Using the Copper API to Create or Update Leads (with Python examples)
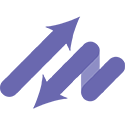
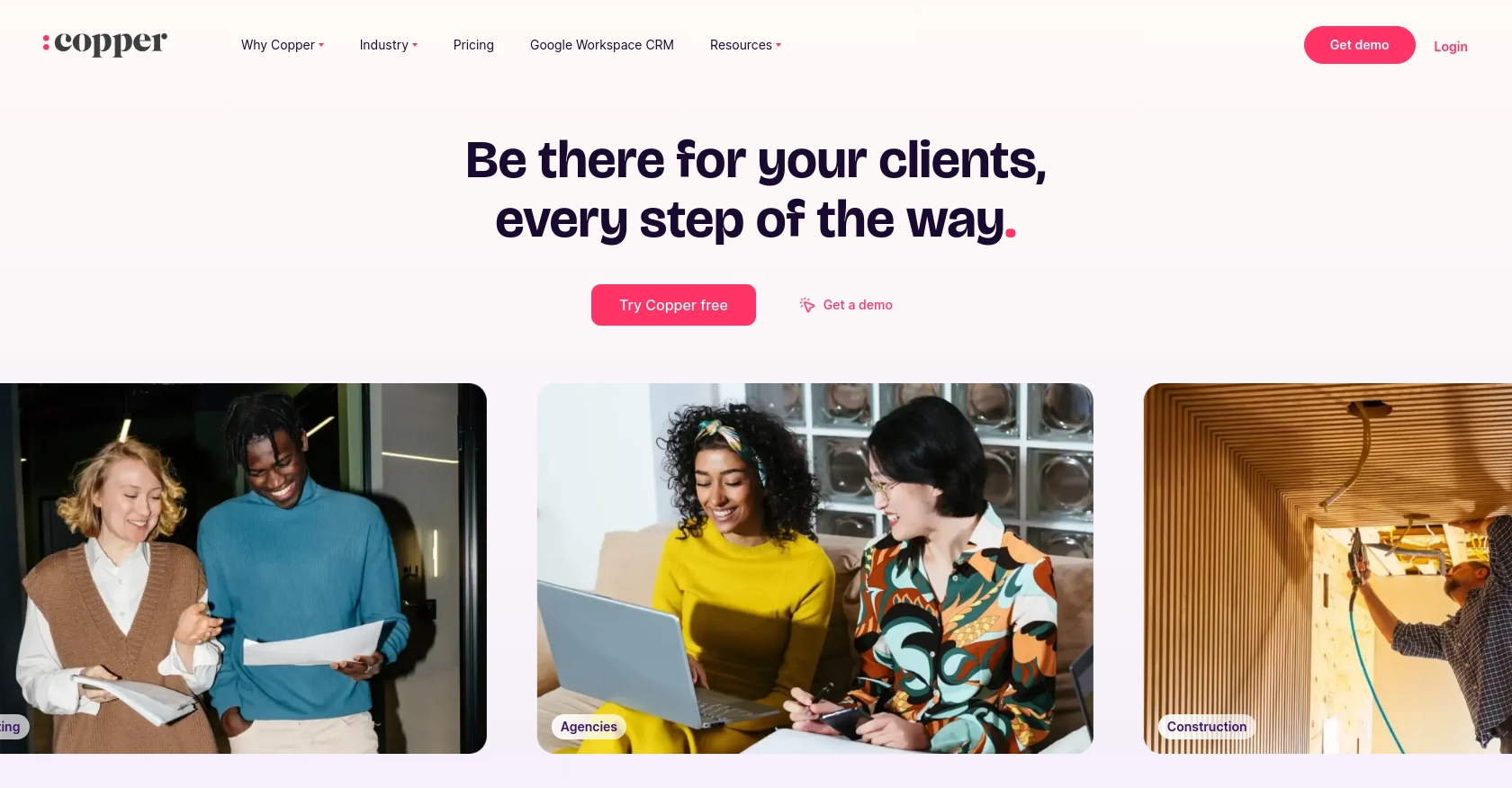
Introduction to Copper CRM API
Copper is a powerful CRM platform designed to seamlessly integrate with Google Workspace, providing businesses with an efficient way to manage customer relationships. Its user-friendly interface and robust features make it a popular choice for companies looking to enhance their sales and marketing efforts.
Developers may want to integrate with Copper's API to automate and streamline CRM processes, such as creating or updating leads. For example, you could use the Copper API to automatically update lead information from an external data source, ensuring your sales team always has the most current information at their fingertips.
Setting Up Your Copper CRM Test or Sandbox Account
Before you can start integrating with the Copper API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Copper CRM Account
- Visit the Copper website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Copper dashboard.
Generate Copper API Key and Secret
To interact with the Copper API, you'll need an API key and secret. Here's how to generate them:
- Navigate to the settings menu in the Copper dashboard.
- Locate the API section and click on "Generate API Key."
- Copy the API key and secret provided. Store them securely as you'll need them for authentication.
Configure Copper API Authentication
Copper uses a custom authentication method. To authenticate your API requests, you'll need to include the API key and secret in your request headers. Here's an example of how to set up authentication in Python:
import hashlib
import hmac
import requests
import time
api_key = 'your_api_key'
api_secret = 'your_api_secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/api/v1/leads"
body = ""
signature = hmac.new(
key=bytes(api_secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
headers = {
'Authorization': f'ApiKey {api_key}',
'X-Signature': signature,
'X-Timestamp': timestamp
}
response = requests.get(f'https://api.copper.co{path}', headers=headers)
print(response.json())
Ensure you replace your_api_key
and your_api_secret
with the actual values you generated earlier.
Verify Copper API Access
To confirm that your setup is correct, make a simple API call to retrieve leads:
response = requests.get(f'https://api.copper.co{path}', headers=headers)
if response.status_code == 200:
print("API access verified. Leads retrieved successfully.")
else:
print("Failed to verify API access. Check your credentials and try again.")
By following these steps, you will have successfully set up your Copper CRM test or sandbox account, allowing you to proceed with API integration and development.
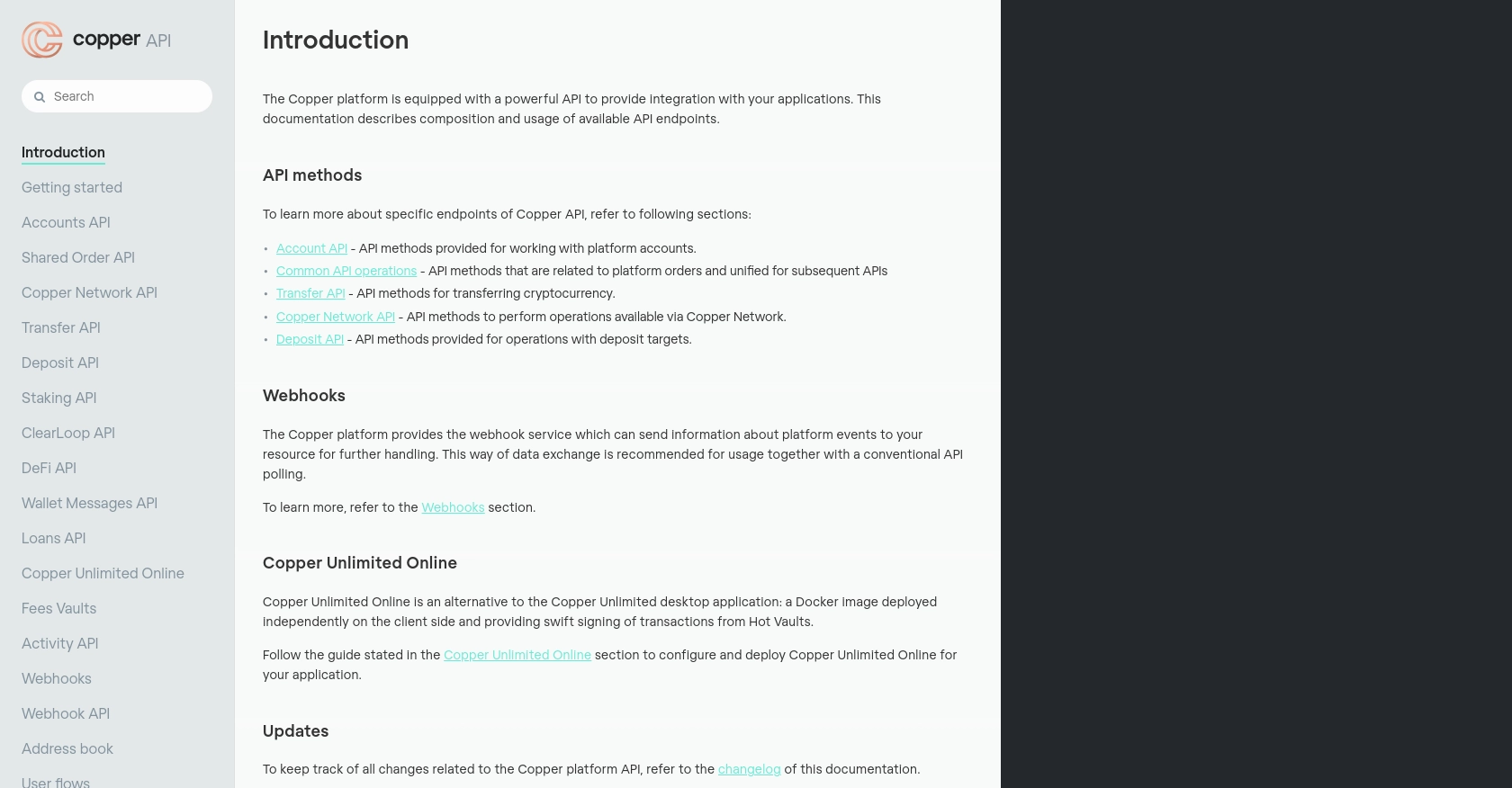
sbb-itb-96038d7
Making API Calls to Copper CRM with Python
To effectively interact with the Copper API, you'll need to understand how to make API calls using Python. This section will guide you through the process of creating and updating leads using Copper's API.
Setting Up Your Python Environment for Copper API Integration
Before making API calls, ensure you have Python installed on your machine. We recommend using Python 3.6 or later. Additionally, you'll need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Creating Leads in Copper CRM Using Python
To create a new lead in Copper, you'll need to send a POST request to the Copper API endpoint. Here's an example of how to do this:
import hashlib
import hmac
import requests
import time
api_key = 'your_api_key'
api_secret = 'your_api_secret'
timestamp = str(round(time.time() * 1000))
method = "POST"
path = "/api/v1/leads"
body = '{"name": "New Lead", "email": "lead@example.com"}'
signature = hmac.new(
key=bytes(api_secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
headers = {
'Authorization': f'ApiKey {api_key}',
'X-Signature': signature,
'X-Timestamp': timestamp,
'Content-Type': 'application/json'
}
response = requests.post(f'https://api.copper.co{path}', headers=headers, data=body)
print(response.json())
Replace your_api_key
and your_api_secret
with your actual API credentials. The body
variable contains the lead information you want to create.
Updating Leads in Copper CRM Using Python
To update an existing lead, you'll need to know the lead's ID and send a PUT request. Here's how you can update a lead's information:
lead_id = 'existing_lead_id'
path = f"/api/v1/leads/{lead_id}"
body = '{"name": "Updated Lead Name", "email": "updated@example.com"}'
signature = hmac.new(
key=bytes(api_secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
headers['X-Signature'] = signature
response = requests.put(f'https://api.copper.co{path}', headers=headers, data=body)
print(response.json())
Ensure you replace existing_lead_id
with the actual ID of the lead you wish to update.
Handling API Response and Errors
After making an API call, it's crucial to handle the response correctly. Check the status code to verify if the request was successful:
if response.status_code == 200:
print("Lead processed successfully:", response.json())
else:
print("Error processing lead:", response.status_code, response.json())
By following these steps, you can efficiently create and update leads in Copper CRM using Python, ensuring your CRM data is always up-to-date.
Best Practices for Copper API Integration
When integrating with the Copper API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Secure API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code and consider using environment variables or a secure vault.
- Handle Rate Limiting: Copper API has rate limits to prevent abuse. Ensure your application handles HTTP 429 errors gracefully by implementing retry logic with exponential backoff.
- Validate API Responses: Always check the status code and response body for errors. Implement error handling to manage different HTTP status codes effectively.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application and Copper CRM.
Enhance Your Integration with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Copper. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your product offerings.
Read More
Ready to get started?