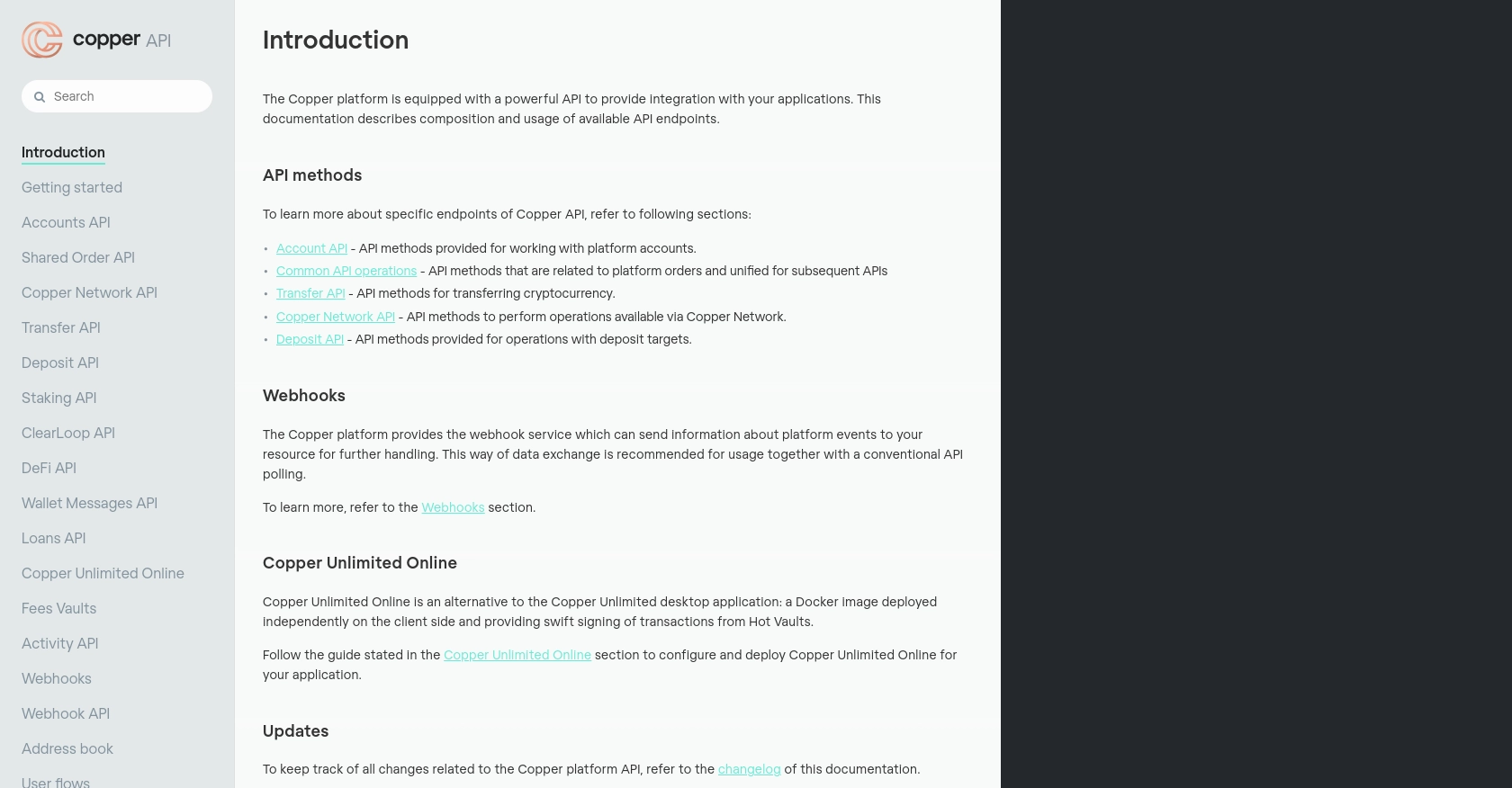
sbb-itb-96038d7
Making API Calls to Retrieve People Data with Copper API in PHP
To interact with the Copper API and retrieve people-related data, you'll need to set up your PHP environment and make authenticated API requests. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up PHP Environment for Copper API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Ensure PHP 7.4 or higher is installed on your system.
- Install Composer, the PHP package manager, to manage dependencies.
- Use Composer to install the
guzzlehttp/guzzle
package for making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Make Copper API Calls
With your environment set up, you can now write the PHP code to interact with the Copper API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key';
$secret = 'Your_API_Secret';
$timestamp = round(microtime(true) * 1000);
$method = 'GET';
$path = '/platform/people';
$body = '';
$signature = hash_hmac('sha256', $timestamp . $method . $path . $body, $secret);
$response = $client->request('GET', 'https://api.copper.co' . $path, [
'headers' => [
'Authorization' => 'ApiKey ' . $apiKey,
'X-Timestamp' => $timestamp,
'X-Signature' => $signature,
'Content-Type' => 'application/json'
]
]);
$data = json_decode($response->getBody(), true);
print_r($data);
Understanding the API Response and Handling Errors
After executing the API call, you should receive a response containing the people data. Here's how to handle the response and potential errors:
- Check the HTTP status code to ensure the request was successful (status code 200).
- If the request fails, handle errors by checking the status code and response message.
if ($response->getStatusCode() === 200) {
$people = json_decode($response->getBody(), true);
foreach ($people['data'] as $person) {
echo 'Name: ' . $person['name'] . "\n";
}
} else {
echo 'Error: ' . $response->getStatusCode() . ' - ' . $response->getReasonPhrase();
}
By following these steps, you can successfully retrieve and manage people-related data from Copper using PHP, enhancing your application's capabilities.
Conclusion: Best Practices for Integrating Copper API with PHP
Integrating the Copper API using PHP offers a powerful way to manage digital assets and streamline financial operations. By following the steps outlined in this guide, you can efficiently retrieve and manage people-related data, enhancing your application's functionality.
Best Practices for Secure and Efficient Copper API Integration
- Secure Storage of API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Copper's API rate limits. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Copper is transformed and standardized to fit your application's data model.
- Error Handling: Implement robust error handling to manage API call failures and unexpected responses. Log errors for monitoring and debugging purposes.
Call to Action: Simplify Your Integrations with Endgrate
While integrating with Copper API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration efforts and enhance your application's capabilities.
Read More
Ready to get started?